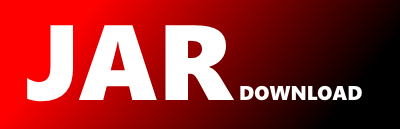
org.aksw.combinatorics.algos.StateProblemContainer Maven / Gradle / Ivy
The newest version!
package org.aksw.combinatorics.algos;
import java.util.function.BinaryOperator;
import java.util.function.Predicate;
import java.util.stream.Stream;
import org.aksw.combinatorics.solvers.GenericProblem;
import org.aksw.combinatorics.solvers.Problem;
import org.aksw.combinatorics.solvers.collections.ProblemContainer;
import org.aksw.combinatorics.solvers.collections.ProblemContainerPick;
import org.aksw.isomorphism.ActionProblemContainer;
import org.aksw.state_space_search.core.Action;
import org.aksw.state_space_search.core.State;
/**
* Class that wraps a {@link Problem} with the {@link State} interface.
*
* @author raven
*
* @param
*/
public class StateProblemContainer
implements State
{
protected ProblemContainer problemContainer;
protected Predicate isUnsolveable;
protected S baseSolution;
protected BinaryOperator solutionCombiner;
public StateProblemContainer(S baseSolution, Predicate isUnsolveable, ProblemContainer problemContainer, BinaryOperator solutionCombiner) {
super();
this.baseSolution = baseSolution;
this.problemContainer = problemContainer;
this.solutionCombiner = solutionCombiner;
}
@Override
public boolean isFinal() {
boolean result = problemContainer.isEmpty() || isUnsolveable.test(baseSolution);
return result;
}
@Override
public S getSolution() {
return baseSolution;
}
/**
* Actions are created by means of first solving the cheapest open problem
* generating an action for each obtained solution.
* (assumes a non-final state)
*
*
*/
@Override
public Stream> getActions() {
ProblemContainerPick pick = problemContainer.pick();
GenericProblem picked = pick.getPicked();
ProblemContainer remaining = pick.getRemaining();
Stream> result = picked
.generateSolutions()
.map(solutionContribution -> {
S partialSolution = solutionCombiner.apply(baseSolution, solutionContribution);
Action r;
//Stream> r;
// If the partial solution is null, then indicate the
// absence of a solution by returning a stream that yields
// null as a 'solution'
if (partialSolution == null) {
//r = Collections. singleton(null).stream();
r = null;
} else {
// This step is optional: it refines problems
// based on the current partial solution
// Depending on your setting, this can give a
// performance boost or penalty
//ProblemContainerImpl openProblems = remaining;
ProblemContainer openProblems = remaining.refine(partialSolution);
r = new ActionProblemContainer(partialSolution, isUnsolveable, openProblems, solutionCombiner);
//
// if (openProblems.isEmpty()) {
// r = Collections. emptySet().stream();
// } else {
// r =
// //ProblemSolver nextState = new ProblemSolver(openProblems, baseSolution, solutionCombiner);
// //r = nextState.streamSolutions();
// }
}
// Stream> s = r.map(x -> ); //(partialSolution, partialSolution));
return r;
})
;
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy