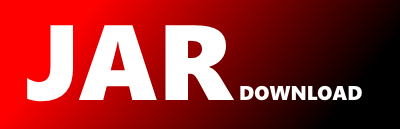
org.aksw.sparqlify.database.PatriciaPrefixMapStoreAccessor Maven / Gradle / Ivy
package org.aksw.sparqlify.database;
//public class PatriciaPrefixMapStoreAccessor
// implements MapStoreAccessor
//{
// public static Set> supportedConstraintClasses = new HashSet>();
//
// static
// {
// supportedConstraintClasses.add(EqualsConstraint.class);
// supportedConstraintClasses.add(StartsWithConstraint.class);
// supportedConstraintClasses.add(IsPrefixOfConstraint.class);
// }
//
// public Set> getSupportedConstraintClasses() {
// return supportedConstraintClasses;
// }
//
//
// private int indexColumn;
// private Transformer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy