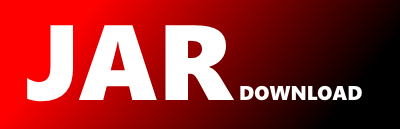
org.aksw.jenax.graphql.sparql.AsyncCombiner Maven / Gradle / Ivy
package org.aksw.jenax.graphql.sparql;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutorService;
import java.util.function.Function;
import java.util.stream.Collectors;
import com.google.common.util.concurrent.Futures;
import com.google.common.util.concurrent.ListenableFuture;
import com.google.common.util.concurrent.ListeningExecutorService;
import com.google.common.util.concurrent.MoreExecutors;
/**
* Assemble a result from a collection of async contributions.
* A small wrapper around guava's Futures API.
*
* Example:
*
* {@code
* ListenableFuture future =
* AsyncCombiner.of(executorService, combineXsIntoY)
* .addTask(a).addTask(b)
* .exec() }
*
*/
public class AsyncCombiner {
protected ListeningExecutorService executorService;
protected Function, O> combiner;
protected List> tasks = new ArrayList<>();
protected AsyncCombiner(ListeningExecutorService executorService, Function, O> combiner) {
super();
this.executorService = Objects.requireNonNull(executorService, "executorService must not be null");
this.combiner = Objects.requireNonNull(combiner, "combiner must not be null");
}
public static AsyncCombiner of(ExecutorService executorService, Function, O> combiner) {
return of (MoreExecutors.listeningDecorator(executorService), combiner);
}
public static AsyncCombiner of(ListeningExecutorService executorService, Function, O> combiner) {
return new AsyncCombiner<>(executorService, combiner);
}
public AsyncCombiner addTask(Callable task) {
tasks.add(task);
return this;
}
public ListenableFuture exec() {
List> futures = tasks.stream()
.map(executorService::submit).collect(Collectors.toList());
ListenableFuture result = Futures.transform(
Futures.allAsList(futures), combiner::apply, executorService);
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy