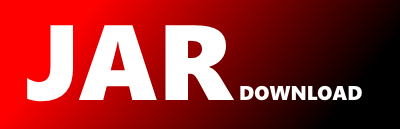
org.alfasoftware.astracli.commandline.AstraMethodInvocation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of astra-cli Show documentation
Show all versions of astra-cli Show documentation
Astra CLI provides a command line interface for interacting with Astra, a Java tool for analysing and refactoring Java source code.
package org.alfasoftware.astracli.commandline;
import java.io.File;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import java.util.stream.Collectors;
import org.alfasoftware.astra.core.matchers.MethodMatcher;
import org.alfasoftware.astra.core.refactoring.UseCase;
import org.alfasoftware.astra.core.refactoring.operations.methods.MethodInvocationRefactor;
import org.alfasoftware.astra.core.utils.ASTOperation;
import org.alfasoftware.astra.core.utils.AstraCore;
import org.apache.log4j.Logger;
import picocli.CommandLine;
@CommandLine.Command(name = "method",
sortOptions = false,
headerHeading = "@|bold,underline Usage:|@%n%n",
synopsisHeading = "%n",
descriptionHeading = "%n@|bold,underline Description:|@%n%n",
parameterListHeading = "%n@|bold,underline Parameters:|@%n",
optionListHeading = "%n@|bold,underline Options:|@%n",
header = "Refactor method invocations.",
description = "Finds method invocations matching given criteria, and performs a refactoring.")
class AstraMethodInvocation implements Runnable {
private static final Logger log = Logger.getLogger(AstraMethodInvocation.class);
@CommandLine.Option(
names = {"-t", "--fqType"},
required = true,
paramLabel = "",
description = "Fully qualified type of the method to match.")
String fqType;
@CommandLine.Option(
names = {"-n", "--name"},
required = true,
paramLabel = "",
description = "Name of the method to match.")
String name;
@CommandLine.Option(
names = {"-p", "--parameters"},
required = true,
paramLabel = "",
description = "Parameters of the method to match.")
List parameters = new ArrayList<>();
@CommandLine.Option(
names = {"-v", "--isVarargs"},
paramLabel = "",
description = "Whether the method to match is varargs or not.")
Boolean isVarargs;
@CommandLine.Option(
names = {"-nn", "--newname"},
paramLabel = "",
description = "New name for the method.")
String newName;
@CommandLine.Option(
names = {"-nt", "--newfqtype"},
paramLabel = "",
description = "New fully qualified type for the method.")
String newFQType;
@CommandLine.Option(
names = {"-d", "--dir"},
required = true,
description = "Set the path to the code checkout")
File directory;
@CommandLine.Option(
names = "--cp",
required = true,
description = "Set the path to the additional jar files. At least the jar containing the 'before' type should be specified.",
split = "[,;]")
File[] classpath;
@Override
public void run() {
MethodMatcher.Builder methodbuilder = MethodMatcher.builder()
.withFullyQualifiedDeclaringType(fqType)
.withMethodName(name)
.withFullyQualifiedParameters(parameters);
if (isVarargs != null) {
methodbuilder = methodbuilder.isVarargs(isVarargs);
}
log.info("Starting [method] refactor: [" + methodbuilder.build() + "]");
MethodInvocationRefactor.Changes changes = new MethodInvocationRefactor.Changes();
if (newName != null) {
changes = changes.toNewMethodName(newName);
}
if (newFQType != null) {
changes = changes.toNewType(newFQType);
}
performMethodRefactor(methodbuilder, changes);
}
private void performMethodRefactor(MethodMatcher.Builder methodbuilder, MethodInvocationRefactor.Changes changes) {
AstraCore.run(directory.getAbsolutePath(), new UseCase() {
@Override
public Set extends ASTOperation> getOperations() {
return new HashSet<>(Collections.singletonList(
MethodInvocationRefactor
.from(methodbuilder.build())
.to(changes)));
}
@Override
public Set getAdditionalClassPathEntries() {
return Arrays.asList(classpath).stream().map(File::getAbsolutePath).collect(Collectors.toSet());
}
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy