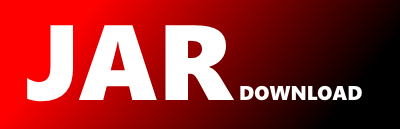
com.tinkerpop.blueprints.impls.neo4j2.Neo4j2Element Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of blueprints-neo4j2-graph Show documentation
Show all versions of blueprints-neo4j2-graph Show documentation
Blueprints property graph implementation for the Neo4j 2 graph database
The newest version!
package com.tinkerpop.blueprints.impls.neo4j2;
import com.tinkerpop.blueprints.Edge;
import com.tinkerpop.blueprints.Element;
import com.tinkerpop.blueprints.Vertex;
import com.tinkerpop.blueprints.util.ElementHelper;
import org.neo4j.graphdb.Node;
import org.neo4j.graphdb.PropertyContainer;
import org.neo4j.graphdb.Relationship;
import java.lang.reflect.Array;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashSet;
import java.util.Iterator;
import java.util.Set;
/**
* @author Marko A. Rodriguez (http://markorodriguez.com)
*/
abstract class Neo4j2Element implements Element {
protected final Neo4j2Graph graph;
protected PropertyContainer rawElement;
public Neo4j2Element(final Neo4j2Graph graph) {
this.graph = graph;
}
public T getProperty(final String key) {
this.graph.autoStartTransaction(false);
if (this.rawElement.hasProperty(key))
return (T) tryConvertCollectionToArrayList(this.rawElement.getProperty(key));
else
return null;
}
public void setProperty(final String key, final Object value) {
ElementHelper.validateProperty(this, key, value);
this.graph.autoStartTransaction(true);
// attempts to take a collection and convert it to an array so that Neo4j can consume it
this.rawElement.setProperty(key, tryConvertCollectionToArray(value));
}
public T removeProperty(final String key) {
if (!this.rawElement.hasProperty(key))
return null;
else {
this.graph.autoStartTransaction(true);
return (T) this.rawElement.removeProperty(key);
}
}
public Set getPropertyKeys() {
this.graph.autoStartTransaction(false);
final Set keys = new HashSet();
for (final String key : this.rawElement.getPropertyKeys()) {
keys.add(key);
}
return keys;
}
public int hashCode() {
return this.getId().hashCode();
}
public PropertyContainer getRawElement() {
return this.rawElement;
}
public Object getId() {
this.graph.autoStartTransaction(false);
if (this.rawElement instanceof Node) {
return ((Node) this.rawElement).getId();
} else {
return ((Relationship) this.rawElement).getId();
}
}
public void remove() {
if (this instanceof Vertex)
this.graph.removeVertex((Vertex) this);
else
this.graph.removeEdge((Edge) this);
}
public boolean equals(final Object object) {
return ElementHelper.areEqual(this, object);
}
private Object tryConvertCollectionToArray(final Object value) {
if (value instanceof Collection>) {
// convert this collection to an array. the collection must
// be all of the same type.
try {
final Collection> collection = (Collection>) value;
Object[] array = null;
final Iterator> objects = collection.iterator();
for (int i = 0; objects.hasNext(); i++) {
Object object = objects.next();
if (array == null) {
array = (Object[]) Array.newInstance(object.getClass(), collection.size());
}
array[i] = object;
}
return array;
} catch (final ArrayStoreException ase) {
// this fires off if the collection is not all of the same type
return value;
}
} else {
return value;
}
}
private Object tryConvertCollectionToArrayList(final Object value) {
if (value.getClass().isArray()) {
// convert primitive array to an ArrayList.
try {
ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy