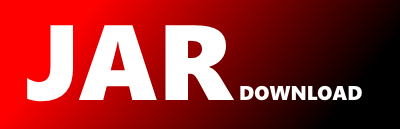
com.tinkerpop.blueprints.impls.neo4j2.batch.Neo4j2BatchEdge Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of blueprints-neo4j2-graph Show documentation
Show all versions of blueprints-neo4j2-graph Show documentation
Blueprints property graph implementation for the Neo4j 2 graph database
The newest version!
package com.tinkerpop.blueprints.impls.neo4j2.batch;
import com.tinkerpop.blueprints.Direction;
import com.tinkerpop.blueprints.Edge;
import com.tinkerpop.blueprints.Vertex;
import com.tinkerpop.blueprints.util.ExceptionFactory;
import com.tinkerpop.blueprints.util.StringFactory;
import java.util.Map;
/**
* @author Marko A. Rodriguez (http://markorodriguez.com)
*/
class Neo4j2BatchEdge extends Neo4j2BatchElement implements Edge {
private final String label;
public Neo4j2BatchEdge(final Neo4j2BatchGraph graph, final Long id, final String label) {
super(graph, id);
this.label = label;
}
public T removeProperty(final String key) {
final Map properties = this.getPropertyMapClone();
final Object value = properties.remove(key);
this.graph.getRawGraph().setRelationshipProperties(this.id, properties);
return (T) value;
}
public void setProperty(final String key, final Object value) {
if (key.isEmpty())
throw ExceptionFactory.propertyKeyCanNotBeEmpty();
if (key.equals(StringFactory.ID))
throw ExceptionFactory.propertyKeyIdIsReserved();
if (key.equals(StringFactory.LABEL))
throw ExceptionFactory.propertyKeyLabelIsReservedForEdges();
final Map properties = this.getPropertyMapClone();
properties.put(key, value);
this.graph.getRawGraph().setRelationshipProperties(this.id, properties);
}
public Map getPropertyMap() {
return this.graph.getRawGraph().getRelationshipProperties(this.id);
}
/**
* @throws UnsupportedOperationException
*/
public Vertex getVertex(final Direction direction) throws UnsupportedOperationException {
throw new UnsupportedOperationException();
}
public String getLabel() {
return this.label;
}
public String toString() {
return "e[" + this.id + "][?-" + this.label + "->?]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy