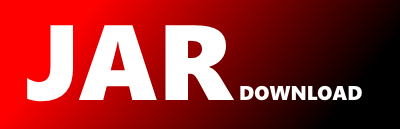
org.allurefw.report.entity.Statistic Maven / Gradle / Ivy
The newest version!
package org.allurefw.report.entity;
import java.io.Serializable;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for Statistic complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Statistic">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="passed" type="{http://www.w3.org/2001/XMLSchema}long" default="0" />
* <attribute name="pending" type="{http://www.w3.org/2001/XMLSchema}long" default="0" />
* <attribute name="canceled" type="{http://www.w3.org/2001/XMLSchema}long" default="0" />
* <attribute name="failed" type="{http://www.w3.org/2001/XMLSchema}long" default="0" />
* <attribute name="broken" type="{http://www.w3.org/2001/XMLSchema}long" default="0" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Statistic")
public class Statistic implements Serializable, ExtraStatisticMethods
{
private final static long serialVersionUID = 1L;
@XmlAttribute(name = "passed")
protected Long passed;
@XmlAttribute(name = "pending")
protected Long pending;
@XmlAttribute(name = "canceled")
protected Long canceled;
@XmlAttribute(name = "failed")
protected Long failed;
@XmlAttribute(name = "broken")
protected Long broken;
/**
* Gets the value of the passed property.
*
* @return
* possible object is
* {@link Long }
*
*/
public long getPassed() {
if (passed == null) {
return 0L;
} else {
return passed;
}
}
/**
* Sets the value of the passed property.
*
* @param value
* allowed object is
* {@link Long }
*
*/
public void setPassed(Long value) {
this.passed = value;
}
/**
* Gets the value of the pending property.
*
* @return
* possible object is
* {@link Long }
*
*/
public long getPending() {
if (pending == null) {
return 0L;
} else {
return pending;
}
}
/**
* Sets the value of the pending property.
*
* @param value
* allowed object is
* {@link Long }
*
*/
public void setPending(Long value) {
this.pending = value;
}
/**
* Gets the value of the canceled property.
*
* @return
* possible object is
* {@link Long }
*
*/
public long getCanceled() {
if (canceled == null) {
return 0L;
} else {
return canceled;
}
}
/**
* Sets the value of the canceled property.
*
* @param value
* allowed object is
* {@link Long }
*
*/
public void setCanceled(Long value) {
this.canceled = value;
}
/**
* Gets the value of the failed property.
*
* @return
* possible object is
* {@link Long }
*
*/
public long getFailed() {
if (failed == null) {
return 0L;
} else {
return failed;
}
}
/**
* Sets the value of the failed property.
*
* @param value
* allowed object is
* {@link Long }
*
*/
public void setFailed(Long value) {
this.failed = value;
}
/**
* Gets the value of the broken property.
*
* @return
* possible object is
* {@link Long }
*
*/
public long getBroken() {
if (broken == null) {
return 0L;
} else {
return broken;
}
}
/**
* Sets the value of the broken property.
*
* @param value
* allowed object is
* {@link Long }
*
*/
public void setBroken(Long value) {
this.broken = value;
}
public Statistic withPassed(Long value) {
setPassed(value);
return this;
}
public Statistic withPending(Long value) {
setPending(value);
return this;
}
public Statistic withCanceled(Long value) {
setCanceled(value);
return this;
}
public Statistic withFailed(Long value) {
setFailed(value);
return this;
}
public Statistic withBroken(Long value) {
setBroken(value);
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy