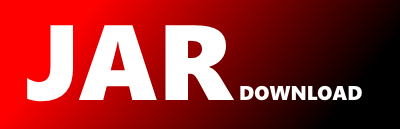
alluxio.cli.ValidateEnv Maven / Gradle / Ivy
/*
* The Alluxio Open Foundation licenses this work under the Apache License, version 2.0
* (the "License"). You may not use this work except in compliance with the License, which is
* available at www.apache.org/licenses/LICENSE-2.0
*
* This software is distributed on an "AS IS" basis, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied, as more fully set forth in the License.
*
* See the NOTICE file distributed with this work for information regarding copyright ownership.
*/
package alluxio.cli;
import alluxio.Constants;
import alluxio.cli.hdfs.HdfsConfParityValidationTask;
import alluxio.cli.hdfs.HdfsConfValidationTask;
import alluxio.cli.hdfs.HdfsProxyUserValidationTask;
import alluxio.cli.hdfs.HdfsVersionValidationTask;
import alluxio.cli.hdfs.SecureHdfsValidationTask;
import alluxio.conf.AlluxioConfiguration;
import alluxio.conf.Configuration;
import alluxio.conf.PropertyKey;
import alluxio.exception.status.InvalidArgumentException;
import alluxio.util.CommonUtils;
import alluxio.util.ConfigurationUtils;
import alluxio.util.network.NetworkAddressUtils.ServiceType;
import org.apache.commons.cli.CommandLine;
import org.apache.commons.cli.CommandLineParser;
import org.apache.commons.cli.DefaultParser;
import org.apache.commons.cli.HelpFormatter;
import org.apache.commons.cli.Option;
import org.apache.commons.cli.Options;
import org.apache.commons.cli.ParseException;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.TreeMap;
/**
* Utility for checking Alluxio environment.
*/
// TODO(yanqin): decouple ValidationTask implementations for easier dependency management
public final class ValidateEnv {
private static final String USAGE = "validateEnv COMMAND [NAME] [OPTIONS]\n\n"
+ "Validate environment for Alluxio.\n\n"
+ "COMMAND can be one of the following values:\n"
+ "local: run all validation tasks on local\n"
+ "master: run master validation tasks on local\n"
+ "worker: run worker validation tasks on local\n"
+ "all: run corresponding validation tasks on all master nodes and worker nodes\n"
+ "masters: run master validation tasks on all master nodes\n"
+ "workers: run worker validation tasks on all worker nodes\n\n"
+ "list: list all validation tasks\n\n"
+ "For all commands except list:\n"
+ "NAME can be any task full name or prefix.\n"
+ "When NAME is given, only tasks with name starts with the prefix will run.\n"
+ "For example, specifying NAME \"master\" or \"ma\" will run both tasks named "
+ "\"master.rpc.port.available\" and \"master.web.port.available\" but not "
+ "\"worker.rpc.port.available\".\n"
+ "If NAME is not given, all tasks for the given TARGET will run.\n\n"
+ "OPTIONS can be a list of command line options. Each option has the"
+ " format \"- [optionValue]\"\n";
private static final String ALLUXIO_MASTER_CLASS = "alluxio.master.AlluxioMaster";
private static final String ALLUXIO_WORKER_CLASS = "alluxio.worker.AlluxioWorker";
private static final String ALLUXIO_PROXY_CLASS = "alluxio.proxy.AlluxioProxy";
private static final Options OPTIONS = new Options();
private final Map mTasks = new HashMap<>();
private final Map mTaskDescriptions = new HashMap<>();
private final List mCommonTasks = new ArrayList<>();
private final List mClusterTasks = new ArrayList<>();
private final List mMasterTasks = new ArrayList<>();
private final List mWorkerTasks = new ArrayList<>();
private final Map> mTargetTasks;
private final AlluxioConfiguration mConf;
private final String mPath;
/**
* Initializes from the target UFS path and configurations.
*
* @param path the UFS path
* @param conf the UFS configurtions
* */
public ValidateEnv(String path, AlluxioConfiguration conf) {
mPath = path;
mConf = conf;
// HDFS configuration validations
registerTask("ufs.hdfs.config.correctness",
"This validates HDFS configuration files like core-site.xml and hdfs-site.xml.",
new HdfsConfValidationTask(mPath, mConf), mCommonTasks);
registerTask("ufs.hdfs.config.parity",
"If a Hadoop config directory is specified, this compares the Hadoop config "
+ "directory with the HDFS configuration paths given to Alluxio, "
+ "and see if they are consistent.",
new HdfsConfParityValidationTask(mPath, mConf), mCommonTasks);
registerTask("ufs.hdfs.config.proxyuser",
"This validates proxy user configuration in HDFS for Alluxio. "
+ "This is needed to enable impersonation for Alluxio.",
new HdfsProxyUserValidationTask(mPath, mConf), mCommonTasks);
registerTask("ufs.hdfs.config.version",
"This validates version compatibility between Alluxio and HDFS.",
new HdfsVersionValidationTask(mConf), mCommonTasks);
// port availability validations
registerTask("master.rpc.port.available",
"validate master RPC port is available",
new PortAvailabilityValidationTask(ServiceType.MASTER_RPC, ALLUXIO_MASTER_CLASS, mConf),
mMasterTasks);
registerTask("master.web.port.available",
"validate master web port is available",
new PortAvailabilityValidationTask(ServiceType.MASTER_WEB, ALLUXIO_MASTER_CLASS, mConf),
mMasterTasks);
registerTask("worker.rpc.port.available",
"validate worker RPC port is available",
new PortAvailabilityValidationTask(ServiceType.WORKER_RPC, ALLUXIO_WORKER_CLASS, mConf),
mWorkerTasks);
registerTask("worker.web.port.available",
"validate worker web port is available",
new PortAvailabilityValidationTask(ServiceType.WORKER_WEB, ALLUXIO_WORKER_CLASS, mConf),
mWorkerTasks);
registerTask("proxy.web.port.available",
"validate proxy web port is available",
new PortAvailabilityValidationTask(ServiceType.PROXY_WEB, ALLUXIO_PROXY_CLASS, mConf),
mCommonTasks);
// security configuration validations
registerTask("master.ufs.hdfs.security.kerberos",
"This validates kerberos security configurations for Alluxio masters.",
new SecureHdfsValidationTask("master", mPath, mConf), mMasterTasks);
registerTask("worker.ufs.hdfs.security.kerberos",
"This validates kerberos security configurations for Alluxio workers.",
new SecureHdfsValidationTask("worker", mPath, mConf), mWorkerTasks);
// ssh validations
registerTask("ssh.nodes.reachable",
"validate SSH port on all Alluxio nodes are reachable",
new SshValidationTask(mConf), mCommonTasks);
// UFS validations
registerTask("ufs.version",
"This validates the a configured UFS library version is available on the system.",
new UfsVersionValidationTask(mPath, mConf), mCommonTasks);
registerTask("ufs.path.accessible",
"This validates the under file system location is accessible to Alluxio.",
new UfsDirectoryValidationTask(mPath, mConf), mCommonTasks);
registerTask("ufs.path.superuser",
"This validates Alluxio has super user privilege on the under file system.",
new UfsSuperUserValidationTask(mPath, mConf), mCommonTasks);
// RAM disk validations
registerTask("worker.ramdisk.mount.privilege",
"validate user has the correct privilege to mount ramdisk",
new RamDiskMountPrivilegeValidationTask(mConf), mWorkerTasks);
// User limit validations
registerTask("ulimit.nofile",
"validate ulimit for number of open files is set appropriately",
UserLimitValidationTask.createOpenFilesLimitValidationTask(), mCommonTasks);
registerTask("ulimit.nproc",
"validate ulimit for number of processes is set appropriately",
UserLimitValidationTask.createUserProcessesLimitValidationTask(), mCommonTasks);
// space validations
registerTask("worker.storage.space",
"validate tiered storage locations have enough space",
new StorageSpaceValidationTask(mConf), mWorkerTasks);
registerTask("cluster.conf.consistent",
"validate configuration consistency across the cluster",
new ClusterConfConsistencyValidationTask(mConf), mClusterTasks);
// java option validations
registerTask("java.native.libs",
String.format("This validates if java native libraries defined at %s all exist.",
NativeLibValidationTask.NATIVE_LIB_PATH),
new NativeLibValidationTask(), mCommonTasks);
mTargetTasks = initializeTargetTasks();
}
private Map> initializeTargetTasks() {
Map> targetMap = new TreeMap<>();
List allMasterTasks = new ArrayList<>(mCommonTasks);
allMasterTasks.addAll(mMasterTasks);
targetMap.put("master", allMasterTasks);
List allWorkerTasks = new ArrayList<>(mCommonTasks);
allWorkerTasks.addAll(mWorkerTasks);
targetMap.put("worker", allWorkerTasks);
targetMap.put("local", mTasks.keySet());
targetMap.put("cluster", new ArrayList<>(mClusterTasks));
return targetMap;
}
private ValidationTask registerTask(String name, String description,
AbstractValidationTask task, List tasks) {
mTasks.put(task, name);
mTaskDescriptions.put(name, description);
tasks.add(task);
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy