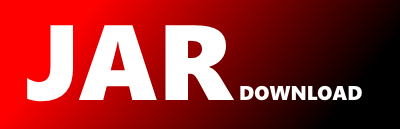
ameba.http.session.CacheSession Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ameba-session Show documentation
Show all versions of ameba-session Show documentation
A useful Java framework session!
package ameba.http.session;
import ameba.cache.Cache;
import java.util.Map;
/**
* @author icode
*/
public class CacheSession extends AbstractSession {
private static final String SESSION_PRE_KEY = CacheSession.class.getName() + ".__SESSION__.";
private SessionStore store;
private boolean fetched = false;
private boolean isDelete = false;
private boolean isTouch = false;
protected CacheSession(String id, String host, long defaultTimeout, boolean isNew) {
super(id, host, defaultTimeout, isNew);
}
public static AbstractSession get(String id) {
return Cache.get(getKey(id));
}
private static String getKey(String id) {
return SESSION_PRE_KEY + id;
}
@Override
public void setAttribute(Object key, Object value) {
getStore().getAttributes().put(key, value);
}
@Override
@SuppressWarnings("unchecked")
public V getAttribute(Object key) {
return (V) getStore().getAttributes().get(key);
}
@Override
public Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy