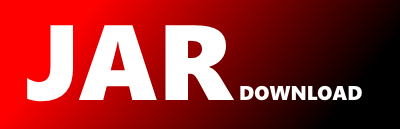
ameba.http.session.Session Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ameba-session Show documentation
Show all versions of ameba-session Show documentation
A useful Java framework session!
package ameba.http.session;
import ameba.core.Requests;
import ameba.util.Times;
import com.google.common.collect.Maps;
import javax.ws.rs.core.Cookie;
import javax.ws.rs.core.NewCookie;
import java.lang.invoke.MethodHandle;
import java.util.Map;
/**
* @author icode
*/
public class Session {
public static final String REQ_SESSION_KEY = Session.class.getName() + ".SESSION_VALUE";
public static final String SET_COOKIE_KEY = SessionFilter.class.getName() + ".__SET_SESSION_COOKIE__";
static MethodHandle GET_SESSION_METHOD_HANDLE;
static MethodHandle NEW_SESSION_ID_METHOD_HANDLE;
static MethodHandle SESSION_CONSTRUCTOR_HANDLE;
static long SESSION_TIMEOUT = Times.parseDuration("2h");
static int COOKIE_MAX_AGE = NewCookie.DEFAULT_MAX_AGE;
static String SESSION_ID_COOKIE_KEY = "s";
public static AbstractSession get() {
return get(true);
}
public static AbstractSession get(boolean create) {
AbstractSession session = (AbstractSession) Requests.getProperty(REQ_SESSION_KEY);
if (session == null && create && Requests.getRequest() != null) {
String sid;
try {
sid = (String) NEW_SESSION_ID_METHOD_HANDLE.invoke();
} catch (Throwable throwable) {
throw new SessionExcption(throwable);
}
NewCookie cookie = new NewCookie(
SESSION_ID_COOKIE_KEY,
sid,
"/",
null,
Cookie.DEFAULT_VERSION,
null,
COOKIE_MAX_AGE,
null,
Requests.getSecurityContext().isSecure(),
true);
Requests.setProperty(SET_COOKIE_KEY, cookie);
session = createSession(sid, Requests.getRemoteRealAddr(), true);
Requests.setProperty(REQ_SESSION_KEY, session);
}
return session;
}
public static AbstractSession get(String id) {
try {
return (AbstractSession) GET_SESSION_METHOD_HANDLE.invoke(id);
} catch (Throwable throwable) {
throw new SessionExcption(throwable);
}
}
static AbstractSession createSession(String sessionId, String host, boolean isNew) {
AbstractSession session;
if (SESSION_CONSTRUCTOR_HANDLE != null) {
try {
session = (AbstractSession) SESSION_CONSTRUCTOR_HANDLE.invoke(sessionId, host, SESSION_TIMEOUT, isNew);
} catch (Throwable throwable) {
throw new SessionExcption("new session instance error");
}
} else {
session = new CacheSession(sessionId, host, SESSION_TIMEOUT, isNew);
}
return session;
}
/**
* Add an attribute to this session.
*
* @param key key
* @param value value
*/
public static void setAttribute(Object key, Object value) {
get().setAttribute(key, value);
}
/**
* Return an attribute.
*
* @param key key
* @return an attribute
*/
public static V getAttribute(Object key) {
if (get(false) != null)
return get().getAttribute(key);
else return null;
}
/**
* Return attributes.
*
* @return attributes
*/
public static Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy