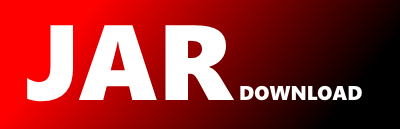
org.analogweb.util.ReflectionUtils Maven / Gradle / Ivy
package org.analogweb.util;
import java.lang.annotation.Annotation;
import java.lang.reflect.Constructor;
import java.lang.reflect.Field;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import java.security.AccessController;
import java.security.PrivilegedAction;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashSet;
import java.util.LinkedList;
import java.util.List;
import java.util.Set;
import org.analogweb.InvocationMetadata;
import org.analogweb.util.logging.Log;
import org.analogweb.util.logging.Logs;
/**
* @author snowgoose
*/
public final class ReflectionUtils {
private static final Log log = Logs.getLog(ReflectionUtils.class);
@SuppressWarnings("unchecked")
public static T getMethodParameterAnnotation(Method method, Class annotationClass,
int parameterIndex) {
Annotation[][] annotations = method.getParameterAnnotations();
if (annotations.length > parameterIndex) {
for (Annotation annotation : annotations[parameterIndex]) {
if (annotation.annotationType().equals(annotationClass)) {
return (T) annotation;
}
}
}
return null;
}
public static T getInstanceQuietly(Class clazz) {
try {
return clazz.newInstance();
} catch (InstantiationException e) {
log.log("TU000008", e, new Object[] { clazz });
} catch (IllegalAccessException e) {
log.log("TU000008", e, new Object[] { clazz });
}
return null;
}
public static Object getInstanceQuietly(Constructor> constructor, Object... args) {
return getInstanceQuietly(Object.class, constructor, args);
}
@SuppressWarnings("unchecked")
public static T getInstanceQuietly(Class type, Constructor> constructor, Object... args) {
try {
if (constructor == null) {
return null;
}
return (T) constructor.newInstance(args);
} catch (IllegalArgumentException e) {
log.log("TU000008", e, new Object[] { constructor.getDeclaringClass() });
} catch (InstantiationException e) {
log.log("TU000008", e, new Object[] { constructor.getDeclaringClass() });
} catch (IllegalAccessException e) {
log.log("TU000008", e, new Object[] { constructor.getDeclaringClass() });
} catch (InvocationTargetException e) {
log.log("TU000008", e, new Object[] { constructor.getDeclaringClass() });
}
return null;
}
public static List> filterClassAsImplementsInterface(Class filteringType,
Collection> collectedClasses) {
return filterClassAsImplementsInterface(filteringType, collectedClasses, ASSIGNABLE_FROM_FILTER);
}
public static List> filterClassAsImplementsInterface(Class filteringType,
Collection> collectedClasses, TypeFilter typeFilter) {
List> implementsTypes = new ArrayList>();
for (Class> clazz : collectedClasses) {
Class filterResult = typeFilter.filterType(clazz, filteringType);
if (filterResult != null) {
implementsTypes.add(filterResult);
}
}
return implementsTypes;
}
public static Method[] getMethods(Class> clazz) {
return clazz.getMethods();
}
public interface TypeFilter {
Class filterType(Class> actualClass, Class filteringType);
}
private static final TypeFilter ASSIGNABLE_FROM_FILTER = new TypeFilter() {
@Override
@SuppressWarnings("unchecked")
public Class filterType(Class> actualClass, Class filteringType) {
if (filteringType.isAssignableFrom(actualClass)) {
return (Class) actualClass;
} else {
return null;
}
}
};
public static void writeValueToField(Field field, Object instance, Object value) {
if (field == null) {
return;
}
try {
setAccessible(field);
field.set(instance, value);
} catch (IllegalAccessException e) {
// swallow
log.log("TU000009", e, field);
}
}
public static void writeValueToField(String fieldName, Object instance, Object value) {
Field field = getAccessibleField(instance.getClass(), fieldName);
if (field == null) {
return;
}
try {
field.set(instance, value);
} catch (IllegalAccessException e) {
// swallow
log.log("TU000009", e, field);
}
}
public static Field getAccessibleField(final Class> targetClass, final String fieldName) {
Field field;
try {
field = targetClass.getDeclaredField(fieldName);
if (Modifier.isPublic(field.getModifiers()) == false) {
setAccessible(field);
}
return field;
} catch (SecurityException e) {
return null;
} catch (NoSuchFieldException e) {
return null;
}
}
public static void setAccessible(final Field field) {
AccessController.doPrivileged(new PrivilegedAction() {
@Override
public Field run() {
try {
if (Modifier.isPublic(field.getModifiers()) == false && !field.isAccessible()) {
field.setAccessible(true);
}
return field;
} catch (SecurityException e) {
// ignore.
return null;
}
}
});
}
public static Object getValueOfField(final String fieldName, final int modifier, final Object instance) {
return AccessController.doPrivileged(new PrivilegedAction
© 2015 - 2025 Weber Informatics LLC | Privacy Policy