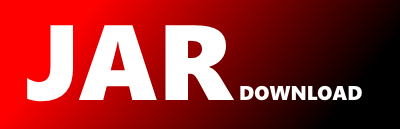
org.anarres.cpp.ResourceFileSystem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jcpp Show documentation
Show all versions of jcpp Show documentation
An embeddable C Preprocessor for the JVM.
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package org.anarres.cpp;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import javax.annotation.Nonnull;
/**
*
* @author shevek
*/
public class ResourceFileSystem implements VirtualFileSystem {
private final ClassLoader loader;
public ResourceFileSystem(@Nonnull ClassLoader loader) {
this.loader = loader;
}
@Override
public VirtualFile getFile(String path) {
return new ResourceFile(loader, path);
}
@Override
public VirtualFile getFile(String dir, String name) {
return getFile(dir + "/" + name);
}
private class ResourceFile implements VirtualFile {
private final ClassLoader loader;
private final String path;
public ResourceFile(ClassLoader loader, String path) {
this.loader = loader;
this.path = path;
}
@Override
public boolean isFile() {
throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates.
}
@Override
public String getPath() {
return path;
}
@Override
public String getName() {
return path.substring(path.lastIndexOf('/') + 1);
}
@Override
public ResourceFile getParentFile() {
int idx = path.lastIndexOf('/');
if (idx < 1)
return null;
return new ResourceFile(loader, path.substring(0, idx));
}
@Override
public ResourceFile getChildFile(String name) {
return new ResourceFile(loader, path + "/" + name);
}
@Override
public Source getSource() throws IOException {
InputStream stream = loader.getResourceAsStream(path);
BufferedReader reader = new BufferedReader(new InputStreamReader(stream));
return new LexerSource(reader, true);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy