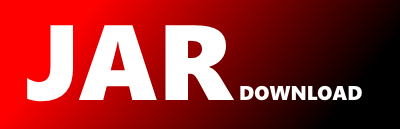
org.androidannotations.rest.spring.annotations.Rest Maven / Gradle / Ivy
Show all versions of rest-spring-api Show documentation
/**
* Copyright (C) 2010-2016 eBusiness Information, Excilys Group
* Copyright (C) 2016-2017 the AndroidAnnotations project
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed To in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package org.androidannotations.rest.spring.annotations;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
/**
*
* Apply @{@link Rest} on an interface to create a RestService class that will
* contain implementation of rest calls related to the methods you define in the
* interface.
*
*
* You should then inject your RestService class by using {@link RestService}
* annotation in any enhanced classes.
*
*
* Note: Implementation is based on Spring Android Rest-template library. So you MUST have the
* library in your classpath and we highly recommend you to take some time to
* read this document and understand how the library works.
*
*
*
* Converters
*
* Every {@link Rest} annotated interface MUST define at least one
* {@link #converters()} to tell the library how to convert received data into
* Java objects.
*
*
* {@link #converters()} value MAY contain one or several
* {@link org.springframework.http.converter.HttpMessageConverter} sub-classes
*
*
*
* Example : The following RestClient will use Jackson to deserialize received data
* as Java objects.
*
*
* @Rest(converters = MappingJackson2HttpMessageConverter.class)
* public interface RestClient {
*
* @Get("http://myserver/events")
* EventList getEvents();
* }
*
*
*
*
*
* Root url
*
* If you don't wan't to repeat the root URL in each method, you MAY like the
* {@link #rootUrl()} field. It let you define a common root URL which will be
* prefixed on every method of your RestClient.
*
*
*
*
* Example :
*
*
* @Rest(rootUrl = "http://myserver", converters = MappingJackson2HttpMessageConverter.class)
* public interface RestClient {
*
* @Get("/events")
* EventList getEvents();
*
* @Get("/lastevent")
* Event getLastEvent();
* }
*
*
*
*
*
* Interceptors
*
* Sometimes you may want to do extra processing right before or after requests.
* {@link #interceptors()} field let you define one or several
* {@link org.springframework.http.client.ClientHttpRequestInterceptor}.
*
*
* An interceptor allow the developer to customize the execution flow of
* requests. It may be useful to handle custom authentication, automatically log
* each requests, and so on.
*
*
*
* Example :
*
*
* @Rest(converters = MappingJacksonHttpMessageConverter.class, interceptors = HttpBasicAuthenticatorInterceptor.class)
* public interface MyRestClient {
*
* @Get("/events")
* EventList getEvents();
* }
*
* public class HttpBasicAuthenticatorInterceptor implements ClientHttpRequestInterceptor {
*
* @Override
* public ClientHttpResponse intercept(HttpRequest request, byte[] data, ClientHttpRequestExecution execution) throws IOException {
* // do something before sending request
* return execution.execute(request, data);
* }
* }
*
*
*
*
*
* You can also inject {@link org.androidannotations.annotations.EBean EBean}
* interceptors. Just add the annotated class (not the generated one) to the
* {@link Rest#interceptors() interceptors()} parameter, and the interceptor
* will be added with all of its dependencies.
*
*
*
* RequestFactory
*
* You can use your own request factory if you want to customize how requests
* are created. The {@link #requestFactory()} parameter lets you define the
* {@link org.springframework.http.client.ClientHttpRequestFactory
* ClientHttpRequestFactory}.
*
*
*
* You can inject {@link org.androidannotations.annotations.EBean EBean} request
* factories just like as interceptors.
*
*
*
* Example :
*
*
* @Rest(converters = MappingJacksonHttpMessageConverter.class, requestFactory = MyRequestFactory.class)
* public interface MyRestClient {
*
* @Get("/events")
* EventList getEvents();
* }
*
* public class MyRequestFactory implements ClientHttpRequestFactory {
*
* @Override
* public ClientHttpRequest createRequest(URI uri, HttpMethod httpMethod) throws IOException {
* // create and return the request
* }
* }
*
*
*
*
* ResponseErrorHandler
*
* You can use your own error handler to customize how errors
* are handled. The {@link #responseErrorHandler()} parameter lets you define the
* {@link org.springframework.web.client.ResponseErrorHandler
* ResponseErrorHandler}.
*
*
*
* You can inject an {@link org.androidannotations.annotations.EBean EBean} response errork
* handler just like as a request factory.
*
*
*
* Example :
*
*
* @Rest(converters = MappingJacksonHttpMessageConverter.class, responseErrorHandler = MyResponseErrorHandler.class)
* public interface MyRestClient {
*
* @Get("/events")
* EventList getEvents();
* }
*
* public class MyResponseErrorHandler implements ResponseErrorHandler {
*
* @Override
* void handleError(ClientHttpResponse response) throws IOException {
* // handles the error in the given response
* }
*
* @Override
* boolean hasError(ClientHttpResponse response) throws IOException {
* // indicates whether the given response has any errors
* return true;
* }
* }
*
*
*
*
* Magic methods
*
* AA will automatically detect and implement some methods in {@link Rest}
* annotated interface. These methods will let you dynamically customize the
* RestClient.
*
* RootUrl
*
* We seen earlier that root url can be set via {@link #rootUrl()} annotation
* field, but it only takes a constant. If you want to dynamically inject or
* retrieve the root url, you can add the following code :
*
*
*
*
* @Rest(converters = MappingJacksonHttpMessageConverter.class)
* public interface MyRestClient {
*
* void setRootUrl(String rootUrl);
*
* String getRootUrl();
* }
*
*
*
*
* RestTemplate
*
* If you want to configure the injected RestTemplate used internally, AA will
* also detect getter and setter for this object.
*
*
*
*
* @Rest(converters = MappingJacksonHttpMessageConverter.class)
* public interface MyRestClient {
*
* RestTemplate getRestTemplate();
*
* void setRestTemplate(RestTemplate restTemplate);
* }
*
*
*
*
* Bundle interfaces
*
* Since 3.0, we provide some bundle interfaces, which declare the magic methods
* listed above. You can extend from these interfaces, so you do not have to
* write them directly in your {@link Rest} annotated interface.
*
*
* Available bundle interfaces :
*
*
* - RestClientRootUrl: provide
getRootUrl()
and
* setRootUrl()
* - RestClientSupport: provide
getRestTemplate()
and
* setRestTemplate()
* - RestClientHeaders: provide
getHeader()
,
* setHeader()
, getCookie()
, setCookie()
,
* setAuthentication()
and setHttpBasicAuth()
*
*
*
* @see RestService
* @see org.androidannotations.rest.spring.api.RestClientSupport
* @see org.androidannotations.rest.spring.api.RestClientRootUrl
* @see org.androidannotations.rest.spring.api.RestClientHeaders
*/
@Retention(RetentionPolicy.CLASS)
@Target(ElementType.TYPE)
public @interface Rest {
/**
* The root url of the web service.
*
* @return the root url of the web service
*/
String rootUrl() default "";
/**
* The classes of the converters which should be used to convert received
* data into Java objects.
*
* @return the converter classes
*/
Class>[] converters();
/**
* The classes of interceptors which are used to do extra processing before
* or after requests.
*
* @return the interceptor classes
*/
Class>[] interceptors() default {};
/**
* The request factory class which is used to create the HTTP requests.
*
* @return the request factory class
*/
Class> requestFactory() default Void.class;
/**
* The response error handler class which is used to handle errors.
*
* @return the response error handler class
*/
Class> responseErrorHandler() default Void.class;
}