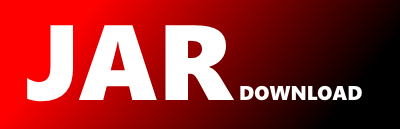
org.andromda.metafacades.emf.uml22.AssociationFacadeLogic Maven / Gradle / Ivy
Show all versions of andromda-metafacades-emf-uml22 Show documentation
// license-header java merge-point
//
// Attention: generated code (by MetafacadeLogic.vsl) - do not modify!
//
package org.andromda.metafacades.emf.uml22;
import java.util.Collection;
import java.util.List;
import org.andromda.core.metafacade.MetafacadeBase;
import org.andromda.core.metafacade.ModelValidationMessage;
import org.andromda.metafacades.uml.AssociationEndFacade;
import org.andromda.metafacades.uml.AssociationFacade;
import org.apache.log4j.Logger;
import org.eclipse.uml2.uml.Association;
/**
*
* An association describes a set of tuples whose values refer to
* typed instances. An instance of an association is called a link.
*
* MetafacadeLogic for AssociationFacade
*
* @see AssociationFacade
*/
public abstract class AssociationFacadeLogic
extends GeneralizableElementFacadeLogicImpl
implements AssociationFacade
{
/**
* The underlying UML object
* @see Association
*/
protected Association metaObject;
/** Create Metafacade implementation instance using the MetafacadeFactory from the context
* @param metaObjectIn
* @param context
*/
protected AssociationFacadeLogic(Association metaObjectIn, String context)
{
super(metaObjectIn, getContext(context));
this.metaObject = metaObjectIn;
}
/**
* The logger instance.
*/
private static final Logger logger = Logger.getLogger(AssociationFacadeLogic.class);
/**
* Gets the context for this metafacade logic instance.
* @param context String. Set to AssociationFacade if null
* @return context String
*/
private static String getContext(String context)
{
if (context == null)
{
context = "org.andromda.metafacades.uml.AssociationFacade";
}
return context;
}
/** Reset context only for non-root metafacades
* @param context
*/
@Override
public void resetMetafacadeContext(String context)
{
if (!this.contextRoot) // reset context only for non-root metafacades
{
context = getContext(context); // to have same value as in original constructor call
setMetafacadeContext (context);
}
}
/**
* @return boolean true always
* @see AssociationFacade
*/
public boolean isAssociationFacadeMetaType()
{
return true;
}
// --------------- attributes ---------------------
/**
* @see AssociationFacade#getRelationName()
* @return String
*/
protected abstract String handleGetRelationName();
private String __relationName1a;
private boolean __relationName1aSet = false;
/**
*
* A name suited for naming this relationship. This name will be
* constructed from both association ends.
*
* @return (String)handleGetRelationName()
*/
public final String getRelationName()
{
String relationName1a = this.__relationName1a;
if (!this.__relationName1aSet)
{
// relationName has no pre constraints
relationName1a = handleGetRelationName();
// relationName has no post constraints
this.__relationName1a = relationName1a;
if (isMetafacadePropertyCachingEnabled())
{
this.__relationName1aSet = true;
}
}
return relationName1a;
}
/**
* @see AssociationFacade#isMany2Many()
* @return boolean
*/
protected abstract boolean handleIsMany2Many();
private boolean __many2Many2a;
private boolean __many2Many2aSet = false;
/**
*
* Indicates whether or not this associations represents a
* many-to-many relation.
*
* @return (boolean)handleIsMany2Many()
*/
public final boolean isMany2Many()
{
boolean many2Many2a = this.__many2Many2a;
if (!this.__many2Many2aSet)
{
// many2Many has no pre constraints
many2Many2a = handleIsMany2Many();
// many2Many has no post constraints
this.__many2Many2a = many2Many2a;
if (isMetafacadePropertyCachingEnabled())
{
this.__many2Many2aSet = true;
}
}
return many2Many2a;
}
/**
* @see AssociationFacade#isAssociationClass()
* @return boolean
*/
protected abstract boolean handleIsAssociationClass();
private boolean __associationClass3a;
private boolean __associationClass3aSet = false;
/**
*
* @return (boolean)handleIsAssociationClass()
*/
public final boolean isAssociationClass()
{
boolean associationClass3a = this.__associationClass3a;
if (!this.__associationClass3aSet)
{
// associationClass has no pre constraints
associationClass3a = handleIsAssociationClass();
// associationClass has no post constraints
this.__associationClass3a = associationClass3a;
if (isMetafacadePropertyCachingEnabled())
{
this.__associationClass3aSet = true;
}
}
return associationClass3a;
}
/**
* @see AssociationFacade#isAbstract()
* @return boolean
*/
protected abstract boolean handleIsAbstract();
private boolean __abstract4a;
private boolean __abstract4aSet = false;
/**
*
* Indicates if this association is 'abstract'.
*
* @return (boolean)handleIsAbstract()
*/
public final boolean isAbstract()
{
boolean abstract4a = this.__abstract4a;
if (!this.__abstract4aSet)
{
// abstract has no pre constraints
abstract4a = handleIsAbstract();
// abstract has no post constraints
this.__abstract4a = abstract4a;
if (isMetafacadePropertyCachingEnabled())
{
this.__abstract4aSet = true;
}
}
return abstract4a;
}
/**
* @see AssociationFacade#isLeaf()
* @return boolean
*/
protected abstract boolean handleIsLeaf();
private boolean __leaf5a;
private boolean __leaf5aSet = false;
/**
*
* True if this association cannot be extended and represent a leaf
* in the inheritance tree.
*
* @return (boolean)handleIsLeaf()
*/
public final boolean isLeaf()
{
boolean leaf5a = this.__leaf5a;
if (!this.__leaf5aSet)
{
// leaf has no pre constraints
leaf5a = handleIsLeaf();
// leaf has no post constraints
this.__leaf5a = leaf5a;
if (isMetafacadePropertyCachingEnabled())
{
this.__leaf5aSet = true;
}
}
return leaf5a;
}
/**
* @see AssociationFacade#isDerived()
* @return boolean
*/
protected abstract boolean handleIsDerived();
private boolean __derived6a;
private boolean __derived6aSet = false;
/**
*
* UML2: Returns the value of the 'Is Derived' attribute. The
* default value is "false". If isDerived is true, the value of the
* attribute is derived from information elsewhere. Specifies
* whether the Property is derived, i.e., whether its value or
* values can be computed from other information.
*
* @return (boolean)handleIsDerived()
*/
public final boolean isDerived()
{
boolean derived6a = this.__derived6a;
if (!this.__derived6aSet)
{
// derived has no pre constraints
derived6a = handleIsDerived();
// derived has no post constraints
this.__derived6a = derived6a;
if (isMetafacadePropertyCachingEnabled())
{
this.__derived6aSet = true;
}
}
return derived6a;
}
/**
* @see AssociationFacade#isBinary()
* @return boolean
*/
protected abstract boolean handleIsBinary();
private boolean __binary7a;
private boolean __binary7aSet = false;
/**
*
* UML2: Determines whether this association is a binary
* association, i.e. whether it has exactly two member ends. UML2
* allows association classes in the association itself (many2many
* with association attributes). Default=true: only two member
* ends.
*
* @return (boolean)handleIsBinary()
*/
public final boolean isBinary()
{
boolean binary7a = this.__binary7a;
if (!this.__binary7aSet)
{
// binary has no pre constraints
binary7a = handleIsBinary();
// binary has no post constraints
this.__binary7a = binary7a;
if (isMetafacadePropertyCachingEnabled())
{
this.__binary7aSet = true;
}
}
return binary7a;
}
// ------------- associations ------------------
/**
*
* The association owning this association end.
*
* @return (List)handleGetAssociationEnds()
*/
public final List getAssociationEnds()
{
List getAssociationEnds1r = null;
// association has no pre constraints
List result = handleGetAssociationEnds();
List shieldedResult = this.shieldedElements(result);
try
{
getAssociationEnds1r = (List)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
AssociationFacadeLogic.logger.warn("incorrect metafacade cast for AssociationFacadeLogic.getAssociationEnds List " + result + ": " + shieldedResult);
}
// association has no post constraints
return getAssociationEnds1r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return List
*/
protected abstract List handleGetAssociationEnds();
private AssociationEndFacade __getAssociationEndA2r;
private boolean __getAssociationEndA2rSet = false;
/**
*
* @return (AssociationEndFacade)handleGetAssociationEndA()
*/
public final AssociationEndFacade getAssociationEndA()
{
AssociationEndFacade getAssociationEndA2r = this.__getAssociationEndA2r;
if (!this.__getAssociationEndA2rSet)
{
// associationFacade has no pre constraints
Object result = handleGetAssociationEndA();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getAssociationEndA2r = (AssociationEndFacade)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
AssociationFacadeLogic.logger.warn("incorrect metafacade cast for AssociationFacadeLogic.getAssociationEndA AssociationEndFacade " + result + ": " + shieldedResult);
}
// associationFacade has no post constraints
this.__getAssociationEndA2r = getAssociationEndA2r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getAssociationEndA2rSet = true;
}
}
return getAssociationEndA2r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetAssociationEndA();
private AssociationEndFacade __getAssociationEndB3r;
private boolean __getAssociationEndB3rSet = false;
/**
*
* @return (AssociationEndFacade)handleGetAssociationEndB()
*/
public final AssociationEndFacade getAssociationEndB()
{
AssociationEndFacade getAssociationEndB3r = this.__getAssociationEndB3r;
if (!this.__getAssociationEndB3rSet)
{
// associationFacade has no pre constraints
Object result = handleGetAssociationEndB();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getAssociationEndB3r = (AssociationEndFacade)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
AssociationFacadeLogic.logger.warn("incorrect metafacade cast for AssociationFacadeLogic.getAssociationEndB AssociationEndFacade " + result + ": " + shieldedResult);
}
// associationFacade has no post constraints
this.__getAssociationEndB3r = getAssociationEndB3r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getAssociationEndB3rSet = true;
}
}
return getAssociationEndB3r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetAssociationEndB();
/**
* @param validationMessages Collection
* @see GeneralizableElementFacadeLogicImpl#validateInvariants(Collection validationMessages)
*/
@Override
public void validateInvariants(Collection validationMessages)
{
super.validateInvariants(validationMessages);
}
}