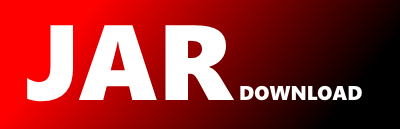
org.andromda.metafacades.emf.uml22.EntityAttributeLogic Maven / Gradle / Ivy
Show all versions of andromda-metafacades-emf-uml22 Show documentation
// license-header java merge-point
//
// Attention: generated code (by MetafacadeLogic.vsl) - do not modify!
//
package org.andromda.metafacades.emf.uml22;
import java.util.Collection;
import org.andromda.core.metafacade.ModelValidationMessage;
import org.andromda.metafacades.uml.EntityAttribute;
import org.andromda.metafacades.uml.TypeMappings;
/**
*
* Represents an attribute of an entity.
*
* MetafacadeLogic for EntityAttribute
*
* @see EntityAttribute
*/
public abstract class EntityAttributeLogic
extends AttributeFacadeLogicImpl
implements EntityAttribute
{
/**
* The underlying UML object
* @see Object
*/
protected Object metaObject;
/** Create Metafacade implementation instance using the MetafacadeFactory from the context
* @param metaObjectIn
* @param context
*/
protected EntityAttributeLogic(Object metaObjectIn, String context)
{
super((Attribute)metaObjectIn, getContext(context));
this.metaObject = metaObjectIn;
}
/**
* Gets the context for this metafacade logic instance.
* @param context String. Set to EntityAttribute if null
* @return context String
*/
private static String getContext(String context)
{
if (context == null)
{
context = "org.andromda.metafacades.uml.EntityAttribute";
}
return context;
}
/** Reset context only for non-root metafacades
* @param context
*/
@Override
public void resetMetafacadeContext(String context)
{
if (!this.contextRoot) // reset context only for non-root metafacades
{
context = getContext(context); // to have same value as in original constructor call
setMetafacadeContext (context);
}
}
/**
* @return boolean true always
* @see EntityAttribute
*/
public boolean isEntityAttributeMetaType()
{
return true;
}
// --------------- attributes ---------------------
/**
* @see EntityAttribute#getColumnLength()
* @return String
*/
protected abstract String handleGetColumnLength();
private String __columnLength1a;
private boolean __columnLength1aSet = false;
/**
*
* The length of the column that persists this entity attribute.
*
* @return (String)handleGetColumnLength()
*/
public final String getColumnLength()
{
String columnLength1a = this.__columnLength1a;
if (!this.__columnLength1aSet)
{
// columnLength has no pre constraints
columnLength1a = handleGetColumnLength();
// columnLength has no post constraints
this.__columnLength1a = columnLength1a;
if (isMetafacadePropertyCachingEnabled())
{
this.__columnLength1aSet = true;
}
}
return columnLength1a;
}
/**
* @see EntityAttribute#getColumnName()
* @return String
*/
protected abstract String handleGetColumnName();
private String __columnName2a;
private boolean __columnName2aSet = false;
/**
*
* The name of the table column to which this entity is mapped.
*
* @return (String)handleGetColumnName()
*/
public final String getColumnName()
{
String columnName2a = this.__columnName2a;
if (!this.__columnName2aSet)
{
// columnName has no pre constraints
columnName2a = handleGetColumnName();
// columnName has no post constraints
this.__columnName2a = columnName2a;
if (isMetafacadePropertyCachingEnabled())
{
this.__columnName2aSet = true;
}
}
return columnName2a;
}
/**
* @see EntityAttribute#getJdbcMappings()
* @return TypeMappings
*/
protected abstract TypeMappings handleGetJdbcMappings();
private TypeMappings __jdbcMappings3a;
private boolean __jdbcMappings3aSet = false;
/**
*
* The PIM to language specific mappings for JDBC.
*
* @return (TypeMappings)handleGetJdbcMappings()
*/
public final TypeMappings getJdbcMappings()
{
TypeMappings jdbcMappings3a = this.__jdbcMappings3a;
if (!this.__jdbcMappings3aSet)
{
// jdbcMappings has no pre constraints
jdbcMappings3a = handleGetJdbcMappings();
// jdbcMappings has no post constraints
this.__jdbcMappings3a = jdbcMappings3a;
if (isMetafacadePropertyCachingEnabled())
{
this.__jdbcMappings3aSet = true;
}
}
return jdbcMappings3a;
}
/**
* @see EntityAttribute#getJdbcType()
* @return String
*/
protected abstract String handleGetJdbcType();
private String __jdbcType4a;
private boolean __jdbcType4aSet = false;
/**
*
* The JDBC type for this entity attribute.
*
* @return (String)handleGetJdbcType()
*/
public final String getJdbcType()
{
String jdbcType4a = this.__jdbcType4a;
if (!this.__jdbcType4aSet)
{
// jdbcType has no pre constraints
jdbcType4a = handleGetJdbcType();
// jdbcType has no post constraints
this.__jdbcType4a = jdbcType4a;
if (isMetafacadePropertyCachingEnabled())
{
this.__jdbcType4aSet = true;
}
}
return jdbcType4a;
}
/**
* @see EntityAttribute#getSqlMappings()
* @return TypeMappings
*/
protected abstract TypeMappings handleGetSqlMappings();
private TypeMappings __sqlMappings5a;
private boolean __sqlMappings5aSet = false;
/**
*
* The SQL mappings (i.e. the mappings which provide PIM to SQL
* mappings).
*
* @return (TypeMappings)handleGetSqlMappings()
*/
public final TypeMappings getSqlMappings()
{
TypeMappings sqlMappings5a = this.__sqlMappings5a;
if (!this.__sqlMappings5aSet)
{
// sqlMappings has no pre constraints
sqlMappings5a = handleGetSqlMappings();
// sqlMappings has no post constraints
this.__sqlMappings5a = sqlMappings5a;
if (isMetafacadePropertyCachingEnabled())
{
this.__sqlMappings5aSet = true;
}
}
return sqlMappings5a;
}
/**
* @see EntityAttribute#getSqlType()
* @return String
*/
protected abstract String handleGetSqlType();
private String __sqlType6a;
private boolean __sqlType6aSet = false;
/**
*
* The SQL type for this attribute.
*
* @return (String)handleGetSqlType()
*/
public final String getSqlType()
{
String sqlType6a = this.__sqlType6a;
if (!this.__sqlType6aSet)
{
// sqlType has no pre constraints
sqlType6a = handleGetSqlType();
// sqlType has no post constraints
this.__sqlType6a = sqlType6a;
if (isMetafacadePropertyCachingEnabled())
{
this.__sqlType6aSet = true;
}
}
return sqlType6a;
}
/**
* @see EntityAttribute#isIdentifier()
* @return boolean
*/
protected abstract boolean handleIsIdentifier();
private boolean __identifier7a;
private boolean __identifier7aSet = false;
/**
*
* True if this attribute is an identifier for its entity.
*
* @return (boolean)handleIsIdentifier()
*/
public final boolean isIdentifier()
{
boolean identifier7a = this.__identifier7a;
if (!this.__identifier7aSet)
{
// identifier has no pre constraints
identifier7a = handleIsIdentifier();
// identifier has no post constraints
this.__identifier7a = identifier7a;
if (isMetafacadePropertyCachingEnabled())
{
this.__identifier7aSet = true;
}
}
return identifier7a;
}
/**
* @see EntityAttribute#getColumnIndex()
* @return String
*/
protected abstract String handleGetColumnIndex();
private String __columnIndex8a;
private boolean __columnIndex8aSet = false;
/**
*
* The name of the index to create on a column that persists the
* entity attribute.
*
* @return (String)handleGetColumnIndex()
*/
public final String getColumnIndex()
{
String columnIndex8a = this.__columnIndex8a;
if (!this.__columnIndex8aSet)
{
// columnIndex has no pre constraints
columnIndex8a = handleGetColumnIndex();
// columnIndex has no post constraints
this.__columnIndex8a = columnIndex8a;
if (isMetafacadePropertyCachingEnabled())
{
this.__columnIndex8aSet = true;
}
}
return columnIndex8a;
}
/**
* @see EntityAttribute#isTransient()
* @return boolean
*/
protected abstract boolean handleIsTransient();
private boolean __transient9a;
private boolean __transient9aSet = false;
/**
*
* Indicates this attribute should be ignored by the persistence
* layer.
*
* @return (boolean)handleIsTransient()
*/
public final boolean isTransient()
{
boolean transient9a = this.__transient9a;
if (!this.__transient9aSet)
{
// transient has no pre constraints
transient9a = handleIsTransient();
// transient has no post constraints
this.__transient9a = transient9a;
if (isMetafacadePropertyCachingEnabled())
{
this.__transient9aSet = true;
}
}
return transient9a;
}
/**
* @see EntityAttribute#getUniqueGroup()
* @return String
*/
protected abstract String handleGetUniqueGroup();
private String __uniqueGroup10a;
private boolean __uniqueGroup10aSet = false;
/**
*
* The name of the unique-key that this unique attribute belongs
*
* @return (String)handleGetUniqueGroup()
*/
public final String getUniqueGroup()
{
String uniqueGroup10a = this.__uniqueGroup10a;
if (!this.__uniqueGroup10aSet)
{
// uniqueGroup has no pre constraints
uniqueGroup10a = handleGetUniqueGroup();
// uniqueGroup has no post constraints
this.__uniqueGroup10a = uniqueGroup10a;
if (isMetafacadePropertyCachingEnabled())
{
this.__uniqueGroup10aSet = true;
}
}
return uniqueGroup10a;
}
// ------------- associations ------------------
/**
* @param validationMessages Collection
* @see AttributeFacadeLogicImpl#validateInvariants(Collection validationMessages)
*/
@Override
public void validateInvariants(Collection validationMessages)
{
super.validateInvariants(validationMessages);
}
}