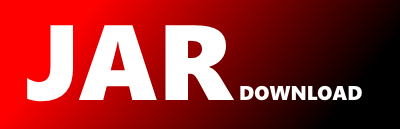
org.andromda.metafacades.emf.uml22.EntityLogic Maven / Gradle / Ivy
Show all versions of andromda-metafacades-emf-uml22 Show documentation
// license-header java merge-point
//
// Attention: generated code (by MetafacadeLogic.vsl) - do not modify!
//
package org.andromda.metafacades.emf.uml22;
import java.util.Collection;
import java.util.List;
import org.andromda.core.metafacade.MetafacadeBase;
import org.andromda.core.metafacade.ModelValidationMessage;
import org.andromda.metafacades.uml.DependencyFacade;
import org.andromda.metafacades.uml.Entity;
import org.andromda.metafacades.uml.EntityAssociationEnd;
import org.andromda.metafacades.uml.EntityAttribute;
import org.andromda.metafacades.uml.EntityQueryOperation;
import org.andromda.metafacades.uml.OperationFacade;
import org.andromda.translation.ocl.validation.OCLCollections;
import org.andromda.translation.ocl.validation.OCLExpressions;
import org.andromda.translation.ocl.validation.OCLIntrospector;
import org.andromda.translation.ocl.validation.OCLResultEnsurer;
import org.apache.commons.collections.Predicate;
import org.apache.log4j.Logger;
import org.eclipse.uml2.uml.Classifier;
/**
*
* Represents a persistent entity.
*
* MetafacadeLogic for Entity
*
* @see Entity
*/
public abstract class EntityLogic
extends ClassifierFacadeLogicImpl
implements Entity
{
/**
* The underlying UML object
* @see Object
*/
protected Object metaObject;
/** Create Metafacade implementation instance using the MetafacadeFactory from the context
* @param metaObjectIn
* @param context
*/
protected EntityLogic(Object metaObjectIn, String context)
{
super((Classifier)metaObjectIn, getContext(context));
this.metaObject = metaObjectIn;
}
/**
* The logger instance.
*/
private static final Logger logger = Logger.getLogger(EntityLogic.class);
/**
* Gets the context for this metafacade logic instance.
* @param context String. Set to Entity if null
* @return context String
*/
private static String getContext(String context)
{
if (context == null)
{
context = "org.andromda.metafacades.uml.Entity";
}
return context;
}
/** Reset context only for non-root metafacades
* @param context
*/
@Override
public void resetMetafacadeContext(String context)
{
if (!this.contextRoot) // reset context only for non-root metafacades
{
context = getContext(context); // to have same value as in original constructor call
setMetafacadeContext (context);
}
}
/**
* @return boolean true always
* @see Entity
*/
public boolean isEntityMetaType()
{
return true;
}
// --------------- attributes ---------------------
/**
* @see Entity#getTableName()
* @return String
*/
protected abstract String handleGetTableName();
private String __tableName1a;
private boolean __tableName1aSet = false;
/**
*
* The name of the database table to which this entity is
* persisted.
*
* @return (String)handleGetTableName()
*/
public final String getTableName()
{
String tableName1a = this.__tableName1a;
if (!this.__tableName1aSet)
{
// tableName has no pre constraints
tableName1a = handleGetTableName();
// tableName has no post constraints
this.__tableName1a = tableName1a;
if (isMetafacadePropertyCachingEnabled())
{
this.__tableName1aSet = true;
}
}
return tableName1a;
}
/**
* @see Entity#isIdentifiersPresent()
* @return boolean
*/
protected abstract boolean handleIsIdentifiersPresent();
/**
*
* True if the entity has any identifiers defined, false otherwise.
*
* @return (boolean)handleIsIdentifiersPresent()
*/
public final boolean isIdentifiersPresent()
{
boolean identifiersPresent2a = false;
// identifiersPresent has no pre constraints
identifiersPresent2a = handleIsIdentifiersPresent();
// identifiersPresent has no post constraints
return identifiersPresent2a;
}
/**
* @see Entity#getMaxSqlNameLength()
* @return short
*/
protected abstract short handleGetMaxSqlNameLength();
private short __maxSqlNameLength3a;
private boolean __maxSqlNameLength3aSet = false;
/**
*
* The maximum length a SQL name may be.
*
* @return (short)handleGetMaxSqlNameLength()
*/
public final short getMaxSqlNameLength()
{
short maxSqlNameLength3a = this.__maxSqlNameLength3a;
if (!this.__maxSqlNameLength3aSet)
{
// maxSqlNameLength has no pre constraints
maxSqlNameLength3a = handleGetMaxSqlNameLength();
// maxSqlNameLength has no post constraints
this.__maxSqlNameLength3a = maxSqlNameLength3a;
if (isMetafacadePropertyCachingEnabled())
{
this.__maxSqlNameLength3aSet = true;
}
}
return maxSqlNameLength3a;
}
/**
* @see Entity#isChild()
* @return boolean
*/
protected abstract boolean handleIsChild();
private boolean __child4a;
private boolean __child4aSet = false;
/**
*
* Returns true/false depending on whether or not this entity
* represetns a child in an association (this occurs when this
* entity is on the opposite end of an assocation end defined as
* composite).
*
* @return (boolean)handleIsChild()
*/
public final boolean isChild()
{
boolean child4a = this.__child4a;
if (!this.__child4aSet)
{
// child has no pre constraints
child4a = handleIsChild();
// child has no post constraints
this.__child4a = child4a;
if (isMetafacadePropertyCachingEnabled())
{
this.__child4aSet = true;
}
}
return child4a;
}
/**
* @see Entity#isUsingForeignIdentifier()
* @return boolean
*/
protected abstract boolean handleIsUsingForeignIdentifier();
/**
*
* Indicates whether or not this entity is using a foreign
* identifier as its identifiers. That is: the foreignIdentifier
* flag was set on an incoming association end and the entity is
* therefore using the related foreign parent entity's identifier.
*
* @return (boolean)handleIsUsingForeignIdentifier()
*/
public final boolean isUsingForeignIdentifier()
{
boolean usingForeignIdentifier5a = false;
// usingForeignIdentifier has no pre constraints
usingForeignIdentifier5a = handleIsUsingForeignIdentifier();
// usingForeignIdentifier has no post constraints
return usingForeignIdentifier5a;
}
/**
* @see Entity#isDynamicIdentifiersPresent()
* @return boolean
*/
protected abstract boolean handleIsDynamicIdentifiersPresent();
/**
*
* True if the entity has its identifiers dynamically added, false
* otherwise.
*
* @return (boolean)handleIsDynamicIdentifiersPresent()
*/
public final boolean isDynamicIdentifiersPresent()
{
boolean dynamicIdentifiersPresent6a = false;
// dynamicIdentifiersPresent has no pre constraints
dynamicIdentifiersPresent6a = handleIsDynamicIdentifiersPresent();
// dynamicIdentifiersPresent has no post constraints
return dynamicIdentifiersPresent6a;
}
/**
* @see Entity#isUsingAssignedIdentifier()
* @return boolean
*/
protected abstract boolean handleIsUsingAssignedIdentifier();
/**
*
* Indiciates if this entity is using an assigned identifier or
* not.
*
* @return (boolean)handleIsUsingAssignedIdentifier()
*/
public final boolean isUsingAssignedIdentifier()
{
boolean usingAssignedIdentifier7a = false;
// usingAssignedIdentifier has no pre constraints
usingAssignedIdentifier7a = handleIsUsingAssignedIdentifier();
// usingAssignedIdentifier has no post constraints
return usingAssignedIdentifier7a;
}
/**
* @see Entity#getSchema()
* @return String
*/
protected abstract String handleGetSchema();
private String __schema8a;
private boolean __schema8aSet = false;
/**
*
* The name of the schema that contains the database table
*
* @return (String)handleGetSchema()
*/
public final String getSchema()
{
String schema8a = this.__schema8a;
if (!this.__schema8aSet)
{
// schema has no pre constraints
schema8a = handleGetSchema();
// schema has no post constraints
this.__schema8a = schema8a;
if (isMetafacadePropertyCachingEnabled())
{
this.__schema8aSet = true;
}
}
return schema8a;
}
/**
* @see Entity#isCompositeIdentifier()
* @return boolean
*/
protected abstract boolean handleIsCompositeIdentifier();
/**
*
* @return (boolean)handleIsCompositeIdentifier()
*/
public final boolean isCompositeIdentifier()
{
boolean compositeIdentifier9a = false;
// compositeIdentifier has no pre constraints
compositeIdentifier9a = handleIsCompositeIdentifier();
// compositeIdentifier has no post constraints
return compositeIdentifier9a;
}
/**
* @see Entity#getEmbeddedValues()
* @return Collection
*/
protected abstract Collection handleGetEmbeddedValues();
private Collection __embeddedValues10a;
private boolean __embeddedValues10aSet = false;
/**
*
* The embedded values belonging to this entity.
*
* @return (Collection)handleGetEmbeddedValues()
*/
public final Collection getEmbeddedValues()
{
Collection embeddedValues10a = this.__embeddedValues10a;
if (!this.__embeddedValues10aSet)
{
// embeddedValues has no pre constraints
embeddedValues10a = handleGetEmbeddedValues();
// embeddedValues has no post constraints
this.__embeddedValues10a = embeddedValues10a;
if (isMetafacadePropertyCachingEnabled())
{
this.__embeddedValues10aSet = true;
}
}
return embeddedValues10a;
}
/**
* @see Entity#getIdentifierName()
* @return String
*/
protected abstract String handleGetIdentifierName();
private String __identifierName11a;
private boolean __identifierName11aSet = false;
/**
*
* The name of the identifier. If composite identifier add the Pk
* sufix. If not composite returns the atribute name of the
* identifier.
*
* @return (String)handleGetIdentifierName()
*/
public final String getIdentifierName()
{
String identifierName11a = this.__identifierName11a;
if (!this.__identifierName11aSet)
{
// identifierName has no pre constraints
identifierName11a = handleGetIdentifierName();
// identifierName has no post constraints
this.__identifierName11a = identifierName11a;
if (isMetafacadePropertyCachingEnabled())
{
this.__identifierName11aSet = true;
}
}
return identifierName11a;
}
/**
* @see Entity#getFullyQualifiedIdentifierTypeName()
* @return String
*/
protected abstract String handleGetFullyQualifiedIdentifierTypeName();
private String __fullyQualifiedIdentifierTypeName12a;
private boolean __fullyQualifiedIdentifierTypeName12aSet = false;
/**
*
* The full name of the type of the identifier. If composite
* identifier add the PK sufix to the class name. If not, retorns
* the fully qualified name of the identifier.
*
* @return (String)handleGetFullyQualifiedIdentifierTypeName()
*/
public final String getFullyQualifiedIdentifierTypeName()
{
String fullyQualifiedIdentifierTypeName12a = this.__fullyQualifiedIdentifierTypeName12a;
if (!this.__fullyQualifiedIdentifierTypeName12aSet)
{
// fullyQualifiedIdentifierTypeName has no pre constraints
fullyQualifiedIdentifierTypeName12a = handleGetFullyQualifiedIdentifierTypeName();
// fullyQualifiedIdentifierTypeName has no post constraints
this.__fullyQualifiedIdentifierTypeName12a = fullyQualifiedIdentifierTypeName12a;
if (isMetafacadePropertyCachingEnabled())
{
this.__fullyQualifiedIdentifierTypeName12aSet = true;
}
}
return fullyQualifiedIdentifierTypeName12a;
}
/**
* @see Entity#getIdentifierTypeName()
* @return String
*/
protected abstract String handleGetIdentifierTypeName();
private String __identifierTypeName13a;
private boolean __identifierTypeName13aSet = false;
/**
*
* The name of the type of the identifier. If composite identifier
* add the PK sufix to the class name. If not, retorns the name of
* the identifier.
*
* @return (String)handleGetIdentifierTypeName()
*/
public final String getIdentifierTypeName()
{
String identifierTypeName13a = this.__identifierTypeName13a;
if (!this.__identifierTypeName13aSet)
{
// identifierTypeName has no pre constraints
identifierTypeName13a = handleGetIdentifierTypeName();
// identifierTypeName has no post constraints
this.__identifierTypeName13a = identifierTypeName13a;
if (isMetafacadePropertyCachingEnabled())
{
this.__identifierTypeName13aSet = true;
}
}
return identifierTypeName13a;
}
/**
* @see Entity#getIdentifierGetterName()
* @return String
*/
protected abstract String handleGetIdentifierGetterName();
private String __identifierGetterName14a;
private boolean __identifierGetterName14aSet = false;
/**
*
* The getter name of the identifier.
*
* @return (String)handleGetIdentifierGetterName()
*/
public final String getIdentifierGetterName()
{
String identifierGetterName14a = this.__identifierGetterName14a;
if (!this.__identifierGetterName14aSet)
{
// identifierGetterName has no pre constraints
identifierGetterName14a = handleGetIdentifierGetterName();
// identifierGetterName has no post constraints
this.__identifierGetterName14a = identifierGetterName14a;
if (isMetafacadePropertyCachingEnabled())
{
this.__identifierGetterName14aSet = true;
}
}
return identifierGetterName14a;
}
/**
* @see Entity#getIdentifierSetterName()
* @return String
*/
protected abstract String handleGetIdentifierSetterName();
private String __identifierSetterName15a;
private boolean __identifierSetterName15aSet = false;
/**
*
* The setter name of the identifier.
*
* @return (String)handleGetIdentifierSetterName()
*/
public final String getIdentifierSetterName()
{
String identifierSetterName15a = this.__identifierSetterName15a;
if (!this.__identifierSetterName15aSet)
{
// identifierSetterName has no pre constraints
identifierSetterName15a = handleGetIdentifierSetterName();
// identifierSetterName has no post constraints
this.__identifierSetterName15a = identifierSetterName15a;
if (isMetafacadePropertyCachingEnabled())
{
this.__identifierSetterName15aSet = true;
}
}
return identifierSetterName15a;
}
// ---------------- business methods ----------------------
/**
* Method to be implemented in descendants
*
* Gets the attributes as a list within an operation call,
* optionally including the type names and the identifier
* attributes.
*
* @param withIdentifiers
* @return String
*/
protected abstract String handleGetOperationCallFromAttributes(boolean withIdentifiers);
/**
*
* Gets the attributes as a list within an operation call,
* optionally including the type names and the identifier
* attributes.
*
* @param withIdentifiers boolean
*
* True if you want to include identifiers within the attribute
* list, false otherwise.
*
* @return handleGetOperationCallFromAttributes(withIdentifiers)
*/
public String getOperationCallFromAttributes(boolean withIdentifiers)
{
// getOperationCallFromAttributes has no pre constraints
String returnValue = handleGetOperationCallFromAttributes(withIdentifiers);
// getOperationCallFromAttributes has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Gets all identifiers for an entity. If 'follow' is true, and if
* no identifiers can be found on the entity, a search up the
* inheritance chain will be performed, and the identifiers from
* the first super class having them will be used. If no
* identifiers exist, a default identifier will be created if the
* allowDefaultIdentifiers property is set to true.
*
* @param follow
* @return Collection
*/
protected abstract Collection handleGetIdentifiers(boolean follow);
/**
*
* Gets all identifiers for an entity. If 'follow' is true, and if
* no identifiers can be found on the entity, a search up the
* inheritance chain will be performed, and the identifiers from
* the first super class having them will be used. If no
* identifiers exist, a default identifier will be created if the
* allowDefaultIdentifiers property is set to true.
*
* @param follow boolean
* @return handleGetIdentifiers(follow)
*/
public Collection getIdentifiers(boolean follow)
{
// getIdentifiers has no pre constraints
Collection returnValue = handleGetIdentifiers(follow);
// getIdentifiers has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Gets the attributes as a list within an operation call. If
* 'withTypeNames' is true, it will include the type names, if
* 'withIdentifiers' is true it will include the identifiers. If
* 'follow' is true it will follow the inheritance hierarchy and
* get the attributes of the super class as well.
*
* @param withIdentifiers
* @param follow
* @return String
*/
protected abstract String handleGetOperationCallFromAttributes(boolean withIdentifiers, boolean follow);
/**
*
* Gets the attributes as a list within an operation call. If
* 'withTypeNames' is true, it will include the type names, if
* 'withIdentifiers' is true it will include the identifiers. If
* 'follow' is true it will follow the inheritance hierarchy and
* get the attributes of the super class as well.
*
* @param withIdentifiers boolean
*
* If true, identifiers will be included in the list.
*
* @param follow boolean
*
* If this is true, the inheritance hierarchy will be followed when
* retrieving all attrbutes.
*
* @return handleGetOperationCallFromAttributes(withIdentifiers, follow)
*/
public String getOperationCallFromAttributes(boolean withIdentifiers, boolean follow)
{
// getOperationCallFromAttributes has no pre constraints
String returnValue = handleGetOperationCallFromAttributes(withIdentifiers, follow);
// getOperationCallFromAttributes has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Returns all attributes that are specified as 'required' in the
* model. If 'follow' is true, then required attributes in super
* classes will also be returned, if false, just the ones directly
* on the entity will be returned. If 'withIdentifiers' is true,
* the identifiers will be include, if false, no identifiers will
* be included.
*
* @param follow
* @param withIdentifiers
* @return Collection
*/
protected abstract Collection handleGetRequiredAttributes(boolean follow, boolean withIdentifiers);
/**
*
* Returns all attributes that are specified as 'required' in the
* model. If 'follow' is true, then required attributes in super
* classes will also be returned, if false, just the ones directly
* on the entity will be returned. If 'withIdentifiers' is true,
* the identifiers will be include, if false, no identifiers will
* be included.
*
* @param follow boolean
*
* Whether or not to follow the inheritance hierarchy when
* retreiving the attributes. If true, the required attributes in
* super classes will also be returned, if false, just the ones
* directly on the entity will be returned.
*
* @param withIdentifiers boolean
*
* Whether or not to include identifiers in the list of required
* attributes.
*
* @return handleGetRequiredAttributes(follow, withIdentifiers)
*/
public Collection getRequiredAttributes(boolean follow, boolean withIdentifiers)
{
// getRequiredAttributes has no pre constraints
Collection returnValue = handleGetRequiredAttributes(follow, withIdentifiers);
// getRequiredAttributes has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Gets all required properties for this entity. These consist of
* any required attributes as well as navigable associations that
* are marked as 'required'. If 'follow' is true, then the
* inheritance hierchy will be followed and all required properties
* from super classes will be included as well.
*
*
* If 'withIdentifiers' is true, the identifiers will be include,
* if false, no identifiers will be included.
*
* @param follow
* @param withIdentifiers
* @return Collection
*/
protected abstract Collection handleGetRequiredProperties(boolean follow, boolean withIdentifiers);
/**
*
* Gets all required properties for this entity. These consist of
* any required attributes as well as navigable associations that
* are marked as 'required'. If 'follow' is true, then the
* inheritance hierchy will be followed and all required properties
* from super classes will be included as well.
*
*
* If 'withIdentifiers' is true, the identifiers will be include,
* if false, no identifiers will be included.
*
* @param follow boolean
*
* Whether or not to follow the inheritance hierarchy when
* retreiving the properties. If true, the required propertis in
* super classes will also be returned, if false, just the ones
* directly on the entity will be returned.
*
* @param withIdentifiers boolean
*
* Whether or not to include identifiers in the list of required
* attributes.
*
* @return handleGetRequiredProperties(follow, withIdentifiers)
*/
public Collection getRequiredProperties(boolean follow, boolean withIdentifiers)
{
// getRequiredProperties has no pre constraints
Collection returnValue = handleGetRequiredProperties(follow, withIdentifiers);
// getRequiredProperties has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Gets all attributes of the entity, and optionally retieves the
* super entities attributes as well as excludes the entity's
* identifiers if 'withIdentifiers' is set to false.
*
* @param follow
* @param withIdentifiers
* @return Collection
*/
protected abstract Collection handleGetAttributes(boolean follow, boolean withIdentifiers);
/**
*
* Gets all attributes of the entity, and optionally retieves the
* super entities attributes as well as excludes the entity's
* identifiers if 'withIdentifiers' is set to false.
*
* @param follow boolean
*
* Whether or not to follow the inheritance hierarchy when
* retreiving attributes.
*
* @param withIdentifiers boolean
*
* Whether or not to include identifiers in the returned
* attributes.
*
* @return handleGetAttributes(follow, withIdentifiers)
*/
public Collection getAttributes(boolean follow, boolean withIdentifiers)
{
// getAttributes has no pre constraints
Collection returnValue = handleGetAttributes(follow, withIdentifiers);
// getAttributes has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Gets a comma separated list of attribute types. If 'follow' is
* true, will travel up the inheritance hierarchy to include
* attributes in parent entities as well. If 'withIdentifiers' is
* true, will include identifiers.
*
* @param follow
* @param withIdentifiers
* @return String
*/
protected abstract String handleGetAttributeTypeList(boolean follow, boolean withIdentifiers);
/**
*
* Gets a comma separated list of attribute types. If 'follow' is
* true, will travel up the inheritance hierarchy to include
* attributes in parent entities as well. If 'withIdentifiers' is
* true, will include identifiers.
*
* @param follow boolean
*
* Whether or not to 'follow' the inheritance hierarchy.
*
* @param withIdentifiers boolean
*
* Whether or not to include identifiers.
*
* @return handleGetAttributeTypeList(follow, withIdentifiers)
*/
public String getAttributeTypeList(boolean follow, boolean withIdentifiers)
{
// getAttributeTypeList has no pre constraints
String returnValue = handleGetAttributeTypeList(follow, withIdentifiers);
// getAttributeTypeList has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Gets a comma separated list of attribute names. If 'follow' is
* true, will travel up the inheritance hiearchy to include
* attributes in parent entities as well. If 'withIdentifiers' is
* true, will include identifiers.
*
* @param follow
* @param withIdentifiers
* @return String
*/
protected abstract String handleGetAttributeNameList(boolean follow, boolean withIdentifiers);
/**
*
* Gets a comma separated list of attribute names. If 'follow' is
* true, will travel up the inheritance hiearchy to include
* attributes in parent entities as well. If 'withIdentifiers' is
* true, will include identifiers.
*
* @param follow boolean
*
* Whether or not to 'follow' the inheritance hierarchy.
*
* @param withIdentifiers boolean
*
* Whether or not to include identifiers in the returned
* attributes.
*
* @return handleGetAttributeNameList(follow, withIdentifiers)
*/
public String getAttributeNameList(boolean follow, boolean withIdentifiers)
{
// getAttributeNameList has no pre constraints
String returnValue = handleGetAttributeNameList(follow, withIdentifiers);
// getAttributeNameList has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Gets a comma separated list of attribute types with are
* required. If 'follow' is true, will travel up the inheritance
* hierarchy to include attributes in parent entities as well. If
* 'withIdentifiers' is true, will include identifiers.
*
* @param follow
* @param withIdentifiers
* @return String
*/
protected abstract String handleGetRequiredAttributeTypeList(boolean follow, boolean withIdentifiers);
/**
*
* Gets a comma separated list of attribute types with are
* required. If 'follow' is true, will travel up the inheritance
* hierarchy to include attributes in parent entities as well. If
* 'withIdentifiers' is true, will include identifiers.
*
* @param follow boolean
*
* Whether or not to 'follow' the inheritance hierarchy.
*
* @param withIdentifiers boolean
*
* Whether or not to include identifiers in the list of required
* attributes.
*
* @return handleGetRequiredAttributeTypeList(follow, withIdentifiers)
*/
public String getRequiredAttributeTypeList(boolean follow, boolean withIdentifiers)
{
// getRequiredAttributeTypeList has no pre constraints
String returnValue = handleGetRequiredAttributeTypeList(follow, withIdentifiers);
// getRequiredAttributeTypeList has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Gets a comma separated list of required attribute names. If
* 'follow' is true, will travel up the inheritance hierarchy to
* include attributes in parent entities as well. If
* 'withIdentifiers' is true, will include identifiers.
*
* @param follow
* @param withIdentifiers
* @return String
*/
protected abstract String handleGetRequiredAttributeNameList(boolean follow, boolean withIdentifiers);
/**
*
* Gets a comma separated list of required attribute names. If
* 'follow' is true, will travel up the inheritance hierarchy to
* include attributes in parent entities as well. If
* 'withIdentifiers' is true, will include identifiers.
*
* @param follow boolean
*
* Whether or not to follow the inheritance hierarchy when
* retreiving the attributes. If true, the required attributes in
* super classes will also be used, if false, just the ones
* directly on the entity will be added to the list.
*
* @param withIdentifiers boolean
*
* Whether or not to include identifiers in the list of required
* attribute names.
*
* @return handleGetRequiredAttributeNameList(follow, withIdentifiers)
*/
public String getRequiredAttributeNameList(boolean follow, boolean withIdentifiers)
{
// getRequiredAttributeNameList has no pre constraints
String returnValue = handleGetRequiredAttributeNameList(follow, withIdentifiers);
// getRequiredAttributeNameList has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Gets all query operations for an entity. If 'follow' is true,
* and if no query operations can be found on the entity, a search
* up the inheritance chain will be performed, and the identifiers
* from the first super class having them will be used. If no
* identifiers exist, a default identifier will be created if the
* allowDefaultIdentifiers property is set to true.
*
* @param follow
* @return Collection
*/
protected abstract Collection handleGetQueryOperations(boolean follow);
/**
*
* Gets all query operations for an entity. If 'follow' is true,
* and if no query operations can be found on the entity, a search
* up the inheritance chain will be performed, and the identifiers
* from the first super class having them will be used. If no
* identifiers exist, a default identifier will be created if the
* allowDefaultIdentifiers property is set to true.
*
* @param follow boolean
* @return handleGetQueryOperations(follow)
*/
public Collection getQueryOperations(boolean follow)
{
// getQueryOperations has no pre constraints
Collection returnValue = handleGetQueryOperations(follow);
// getQueryOperations has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Creates a comma separated list of the required property names.
*
* @param follow
* @param withIdentifiers
* @return String
*/
protected abstract String handleGetRequiredPropertyNameList(boolean follow, boolean withIdentifiers);
/**
*
* Creates a comma separated list of the required property names.
*
* @param follow boolean
*
* Whether or not to follow the inheritance hierarchy when
* retrieving the properties.
*
* @param withIdentifiers boolean
*
* Whether or not to include identifiers in the list.
*
* @return handleGetRequiredPropertyNameList(follow, withIdentifiers)
*/
public String getRequiredPropertyNameList(boolean follow, boolean withIdentifiers)
{
// getRequiredPropertyNameList has no pre constraints
String returnValue = handleGetRequiredPropertyNameList(follow, withIdentifiers);
// getRequiredPropertyNameList has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* A comma separated list of the required property types.
*
* @param follow
* @param withIdentifiers
* @return String
*/
protected abstract String handleGetRequiredPropertyTypeList(boolean follow, boolean withIdentifiers);
/**
*
* A comma separated list of the required property types.
*
* @param follow boolean
*
* Whether or not to follow the inheritance hierarchy
*
* @param withIdentifiers boolean
*
* Whether or not to include identifiers in the list.
*
* @return handleGetRequiredPropertyTypeList(follow, withIdentifiers)
*/
public String getRequiredPropertyTypeList(boolean follow, boolean withIdentifiers)
{
// getRequiredPropertyTypeList has no pre constraints
String returnValue = handleGetRequiredPropertyTypeList(follow, withIdentifiers);
// getRequiredPropertyTypeList has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Gets all properties of this entity, this includes the attributes
* and navigable association ends of the entity. The 'follow' flag
* indcates whether or not the inheritance hierarchy should be
* followed when getting all the properties. The 'withIdentifiers'
* flag indicates whether or not identifiers should be included in
* the collection of properties.
*
* @param follow
* @param withIdentifiers
* @return Collection
*/
protected abstract Collection handleGetProperties(boolean follow, boolean withIdentifiers);
/**
*
* Gets all properties of this entity, this includes the attributes
* and navigable association ends of the entity. The 'follow' flag
* indcates whether or not the inheritance hierarchy should be
* followed when getting all the properties. The 'withIdentifiers'
* flag indicates whether or not identifiers should be included in
* the collection of properties.
*
* @param follow boolean
* @param withIdentifiers boolean
* @return handleGetProperties(follow, withIdentifiers)
*/
public Collection getProperties(boolean follow, boolean withIdentifiers)
{
// getProperties has no pre constraints
Collection returnValue = handleGetProperties(follow, withIdentifiers);
// getProperties has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Gets all the associationEnds of this entity marked with the
* identifiers stereotype.
*
* @return Collection
*/
protected abstract Collection handleGetIdentifierAssociationEnds();
/**
*
* Gets all the associationEnds of this entity marked with the
* identifiers stereotype.
*
* @return handleGetIdentifierAssociationEnds()
*/
public Collection getIdentifierAssociationEnds()
{
// getIdentifierAssociationEnds has no pre constraints
Collection returnValue = handleGetIdentifierAssociationEnds();
// getIdentifierAssociationEnds has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Gets all attributes of the entity, and optionally retieves the
* super entities attributes as well as excludes the entity's
* identifiers if 'withIdentifiers' is set to false and exclude
* derived attributes if 'withDerived' is set to false.
*
* @param follow
* @param withIdentifiers
* @param withDerived
* @return Collection
*/
protected abstract Collection handleGetAttributes(boolean follow, boolean withIdentifiers, boolean withDerived);
/**
*
* Gets all attributes of the entity, and optionally retieves the
* super entities attributes as well as excludes the entity's
* identifiers if 'withIdentifiers' is set to false and exclude
* derived attributes if 'withDerived' is set to false.
*
* @param follow boolean
*
* Whether or not to follow the inheritance hierarchy when
* retreiving attributes.
*
* @param withIdentifiers boolean
*
* Whether or not to include identifiers in the returned
* attributes.
*
* @param withDerived boolean
*
* Whether or not to include derived attributes in the returned
* attributes.
*
* @return handleGetAttributes(follow, withIdentifiers, withDerived)
*/
public Collection getAttributes(boolean follow, boolean withIdentifiers, boolean withDerived)
{
// getAttributes has no pre constraints
Collection returnValue = handleGetAttributes(follow, withIdentifiers, withDerived);
// getAttributes has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Gets a comma separated list of attribute names. If 'follow' is
* true, will travel up the inheritance hiearchy to include
* attributes in parent entities as well. If 'withIdentifiers' is
* true, will include identifiers and if 'withDerived' is set to
* true, will include derived attributes.
*
* @param follow
* @param withIdentifiers
* @param withDerived
* @return String
*/
protected abstract String handleGetAttributeNameList(boolean follow, boolean withIdentifiers, boolean withDerived);
/**
*
* Gets a comma separated list of attribute names. If 'follow' is
* true, will travel up the inheritance hiearchy to include
* attributes in parent entities as well. If 'withIdentifiers' is
* true, will include identifiers and if 'withDerived' is set to
* true, will include derived attributes.
*
* @param follow boolean
*
* Whether or not to 'follow' the inheritance hierarchy.
*
* @param withIdentifiers boolean
*
* Whether or not to include identifiers in the returned
* attributes.
*
* @param withDerived boolean
*
* Whether or not to include derived attributes in the returned
* attributes.
*
* @return handleGetAttributeNameList(follow, withIdentifiers, withDerived)
*/
public String getAttributeNameList(boolean follow, boolean withIdentifiers, boolean withDerived)
{
// getAttributeNameList has no pre constraints
String returnValue = handleGetAttributeNameList(follow, withIdentifiers, withDerived);
// getAttributeNameList has no post constraints
return returnValue;
}
// ------------- associations ------------------
/**
*
* @return (Collection)handleGetEntityReferences()
*/
public final Collection getEntityReferences()
{
Collection getEntityReferences1r = null;
// entity has no pre constraints
Collection result = handleGetEntityReferences();
List shieldedResult = this.shieldedElements(result);
try
{
getEntityReferences1r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
EntityLogic.logger.warn("incorrect metafacade cast for EntityLogic.getEntityReferences Collection " + result + ": " + shieldedResult);
}
// entity has no post constraints
return getEntityReferences1r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetEntityReferences();
/**
*
* @return (EntityAssociationEnd)handleGetParentEnd()
*/
public final EntityAssociationEnd getParentEnd()
{
EntityAssociationEnd getParentEnd2r = null;
// entity has no pre constraints
Object result = handleGetParentEnd();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getParentEnd2r = (EntityAssociationEnd)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
EntityLogic.logger.warn("incorrect metafacade cast for EntityLogic.getParentEnd EntityAssociationEnd " + result + ": " + shieldedResult);
}
// entity has no post constraints
return getParentEnd2r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetParentEnd();
/**
*
* @return (Collection)handleGetChildEnds()
*/
public final Collection getChildEnds()
{
Collection getChildEnds3r = null;
// entity has no pre constraints
Collection result = handleGetChildEnds();
List shieldedResult = this.shieldedElements(result);
try
{
getChildEnds3r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
EntityLogic.logger.warn("incorrect metafacade cast for EntityLogic.getChildEnds Collection " + result + ": " + shieldedResult);
}
// entity has no post constraints
return getChildEnds3r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetChildEnds();
/**
*
* @return (Collection)handleGetQueryOperations()
*/
public final Collection getQueryOperations()
{
Collection getQueryOperations4r = null;
// entity has no pre constraints
Collection result = handleGetQueryOperations();
List shieldedResult = this.shieldedElements(result);
try
{
getQueryOperations4r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
EntityLogic.logger.warn("incorrect metafacade cast for EntityLogic.getQueryOperations Collection " + result + ": " + shieldedResult);
}
// entity has no post constraints
return getQueryOperations4r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetQueryOperations();
/**
*
* @return (Collection)handleGetBusinessOperations()
*/
public final Collection getBusinessOperations()
{
Collection getBusinessOperations5r = null;
// entity has no pre constraints
Collection result = handleGetBusinessOperations();
List shieldedResult = this.shieldedElements(result);
try
{
getBusinessOperations5r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
EntityLogic.logger.warn("incorrect metafacade cast for EntityLogic.getBusinessOperations Collection " + result + ": " + shieldedResult);
}
// entity has no post constraints
return getBusinessOperations5r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetBusinessOperations();
/**
*
* @return (Collection)handleGetIdentifiers()
*/
public final Collection getIdentifiers()
{
Collection getIdentifiers6r = null;
// entity has no pre constraints
Collection result = handleGetIdentifiers();
List shieldedResult = this.shieldedElements(result);
try
{
getIdentifiers6r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
EntityLogic.logger.warn("incorrect metafacade cast for EntityLogic.getIdentifiers Collection " + result + ": " + shieldedResult);
}
// entity has no post constraints
return getIdentifiers6r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetIdentifiers();
/**
*
* @return (Collection)handleGetAllEntityReferences()
*/
public final Collection getAllEntityReferences()
{
Collection getAllEntityReferences7r = null;
// entity has no pre constraints
Collection result = handleGetAllEntityReferences();
List shieldedResult = this.shieldedElements(result);
try
{
getAllEntityReferences7r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
EntityLogic.logger.warn("incorrect metafacade cast for EntityLogic.getAllEntityReferences Collection " + result + ": " + shieldedResult);
}
// entity has no post constraints
return getAllEntityReferences7r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetAllEntityReferences();
/**
* Constraint: org::andromda::metafacades::uml::Entity::entity must have at least one primary key
* Error: Each entity must have at least one identifier defined.
* OCL: context Entity inv: identifiersPresent
* Constraint: org::andromda::metafacades::uml::Entity::entity must have all public attributes
* Error: All attributes on an entity must have a public visibility.
* OCL: context Entity
inv : attributes -> forAll(visibility = 'public')
* Constraint: org::andromda::metafacades::uml::Entity::entity must have public associations
* Error: All navigable connecting association ends must have a public visibility.
* OCL: context Entity
inv : associationEnds -> forAll(otherEnd.navigable implies otherEnd.visibility = 'public')
* Constraint: org::andromda::metafacades::uml::Entity::entities can only generalize other entities
* Error: An entity can only generalize another entity.
* OCL: context Entity
inv : generalization -> notEmpty() implies generalization.oclIsKindOf(Entity)
* Constraint: org::andromda::metafacades::uml::Entity::entities can only specialize other entites
* Error: An entity can only specialize another entity.
* OCL: context Entity inv : specializations -> notEmpty() implies specializations -> forAll(oclIsKindOf(Entity))
* @param validationMessages Collection
* @see ClassifierFacadeLogicImpl#validateInvariants(Collection validationMessages)
*/
@Override
public void validateInvariants(Collection validationMessages)
{
super.validateInvariants(validationMessages);
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure(OCLIntrospector.invoke(contextElement,"identifiersPresent"));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::Entity::entity must have at least one primary key",
"Each entity must have at least one identifier defined."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::Entity::entity must have at least one primary key' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure(OCLCollections.forAll(OCLIntrospector.invoke(contextElement,"attributes"),new Predicate(){public boolean evaluate(Object object){return Boolean.valueOf(String.valueOf(OCLExpressions.equal(OCLIntrospector.invoke(object,"visibility"),"public"))).booleanValue();}}));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::Entity::entity must have all public attributes",
"All attributes on an entity must have a public visibility."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::Entity::entity must have all public attributes' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure(OCLCollections.forAll(OCLIntrospector.invoke(contextElement,"associationEnds"),new Predicate(){public boolean evaluate(Object object){return Boolean.valueOf(String.valueOf((Boolean.valueOf(String.valueOf(OCLIntrospector.invoke(object,"otherEnd.navigable"))).booleanValue()?OCLExpressions.equal(OCLIntrospector.invoke(object,"otherEnd.visibility"),"public"):true))).booleanValue();}}));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::Entity::entity must have public associations",
"All navigable connecting association ends must have a public visibility."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::Entity::entity must have public associations' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure((Boolean.valueOf(String.valueOf(OCLCollections.notEmpty(OCLIntrospector.invoke(contextElement,"generalization")))).booleanValue()?OCLIntrospector.invoke(contextElement,"generalization") instanceof Entity:true));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::Entity::entities can only generalize other entities",
"An entity can only generalize another entity."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::Entity::entities can only generalize other entities' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure((Boolean.valueOf(String.valueOf(OCLCollections.notEmpty(OCLIntrospector.invoke(contextElement,"specializations")))).booleanValue()?OCLCollections.forAll(OCLIntrospector.invoke(contextElement,"specializations"),new Predicate(){public boolean evaluate(Object object){return Boolean.valueOf(String.valueOf(object instanceof Entity)).booleanValue();}}):true));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::Entity::entities can only specialize other entites",
"An entity can only specialize another entity."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::Entity::entities can only specialize other entites' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
}
}