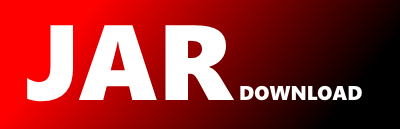
org.andromda.metafacades.emf.uml22.FrontEndActionLogic Maven / Gradle / Ivy
Show all versions of andromda-metafacades-emf-uml22 Show documentation
// license-header java merge-point
//
// Attention: generated code (by MetafacadeLogic.vsl) - do not modify!
//
package org.andromda.metafacades.emf.uml22;
import java.util.Collection;
import java.util.List;
import org.andromda.core.metafacade.MetafacadeBase;
import org.andromda.core.metafacade.ModelValidationMessage;
import org.andromda.metafacades.uml.FrontEndAction;
import org.andromda.metafacades.uml.FrontEndActionState;
import org.andromda.metafacades.uml.FrontEndController;
import org.andromda.metafacades.uml.FrontEndControllerOperation;
import org.andromda.metafacades.uml.FrontEndForward;
import org.andromda.metafacades.uml.FrontEndParameter;
import org.andromda.metafacades.uml.FrontEndView;
import org.andromda.metafacades.uml.ParameterFacade;
import org.andromda.metafacades.uml.StateVertexFacade;
import org.andromda.translation.ocl.validation.OCLCollections;
import org.andromda.translation.ocl.validation.OCLIntrospector;
import org.andromda.translation.ocl.validation.OCLResultEnsurer;
import org.apache.commons.collections.Transformer;
import org.apache.log4j.Logger;
/**
*
* Represents a "front-end" action. An action is some action that
* is taken when a front-end even occurs.
*
* MetafacadeLogic for FrontEndAction
*
* @see FrontEndAction
*/
public abstract class FrontEndActionLogic
extends FrontEndForwardLogicImpl
implements FrontEndAction
{
/**
* The underlying UML object
* @see Object
*/
protected Object metaObject;
/** Create Metafacade implementation instance using the MetafacadeFactory from the context
* @param metaObjectIn
* @param context
*/
protected FrontEndActionLogic(Object metaObjectIn, String context)
{
super(metaObjectIn, getContext(context));
this.metaObject = metaObjectIn;
}
/**
* The logger instance.
*/
private static final Logger logger = Logger.getLogger(FrontEndActionLogic.class);
/**
* Gets the context for this metafacade logic instance.
* @param context String. Set to FrontEndAction if null
* @return context String
*/
private static String getContext(String context)
{
if (context == null)
{
context = "org.andromda.metafacades.uml.FrontEndAction";
}
return context;
}
/** Reset context only for non-root metafacades
* @param context
*/
@Override
public void resetMetafacadeContext(String context)
{
if (!this.contextRoot) // reset context only for non-root metafacades
{
context = getContext(context); // to have same value as in original constructor call
setMetafacadeContext (context);
}
}
/**
* @return boolean true always
* @see FrontEndAction
*/
public boolean isFrontEndActionMetaType()
{
return true;
}
// --------------- attributes ---------------------
/**
* @see FrontEndAction#isUseCaseStart()
* @return boolean
*/
protected abstract boolean handleIsUseCaseStart();
private boolean __useCaseStart1a;
private boolean __useCaseStart1aSet = false;
/**
*
* Indicates if this action represents the beginning of the
* front-end use case or not.
*
* @return (boolean)handleIsUseCaseStart()
*/
public final boolean isUseCaseStart()
{
boolean useCaseStart1a = this.__useCaseStart1a;
if (!this.__useCaseStart1aSet)
{
// useCaseStart has no pre constraints
useCaseStart1a = handleIsUseCaseStart();
// useCaseStart has no post constraints
this.__useCaseStart1a = useCaseStart1a;
if (isMetafacadePropertyCachingEnabled())
{
this.__useCaseStart1aSet = true;
}
}
return useCaseStart1a;
}
// ---------------- business methods ----------------------
/**
* Method to be implemented in descendants
*
* Finds the parameter on this action having the given name, if no
* parameter is found, null is returned instead.
*
* @param name
* @return ParameterFacade
*/
protected abstract ParameterFacade handleFindParameter(String name);
/**
*
* Finds the parameter on this action having the given name, if no
* parameter is found, null is returned instead.
*
* @param name String
*
* The name of the parameter to find on the owner action.
*
* @return handleFindParameter(name)
*/
public ParameterFacade findParameter(String name)
{
// findParameter has no pre constraints
ParameterFacade returnValue = handleFindParameter(name);
// findParameter has no post constraints
return returnValue;
}
// ------------- associations ------------------
private List __getParameters1r;
private boolean __getParameters1rSet = false;
/**
*
* The action to which this parameter belongs (if it belongs to an
* action), otherwise it returns null.
*
* @return (List)handleGetParameters()
*/
public final List getParameters()
{
List getParameters1r = this.__getParameters1r;
if (!this.__getParameters1rSet)
{
// action has no pre constraints
List result = handleGetParameters();
List shieldedResult = this.shieldedElements(result);
try
{
getParameters1r = (List)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndActionLogic.logger.warn("incorrect metafacade cast for FrontEndActionLogic.getParameters List " + result + ": " + shieldedResult);
}
// action has no post constraints
this.__getParameters1r = getParameters1r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getParameters1rSet = true;
}
}
return getParameters1r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return List
*/
protected abstract List handleGetParameters();
private List __getDeferredOperations3r;
private boolean __getDeferredOperations3rSet = false;
/**
*
* All those actions that contain at least one front-end action
* state that is deferring to this operation.
*
* @return (List)handleGetDeferredOperations()
*/
public final List getDeferredOperations()
{
List getDeferredOperations3r = this.__getDeferredOperations3r;
if (!this.__getDeferredOperations3rSet)
{
// deferringActions has no pre constraints
List result = handleGetDeferredOperations();
List shieldedResult = this.shieldedElements(result);
try
{
getDeferredOperations3r = (List)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndActionLogic.logger.warn("incorrect metafacade cast for FrontEndActionLogic.getDeferredOperations List " + result + ": " + shieldedResult);
}
// deferringActions has no post constraints
this.__getDeferredOperations3r = getDeferredOperations3r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getDeferredOperations3rSet = true;
}
}
return getDeferredOperations3r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return List
*/
protected abstract List handleGetDeferredOperations();
private FrontEndController __getController4r;
private boolean __getController4rSet = false;
/**
*
* All actions that defer to at least one operation of this
* controller.
*
* @return (FrontEndController)handleGetController()
*/
public final FrontEndController getController()
{
FrontEndController getController4r = this.__getController4r;
if (!this.__getController4rSet)
{
// deferringActions has no pre constraints
Object result = handleGetController();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getController4r = (FrontEndController)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndActionLogic.logger.warn("incorrect metafacade cast for FrontEndActionLogic.getController FrontEndController " + result + ": " + shieldedResult);
}
// deferringActions has no post constraints
this.__getController4r = getController4r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getController4rSet = true;
}
}
return getController4r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetController();
private List __getActionStates5r;
private boolean __getActionStates5rSet = false;
/**
*
* The actions that pass through this action state.
*
* @return (List)handleGetActionStates()
*/
public final List getActionStates()
{
List getActionStates5r = this.__getActionStates5r;
if (!this.__getActionStates5rSet)
{
// containerActions has no pre constraints
List result = handleGetActionStates();
List shieldedResult = this.shieldedElements(result);
try
{
getActionStates5r = (List)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndActionLogic.logger.warn("incorrect metafacade cast for FrontEndActionLogic.getActionStates List " + result + ": " + shieldedResult);
}
// containerActions has no post constraints
this.__getActionStates5r = getActionStates5r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getActionStates5rSet = true;
}
}
return getActionStates5r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return List
*/
protected abstract List handleGetActionStates();
private List __getDecisionTransitions7r;
private boolean __getDecisionTransitions7rSet = false;
/**
*
* @return (List)handleGetDecisionTransitions()
*/
public final List getDecisionTransitions()
{
List getDecisionTransitions7r = this.__getDecisionTransitions7r;
if (!this.__getDecisionTransitions7rSet)
{
// frontEndAction has no pre constraints
List result = handleGetDecisionTransitions();
List shieldedResult = this.shieldedElements(result);
try
{
getDecisionTransitions7r = (List)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndActionLogic.logger.warn("incorrect metafacade cast for FrontEndActionLogic.getDecisionTransitions List " + result + ": " + shieldedResult);
}
// frontEndAction has no post constraints
this.__getDecisionTransitions7r = getDecisionTransitions7r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getDecisionTransitions7rSet = true;
}
}
return getDecisionTransitions7r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return List
*/
protected abstract List handleGetDecisionTransitions();
private List __getTransitions10r;
private boolean __getTransitions10rSet = false;
/**
*
* The front-end actions directly containing this front-end
* forward.
*
* @return (List)handleGetTransitions()
*/
public final List getTransitions()
{
List getTransitions10r = this.__getTransitions10r;
if (!this.__getTransitions10rSet)
{
// actions has no pre constraints
List result = handleGetTransitions();
List shieldedResult = this.shieldedElements(result);
try
{
getTransitions10r = (List)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndActionLogic.logger.warn("incorrect metafacade cast for FrontEndActionLogic.getTransitions List " + result + ": " + shieldedResult);
}
// actions has no post constraints
this.__getTransitions10r = getTransitions10r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getTransitions10rSet = true;
}
}
return getTransitions10r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return List
*/
protected abstract List handleGetTransitions();
private List __getFormFields11r;
private boolean __getFormFields11rSet = false;
/**
*
* @return (List)handleGetFormFields()
*/
public final List getFormFields()
{
List getFormFields11r = this.__getFormFields11r;
if (!this.__getFormFields11rSet)
{
// frontEndAction has no pre constraints
List result = handleGetFormFields();
List shieldedResult = this.shieldedElements(result);
try
{
getFormFields11r = (List)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndActionLogic.logger.warn("incorrect metafacade cast for FrontEndActionLogic.getFormFields List " + result + ": " + shieldedResult);
}
// frontEndAction has no post constraints
this.__getFormFields11r = getFormFields11r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getFormFields11rSet = true;
}
}
return getFormFields11r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return List
*/
protected abstract List handleGetFormFields();
private List __getActionForwards12r;
private boolean __getActionForwards12rSet = false;
/**
*
* @return (List)handleGetActionForwards()
*/
public final List getActionForwards()
{
List getActionForwards12r = this.__getActionForwards12r;
if (!this.__getActionForwards12rSet)
{
// frontEndAction has no pre constraints
List result = handleGetActionForwards();
List shieldedResult = this.shieldedElements(result);
try
{
getActionForwards12r = (List)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndActionLogic.logger.warn("incorrect metafacade cast for FrontEndActionLogic.getActionForwards List " + result + ": " + shieldedResult);
}
// frontEndAction has no post constraints
this.__getActionForwards12r = getActionForwards12r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getActionForwards12rSet = true;
}
}
return getActionForwards12r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return List
*/
protected abstract List handleGetActionForwards();
private List __getTargetViews13r;
private boolean __getTargetViews13rSet = false;
/**
*
* @return (List)handleGetTargetViews()
*/
public final List getTargetViews()
{
List getTargetViews13r = this.__getTargetViews13r;
if (!this.__getTargetViews13rSet)
{
// frontEndAction has no pre constraints
List result = handleGetTargetViews();
List shieldedResult = this.shieldedElements(result);
try
{
getTargetViews13r = (List)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndActionLogic.logger.warn("incorrect metafacade cast for FrontEndActionLogic.getTargetViews List " + result + ": " + shieldedResult);
}
// frontEndAction has no post constraints
this.__getTargetViews13r = getTargetViews13r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getTargetViews13rSet = true;
}
}
return getTargetViews13r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return List
*/
protected abstract List handleGetTargetViews();
/**
*
* @return (StateVertexFacade)handleGetInput()
*/
public final StateVertexFacade getInput()
{
StateVertexFacade getInput16r = null;
// frontEndAction has no pre constraints
Object result = handleGetInput();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getInput16r = (StateVertexFacade)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndActionLogic.logger.warn("incorrect metafacade cast for FrontEndActionLogic.getInput StateVertexFacade " + result + ": " + shieldedResult);
}
// frontEndAction has no post constraints
return getInput16r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetInput();
/**
* Constraint: org::andromda::metafacades::uml::FrontEndAction::parameters must be unique
* Error: Each front-end action parameter must have a unique name.
* OCL: context FrontEndAction
inv : parameters -> isUnique(name)
* Constraint: org::andromda::metafacades::uml::FrontEndAction::each action must carry a trigger
* Error: Each action transition coming out of a view must have a trigger (the name is sufficient), it is recommended to add a trigger of type 'signal'.
* OCL: context FrontEndAction inv: exitingView implies triggerPresent
* @param validationMessages Collection
* @see FrontEndForwardLogicImpl#validateInvariants(Collection validationMessages)
*/
@Override
public void validateInvariants(Collection validationMessages)
{
super.validateInvariants(validationMessages);
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure(OCLCollections.isUnique(OCLIntrospector.invoke(contextElement,"parameters"),new Transformer(){public Object transform(Object object){return OCLIntrospector.invoke(object,"name");}}));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::FrontEndAction::parameters must be unique",
"Each front-end action parameter must have a unique name."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::FrontEndAction::parameters must be unique' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure((Boolean.valueOf(String.valueOf(Boolean.valueOf(String.valueOf(OCLIntrospector.invoke(contextElement,"exitingView"))).booleanValue())).booleanValue()?Boolean.valueOf(String.valueOf(OCLIntrospector.invoke(contextElement,"triggerPresent"))).booleanValue():true));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::FrontEndAction::each action must carry a trigger",
"Each action transition coming out of a view must have a trigger (the name is sufficient), it is recommended to add a trigger of type 'signal'."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::FrontEndAction::each action must carry a trigger' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
}
}