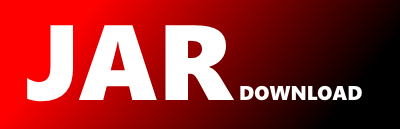
org.andromda.metafacades.emf.uml22.FrontEndActivityGraphLogic Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of andromda-metafacades-emf-uml22 Show documentation
Show all versions of andromda-metafacades-emf-uml22 Show documentation
The Eclipse EMF UML2 v2.X metafacades. This is the set of EMF UML2 2.X metafacades
implementations. These implement the common UML metafacades for .uml model files.
The newest version!
// license-header java merge-point
//
// Attention: generated code (by MetafacadeLogic.vsl) - do not modify!
//
package org.andromda.metafacades.emf.uml22;
import java.util.Collection;
import org.andromda.core.metafacade.MetafacadeBase;
import org.andromda.core.metafacade.ModelValidationMessage;
import org.andromda.metafacades.uml.FrontEndAction;
import org.andromda.metafacades.uml.FrontEndActivityGraph;
import org.andromda.metafacades.uml.FrontEndController;
import org.apache.log4j.Logger;
import org.eclipse.uml2.uml.StateMachine;
/**
*
* Represents the activity graph describing the details of a
* presentation tier or "front end" use case.
*
* MetafacadeLogic for FrontEndActivityGraph
*
* @see FrontEndActivityGraph
*/
public abstract class FrontEndActivityGraphLogic
extends ActivityGraphFacadeLogicImpl
implements FrontEndActivityGraph
{
/**
* The underlying UML object
* @see Object
*/
protected Object metaObject;
/** Create Metafacade implementation instance using the MetafacadeFactory from the context
* @param metaObjectIn
* @param context
*/
protected FrontEndActivityGraphLogic(Object metaObjectIn, String context)
{
super((StateMachine)metaObjectIn, getContext(context));
this.metaObject = metaObjectIn;
}
/**
* The logger instance.
*/
private static final Logger logger = Logger.getLogger(FrontEndActivityGraphLogic.class);
/**
* Gets the context for this metafacade logic instance.
* @param context String. Set to FrontEndActivityGraph if null
* @return context String
*/
private static String getContext(String context)
{
if (context == null)
{
context = "org.andromda.metafacades.uml.FrontEndActivityGraph";
}
return context;
}
/** Reset context only for non-root metafacades
* @param context
*/
@Override
public void resetMetafacadeContext(String context)
{
if (!this.contextRoot) // reset context only for non-root metafacades
{
context = getContext(context); // to have same value as in original constructor call
setMetafacadeContext (context);
}
}
/**
* @return boolean true always
* @see FrontEndActivityGraph
*/
public boolean isFrontEndActivityGraphMetaType()
{
return true;
}
// --------------- attributes ---------------------
/**
* @see FrontEndActivityGraph#isContainedInFrontEndUseCase()
* @return boolean
*/
protected abstract boolean handleIsContainedInFrontEndUseCase();
private boolean __containedInFrontEndUseCase1a;
private boolean __containedInFrontEndUseCase1aSet = false;
/**
*
* True if this element is contained in a FrontEndUseCase.
*
* @return (boolean)handleIsContainedInFrontEndUseCase()
*/
public final boolean isContainedInFrontEndUseCase()
{
boolean containedInFrontEndUseCase1a = this.__containedInFrontEndUseCase1a;
if (!this.__containedInFrontEndUseCase1aSet)
{
// containedInFrontEndUseCase has no pre constraints
containedInFrontEndUseCase1a = handleIsContainedInFrontEndUseCase();
// containedInFrontEndUseCase has no post constraints
this.__containedInFrontEndUseCase1a = containedInFrontEndUseCase1a;
if (isMetafacadePropertyCachingEnabled())
{
this.__containedInFrontEndUseCase1aSet = true;
}
}
return containedInFrontEndUseCase1a;
}
// ------------- associations ------------------
private FrontEndController __getController2r;
private boolean __getController2rSet = false;
/**
*
* @return (FrontEndController)handleGetController()
*/
public final FrontEndController getController()
{
FrontEndController getController2r = this.__getController2r;
if (!this.__getController2rSet)
{
// frontEndActivityGraph has no pre constraints
Object result = handleGetController();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getController2r = (FrontEndController)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndActivityGraphLogic.logger.warn("incorrect metafacade cast for FrontEndActivityGraphLogic.getController FrontEndController " + result + ": " + shieldedResult);
}
// frontEndActivityGraph has no post constraints
this.__getController2r = getController2r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getController2rSet = true;
}
}
return getController2r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetController();
private FrontEndAction __getInitialAction5r;
private boolean __getInitialAction5rSet = false;
/**
*
* @return (FrontEndAction)handleGetInitialAction()
*/
public final FrontEndAction getInitialAction()
{
FrontEndAction getInitialAction5r = this.__getInitialAction5r;
if (!this.__getInitialAction5rSet)
{
// frontEndActivityGraph has no pre constraints
Object result = handleGetInitialAction();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getInitialAction5r = (FrontEndAction)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndActivityGraphLogic.logger.warn("incorrect metafacade cast for FrontEndActivityGraphLogic.getInitialAction FrontEndAction " + result + ": " + shieldedResult);
}
// frontEndActivityGraph has no post constraints
this.__getInitialAction5r = getInitialAction5r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getInitialAction5rSet = true;
}
}
return getInitialAction5r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetInitialAction();
/**
* @param validationMessages Collection
* @see ActivityGraphFacadeLogicImpl#validateInvariants(Collection validationMessages)
*/
@Override
public void validateInvariants(Collection validationMessages)
{
super.validateInvariants(validationMessages);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy