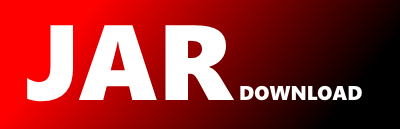
org.andromda.metafacades.emf.uml22.FrontEndControllerOperationLogic Maven / Gradle / Ivy
Show all versions of andromda-metafacades-emf-uml22 Show documentation
// license-header java merge-point
//
// Attention: generated code (by MetafacadeLogic.vsl) - do not modify!
//
package org.andromda.metafacades.emf.uml22;
import java.util.Collection;
import java.util.List;
import org.andromda.core.metafacade.MetafacadeBase;
import org.andromda.core.metafacade.ModelValidationMessage;
import org.andromda.metafacades.uml.FrontEndAction;
import org.andromda.metafacades.uml.FrontEndActivityGraph;
import org.andromda.metafacades.uml.FrontEndControllerOperation;
import org.andromda.metafacades.uml.FrontEndParameter;
import org.andromda.translation.ocl.validation.OCLExpressions;
import org.andromda.translation.ocl.validation.OCLIntrospector;
import org.andromda.translation.ocl.validation.OCLResultEnsurer;
import org.apache.log4j.Logger;
import org.eclipse.uml2.uml.Operation;
/**
*
* Represents an operation modeled on a controller.
*
* MetafacadeLogic for FrontEndControllerOperation
*
* @see FrontEndControllerOperation
*/
public abstract class FrontEndControllerOperationLogic
extends OperationFacadeLogicImpl
implements FrontEndControllerOperation
{
/**
* The underlying UML object
* @see Object
*/
protected Object metaObject;
/** Create Metafacade implementation instance using the MetafacadeFactory from the context
* @param metaObjectIn
* @param context
*/
protected FrontEndControllerOperationLogic(Object metaObjectIn, String context)
{
super((Operation)metaObjectIn, getContext(context));
this.metaObject = metaObjectIn;
}
/**
* The logger instance.
*/
private static final Logger logger = Logger.getLogger(FrontEndControllerOperationLogic.class);
/**
* Gets the context for this metafacade logic instance.
* @param context String. Set to FrontEndControllerOperation if null
* @return context String
*/
private static String getContext(String context)
{
if (context == null)
{
context = "org.andromda.metafacades.uml.FrontEndControllerOperation";
}
return context;
}
/** Reset context only for non-root metafacades
* @param context
*/
@Override
public void resetMetafacadeContext(String context)
{
if (!this.contextRoot) // reset context only for non-root metafacades
{
context = getContext(context); // to have same value as in original constructor call
setMetafacadeContext (context);
}
}
/**
* @return boolean true always
* @see FrontEndControllerOperation
*/
public boolean isFrontEndControllerOperationMetaType()
{
return true;
}
// --------------- attributes ---------------------
/**
* @see FrontEndControllerOperation#isOwnerIsController()
* @return boolean
*/
protected abstract boolean handleIsOwnerIsController();
private boolean __ownerIsController1a;
private boolean __ownerIsController1aSet = false;
/**
*
* Indicates if the owner of this operation is a controller.
*
* @return (boolean)handleIsOwnerIsController()
*/
public final boolean isOwnerIsController()
{
boolean ownerIsController1a = this.__ownerIsController1a;
if (!this.__ownerIsController1aSet)
{
// ownerIsController has no pre constraints
ownerIsController1a = handleIsOwnerIsController();
// ownerIsController has no post constraints
this.__ownerIsController1a = ownerIsController1a;
if (isMetafacadePropertyCachingEnabled())
{
this.__ownerIsController1aSet = true;
}
}
return ownerIsController1a;
}
/**
* @see FrontEndControllerOperation#isAllArgumentsHaveFormFields()
* @return boolean
*/
protected abstract boolean handleIsAllArgumentsHaveFormFields();
private boolean __allArgumentsHaveFormFields2a;
private boolean __allArgumentsHaveFormFields2aSet = false;
/**
*
* For each front-end controller operation argument there must
* exist a form field for each action deferring to that operation.
* This form field must carry the same name and must be of the same
* type. True if this is the case, false otherwise.
*
* @return (boolean)handleIsAllArgumentsHaveFormFields()
*/
public final boolean isAllArgumentsHaveFormFields()
{
boolean allArgumentsHaveFormFields2a = this.__allArgumentsHaveFormFields2a;
if (!this.__allArgumentsHaveFormFields2aSet)
{
// allArgumentsHaveFormFields has no pre constraints
allArgumentsHaveFormFields2a = handleIsAllArgumentsHaveFormFields();
// allArgumentsHaveFormFields has no post constraints
this.__allArgumentsHaveFormFields2a = allArgumentsHaveFormFields2a;
if (isMetafacadePropertyCachingEnabled())
{
this.__allArgumentsHaveFormFields2aSet = true;
}
}
return allArgumentsHaveFormFields2a;
}
// ------------- associations ------------------
private List __getFormFields2r;
private boolean __getFormFields2rSet = false;
/**
*
* Gets the controller operation to which this parameter belongs.
*
* @return (List)handleGetFormFields()
*/
public final List getFormFields()
{
List getFormFields2r = this.__getFormFields2r;
if (!this.__getFormFields2rSet)
{
// controllerOperation has no pre constraints
List result = handleGetFormFields();
List shieldedResult = this.shieldedElements(result);
try
{
getFormFields2r = (List)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndControllerOperationLogic.logger.warn("incorrect metafacade cast for FrontEndControllerOperationLogic.getFormFields List " + result + ": " + shieldedResult);
}
// controllerOperation has no post constraints
this.__getFormFields2r = getFormFields2r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getFormFields2rSet = true;
}
}
return getFormFields2r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return List
*/
protected abstract List handleGetFormFields();
private List __getDeferringActions3r;
private boolean __getDeferringActions3rSet = false;
/**
*
* The controller operations to which this action defers, the order
* is preserved.
*
* @return (List)handleGetDeferringActions()
*/
public final List getDeferringActions()
{
List getDeferringActions3r = this.__getDeferringActions3r;
if (!this.__getDeferringActions3rSet)
{
// deferredOperations has no pre constraints
List result = handleGetDeferringActions();
List shieldedResult = this.shieldedElements(result);
try
{
getDeferringActions3r = (List)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndControllerOperationLogic.logger.warn("incorrect metafacade cast for FrontEndControllerOperationLogic.getDeferringActions List " + result + ": " + shieldedResult);
}
// deferredOperations has no post constraints
this.__getDeferringActions3r = getDeferringActions3r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getDeferringActions3rSet = true;
}
}
return getDeferringActions3r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return List
*/
protected abstract List handleGetDeferringActions();
private FrontEndActivityGraph __getActivityGraph4r;
private boolean __getActivityGraph4rSet = false;
/**
*
* @return (FrontEndActivityGraph)handleGetActivityGraph()
*/
public final FrontEndActivityGraph getActivityGraph()
{
FrontEndActivityGraph getActivityGraph4r = this.__getActivityGraph4r;
if (!this.__getActivityGraph4rSet)
{
// frontEndControllerOperation has no pre constraints
Object result = handleGetActivityGraph();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getActivityGraph4r = (FrontEndActivityGraph)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndControllerOperationLogic.logger.warn("incorrect metafacade cast for FrontEndControllerOperationLogic.getActivityGraph FrontEndActivityGraph " + result + ": " + shieldedResult);
}
// frontEndControllerOperation has no post constraints
this.__getActivityGraph4r = getActivityGraph4r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getActivityGraph4rSet = true;
}
}
return getActivityGraph4r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetActivityGraph();
/**
* Constraint: org::andromda::metafacades::uml::FrontEndControllerOperation::all arguments need an event parameter
* Error: For each controller operation argument there must exist a parameter for each action deferring to that operation. This parameter must carry the same name and must be of the same type.
* OCL: context FrontEndControllerOperation inv: allArgumentsHaveFormFields
* Constraint: org::andromda::metafacades::uml::FrontEndControllerOperation::the operation name may not be the same as the use-case name
* Error: It is not allowed to give a controller operation the same name as the use-case for which it is defined, please either rename your operation or your use-case.
* OCL: context FrontEndControllerOperation inv: name <> owner.useCase.name
* @param validationMessages Collection
* @see OperationFacadeLogicImpl#validateInvariants(Collection validationMessages)
*/
@Override
public void validateInvariants(Collection validationMessages)
{
super.validateInvariants(validationMessages);
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure(OCLIntrospector.invoke(contextElement,"allArgumentsHaveFormFields"));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::FrontEndControllerOperation::all arguments need an event parameter",
"For each controller operation argument there must exist a parameter for each action deferring to that operation. This parameter must carry the same name and must be of the same type."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::FrontEndControllerOperation::all arguments need an event parameter' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure(OCLExpressions.notEqual(OCLIntrospector.invoke(contextElement,"name"),OCLIntrospector.invoke(contextElement,"owner.useCase.name")));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::FrontEndControllerOperation::the operation name may not be the same as the use-case name",
"It is not allowed to give a controller operation the same name as the use-case for which it is defined, please either rename your operation or your use-case."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::FrontEndControllerOperation::the operation name may not be the same as the use-case name' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
}
}