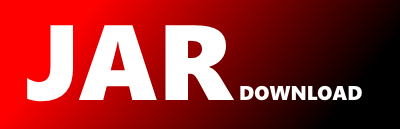
org.andromda.metafacades.emf.uml22.FrontEndUseCaseLogic Maven / Gradle / Ivy
Show all versions of andromda-metafacades-emf-uml22 Show documentation
// license-header java merge-point
//
// Attention: generated code (by MetafacadeLogic.vsl) - do not modify!
//
package org.andromda.metafacades.emf.uml22;
import java.util.Collection;
import java.util.List;
import org.andromda.core.metafacade.MetafacadeBase;
import org.andromda.core.metafacade.ModelValidationMessage;
import org.andromda.metafacades.uml.FrontEndAction;
import org.andromda.metafacades.uml.FrontEndActivityGraph;
import org.andromda.metafacades.uml.FrontEndController;
import org.andromda.metafacades.uml.FrontEndFinalState;
import org.andromda.metafacades.uml.FrontEndParameter;
import org.andromda.metafacades.uml.FrontEndUseCase;
import org.andromda.metafacades.uml.FrontEndView;
import org.andromda.metafacades.uml.Role;
import org.andromda.translation.ocl.validation.OCLCollections;
import org.andromda.translation.ocl.validation.OCLExpressions;
import org.andromda.translation.ocl.validation.OCLIntrospector;
import org.andromda.translation.ocl.validation.OCLResultEnsurer;
import org.apache.commons.collections.Predicate;
import org.apache.commons.collections.Transformer;
import org.apache.log4j.Logger;
import org.eclipse.uml2.uml.UseCase;
/**
*
* Represents a use case used in the "front end" of an application.
*
* MetafacadeLogic for FrontEndUseCase
*
* @see FrontEndUseCase
*/
public abstract class FrontEndUseCaseLogic
extends UseCaseFacadeLogicImpl
implements FrontEndUseCase
{
/**
* The underlying UML object
* @see Object
*/
protected Object metaObject;
/** Create Metafacade implementation instance using the MetafacadeFactory from the context
* @param metaObjectIn
* @param context
*/
protected FrontEndUseCaseLogic(Object metaObjectIn, String context)
{
super((UseCase)metaObjectIn, getContext(context));
this.metaObject = metaObjectIn;
}
/**
* The logger instance.
*/
private static final Logger logger = Logger.getLogger(FrontEndUseCaseLogic.class);
/**
* Gets the context for this metafacade logic instance.
* @param context String. Set to FrontEndUseCase if null
* @return context String
*/
private static String getContext(String context)
{
if (context == null)
{
context = "org.andromda.metafacades.uml.FrontEndUseCase";
}
return context;
}
/** Reset context only for non-root metafacades
* @param context
*/
@Override
public void resetMetafacadeContext(String context)
{
if (!this.contextRoot) // reset context only for non-root metafacades
{
context = getContext(context); // to have same value as in original constructor call
setMetafacadeContext (context);
}
}
/**
* @return boolean true always
* @see FrontEndUseCase
*/
public boolean isFrontEndUseCaseMetaType()
{
return true;
}
// --------------- attributes ---------------------
/**
* @see FrontEndUseCase#isEntryUseCase()
* @return boolean
*/
protected abstract boolean handleIsEntryUseCase();
private boolean __entryUseCase1a;
private boolean __entryUseCase1aSet = false;
/**
*
* True if this use-case is the entry point to the front end.
*
* @return (boolean)handleIsEntryUseCase()
*/
public final boolean isEntryUseCase()
{
boolean entryUseCase1a = this.__entryUseCase1a;
if (!this.__entryUseCase1aSet)
{
// entryUseCase has no pre constraints
entryUseCase1a = handleIsEntryUseCase();
// entryUseCase has no post constraints
this.__entryUseCase1a = entryUseCase1a;
if (isMetafacadePropertyCachingEnabled())
{
this.__entryUseCase1aSet = true;
}
}
return entryUseCase1a;
}
/**
* @see FrontEndUseCase#isSecured()
* @return boolean
*/
protected abstract boolean handleIsSecured();
private boolean __secured2a;
private boolean __secured2aSet = false;
/**
*
* Indicates if this use case is "secured". This is true when
* there is at least one role associated to it.
*
* @return (boolean)handleIsSecured()
*/
public final boolean isSecured()
{
boolean secured2a = this.__secured2a;
if (!this.__secured2aSet)
{
// secured has no pre constraints
secured2a = handleIsSecured();
// secured has no post constraints
this.__secured2a = secured2a;
if (isMetafacadePropertyCachingEnabled())
{
this.__secured2aSet = true;
}
}
return secured2a;
}
// ------------- associations ------------------
private FrontEndController __getController1r;
private boolean __getController1rSet = false;
/**
*
* Returns the use-case "controlled" by this controller.
*
* @return (FrontEndController)handleGetController()
*/
public final FrontEndController getController()
{
FrontEndController getController1r = this.__getController1r;
if (!this.__getController1rSet)
{
// useCase has no pre constraints
Object result = handleGetController();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getController1r = (FrontEndController)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndUseCaseLogic.logger.warn("incorrect metafacade cast for FrontEndUseCaseLogic.getController FrontEndController " + result + ": " + shieldedResult);
}
// useCase has no post constraints
this.__getController1r = getController1r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getController1rSet = true;
}
}
return getController1r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetController();
private FrontEndActivityGraph __getActivityGraph2r;
private boolean __getActivityGraph2rSet = false;
/**
*
* The use case to which this activity graph belongs.
*
* @return (FrontEndActivityGraph)handleGetActivityGraph()
*/
public final FrontEndActivityGraph getActivityGraph()
{
FrontEndActivityGraph getActivityGraph2r = this.__getActivityGraph2r;
if (!this.__getActivityGraph2rSet)
{
// frontEndUseCase has no pre constraints
Object result = handleGetActivityGraph();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getActivityGraph2r = (FrontEndActivityGraph)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndUseCaseLogic.logger.warn("incorrect metafacade cast for FrontEndUseCaseLogic.getActivityGraph FrontEndActivityGraph " + result + ": " + shieldedResult);
}
// frontEndUseCase has no post constraints
this.__getActivityGraph2r = getActivityGraph2r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getActivityGraph2rSet = true;
}
}
return getActivityGraph2r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetActivityGraph();
private List __getAllUseCases4r;
private boolean __getAllUseCases4rSet = false;
/**
*
* @return (List)handleGetAllUseCases()
*/
public final List getAllUseCases()
{
List getAllUseCases4r = this.__getAllUseCases4r;
if (!this.__getAllUseCases4rSet)
{
// frontEndUseCase has no pre constraints
List result = handleGetAllUseCases();
List shieldedResult = this.shieldedElements(result);
try
{
getAllUseCases4r = (List)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndUseCaseLogic.logger.warn("incorrect metafacade cast for FrontEndUseCaseLogic.getAllUseCases List " + result + ": " + shieldedResult);
}
// frontEndUseCase has no post constraints
this.__getAllUseCases4r = getAllUseCases4r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getAllUseCases4rSet = true;
}
}
return getAllUseCases4r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return List
*/
protected abstract List handleGetAllUseCases();
private List __getRoles5r;
private boolean __getRoles5rSet = false;
/**
*
* @return (List)handleGetRoles()
*/
public final List getRoles()
{
List getRoles5r = this.__getRoles5r;
if (!this.__getRoles5rSet)
{
// frontEndUseCase has no pre constraints
List result = handleGetRoles();
List shieldedResult = this.shieldedElements(result);
try
{
getRoles5r = (List)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndUseCaseLogic.logger.warn("incorrect metafacade cast for FrontEndUseCaseLogic.getRoles List " + result + ": " + shieldedResult);
}
// frontEndUseCase has no post constraints
this.__getRoles5r = getRoles5r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getRoles5rSet = true;
}
}
return getRoles5r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return List
*/
protected abstract List handleGetRoles();
private List __getAllRoles6r;
private boolean __getAllRoles6rSet = false;
/**
*
* @return (List)handleGetAllRoles()
*/
public final List getAllRoles()
{
List getAllRoles6r = this.__getAllRoles6r;
if (!this.__getAllRoles6rSet)
{
// frontEndUseCase has no pre constraints
List result = handleGetAllRoles();
List shieldedResult = this.shieldedElements(result);
try
{
getAllRoles6r = (List)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndUseCaseLogic.logger.warn("incorrect metafacade cast for FrontEndUseCaseLogic.getAllRoles List " + result + ": " + shieldedResult);
}
// frontEndUseCase has no post constraints
this.__getAllRoles6r = getAllRoles6r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getAllRoles6rSet = true;
}
}
return getAllRoles6r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return List
*/
protected abstract List handleGetAllRoles();
private List __getViews7r;
private boolean __getViews7rSet = false;
/**
*
* The use-case of which this view is a member.
*
* @return (List)handleGetViews()
*/
public final List getViews()
{
List getViews7r = this.__getViews7r;
if (!this.__getViews7rSet)
{
// useCase has no pre constraints
List result = handleGetViews();
List shieldedResult = this.shieldedElements(result);
try
{
getViews7r = (List)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndUseCaseLogic.logger.warn("incorrect metafacade cast for FrontEndUseCaseLogic.getViews List " + result + ": " + shieldedResult);
}
// useCase has no post constraints
this.__getViews7r = getViews7r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getViews7rSet = true;
}
}
return getViews7r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return List
*/
protected abstract List handleGetViews();
private List __getReferencingFinalStates9r;
private boolean __getReferencingFinalStates9rSet = false;
/**
*
* The use case the final state is "targetting".
*
* @return (List)handleGetReferencingFinalStates()
*/
public final List getReferencingFinalStates()
{
List getReferencingFinalStates9r = this.__getReferencingFinalStates9r;
if (!this.__getReferencingFinalStates9rSet)
{
// targetUseCase has no pre constraints
List result = handleGetReferencingFinalStates();
List shieldedResult = this.shieldedElements(result);
try
{
getReferencingFinalStates9r = (List)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndUseCaseLogic.logger.warn("incorrect metafacade cast for FrontEndUseCaseLogic.getReferencingFinalStates List " + result + ": " + shieldedResult);
}
// targetUseCase has no post constraints
this.__getReferencingFinalStates9r = getReferencingFinalStates9r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getReferencingFinalStates9rSet = true;
}
}
return getReferencingFinalStates9r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return List
*/
protected abstract List handleGetReferencingFinalStates();
private List __getActions10r;
private boolean __getActions10rSet = false;
/**
*
* @return (List)handleGetActions()
*/
public final List getActions()
{
List getActions10r = this.__getActions10r;
if (!this.__getActions10rSet)
{
// frontEndUseCase has no pre constraints
List result = handleGetActions();
List shieldedResult = this.shieldedElements(result);
try
{
getActions10r = (List)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndUseCaseLogic.logger.warn("incorrect metafacade cast for FrontEndUseCaseLogic.getActions List " + result + ": " + shieldedResult);
}
// frontEndUseCase has no post constraints
this.__getActions10r = getActions10r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getActions10rSet = true;
}
}
return getActions10r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return List
*/
protected abstract List handleGetActions();
private FrontEndView __getInitialView11r;
private boolean __getInitialView11rSet = false;
/**
*
* @return (FrontEndView)handleGetInitialView()
*/
public final FrontEndView getInitialView()
{
FrontEndView getInitialView11r = this.__getInitialView11r;
if (!this.__getInitialView11rSet)
{
// frontEndUseCase has no pre constraints
Object result = handleGetInitialView();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getInitialView11r = (FrontEndView)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndUseCaseLogic.logger.warn("incorrect metafacade cast for FrontEndUseCaseLogic.getInitialView FrontEndView " + result + ": " + shieldedResult);
}
// frontEndUseCase has no post constraints
this.__getInitialView11r = getInitialView11r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getInitialView11rSet = true;
}
}
return getInitialView11r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetInitialView();
private List __getViewVariables12r;
private boolean __getViewVariables12rSet = false;
/**
*
* @return (List)handleGetViewVariables()
*/
public final List getViewVariables()
{
List getViewVariables12r = this.__getViewVariables12r;
if (!this.__getViewVariables12rSet)
{
// frontEndUseCase has no pre constraints
List result = handleGetViewVariables();
List shieldedResult = this.shieldedElements(result);
try
{
getViewVariables12r = (List)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndUseCaseLogic.logger.warn("incorrect metafacade cast for FrontEndUseCaseLogic.getViewVariables List " + result + ": " + shieldedResult);
}
// frontEndUseCase has no post constraints
this.__getViewVariables12r = getViewVariables12r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getViewVariables12rSet = true;
}
}
return getViewVariables12r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return List
*/
protected abstract List handleGetViewVariables();
/**
* Constraint: org::andromda::metafacades::uml::FrontEndUseCase::each usecase needs one and only one graph
* Error: Each use-case needs one and only one activity graph.
* OCL: context FrontEndUseCase inv: activityGraph->notEmpty()
* Constraint: org::andromda::metafacades::uml::FrontEndUseCase::one and only one usecase must be a FrontEndApplication
* Error: One and only one use-case must be marked as the application entry use-case. Currently this is done by adding the FrontEndApplication stereotype to it.
* OCL: context FrontEndUseCase inv: allUseCases->one(entryUseCase = true)
* Constraint: org::andromda::metafacades::uml::FrontEndUseCase::non-empty unique usecase name
* Error: Each use-case must have a non-empty name that is unique among all use-cases.
* OCL: context FrontEndUseCase inv: name->notEmpty() and model.allUseCases->isUnique(name)
* Constraint: org::andromda::metafacades::uml::FrontEndUseCase::each front-end use-case needs to be in a package
* Error: Each front-end use-case is required to be modeled in a package, doing otherwise will result in uncompileable code due to filename collisions.
* OCL: context FrontEndUseCase inv: packageName->notEmpty()
* @param validationMessages Collection
* @see UseCaseFacadeLogicImpl#validateInvariants(Collection validationMessages)
*/
@Override
public void validateInvariants(Collection validationMessages)
{
super.validateInvariants(validationMessages);
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure(OCLCollections.notEmpty(OCLIntrospector.invoke(contextElement,"activityGraph")));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::FrontEndUseCase::each usecase needs one and only one graph",
"Each use-case needs one and only one activity graph."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::FrontEndUseCase::each usecase needs one and only one graph' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure(OCLCollections.one(OCLIntrospector.invoke(contextElement,"allUseCases"),new Predicate(){public boolean evaluate(Object object){return Boolean.valueOf(String.valueOf(OCLExpressions.equal(OCLIntrospector.invoke(object,"entryUseCase"),true))).booleanValue();}}));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::FrontEndUseCase::one and only one usecase must be a FrontEndApplication",
"One and only one use-case must be marked as the application entry use-case. Currently this is done by adding the FrontEndApplication stereotype to it."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::FrontEndUseCase::one and only one usecase must be a FrontEndApplication' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure(OCLCollections.notEmpty(OCLIntrospector.invoke(contextElement,"name"))&&OCLCollections.isUnique(OCLIntrospector.invoke(contextElement,"model.allUseCases"),new Transformer(){public Object transform(Object object){return OCLIntrospector.invoke(object,"name");}}));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::FrontEndUseCase::non-empty unique usecase name",
"Each use-case must have a non-empty name that is unique among all use-cases."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::FrontEndUseCase::non-empty unique usecase name' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure(OCLCollections.notEmpty(OCLIntrospector.invoke(contextElement,"packageName")));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::FrontEndUseCase::each front-end use-case needs to be in a package",
"Each front-end use-case is required to be modeled in a package, doing otherwise will result in uncompileable code due to filename collisions."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::FrontEndUseCase::each front-end use-case needs to be in a package' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
}
}