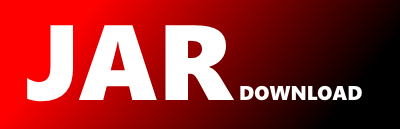
org.andromda.metafacades.emf.uml22.FrontEndViewLogic Maven / Gradle / Ivy
Show all versions of andromda-metafacades-emf-uml22 Show documentation
// license-header java merge-point
//
// Attention: generated code (by MetafacadeLogic.vsl) - do not modify!
//
package org.andromda.metafacades.emf.uml22;
import java.util.Collection;
import java.util.List;
import org.andromda.core.metafacade.MetafacadeBase;
import org.andromda.core.metafacade.ModelValidationMessage;
import org.andromda.metafacades.uml.FrontEndAction;
import org.andromda.metafacades.uml.FrontEndParameter;
import org.andromda.metafacades.uml.FrontEndUseCase;
import org.andromda.metafacades.uml.FrontEndView;
import org.andromda.translation.ocl.validation.OCLCollections;
import org.andromda.translation.ocl.validation.OCLExpressions;
import org.andromda.translation.ocl.validation.OCLIntrospector;
import org.andromda.translation.ocl.validation.OCLResultEnsurer;
import org.apache.commons.collections.Transformer;
import org.apache.log4j.Logger;
/**
*
* Represents a view within a front end application.
*
* MetafacadeLogic for FrontEndView
*
* @see FrontEndView
*/
public abstract class FrontEndViewLogic
extends FrontEndActionStateLogicImpl
implements FrontEndView
{
/**
* The underlying UML object
* @see Object
*/
protected Object metaObject;
/** Create Metafacade implementation instance using the MetafacadeFactory from the context
* @param metaObjectIn
* @param context
*/
protected FrontEndViewLogic(Object metaObjectIn, String context)
{
super(metaObjectIn, getContext(context));
this.metaObject = metaObjectIn;
}
/**
* The logger instance.
*/
private static final Logger logger = Logger.getLogger(FrontEndViewLogic.class);
/**
* Gets the context for this metafacade logic instance.
* @param context String. Set to FrontEndView if null
* @return context String
*/
private static String getContext(String context)
{
if (context == null)
{
context = "org.andromda.metafacades.uml.FrontEndView";
}
return context;
}
/** Reset context only for non-root metafacades
* @param context
*/
@Override
public void resetMetafacadeContext(String context)
{
if (!this.contextRoot) // reset context only for non-root metafacades
{
context = getContext(context); // to have same value as in original constructor call
setMetafacadeContext (context);
}
}
/**
* @return boolean true always
* @see FrontEndView
*/
public boolean isFrontEndViewMetaType()
{
return true;
}
// --------------- attributes ---------------------
/**
* @see FrontEndView#isFrontEndView()
* @return boolean
*/
protected abstract boolean handleIsFrontEndView();
private boolean __frontEndView1a;
private boolean __frontEndView1aSet = false;
/**
*
* True if this element carries the FrontEndView stereotype.
*
* @return (boolean)handleIsFrontEndView()
*/
public final boolean isFrontEndView()
{
boolean frontEndView1a = this.__frontEndView1a;
if (!this.__frontEndView1aSet)
{
// frontEndView has no pre constraints
frontEndView1a = handleIsFrontEndView();
// frontEndView has no post constraints
this.__frontEndView1a = frontEndView1a;
if (isMetafacadePropertyCachingEnabled())
{
this.__frontEndView1aSet = true;
}
}
return frontEndView1a;
}
// ------------- associations ------------------
private FrontEndUseCase __getUseCase1r;
private boolean __getUseCase1rSet = false;
/**
*
* All views that are part of this use case.
*
* @return (FrontEndUseCase)handleGetUseCase()
*/
public final FrontEndUseCase getUseCase()
{
FrontEndUseCase getUseCase1r = this.__getUseCase1r;
if (!this.__getUseCase1rSet)
{
// views has no pre constraints
Object result = handleGetUseCase();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getUseCase1r = (FrontEndUseCase)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndViewLogic.logger.warn("incorrect metafacade cast for FrontEndViewLogic.getUseCase FrontEndUseCase " + result + ": " + shieldedResult);
}
// views has no post constraints
this.__getUseCase1r = getUseCase1r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getUseCase1rSet = true;
}
}
return getUseCase1r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetUseCase();
private List __getVariables2r;
private boolean __getVariables2rSet = false;
/**
*
* Represents the view in which this parameter will be used.
*
* @return (List)handleGetVariables()
*/
public final List getVariables()
{
List getVariables2r = this.__getVariables2r;
if (!this.__getVariables2rSet)
{
// view has no pre constraints
List result = handleGetVariables();
List shieldedResult = this.shieldedElements(result);
try
{
getVariables2r = (List)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndViewLogic.logger.warn("incorrect metafacade cast for FrontEndViewLogic.getVariables List " + result + ": " + shieldedResult);
}
// view has no post constraints
this.__getVariables2r = getVariables2r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getVariables2rSet = true;
}
}
return getVariables2r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return List
*/
protected abstract List handleGetVariables();
private List __getAllFormFields3r;
private boolean __getAllFormFields3rSet = false;
/**
*
* @return (List)handleGetAllFormFields()
*/
public final List getAllFormFields()
{
List getAllFormFields3r = this.__getAllFormFields3r;
if (!this.__getAllFormFields3rSet)
{
// frontEndView has no pre constraints
List result = handleGetAllFormFields();
List shieldedResult = this.shieldedElements(result);
try
{
getAllFormFields3r = (List)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndViewLogic.logger.warn("incorrect metafacade cast for FrontEndViewLogic.getAllFormFields List " + result + ": " + shieldedResult);
}
// frontEndView has no post constraints
this.__getAllFormFields3r = getAllFormFields3r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getAllFormFields3rSet = true;
}
}
return getAllFormFields3r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return List
*/
protected abstract List handleGetAllFormFields();
private List __getAllActionParameters6r;
private boolean __getAllActionParameters6rSet = false;
/**
*
* @return (List)handleGetAllActionParameters()
*/
public final List getAllActionParameters()
{
List getAllActionParameters6r = this.__getAllActionParameters6r;
if (!this.__getAllActionParameters6rSet)
{
// frontEndView has no pre constraints
List result = handleGetAllActionParameters();
List shieldedResult = this.shieldedElements(result);
try
{
getAllActionParameters6r = (List)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndViewLogic.logger.warn("incorrect metafacade cast for FrontEndViewLogic.getAllActionParameters List " + result + ": " + shieldedResult);
}
// frontEndView has no post constraints
this.__getAllActionParameters6r = getAllActionParameters6r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getAllActionParameters6rSet = true;
}
}
return getAllActionParameters6r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return List
*/
protected abstract List handleGetAllActionParameters();
private List __getTables7r;
private boolean __getTables7rSet = false;
/**
*
* @return (List)handleGetTables()
*/
public final List getTables()
{
List getTables7r = this.__getTables7r;
if (!this.__getTables7rSet)
{
// frontEndView has no pre constraints
List result = handleGetTables();
List shieldedResult = this.shieldedElements(result);
try
{
getTables7r = (List)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndViewLogic.logger.warn("incorrect metafacade cast for FrontEndViewLogic.getTables List " + result + ": " + shieldedResult);
}
// frontEndView has no post constraints
this.__getTables7r = getTables7r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getTables7rSet = true;
}
}
return getTables7r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return List
*/
protected abstract List handleGetTables();
private List __getActions8r;
private boolean __getActions8rSet = false;
/**
*
* The StateVertex (FrontEndView or PseudostateFacade) on which
* this action can be triggered.
*
* @return (List)handleGetActions()
*/
public final List getActions()
{
List getActions8r = this.__getActions8r;
if (!this.__getActions8rSet)
{
// input has no pre constraints
List result = handleGetActions();
List shieldedResult = this.shieldedElements(result);
try
{
getActions8r = (List)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndViewLogic.logger.warn("incorrect metafacade cast for FrontEndViewLogic.getActions List " + result + ": " + shieldedResult);
}
// input has no post constraints
this.__getActions8r = getActions8r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getActions8rSet = true;
}
}
return getActions8r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return List
*/
protected abstract List handleGetActions();
/**
* Constraint: org::andromda::metafacades::uml::FrontEndView::unique view name per usecase
* Error: Each name of a view action state must be unique in the namespace of a front-end use-case.
* OCL: context FrontEndView inv: useCase.views->isUnique(name)
* Constraint: org::andromda::metafacades::uml::FrontEndView::view variables must have unique names
* Error: Each view-variable should have a unique name within the context of a view.
* OCL: context FrontEndView inv : variables->isUnique(name)
* Constraint: org::andromda::metafacades::uml::FrontEndView::front-end views cannot defer operations
* Error: Views cannot defer to operations. All deferrable events modeled on a front-end view will be ignored.
* OCL: context FrontEndView inv: controllerCalls->size() = 0
* Constraint: org::andromda::metafacades::uml::FrontEndView::each action going out of a front-end view must be unique
* Error: Each view must contain actions which each have a unique name, this view has actions with duplicate names.
* OCL: context FrontEndView inv: actions->isUnique(name)
* @param validationMessages Collection
* @see FrontEndActionStateLogicImpl#validateInvariants(Collection validationMessages)
*/
@Override
public void validateInvariants(Collection validationMessages)
{
super.validateInvariants(validationMessages);
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure(OCLCollections.isUnique(OCLIntrospector.invoke(contextElement,"useCase.views"),new Transformer(){public Object transform(Object object){return OCLIntrospector.invoke(object,"name");}}));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::FrontEndView::unique view name per usecase",
"Each name of a view action state must be unique in the namespace of a front-end use-case."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::FrontEndView::unique view name per usecase' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure(OCLCollections.isUnique(OCLIntrospector.invoke(contextElement,"variables"),new Transformer(){public Object transform(Object object){return OCLIntrospector.invoke(object,"name");}}));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::FrontEndView::view variables must have unique names",
"Each view-variable should have a unique name within the context of a view."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::FrontEndView::view variables must have unique names' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure(OCLExpressions.equal(OCLCollections.size(OCLIntrospector.invoke(contextElement,"controllerCalls")),0));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::FrontEndView::front-end views cannot defer operations",
"Views cannot defer to operations. All deferrable events modeled on a front-end view will be ignored."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::FrontEndView::front-end views cannot defer operations' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure(OCLCollections.isUnique(OCLIntrospector.invoke(contextElement,"actions"),new Transformer(){public Object transform(Object object){return OCLIntrospector.invoke(object,"name");}}));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::FrontEndView::each action going out of a front-end view must be unique",
"Each view must contain actions which each have a unique name, this view has actions with duplicate names."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::FrontEndView::each action going out of a front-end view must be unique' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
}
}