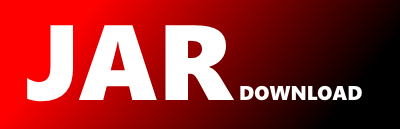
org.andromda.metafacades.emf.uml22.InstanceFacadeLogic Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of andromda-metafacades-emf-uml22 Show documentation
Show all versions of andromda-metafacades-emf-uml22 Show documentation
The Eclipse EMF UML2 v2.X metafacades. This is the set of EMF UML2 2.X metafacades
implementations. These implement the common UML metafacades for .uml model files.
The newest version!
// license-header java merge-point
//
// Attention: generated code (by MetafacadeLogic.vsl) - do not modify!
//
package org.andromda.metafacades.emf.uml22;
import java.util.Collection;
import java.util.List;
import org.andromda.core.metafacade.ModelValidationMessage;
import org.andromda.metafacades.uml.AttributeLinkFacade;
import org.andromda.metafacades.uml.ClassifierFacade;
import org.andromda.metafacades.uml.InstanceFacade;
import org.andromda.metafacades.uml.LinkEndFacade;
import org.andromda.metafacades.uml.LinkFacade;
import org.apache.log4j.Logger;
/**
*
* A representation of the model object 'Instance Specification'.
* Represents an instance in a modeled system. Has the capability
* of being a deployment target in a deployment relationship, in
* the case that it is an instance of a node. Has the capability of
* being a deployed artifact, if it is an instance of an artifact.
*
* MetafacadeLogic for InstanceFacade
*
* @see InstanceFacade
*/
public abstract class InstanceFacadeLogic
extends ModelElementFacadeLogicImpl
implements InstanceFacade
{
/**
* The underlying UML object
* @see ObjectInstance
*/
protected ObjectInstance metaObject;
/** Create Metafacade implementation instance using the MetafacadeFactory from the context
* @param metaObjectIn
* @param context
*/
protected InstanceFacadeLogic(ObjectInstance metaObjectIn, String context)
{
super(metaObjectIn, getContext(context));
this.metaObject = metaObjectIn;
}
/**
* The logger instance.
*/
private static final Logger logger = Logger.getLogger(InstanceFacadeLogic.class);
/**
* Gets the context for this metafacade logic instance.
* @param context String. Set to InstanceFacade if null
* @return context String
*/
private static String getContext(String context)
{
if (context == null)
{
context = "org.andromda.metafacades.uml.InstanceFacade";
}
return context;
}
/** Reset context only for non-root metafacades
* @param context
*/
@Override
public void resetMetafacadeContext(String context)
{
if (!this.contextRoot) // reset context only for non-root metafacades
{
context = getContext(context); // to have same value as in original constructor call
setMetafacadeContext (context);
}
}
/**
* @return boolean true always
* @see InstanceFacade
*/
public boolean isInstanceFacadeMetaType()
{
return true;
}
// ------------- associations ------------------
private Collection __getClassifiers1r;
private boolean __getClassifiers1rSet = false;
/**
*
* @return (Collection)handleGetClassifiers()
*/
public final Collection getClassifiers()
{
Collection getClassifiers1r = this.__getClassifiers1r;
if (!this.__getClassifiers1rSet)
{
// instanceFacade has no pre constraints
Collection result = handleGetClassifiers();
List shieldedResult = this.shieldedElements(result);
try
{
getClassifiers1r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
InstanceFacadeLogic.logger.warn("incorrect metafacade cast for InstanceFacadeLogic.getClassifiers Collection " + result + ": " + shieldedResult);
}
// instanceFacade has no post constraints
this.__getClassifiers1r = getClassifiers1r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getClassifiers1rSet = true;
}
}
return getClassifiers1r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetClassifiers();
private Collection __getLinkEnds2r;
private boolean __getLinkEnds2rSet = false;
/**
*
* The first instance to which this link end is attached.
*
* @return (Collection)handleGetLinkEnds()
*/
public final Collection getLinkEnds()
{
Collection getLinkEnds2r = this.__getLinkEnds2r;
if (!this.__getLinkEnds2rSet)
{
// instance has no pre constraints
Collection result = handleGetLinkEnds();
List shieldedResult = this.shieldedElements(result);
try
{
getLinkEnds2r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
InstanceFacadeLogic.logger.warn("incorrect metafacade cast for InstanceFacadeLogic.getLinkEnds Collection " + result + ": " + shieldedResult);
}
// instance has no post constraints
this.__getLinkEnds2r = getLinkEnds2r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getLinkEnds2rSet = true;
}
}
return getLinkEnds2r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetLinkEnds();
private Collection __getOwnedInstances3r;
private boolean __getOwnedInstances3rSet = false;
/**
*
* @return (Collection)handleGetOwnedInstances()
*/
public final Collection getOwnedInstances()
{
Collection getOwnedInstances3r = this.__getOwnedInstances3r;
if (!this.__getOwnedInstances3rSet)
{
// instanceFacade has no pre constraints
Collection result = handleGetOwnedInstances();
List shieldedResult = this.shieldedElements(result);
try
{
getOwnedInstances3r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
InstanceFacadeLogic.logger.warn("incorrect metafacade cast for InstanceFacadeLogic.getOwnedInstances Collection " + result + ": " + shieldedResult);
}
// instanceFacade has no post constraints
this.__getOwnedInstances3r = getOwnedInstances3r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getOwnedInstances3rSet = true;
}
}
return getOwnedInstances3r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetOwnedInstances();
private Collection __getOwnedLinks5r;
private boolean __getOwnedLinks5rSet = false;
/**
*
* @return (Collection)handleGetOwnedLinks()
*/
public final Collection getOwnedLinks()
{
Collection getOwnedLinks5r = this.__getOwnedLinks5r;
if (!this.__getOwnedLinks5rSet)
{
// instanceFacade has no pre constraints
Collection result = handleGetOwnedLinks();
List shieldedResult = this.shieldedElements(result);
try
{
getOwnedLinks5r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
InstanceFacadeLogic.logger.warn("incorrect metafacade cast for InstanceFacadeLogic.getOwnedLinks Collection " + result + ": " + shieldedResult);
}
// instanceFacade has no post constraints
this.__getOwnedLinks5r = getOwnedLinks5r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getOwnedLinks5rSet = true;
}
}
return getOwnedLinks5r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetOwnedLinks();
private Collection __getAttributeLinks6r;
private boolean __getAttributeLinks6rSet = false;
/**
*
* The owning instance for this link.
*
* @return (Collection)handleGetAttributeLinks()
*/
public final Collection getAttributeLinks()
{
Collection getAttributeLinks6r = this.__getAttributeLinks6r;
if (!this.__getAttributeLinks6rSet)
{
// instance has no pre constraints
Collection result = handleGetAttributeLinks();
List shieldedResult = this.shieldedElements(result);
try
{
getAttributeLinks6r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
InstanceFacadeLogic.logger.warn("incorrect metafacade cast for InstanceFacadeLogic.getAttributeLinks Collection " + result + ": " + shieldedResult);
}
// instance has no post constraints
this.__getAttributeLinks6r = getAttributeLinks6r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getAttributeLinks6rSet = true;
}
}
return getAttributeLinks6r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetAttributeLinks();
/**
*
* The instances this attribute link is attached too. An attribute
* link can have many instance values when its defining feature is
* an attribute with a 'many' multiplicity.
*
* @return (Collection)handleGetSlots()
*/
public final Collection getSlots()
{
Collection getSlots8r = null;
// values has no pre constraints
Collection result = handleGetSlots();
List shieldedResult = this.shieldedElements(result);
try
{
getSlots8r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
InstanceFacadeLogic.logger.warn("incorrect metafacade cast for InstanceFacadeLogic.getSlots Collection " + result + ": " + shieldedResult);
}
// values has no post constraints
return getSlots8r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetSlots();
/**
* @param validationMessages Collection
* @see ModelElementFacadeLogicImpl#validateInvariants(Collection validationMessages)
*/
@Override
public void validateInvariants(Collection validationMessages)
{
super.validateInvariants(validationMessages);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy