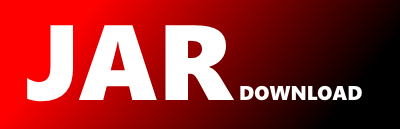
org.andromda.metafacades.emf.uml22.ParameterFacadeLogic Maven / Gradle / Ivy
Show all versions of andromda-metafacades-emf-uml22 Show documentation
// license-header java merge-point
//
// Attention: generated code (by MetafacadeLogic.vsl) - do not modify!
//
package org.andromda.metafacades.emf.uml22;
import java.util.Collection;
import org.andromda.core.metafacade.MetafacadeBase;
import org.andromda.core.metafacade.ModelValidationMessage;
import org.andromda.metafacades.uml.ClassifierFacade;
import org.andromda.metafacades.uml.EventFacade;
import org.andromda.metafacades.uml.OperationFacade;
import org.andromda.metafacades.uml.ParameterFacade;
import org.andromda.translation.ocl.validation.OCLCollections;
import org.andromda.translation.ocl.validation.OCLExpressions;
import org.andromda.translation.ocl.validation.OCLIntrospector;
import org.andromda.translation.ocl.validation.OCLResultEnsurer;
import org.apache.log4j.Logger;
import org.eclipse.uml2.uml.Parameter;
/**
*
* Specification of an argument used to pass information into or
* out of an invocation of a behavioral feature. Parameters are
* allowed to be treated as connectable elements. Parameters have
* support for streaming, exceptions, and parameter sets.
*
* MetafacadeLogic for ParameterFacade
*
* @see ParameterFacade
*/
public abstract class ParameterFacadeLogic
extends ModelElementFacadeLogicImpl
implements ParameterFacade
{
/**
* The underlying UML object
* @see Parameter
*/
protected Parameter metaObject;
/** Create Metafacade implementation instance using the MetafacadeFactory from the context
* @param metaObjectIn
* @param context
*/
protected ParameterFacadeLogic(Parameter metaObjectIn, String context)
{
super(metaObjectIn, getContext(context));
this.metaObject = metaObjectIn;
}
/**
* The logger instance.
*/
private static final Logger logger = Logger.getLogger(ParameterFacadeLogic.class);
/**
* Gets the context for this metafacade logic instance.
* @param context String. Set to ParameterFacade if null
* @return context String
*/
private static String getContext(String context)
{
if (context == null)
{
context = "org.andromda.metafacades.uml.ParameterFacade";
}
return context;
}
/** Reset context only for non-root metafacades
* @param context
*/
@Override
public void resetMetafacadeContext(String context)
{
if (!this.contextRoot) // reset context only for non-root metafacades
{
context = getContext(context); // to have same value as in original constructor call
setMetafacadeContext (context);
}
}
/**
* @return boolean true always
* @see ParameterFacade
*/
public boolean isParameterFacadeMetaType()
{
return true;
}
// --------------- attributes ---------------------
/**
* @see ParameterFacade#getDefaultValue()
* @return String
*/
protected abstract String handleGetDefaultValue();
private String __defaultValue1a;
private boolean __defaultValue1aSet = false;
/**
*
* @return (String)handleGetDefaultValue()
*/
public final String getDefaultValue()
{
String defaultValue1a = this.__defaultValue1a;
if (!this.__defaultValue1aSet)
{
// defaultValue has no pre constraints
defaultValue1a = handleGetDefaultValue();
// defaultValue has no post constraints
this.__defaultValue1a = defaultValue1a;
if (isMetafacadePropertyCachingEnabled())
{
this.__defaultValue1aSet = true;
}
}
return defaultValue1a;
}
/**
* @see ParameterFacade#isReturn()
* @return boolean
*/
protected abstract boolean handleIsReturn();
private boolean __return2a;
private boolean __return2aSet = false;
/**
*
* Whether or not this parameter represents a return parameter.
*
* @return (boolean)handleIsReturn()
*/
public final boolean isReturn()
{
boolean return2a = this.__return2a;
if (!this.__return2aSet)
{
// return has no pre constraints
return2a = handleIsReturn();
// return has no post constraints
this.__return2a = return2a;
if (isMetafacadePropertyCachingEnabled())
{
this.__return2aSet = true;
}
}
return return2a;
}
/**
* @see ParameterFacade#isRequired()
* @return boolean
*/
protected abstract boolean handleIsRequired();
private boolean __required3a;
private boolean __required3aSet = false;
/**
*
* Whether or not this parameter is considered required (i.e must a
* non-empty value).
*
* @return (boolean)handleIsRequired()
*/
public final boolean isRequired()
{
boolean required3a = this.__required3a;
if (!this.__required3aSet)
{
// required has no pre constraints
required3a = handleIsRequired();
// required has no post constraints
this.__required3a = required3a;
if (isMetafacadePropertyCachingEnabled())
{
this.__required3aSet = true;
}
}
return required3a;
}
/**
* @see ParameterFacade#getGetterName()
* @return String
*/
protected abstract String handleGetGetterName();
private String __getterName4a;
private boolean __getterName4aSet = false;
/**
*
* The name to use for accessors getting this parameter from a
* bean.
*
* @return (String)handleGetGetterName()
*/
public final String getGetterName()
{
String getterName4a = this.__getterName4a;
if (!this.__getterName4aSet)
{
// getterName has no pre constraints
getterName4a = handleGetGetterName();
// getterName has no post constraints
this.__getterName4a = getterName4a;
if (isMetafacadePropertyCachingEnabled())
{
this.__getterName4aSet = true;
}
}
return getterName4a;
}
/**
* @see ParameterFacade#getSetterName()
* @return String
*/
protected abstract String handleGetSetterName();
private String __setterName5a;
private boolean __setterName5aSet = false;
/**
*
* The name to use for accessors getting this parameter in a bean.
*
* @return (String)handleGetSetterName()
*/
public final String getSetterName()
{
String setterName5a = this.__setterName5a;
if (!this.__setterName5aSet)
{
// setterName has no pre constraints
setterName5a = handleGetSetterName();
// setterName has no post constraints
this.__setterName5a = setterName5a;
if (isMetafacadePropertyCachingEnabled())
{
this.__setterName5aSet = true;
}
}
return setterName5a;
}
/**
* @see ParameterFacade#isReadable()
* @return boolean
*/
protected abstract boolean handleIsReadable();
private boolean __readable6a;
private boolean __readable6aSet = false;
/**
*
* True if this parameter is readable, aka an in-parameter, or this
* feature is unspecified.
*
* @return (boolean)handleIsReadable()
*/
public final boolean isReadable()
{
boolean readable6a = this.__readable6a;
if (!this.__readable6aSet)
{
// readable has no pre constraints
readable6a = handleIsReadable();
// readable has no post constraints
this.__readable6a = readable6a;
if (isMetafacadePropertyCachingEnabled())
{
this.__readable6aSet = true;
}
}
return readable6a;
}
/**
* @see ParameterFacade#isWritable()
* @return boolean
*/
protected abstract boolean handleIsWritable();
private boolean __writable7a;
private boolean __writable7aSet = false;
/**
*
* True if this parameter is writable, aka an out-parameter, or
* this feature is unspecified.
*
* @return (boolean)handleIsWritable()
*/
public final boolean isWritable()
{
boolean writable7a = this.__writable7a;
if (!this.__writable7aSet)
{
// writable has no pre constraints
writable7a = handleIsWritable();
// writable has no post constraints
this.__writable7a = writable7a;
if (isMetafacadePropertyCachingEnabled())
{
this.__writable7aSet = true;
}
}
return writable7a;
}
/**
* @see ParameterFacade#isDefaultValuePresent()
* @return boolean
*/
protected abstract boolean handleIsDefaultValuePresent();
private boolean __defaultValuePresent8a;
private boolean __defaultValuePresent8aSet = false;
/**
*
* Indicates if the default value is present.
*
* @return (boolean)handleIsDefaultValuePresent()
*/
public final boolean isDefaultValuePresent()
{
boolean defaultValuePresent8a = this.__defaultValuePresent8a;
if (!this.__defaultValuePresent8aSet)
{
// defaultValuePresent has no pre constraints
defaultValuePresent8a = handleIsDefaultValuePresent();
// defaultValuePresent has no post constraints
this.__defaultValuePresent8a = defaultValuePresent8a;
if (isMetafacadePropertyCachingEnabled())
{
this.__defaultValuePresent8aSet = true;
}
}
return defaultValuePresent8a;
}
/**
* @see ParameterFacade#isInParameter()
* @return boolean
*/
protected abstract boolean handleIsInParameter();
private boolean __inParameter9a;
private boolean __inParameter9aSet = false;
/**
*
* True if this parameter is an 'in' parameter.
*
* @return (boolean)handleIsInParameter()
*/
public final boolean isInParameter()
{
boolean inParameter9a = this.__inParameter9a;
if (!this.__inParameter9aSet)
{
// inParameter has no pre constraints
inParameter9a = handleIsInParameter();
// inParameter has no post constraints
this.__inParameter9a = inParameter9a;
if (isMetafacadePropertyCachingEnabled())
{
this.__inParameter9aSet = true;
}
}
return inParameter9a;
}
/**
* @see ParameterFacade#isOutParameter()
* @return boolean
*/
protected abstract boolean handleIsOutParameter();
private boolean __outParameter10a;
private boolean __outParameter10aSet = false;
/**
*
* True if this parameter is an 'out' parameter.
*
* @return (boolean)handleIsOutParameter()
*/
public final boolean isOutParameter()
{
boolean outParameter10a = this.__outParameter10a;
if (!this.__outParameter10aSet)
{
// outParameter has no pre constraints
outParameter10a = handleIsOutParameter();
// outParameter has no post constraints
this.__outParameter10a = outParameter10a;
if (isMetafacadePropertyCachingEnabled())
{
this.__outParameter10aSet = true;
}
}
return outParameter10a;
}
/**
* @see ParameterFacade#isInoutParameter()
* @return boolean
*/
protected abstract boolean handleIsInoutParameter();
private boolean __inoutParameter11a;
private boolean __inoutParameter11aSet = false;
/**
*
* True if this parameter is an inout parameter.
*
* @return (boolean)handleIsInoutParameter()
*/
public final boolean isInoutParameter()
{
boolean inoutParameter11a = this.__inoutParameter11a;
if (!this.__inoutParameter11aSet)
{
// inoutParameter has no pre constraints
inoutParameter11a = handleIsInoutParameter();
// inoutParameter has no post constraints
this.__inoutParameter11a = inoutParameter11a;
if (isMetafacadePropertyCachingEnabled())
{
this.__inoutParameter11aSet = true;
}
}
return inoutParameter11a;
}
/**
* @see ParameterFacade#getUpper()
* @return int
*/
protected abstract int handleGetUpper();
private int __upper12a;
private boolean __upper12aSet = false;
/**
*
* the upper value of the multiplicity (will be -1 for *)
*
*
* -only applicable for UML2
*
* @return (int)handleGetUpper()
*/
public final int getUpper()
{
int upper12a = this.__upper12a;
if (!this.__upper12aSet)
{
// upper has no pre constraints
upper12a = handleGetUpper();
// upper has no post constraints
this.__upper12a = upper12a;
if (isMetafacadePropertyCachingEnabled())
{
this.__upper12aSet = true;
}
}
return upper12a;
}
/**
* @see ParameterFacade#getLower()
* @return int
*/
protected abstract int handleGetLower();
private int __lower13a;
private boolean __lower13aSet = false;
/**
*
* the lower value for the multiplicity
*
*
* -only applicable for UML2
*
* @return (int)handleGetLower()
*/
public final int getLower()
{
int lower13a = this.__lower13a;
if (!this.__lower13aSet)
{
// lower has no pre constraints
lower13a = handleGetLower();
// lower has no post constraints
this.__lower13a = lower13a;
if (isMetafacadePropertyCachingEnabled())
{
this.__lower13aSet = true;
}
}
return lower13a;
}
/**
* @see ParameterFacade#isUnique()
* @return boolean
*/
protected abstract boolean handleIsUnique();
private boolean __unique14a;
private boolean __unique14aSet = false;
/**
*
* If Parameter type isMany (UML2), is the parameter unique within
* the Collection. Unique+Sorted determines pareter implementation
* type. For UML14, always false.
*
* @return (boolean)handleIsUnique()
*/
public final boolean isUnique()
{
boolean unique14a = this.__unique14a;
if (!this.__unique14aSet)
{
// unique has no pre constraints
unique14a = handleIsUnique();
// unique has no post constraints
this.__unique14a = unique14a;
if (isMetafacadePropertyCachingEnabled())
{
this.__unique14aSet = true;
}
}
return unique14a;
}
/**
* @see ParameterFacade#isOrdered()
* @return boolean
*/
protected abstract boolean handleIsOrdered();
private boolean __ordered15a;
private boolean __ordered15aSet = false;
/**
*
* UML2 Only: Is parameter ordered within the Collection type.
* Ordered+Unique determines the implementation Collection Type.
* For UML14, always false.
*
* @return (boolean)handleIsOrdered()
*/
public final boolean isOrdered()
{
boolean ordered15a = this.__ordered15a;
if (!this.__ordered15aSet)
{
// ordered has no pre constraints
ordered15a = handleIsOrdered();
// ordered has no post constraints
this.__ordered15a = ordered15a;
if (isMetafacadePropertyCachingEnabled())
{
this.__ordered15aSet = true;
}
}
return ordered15a;
}
/**
* @see ParameterFacade#isMany()
* @return boolean
*/
protected abstract boolean handleIsMany();
private boolean __many16a;
private boolean __many16aSet = false;
/**
*
* If upper>1 or upper==unlimited. Only applies to UML2. For UML14,
* always false.
*
* @return (boolean)handleIsMany()
*/
public final boolean isMany()
{
boolean many16a = this.__many16a;
if (!this.__many16aSet)
{
// many has no pre constraints
many16a = handleIsMany();
// many has no post constraints
this.__many16a = many16a;
if (isMetafacadePropertyCachingEnabled())
{
this.__many16aSet = true;
}
}
return many16a;
}
/**
* @see ParameterFacade#getGetterSetterTypeName()
* @return String
*/
protected abstract String handleGetGetterSetterTypeName();
private String __getterSetterTypeName17a;
private boolean __getterSetterTypeName17aSet = false;
/**
*
* Fully Qualified TypeName, determined in part by multiplicity
* (for UML2). For UML14, same as getterName.
*
* @return (String)handleGetGetterSetterTypeName()
*/
public final String getGetterSetterTypeName()
{
String getterSetterTypeName17a = this.__getterSetterTypeName17a;
if (!this.__getterSetterTypeName17aSet)
{
// getterSetterTypeName has no pre constraints
getterSetterTypeName17a = handleGetGetterSetterTypeName();
// getterSetterTypeName has no post constraints
this.__getterSetterTypeName17a = getterSetterTypeName17a;
if (isMetafacadePropertyCachingEnabled())
{
this.__getterSetterTypeName17aSet = true;
}
}
return getterSetterTypeName17a;
}
/**
* @see ParameterFacade#isException()
* @return boolean
*/
protected abstract boolean handleIsException();
private boolean __exception18a;
private boolean __exception18aSet = false;
/**
*
* UML2: Returns the value of the 'Is Exception' attribute. The
* default value is "false". Tells whether an output parameter may
* emit a value to the exclusion of the other outputs.
*
* @return (boolean)handleIsException()
*/
public final boolean isException()
{
boolean exception18a = this.__exception18a;
if (!this.__exception18aSet)
{
// exception has no pre constraints
exception18a = handleIsException();
// exception has no post constraints
this.__exception18a = exception18a;
if (isMetafacadePropertyCachingEnabled())
{
this.__exception18aSet = true;
}
}
return exception18a;
}
/**
* @see ParameterFacade#getEffect()
* @return String
*/
protected abstract String handleGetEffect();
private String __effect19a;
private boolean __effect19aSet = false;
/**
*
* UML2: A representation of the literals of the enumeration
* 'Parameter Effect Kind': CREATE, READ, UPDATE, DELETE. The
* datatype ParameterEffectKind is an enumeration that indicates
* the effect of a behavior on values passed in or out of its
* parameters.
*
* @return (String)handleGetEffect()
*/
public final String getEffect()
{
String effect19a = this.__effect19a;
if (!this.__effect19aSet)
{
// effect has no pre constraints
effect19a = handleGetEffect();
// effect has no post constraints
this.__effect19a = effect19a;
if (isMetafacadePropertyCachingEnabled())
{
this.__effect19aSet = true;
}
}
return effect19a;
}
/**
* @see ParameterFacade#getGetterSetterTypeNameImpl()
* @return String
*/
protected abstract String handleGetGetterSetterTypeNameImpl();
private String __getterSetterTypeNameImpl20a;
private boolean __getterSetterTypeNameImpl20aSet = false;
/**
*
* Fully Qualified implementation class of TypeName, determined in
* part by multiplicity (for UML2). If upper multiplicity =1, same
* as getterSetterTypeName.
*
* @return (String)handleGetGetterSetterTypeNameImpl()
*/
public final String getGetterSetterTypeNameImpl()
{
String getterSetterTypeNameImpl20a = this.__getterSetterTypeNameImpl20a;
if (!this.__getterSetterTypeNameImpl20aSet)
{
// getterSetterTypeNameImpl has no pre constraints
getterSetterTypeNameImpl20a = handleGetGetterSetterTypeNameImpl();
// getterSetterTypeNameImpl has no post constraints
this.__getterSetterTypeNameImpl20a = getterSetterTypeNameImpl20a;
if (isMetafacadePropertyCachingEnabled())
{
this.__getterSetterTypeNameImpl20aSet = true;
}
}
return getterSetterTypeNameImpl20a;
}
// ------------- associations ------------------
/**
*
* @return (OperationFacade)handleGetOperation()
*/
public final OperationFacade getOperation()
{
OperationFacade getOperation1r = null;
// parameters has no pre constraints
Object result = handleGetOperation();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getOperation1r = (OperationFacade)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
ParameterFacadeLogic.logger.warn("incorrect metafacade cast for ParameterFacadeLogic.getOperation OperationFacade " + result + ": " + shieldedResult);
}
// parameters has no post constraints
return getOperation1r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetOperation();
/**
*
* The parameters to this event.
*
* @return (EventFacade)handleGetEvent()
*/
public final EventFacade getEvent()
{
EventFacade getEvent2r = null;
// parameters has no pre constraints
Object result = handleGetEvent();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getEvent2r = (EventFacade)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
ParameterFacadeLogic.logger.warn("incorrect metafacade cast for ParameterFacadeLogic.getEvent EventFacade " + result + ": " + shieldedResult);
}
// parameters has no post constraints
return getEvent2r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetEvent();
/**
*
* @return (ClassifierFacade)handleGetType()
*/
public final ClassifierFacade getType()
{
ClassifierFacade getType3r = null;
// parameterFacade has no pre constraints
Object result = handleGetType();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getType3r = (ClassifierFacade)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
ParameterFacadeLogic.logger.warn("incorrect metafacade cast for ParameterFacadeLogic.getType ClassifierFacade " + result + ": " + shieldedResult);
}
// parameterFacade has no post constraints
return getType3r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetType();
/**
* Constraint: org::andromda::metafacades::uml::ParameterFacade::parameter needs a type
* Error: Each parameter needs a type, you cannot leave the type unspecified.
* OCL: context ParameterFacade
inv: return = false implies type->notEmpty() and type.name->notEmpty()
* Constraint: org::andromda::metafacades::uml::ParameterFacade::non return type parameters must be named
* Error: Each parameter that is NOT a return parameter must have a non-empty name.
* OCL: context ParameterFacade
inv: return = false
implies name -> notEmpty()
* Constraint: org::andromda::metafacades::uml::ParameterFacade::primitive parameter cannot be used in Collection
* Error: Primitive parameters cannot be used in Collections (multiplicity > 1). Use the wrapped type instead.
* OCL: context ParameterFacade inv: type.primitive implies (many = false)
* Constraint: org::andromda::metafacades::uml::ParameterFacade::primitive parameter must be required
* Error: Primitive operation parameters must have a multiplicity lower bound > 0 (must be required). Use a wrapped type, or change the multiplicity.
* OCL: context ParameterFacade inv: type.primitive implies (lower > 0)
* Constraint: org::andromda::metafacades::uml::ParameterFacade::wrapped primitive parameter should not be required
* Error: Wrapped primitive operation parameters must have a multiplicity lower bound = 0 (must be optional). Use the unwrapped type, or change the multiplicity.
* OCL: context ParameterFacade inv: type.wrappedPrimitive and many = false implies (lower = 0)
* @param validationMessages Collection
* @see ModelElementFacadeLogicImpl#validateInvariants(Collection validationMessages)
*/
@Override
public void validateInvariants(Collection validationMessages)
{
super.validateInvariants(validationMessages);
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure((Boolean.valueOf(String.valueOf(OCLExpressions.equal(OCLIntrospector.invoke(contextElement,"return"),false))).booleanValue()?OCLCollections.notEmpty(OCLIntrospector.invoke(contextElement,"type")):true)&&OCLCollections.notEmpty(OCLIntrospector.invoke(contextElement,"type.name")));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::ParameterFacade::parameter needs a type",
"Each parameter needs a type, you cannot leave the type unspecified."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::ParameterFacade::parameter needs a type' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure((Boolean.valueOf(String.valueOf(OCLExpressions.equal(OCLIntrospector.invoke(contextElement,"return"),false))).booleanValue()?OCLCollections.notEmpty(OCLIntrospector.invoke(contextElement,"name")):true));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::ParameterFacade::non return type parameters must be named",
"Each parameter that is NOT a return parameter must have a non-empty name."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::ParameterFacade::non return type parameters must be named' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure((Boolean.valueOf(String.valueOf(OCLIntrospector.invoke(contextElement,"type.primitive"))).booleanValue()?(OCLExpressions.equal(OCLIntrospector.invoke(contextElement,"many"),false)):true));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::ParameterFacade::primitive parameter cannot be used in Collection",
"Primitive parameters cannot be used in Collections (multiplicity > 1). Use the wrapped type instead."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::ParameterFacade::primitive parameter cannot be used in Collection' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure((Boolean.valueOf(String.valueOf(OCLIntrospector.invoke(contextElement,"type.primitive"))).booleanValue()?(OCLExpressions.greater(OCLIntrospector.invoke(contextElement,"lower"),0)):true));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::ParameterFacade::primitive parameter must be required",
"Primitive operation parameters must have a multiplicity lower bound > 0 (must be required). Use a wrapped type, or change the multiplicity."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::ParameterFacade::primitive parameter must be required' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure((Boolean.valueOf(String.valueOf(Boolean.valueOf(String.valueOf(OCLIntrospector.invoke(contextElement,"type.wrappedPrimitive"))).booleanValue()&&OCLExpressions.equal(OCLIntrospector.invoke(contextElement,"many"),false))).booleanValue()?(OCLExpressions.equal(OCLIntrospector.invoke(contextElement,"lower"),0)):true));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::ParameterFacade::wrapped primitive parameter should not be required",
"Wrapped primitive operation parameters must have a multiplicity lower bound = 0 (must be optional). Use the unwrapped type, or change the multiplicity."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::ParameterFacade::wrapped primitive parameter should not be required' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
}
}