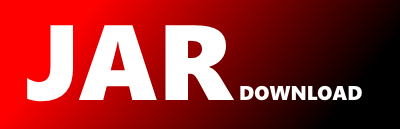
org.andromda.metafacades.emf.uml22.StateMachineFacadeLogic Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of andromda-metafacades-emf-uml22 Show documentation
Show all versions of andromda-metafacades-emf-uml22 Show documentation
The Eclipse EMF UML2 v2.X metafacades. This is the set of EMF UML2 2.X metafacades
implementations. These implement the common UML metafacades for .uml model files.
The newest version!
// license-header java merge-point
//
// Attention: generated code (by MetafacadeLogic.vsl) - do not modify!
//
package org.andromda.metafacades.emf.uml22;
import java.util.Collection;
import java.util.List;
import org.andromda.core.metafacade.MetafacadeBase;
import org.andromda.core.metafacade.ModelValidationMessage;
import org.andromda.metafacades.uml.FinalStateFacade;
import org.andromda.metafacades.uml.ModelElementFacade;
import org.andromda.metafacades.uml.PseudostateFacade;
import org.andromda.metafacades.uml.StateFacade;
import org.andromda.metafacades.uml.StateMachineFacade;
import org.andromda.metafacades.uml.TransitionFacade;
import org.apache.log4j.Logger;
import org.eclipse.uml2.uml.StateMachine;
/**
*
* State machines can be used to express the behavior of part of a
* system. Behavior is modeled as a traversal of a graph of state
* nodes interconnected by one or more joined transition arcs that
* are triggered by the dispatching of series of (event)
* occurrences. During this traversal, the state machine executes a
* series of activities associated with various elements of the
* state machine.
*
* MetafacadeLogic for StateMachineFacade
*
* @see StateMachineFacade
*/
public abstract class StateMachineFacadeLogic
extends ModelElementFacadeLogicImpl
implements StateMachineFacade
{
/**
* The underlying UML object
* @see StateMachine
*/
protected StateMachine metaObject;
/** Create Metafacade implementation instance using the MetafacadeFactory from the context
* @param metaObjectIn
* @param context
*/
protected StateMachineFacadeLogic(StateMachine metaObjectIn, String context)
{
super(metaObjectIn, getContext(context));
this.metaObject = metaObjectIn;
}
/**
* The logger instance.
*/
private static final Logger logger = Logger.getLogger(StateMachineFacadeLogic.class);
/**
* Gets the context for this metafacade logic instance.
* @param context String. Set to StateMachineFacade if null
* @return context String
*/
private static String getContext(String context)
{
if (context == null)
{
context = "org.andromda.metafacades.uml.StateMachineFacade";
}
return context;
}
/** Reset context only for non-root metafacades
* @param context
*/
@Override
public void resetMetafacadeContext(String context)
{
if (!this.contextRoot) // reset context only for non-root metafacades
{
context = getContext(context); // to have same value as in original constructor call
setMetafacadeContext (context);
}
}
/**
* @return boolean true always
* @see StateMachineFacade
*/
public boolean isStateMachineFacadeMetaType()
{
return true;
}
// ------------- associations ------------------
/**
*
* If this model element is the context of an activity graph, this
* represents that activity graph.
*
* @return (ModelElementFacade)handleGetContextElement()
*/
public final ModelElementFacade getContextElement()
{
ModelElementFacade getContextElement1r = null;
// stateMachineContext has no pre constraints
Object result = handleGetContextElement();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getContextElement1r = (ModelElementFacade)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
StateMachineFacadeLogic.logger.warn("incorrect metafacade cast for StateMachineFacadeLogic.getContextElement ModelElementFacade " + result + ": " + shieldedResult);
}
// stateMachineContext has no post constraints
return getContextElement1r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetContextElement();
/**
*
* @return (Collection)handleGetFinalStates()
*/
public final Collection getFinalStates()
{
Collection getFinalStates2r = null;
// stateMachineFacade has no pre constraints
Collection result = handleGetFinalStates();
List shieldedResult = this.shieldedElements(result);
try
{
getFinalStates2r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
StateMachineFacadeLogic.logger.warn("incorrect metafacade cast for StateMachineFacadeLogic.getFinalStates Collection " + result + ": " + shieldedResult);
}
// stateMachineFacade has no post constraints
return getFinalStates2r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetFinalStates();
private TransitionFacade __getInitialTransition4r;
private boolean __getInitialTransition4rSet = false;
/**
*
* @return (TransitionFacade)handleGetInitialTransition()
*/
public final TransitionFacade getInitialTransition()
{
TransitionFacade getInitialTransition4r = this.__getInitialTransition4r;
if (!this.__getInitialTransition4rSet)
{
// stateMachineFacade has no pre constraints
Object result = handleGetInitialTransition();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getInitialTransition4r = (TransitionFacade)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
StateMachineFacadeLogic.logger.warn("incorrect metafacade cast for StateMachineFacadeLogic.getInitialTransition TransitionFacade " + result + ": " + shieldedResult);
}
// stateMachineFacade has no post constraints
this.__getInitialTransition4r = getInitialTransition4r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getInitialTransition4rSet = true;
}
}
return getInitialTransition4r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetInitialTransition();
/**
*
* @return (Collection)handleGetTransitions()
*/
public final Collection getTransitions()
{
Collection getTransitions5r = null;
// stateMachineFacade has no pre constraints
Collection result = handleGetTransitions();
List shieldedResult = this.shieldedElements(result);
try
{
getTransitions5r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
StateMachineFacadeLogic.logger.warn("incorrect metafacade cast for StateMachineFacadeLogic.getTransitions Collection " + result + ": " + shieldedResult);
}
// stateMachineFacade has no post constraints
return getTransitions5r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetTransitions();
/**
*
* @return (Collection)handleGetInitialStates()
*/
public final Collection getInitialStates()
{
Collection getInitialStates6r = null;
// stateMachineFacade has no pre constraints
Collection result = handleGetInitialStates();
List shieldedResult = this.shieldedElements(result);
try
{
getInitialStates6r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
StateMachineFacadeLogic.logger.warn("incorrect metafacade cast for StateMachineFacadeLogic.getInitialStates Collection " + result + ": " + shieldedResult);
}
// stateMachineFacade has no post constraints
return getInitialStates6r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetInitialStates();
private PseudostateFacade __getInitialState7r;
private boolean __getInitialState7rSet = false;
/**
*
* @return (PseudostateFacade)handleGetInitialState()
*/
public final PseudostateFacade getInitialState()
{
PseudostateFacade getInitialState7r = this.__getInitialState7r;
if (!this.__getInitialState7rSet)
{
// stateMachineFacade has no pre constraints
Object result = handleGetInitialState();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getInitialState7r = (PseudostateFacade)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
StateMachineFacadeLogic.logger.warn("incorrect metafacade cast for StateMachineFacadeLogic.getInitialState PseudostateFacade " + result + ": " + shieldedResult);
}
// stateMachineFacade has no post constraints
this.__getInitialState7r = getInitialState7r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getInitialState7rSet = true;
}
}
return getInitialState7r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetInitialState();
/**
*
* @return (Collection)handleGetPseudostates()
*/
public final Collection getPseudostates()
{
Collection getPseudostates8r = null;
// stateMachineFacade has no pre constraints
Collection result = handleGetPseudostates();
List shieldedResult = this.shieldedElements(result);
try
{
getPseudostates8r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
StateMachineFacadeLogic.logger.warn("incorrect metafacade cast for StateMachineFacadeLogic.getPseudostates Collection " + result + ": " + shieldedResult);
}
// stateMachineFacade has no post constraints
return getPseudostates8r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetPseudostates();
private Collection __getStates10r;
private boolean __getStates10rSet = false;
/**
*
* @return (Collection)handleGetStates()
*/
public final Collection getStates()
{
Collection getStates10r = this.__getStates10r;
if (!this.__getStates10rSet)
{
// stateMachineFacade has no pre constraints
Collection result = handleGetStates();
List shieldedResult = this.shieldedElements(result);
try
{
getStates10r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
StateMachineFacadeLogic.logger.warn("incorrect metafacade cast for StateMachineFacadeLogic.getStates Collection " + result + ": " + shieldedResult);
}
// stateMachineFacade has no post constraints
this.__getStates10r = getStates10r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getStates10rSet = true;
}
}
return getStates10r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetStates();
/**
* @param validationMessages Collection
* @see ModelElementFacadeLogicImpl#validateInvariants(Collection validationMessages)
*/
@Override
public void validateInvariants(Collection validationMessages)
{
super.validateInvariants(validationMessages);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy