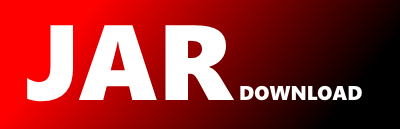
org.andromda.metafacades.uml14.AssociationClassFacadeLogic Maven / Gradle / Ivy
// license-header java merge-point
//
// Attention: generated code (by MetafacadeLogic.vsl) - do not modify!
//
package org.andromda.metafacades.uml14;
import java.util.Collection;
import java.util.List;
import org.andromda.core.common.Introspector;
import org.andromda.core.metafacade.MetafacadeBase;
import org.andromda.core.metafacade.MetafacadeFactory;
import org.andromda.core.metafacade.ModelValidationMessage;
import org.andromda.metafacades.uml.AssociationClassFacade;
import org.andromda.metafacades.uml.AssociationEndFacade;
import org.andromda.metafacades.uml.AssociationFacade;
import org.andromda.metafacades.uml.AttributeFacade;
import org.andromda.metafacades.uml.ClassifierFacade;
import org.andromda.metafacades.uml.ConstraintFacade;
import org.andromda.metafacades.uml.DependencyFacade;
import org.andromda.metafacades.uml.GeneralizableElementFacade;
import org.andromda.metafacades.uml.GeneralizationFacade;
import org.andromda.metafacades.uml.ModelElementFacade;
import org.andromda.metafacades.uml.ModelFacade;
import org.andromda.metafacades.uml.OperationFacade;
import org.andromda.metafacades.uml.PackageFacade;
import org.andromda.metafacades.uml.StateMachineFacade;
import org.andromda.metafacades.uml.StereotypeFacade;
import org.andromda.metafacades.uml.TaggedValueFacade;
import org.andromda.metafacades.uml.TemplateParameterFacade;
import org.andromda.metafacades.uml.TypeMappings;
import org.apache.log4j.Logger;
import org.omg.uml.foundation.core.AssociationClass;
/**
*
* A model element that has both association and class properties.
* An AssociationClass can be seen as an association that also has
* class properties, or as a class that also has association
* properties. It not only connects a set of classifiers but also
* defines a set of features that belong to the relationship itself
* and not to any of the classifiers.
*
* MetafacadeLogic for AssociationClassFacade
*
* @see AssociationClassFacade
*/
public abstract class AssociationClassFacadeLogic
extends MetafacadeBase
implements AssociationClassFacade
{
/**
* The underlying UML object
* @see AssociationClass
*/
protected AssociationClass metaObject;
/** Create Metafacade implementation instance using the MetafacadeFactory from the context
* @param metaObjectIn
* @param context
*/
protected AssociationClassFacadeLogic(AssociationClass metaObjectIn, String context)
{
super(metaObjectIn, getContext(context));
this.superClassifierFacade =
(ClassifierFacade)
MetafacadeFactory.getInstance().createFacadeImpl(
"org.andromda.metafacades.uml.ClassifierFacade",
metaObjectIn,
getContext(context));
this.superAssociationFacade =
(AssociationFacade)
MetafacadeFactory.getInstance().createFacadeImpl(
"org.andromda.metafacades.uml.AssociationFacade",
metaObjectIn,
getContext(context));
this.metaObject = metaObjectIn;
}
/**
* The logger instance.
*/
private static final Logger logger = Logger.getLogger(AssociationClassFacadeLogic.class);
/**
* Gets the context for this metafacade logic instance.
* @param context String. Set to AssociationClassFacade if null
* @return context String
*/
private static String getContext(String context)
{
if (context == null)
{
context = "org.andromda.metafacades.uml.AssociationClassFacade";
}
return context;
}
private ClassifierFacade superClassifierFacade;
private boolean superClassifierFacadeInitialized = false;
/**
* Gets the ClassifierFacade parent instance.
* @return this.superClassifierFacade ClassifierFacade
*/
protected ClassifierFacade getSuperClassifierFacade()
{
if (!this.superClassifierFacadeInitialized)
{
((MetafacadeBase)this.superClassifierFacade).setMetafacadeContext(this.getMetafacadeContext());
this.superClassifierFacadeInitialized = true;
}
return this.superClassifierFacade;
}
private AssociationFacade superAssociationFacade;
private boolean superAssociationFacadeInitialized = false;
/**
* Gets the AssociationFacade parent instance.
* @return this.superAssociationFacade AssociationFacade
*/
protected AssociationFacade getSuperAssociationFacade()
{
if (!this.superAssociationFacadeInitialized)
{
((MetafacadeBase)this.superAssociationFacade).setMetafacadeContext(this.getMetafacadeContext());
this.superAssociationFacadeInitialized = true;
}
return this.superAssociationFacade;
}
/** Reset context only for non-root metafacades
* @param context
* @see org.andromda.core.metafacade.MetafacadeBase#resetMetafacadeContext(String context)
*/
@Override
public void resetMetafacadeContext(String context)
{
if (!this.contextRoot) // reset context only for non-root metafacades
{
context = getContext(context); // to have same value as in original constructor call
setMetafacadeContext (context);
if (this.superClassifierFacadeInitialized)
{
((MetafacadeBase)this.superClassifierFacade).resetMetafacadeContext(context);
}
if (this.superAssociationFacadeInitialized)
{
((MetafacadeBase)this.superAssociationFacade).resetMetafacadeContext(context);
}
}
}
/**
* @return boolean true always
* @see AssociationClassFacade
*/
public boolean isAssociationClassFacadeMetaType()
{
return true;
}
// ------------- associations ------------------
/**
*
* @return (Collection)handleGetConnectionAssociationEnds()
*/
public final Collection getConnectionAssociationEnds()
{
Collection getConnectionAssociationEnds1r = null;
// associationClassFacade has no pre constraints
Collection result = handleGetConnectionAssociationEnds();
List shieldedResult = this.shieldedElements(result);
try
{
getConnectionAssociationEnds1r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
AssociationClassFacadeLogic.logger.warn("incorrect metafacade cast for AssociationClassFacadeLogic.getConnectionAssociationEnds Collection " + result + ": " + shieldedResult);
}
// associationClassFacade has no post constraints
return getConnectionAssociationEnds1r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetConnectionAssociationEnds();
/**
* @return true
* @see ClassifierFacade
*/
public boolean isClassifierFacadeMetaType()
{
return true;
}
/**
* @return true
* @see AssociationFacade
*/
public boolean isAssociationFacadeMetaType()
{
return true;
}
/**
* @return true
* @see GeneralizableElementFacade
*/
public boolean isGeneralizableElementFacadeMetaType()
{
return true;
}
/**
* @return true
* @see ModelElementFacade
*/
public boolean isModelElementFacadeMetaType()
{
return true;
}
// ----------- delegates to ClassifierFacade ------------
/**
*
* Return the attribute which name matches the parameter
*
* @see ClassifierFacade#findAttribute(String name)
*/
public AttributeFacade findAttribute(String name)
{
return this.getSuperClassifierFacade().findAttribute(name);
}
/**
*
* Those abstraction dependencies for which this classifier is the
* client.
*
* @see ClassifierFacade#getAbstractions()
*/
public Collection getAbstractions()
{
return this.getSuperClassifierFacade().getAbstractions();
}
/**
*
* Lists all classes associated to this one and any ancestor
* classes (through generalization). There will be no duplicates.
* The order of the elements is predictable.
*
* @see ClassifierFacade#getAllAssociatedClasses()
*/
public Collection getAllAssociatedClasses()
{
return this.getSuperClassifierFacade().getAllAssociatedClasses();
}
/**
*
* A collection containing all 'properties' of the classifier and
* its ancestors. Properties are any attributes and navigable
* connecting association ends.
*
* @see ClassifierFacade#getAllProperties()
*/
public Collection getAllProperties()
{
return this.getSuperClassifierFacade().getAllProperties();
}
/**
*
* A collection containing all required and/or read-only
* 'properties' of the classifier and its ancestors. Properties are
* any attributes and navigable connecting association ends.
*
* @see ClassifierFacade#getAllRequiredConstructorParameters()
*/
public Collection getAllRequiredConstructorParameters()
{
return this.getSuperClassifierFacade().getAllRequiredConstructorParameters();
}
/**
*
* Gets the array type for this classifier. If this classifier
* already represents an array, it just returns itself.
*
* @see ClassifierFacade#getArray()
*/
public ClassifierFacade getArray()
{
return this.getSuperClassifierFacade().getArray();
}
/**
*
* The name of the classifier as an array.
*
* @see ClassifierFacade#getArrayName()
*/
public String getArrayName()
{
return this.getSuperClassifierFacade().getArrayName();
}
/**
*
* Lists the classes associated to this one, there is no repitition
* of classes. The order of the elements is predictable.
*
* @see ClassifierFacade#getAssociatedClasses()
*/
public Collection getAssociatedClasses()
{
return this.getSuperClassifierFacade().getAssociatedClasses();
}
/**
*
* Gets the association ends belonging to a classifier.
*
* @see ClassifierFacade#getAssociationEnds()
*/
public List getAssociationEnds()
{
return this.getSuperClassifierFacade().getAssociationEnds();
}
/**
*
* Gets the attributes that belong to the classifier.
*
* @see ClassifierFacade#getAttributes()
*/
public List getAttributes()
{
return this.getSuperClassifierFacade().getAttributes();
}
/**
*
* Gets all attributes for the classifier and if 'follow' is true
* goes up the inheritance hierarchy and gets the attributes from
* the super classes as well.
*
* @see ClassifierFacade#getAttributes(boolean follow)
*/
public List getAttributes(boolean follow)
{
return this.getSuperClassifierFacade().getAttributes(follow);
}
/**
*
* The fully qualified name of the classifier as an array.
*
* @see ClassifierFacade#getFullyQualifiedArrayName()
*/
public String getFullyQualifiedArrayName()
{
return this.getSuperClassifierFacade().getFullyQualifiedArrayName();
}
/**
*
* Returns all those operations that could be implemented at this
* classifier's level. This means the operations owned by this
* classifier as well as any realized interface's operations
* (recursively) in case this classifier itself is not already an
* interface, or generalized when this classifier is an interface.
*
* @see ClassifierFacade#getImplementationOperations()
*/
public Collection getImplementationOperations()
{
return this.getSuperClassifierFacade().getImplementationOperations();
}
/**
*
* A comma separated list of the fully qualified names of all
* implemented interfaces.
*
* @see ClassifierFacade#getImplementedInterfaceList()
*/
public String getImplementedInterfaceList()
{
return this.getSuperClassifierFacade().getImplementedInterfaceList();
}
/**
*
* Those attributes that are scoped to an instance of this class.
*
* @see ClassifierFacade#getInstanceAttributes()
*/
public Collection getInstanceAttributes()
{
return this.getSuperClassifierFacade().getInstanceAttributes();
}
/**
*
* Those operations that are scoped to an instance of this class.
*
* @see ClassifierFacade#getInstanceOperations()
*/
public List getInstanceOperations()
{
return this.getSuperClassifierFacade().getInstanceOperations();
}
/**
*
* Those interfaces that are abstractions of this classifier, this
* basically means this classifier realizes them.
*
* @see ClassifierFacade#getInterfaceAbstractions()
*/
public Collection getInterfaceAbstractions()
{
return this.getSuperClassifierFacade().getInterfaceAbstractions();
}
/**
*
* A String representing a new Constructor declaration for this
* classifier type to be used in a Java environment.
*
* @see ClassifierFacade#getJavaNewString()
*/
public String getJavaNewString()
{
return this.getSuperClassifierFacade().getJavaNewString();
}
/**
*
* A String representing the null-value for this classifier type to
* be used in a Java environment.
*
* @see ClassifierFacade#getJavaNullString()
*/
public String getJavaNullString()
{
return this.getSuperClassifierFacade().getJavaNullString();
}
/**
*
* The other ends of this classifier's association ends which are
* navigable.
*
* @see ClassifierFacade#getNavigableConnectingEnds()
*/
public Collection getNavigableConnectingEnds()
{
return this.getSuperClassifierFacade().getNavigableConnectingEnds();
}
/**
*
* Get the other ends of this classifier's association ends which
* are navigable and if 'follow' is true goes up the inheritance
* hierarchy and gets the super association ends as well.
*
* @see ClassifierFacade#getNavigableConnectingEnds(boolean follow)
*/
public List getNavigableConnectingEnds(boolean follow)
{
return this.getSuperClassifierFacade().getNavigableConnectingEnds(follow);
}
/**
*
* Assuming that the classifier is an array, this will return the
* non array type of the classifier from
*
*
* the model. If the classifier is NOT an array, it will just
* return itself.
*
* @see ClassifierFacade#getNonArray()
*/
public ClassifierFacade getNonArray()
{
return this.getSuperClassifierFacade().getNonArray();
}
/**
*
* The attributes from this classifier in the form of an operation
* call (this example would be in Java): '(String attributeOne,
* String attributeTwo). If there were no attributes on the
* classifier, the result would be an empty '()'.
*
* @see ClassifierFacade#getOperationCallFromAttributes()
*/
public String getOperationCallFromAttributes()
{
return this.getSuperClassifierFacade().getOperationCallFromAttributes();
}
/**
*
* The operations owned by this classifier.
*
* @see ClassifierFacade#getOperations()
*/
public List getOperations()
{
return this.getSuperClassifierFacade().getOperations();
}
/**
*
* A collection containing all 'properties' of the classifier.
* Properties are any attributes and navigable connecting
* association ends.
*
* @see ClassifierFacade#getProperties()
*/
public List getProperties()
{
return this.getSuperClassifierFacade().getProperties();
}
/**
*
* Gets all properties (attributes and navigable association ends)
* for the classifier and if 'follow' is true goes up the
* inheritance hierarchy and gets the properties from the super
* classes as well.
*
* @see ClassifierFacade#getProperties(boolean follow)
*/
public List getProperties(boolean follow)
{
return this.getSuperClassifierFacade().getProperties(follow);
}
/**
*
* A collection containing all required and/or read-only
* 'properties' of the classifier. Properties are any attributes
* and navigable connecting association ends.
*
* @see ClassifierFacade#getRequiredConstructorParameters()
*/
public Collection getRequiredConstructorParameters()
{
return this.getSuperClassifierFacade().getRequiredConstructorParameters();
}
/**
*
* Returns the serial version UID of the underlying model element.
*
* @see ClassifierFacade#getSerialVersionUID()
*/
public long getSerialVersionUID()
{
return this.getSuperClassifierFacade().getSerialVersionUID();
}
/**
*
* Those attributes that are scoped to the definition of this
* class.
*
* @see ClassifierFacade#getStaticAttributes()
*/
public Collection getStaticAttributes()
{
return this.getSuperClassifierFacade().getStaticAttributes();
}
/**
*
* Those operations that are scoped to the definition of this
* class.
*
* @see ClassifierFacade#getStaticOperations()
*/
public List getStaticOperations()
{
return this.getSuperClassifierFacade().getStaticOperations();
}
/**
*
* This class' superclass, returns the generalization if it is a
* ClassifierFacade, null otherwise.
*
* @see ClassifierFacade#getSuperClass()
*/
public ClassifierFacade getSuperClass()
{
return this.getSuperClassifierFacade().getSuperClass();
}
/**
*
* The wrapper name for this classifier if a mapped type has a
* defined wrapper class (ie. 'long' maps to 'Long'). If the
* classifier doesn't have a wrapper defined for it, this method
* will return a null. Note that wrapper mappings must be defined
* for the namespace by defining the 'wrapperMappingsUri', this
* property must point to the location of the mappings file which
* maps the primitives to wrapper types.
*
* @see ClassifierFacade#getWrapperName()
*/
public String getWrapperName()
{
return this.getSuperClassifierFacade().getWrapperName();
}
/**
*
* Indicates if this classifier is 'abstract'.
*
* @see ClassifierFacade#isAbstract()
*/
public boolean isAbstract()
{
return this.getSuperClassifierFacade().isAbstract();
}
/**
*
* True if this classifier represents an array type. False
* otherwise.
*
* @see ClassifierFacade#isArrayType()
*/
public boolean isArrayType()
{
return this.getSuperClassifierFacade().isArrayType();
}
/**
*
* @see ClassifierFacade#isAssociationClass()
*/
public boolean isAssociationClass()
{
return this.getSuperClassifierFacade().isAssociationClass();
}
/**
*
* Returns true if this type represents a Blob type.
*
* @see ClassifierFacade#isBlobType()
*/
public boolean isBlobType()
{
return this.getSuperClassifierFacade().isBlobType();
}
/**
*
* Indicates if this type represents a boolean type or not.
*
* @see ClassifierFacade#isBooleanType()
*/
public boolean isBooleanType()
{
return this.getSuperClassifierFacade().isBooleanType();
}
/**
*
* Indicates if this type represents a char, Character, or
* java.lang.Character type or not.
*
* @see ClassifierFacade#isCharacterType()
*/
public boolean isCharacterType()
{
return this.getSuperClassifierFacade().isCharacterType();
}
/**
*
* Returns true if this type represents a Clob type.
*
* @see ClassifierFacade#isClobType()
*/
public boolean isClobType()
{
return this.getSuperClassifierFacade().isClobType();
}
/**
*
* True if this classifier represents a collection type. False
* otherwise.
*
* @see ClassifierFacade#isCollectionType()
*/
public boolean isCollectionType()
{
return this.getSuperClassifierFacade().isCollectionType();
}
/**
*
* True/false depending on whether or not this classifier
* represents a datatype. A data type is a type whose instances are
* identified only by their value. A data type may contain
* attributes to support the modeling of structured data types.
*
* @see ClassifierFacade#isDataType()
*/
public boolean isDataType()
{
return this.getSuperClassifierFacade().isDataType();
}
/**
*
* True when this classifier is a date type.
*
* @see ClassifierFacade#isDateType()
*/
public boolean isDateType()
{
return this.getSuperClassifierFacade().isDateType();
}
/**
*
* Indicates if this type represents a Double type or not.
*
* @see ClassifierFacade#isDoubleType()
*/
public boolean isDoubleType()
{
return this.getSuperClassifierFacade().isDoubleType();
}
/**
*
* Indicates whether or not this classifier represents an
* "EmbeddedValue'.
*
* @see ClassifierFacade#isEmbeddedValue()
*/
public boolean isEmbeddedValue()
{
return this.getSuperClassifierFacade().isEmbeddedValue();
}
/**
*
* True if this classifier is in fact marked as an enumeration.
*
* @see ClassifierFacade#isEnumeration()
*/
public boolean isEnumeration()
{
return this.getSuperClassifierFacade().isEnumeration();
}
/**
*
* Returns true if this type represents a 'file' type.
*
* @see ClassifierFacade#isFileType()
*/
public boolean isFileType()
{
return this.getSuperClassifierFacade().isFileType();
}
/**
*
* Indicates if this type represents a Float type or not.
*
* @see ClassifierFacade#isFloatType()
*/
public boolean isFloatType()
{
return this.getSuperClassifierFacade().isFloatType();
}
/**
*
* Indicates if this type represents an int or Integer or
* java.lang.Integer type or not.
*
* @see ClassifierFacade#isIntegerType()
*/
public boolean isIntegerType()
{
return this.getSuperClassifierFacade().isIntegerType();
}
/**
*
* True/false depending on whether or not this Classifier
* represents an interface.
*
* @see ClassifierFacade#isInterface()
*/
public boolean isInterface()
{
return this.getSuperClassifierFacade().isInterface();
}
/**
*
* True if this classifier cannot be extended and represent a leaf
* in the inheritance tree.
*
* @see ClassifierFacade#isLeaf()
*/
public boolean isLeaf()
{
return this.getSuperClassifierFacade().isLeaf();
}
/**
*
* True if this classifier represents a list type. False otherwise.
*
* @see ClassifierFacade#isListType()
*/
public boolean isListType()
{
return this.getSuperClassifierFacade().isListType();
}
/**
*
* Indicates if this type represents a Long type or not.
*
* @see ClassifierFacade#isLongType()
*/
public boolean isLongType()
{
return this.getSuperClassifierFacade().isLongType();
}
/**
*
* Indicates whether or not this classifier represents a Map type.
*
* @see ClassifierFacade#isMapType()
*/
public boolean isMapType()
{
return this.getSuperClassifierFacade().isMapType();
}
/**
*
* Indicates whether or not this classifier represents a primitive
* type.
*
* @see ClassifierFacade#isPrimitive()
*/
public boolean isPrimitive()
{
return this.getSuperClassifierFacade().isPrimitive();
}
/**
*
* True if this classifier represents a set type. False otherwise.
*
* @see ClassifierFacade#isSetType()
*/
public boolean isSetType()
{
return this.getSuperClassifierFacade().isSetType();
}
/**
*
* Indicates whether or not this classifier represents a string
* type.
*
* @see ClassifierFacade#isStringType()
*/
public boolean isStringType()
{
return this.getSuperClassifierFacade().isStringType();
}
/**
*
* Indicates whether or not this classifier represents a time type.
*
* @see ClassifierFacade#isTimeType()
*/
public boolean isTimeType()
{
return this.getSuperClassifierFacade().isTimeType();
}
/**
*
* Returns true if this type is a wrapped primitive type.
*
* @see ClassifierFacade#isWrappedPrimitive()
*/
public boolean isWrappedPrimitive()
{
return this.getSuperClassifierFacade().isWrappedPrimitive();
}
/**
*
* Finds the tagged value optional searching the entire inheritance
* hierarchy if 'follow' is set to true.
*
* @see GeneralizableElementFacade#findTaggedValue(String tagName, boolean follow)
*/
public Object findTaggedValue(String tagName, boolean follow)
{
return this.getSuperClassifierFacade().findTaggedValue(tagName, follow);
}
/**
*
* All generalizations for this generalizable element, goes up the
* inheritance tree.
*
* @see GeneralizableElementFacade#getAllGeneralizations()
*/
public Collection getAllGeneralizations()
{
return this.getSuperClassifierFacade().getAllGeneralizations();
}
/**
*
* All specializations (travels down the inheritance hierarchy).
*
* @see GeneralizableElementFacade#getAllSpecializations()
*/
public Collection getAllSpecializations()
{
return this.getSuperClassifierFacade().getAllSpecializations();
}
/**
*
* Gets the direct generalization for this generalizable element.
*
* @see GeneralizableElementFacade#getGeneralization()
*/
public GeneralizableElementFacade getGeneralization()
{
return this.getSuperClassifierFacade().getGeneralization();
}
/**
*
* Gets the actual links that this generalization element is part
* of (it plays either the specialization or generalization).
*
* @see GeneralizableElementFacade#getGeneralizationLinks()
*/
public Collection getGeneralizationLinks()
{
return this.getSuperClassifierFacade().getGeneralizationLinks();
}
/**
*
* A comma separated list of the fully qualified names of all
* generalizations.
*
* @see GeneralizableElementFacade#getGeneralizationList()
*/
public String getGeneralizationList()
{
return this.getSuperClassifierFacade().getGeneralizationList();
}
/**
*
* The element found when you recursively follow the generalization
* path up to the root. If an element has no generalization itself
* will be considered the root.
*
* @see GeneralizableElementFacade#getGeneralizationRoot()
*/
public GeneralizableElementFacade getGeneralizationRoot()
{
return this.getSuperClassifierFacade().getGeneralizationRoot();
}
/**
*
* @see GeneralizableElementFacade#getGeneralizations()
*/
public Collection getGeneralizations()
{
return this.getSuperClassifierFacade().getGeneralizations();
}
/**
*
* Gets the direct specializations (i.e. sub elements) for this
* generalizatble element.
*
* @see GeneralizableElementFacade#getSpecializations()
*/
public Collection getSpecializations()
{
return this.getSuperClassifierFacade().getSpecializations();
}
/**
*
* Copies all tagged values from the given ModelElementFacade to
* this model element facade.
*
* @see ModelElementFacade#copyTaggedValues(ModelElementFacade element)
*/
public void copyTaggedValues(ModelElementFacade element)
{
this.getSuperClassifierFacade().copyTaggedValues(element);
}
/**
*
* Finds the tagged value with the specified 'tagName'. In case
* there are more values the first one found will be returned.
*
* @see ModelElementFacade#findTaggedValue(String tagName)
*/
public Object findTaggedValue(String tagName)
{
return this.getSuperClassifierFacade().findTaggedValue(tagName);
}
/**
*
* Returns all the values for the tagged value with the specified
* name. The returned collection will contains only String
* instances, or will be empty. Never null.
*
* @see ModelElementFacade#findTaggedValues(String tagName)
*/
public Collection findTaggedValues(String tagName)
{
return this.getSuperClassifierFacade().findTaggedValues(tagName);
}
/**
*
* Returns the fully qualified name of the model element. The fully
* qualified name includes complete package qualified name of the
* underlying model element. The templates parameter will be
* replaced by the correct one given the binding relation of the
* parameter to this element.
*
* @see ModelElementFacade#getBindedFullyQualifiedName(ModelElementFacade bindedElement)
*/
public String getBindedFullyQualifiedName(ModelElementFacade bindedElement)
{
return this.getSuperClassifierFacade().getBindedFullyQualifiedName(bindedElement);
}
/**
*
* Gets all constraints belonging to the model element.
*
* @see ModelElementFacade#getConstraints()
*/
public Collection getConstraints()
{
return this.getSuperClassifierFacade().getConstraints();
}
/**
*
* Returns the constraints of the argument kind that have been
* placed onto this model. Typical kinds are "inv", "pre" and
* "post". Other kinds are possible.
*
* @see ModelElementFacade#getConstraints(String kind)
*/
public Collection getConstraints(String kind)
{
return this.getSuperClassifierFacade().getConstraints(kind);
}
/**
*
* Gets the documentation for the model element, The indent
* argument is prefixed to each line. By default this method wraps
* lines after 64 characters.
*
*
* This method is equivalent to getDocumentation(indent,
* 64)
.
*
* @see ModelElementFacade#getDocumentation(String indent)
*/
public String getDocumentation(String indent)
{
return this.getSuperClassifierFacade().getDocumentation(indent);
}
/**
*
* This method returns the documentation for this model element,
* with the lines wrapped after the specified number of characters,
* values of less than 1 will indicate no line wrapping is
* required. By default paragraphs are returned as HTML.
*
*
* This method is equivalent to getDocumentation(indent,
* lineLength, true)
.
*
* @see ModelElementFacade#getDocumentation(String indent, int lineLength)
*/
public String getDocumentation(String indent, int lineLength)
{
return this.getSuperClassifierFacade().getDocumentation(indent, lineLength);
}
/**
*
* @see ModelElementFacade#getDocumentation(String indent, int lineLength, boolean htmlStyle)
*/
public String getDocumentation(String indent, int lineLength, boolean htmlStyle)
{
return this.getSuperClassifierFacade().getDocumentation(indent, lineLength, htmlStyle);
}
/**
*
* The fully qualified name of this model element.
*
* @see ModelElementFacade#getFullyQualifiedName()
*/
public String getFullyQualifiedName()
{
return this.getSuperClassifierFacade().getFullyQualifiedName();
}
/**
*
* Returns the fully qualified name of the model element. The fully
* qualified name includes complete package qualified name of the
* underlying model element. If modelName is true, then the
* original name of the model element (the name contained within
* the model) will be the name returned, otherwise a name from a
* language mapping will be returned.
*
* @see ModelElementFacade#getFullyQualifiedName(boolean modelName)
*/
public String getFullyQualifiedName(boolean modelName)
{
return this.getSuperClassifierFacade().getFullyQualifiedName(modelName);
}
/**
*
* Returns the fully qualified name as a path, the returned value
* always starts with out a slash '/'.
*
* @see ModelElementFacade#getFullyQualifiedNamePath()
*/
public String getFullyQualifiedNamePath()
{
return this.getSuperClassifierFacade().getFullyQualifiedNamePath();
}
/**
*
* Gets the unique identifier of the underlying model element.
*
* @see ModelElementFacade#getId()
*/
public String getId()
{
return this.getSuperClassifierFacade().getId();
}
/**
*
* UML2: Retrieves the keywords for this element. Used to modify
* implementation properties which are not represented by other
* properties, i.e. native, transient, volatile, synchronized,
* (added annotations) override, deprecated. Can also be used to
* suppress compiler warnings: (added annotations) unchecked,
* fallthrough, path, serial, finally, all. Annotations require
* JDK5 compiler level.
*
* @see ModelElementFacade#getKeywords()
*/
public Collection getKeywords()
{
return this.getSuperClassifierFacade().getKeywords();
}
/**
*
* UML2: Retrieves a localized label for this named element.
*
* @see ModelElementFacade#getLabel()
*/
public String getLabel()
{
return this.getSuperClassifierFacade().getLabel();
}
/**
*
* The language mappings that have been set for this model elemnt.
*
* @see ModelElementFacade#getLanguageMappings()
*/
public TypeMappings getLanguageMappings()
{
return this.getSuperClassifierFacade().getLanguageMappings();
}
/**
*
* @see ModelElementFacade#getModel()
*/
public ModelFacade getModel()
{
return this.getSuperClassifierFacade().getModel();
}
/**
*
* The name of the model element.
*
* @see ModelElementFacade#getName()
*/
public String getName()
{
return this.getSuperClassifierFacade().getName();
}
/**
*
* Gets the package to which this model element belongs.
*
* @see ModelElementFacade#getPackage()
*/
public ModelElementFacade getPackage()
{
return this.getSuperClassifierFacade().getPackage();
}
/**
*
* The name of this model element's package.
*
* @see ModelElementFacade#getPackageName()
*/
public String getPackageName()
{
return this.getSuperClassifierFacade().getPackageName();
}
/**
*
* Gets the package name (optionally providing the ability to
* retrieve the model name and not the mapped name).
*
* @see ModelElementFacade#getPackageName(boolean modelName)
*/
public String getPackageName(boolean modelName)
{
return this.getSuperClassifierFacade().getPackageName(modelName);
}
/**
*
* Returns the package as a path, the returned value always starts
* with out a slash '/'.
*
* @see ModelElementFacade#getPackagePath()
*/
public String getPackagePath()
{
return this.getSuperClassifierFacade().getPackagePath();
}
/**
*
* UML2: Returns the value of the 'Qualified Name' attribute. A
* name which allows the NamedElement to be identified within a
* hierarchy of nested Namespaces. It is constructed from the names
* of the containing namespaces starting at the root of the
* hierarchy and ending with the name of the NamedElement itself.
*
* @see ModelElementFacade#getQualifiedName()
*/
public String getQualifiedName()
{
return this.getSuperClassifierFacade().getQualifiedName();
}
/**
*
* Gets the root package for the model element.
*
* @see ModelElementFacade#getRootPackage()
*/
public PackageFacade getRootPackage()
{
return this.getSuperClassifierFacade().getRootPackage();
}
/**
*
* Gets the dependencies for which this model element is the
* source.
*
* @see ModelElementFacade#getSourceDependencies()
*/
public Collection getSourceDependencies()
{
return this.getSuperClassifierFacade().getSourceDependencies();
}
/**
*
* If this model element is the context of an activity graph, this
* represents that activity graph.
*
* @see ModelElementFacade#getStateMachineContext()
*/
public StateMachineFacade getStateMachineContext()
{
return this.getSuperClassifierFacade().getStateMachineContext();
}
/**
*
* The collection of ALL stereotype names for this model element.
*
* @see ModelElementFacade#getStereotypeNames()
*/
public Collection getStereotypeNames()
{
return this.getSuperClassifierFacade().getStereotypeNames();
}
/**
*
* Gets all stereotypes for this model element.
*
* @see ModelElementFacade#getStereotypes()
*/
public Collection getStereotypes()
{
return this.getSuperClassifierFacade().getStereotypes();
}
/**
*
* @see ModelElementFacade#getTaggedValues()
*/
public Collection getTaggedValues()
{
return this.getSuperClassifierFacade().getTaggedValues();
}
/**
*
* Gets the dependencies for which this model element is the
* target.
*
* @see ModelElementFacade#getTargetDependencies()
*/
public Collection getTargetDependencies()
{
return this.getSuperClassifierFacade().getTargetDependencies();
}
/**
*
* @see ModelElementFacade#getTemplateParameter(String parameterName)
*/
public Object getTemplateParameter(String parameterName)
{
return this.getSuperClassifierFacade().getTemplateParameter(parameterName);
}
/**
*
* @see ModelElementFacade#getTemplateParameters()
*/
public Collection getTemplateParameters()
{
return this.getSuperClassifierFacade().getTemplateParameters();
}
/**
*
* The visibility (i.e. public, private, protected or package) of
* the model element, will attempt a lookup for these values in the
* language mappings (if any).
*
* @see ModelElementFacade#getVisibility()
*/
public String getVisibility()
{
return this.getSuperClassifierFacade().getVisibility();
}
/**
*
* Returns true if the model element has the exact stereotype
* (meaning no stereotype inheritance is taken into account when
* searching for the stereotype), false otherwise.
*
* @see ModelElementFacade#hasExactStereotype(String stereotypeName)
*/
public boolean hasExactStereotype(String stereotypeName)
{
return this.getSuperClassifierFacade().hasExactStereotype(stereotypeName);
}
/**
*
* Does the UML Element contain the named Keyword? Keywords can be
* separated by space, comma, pipe, semicolon, or << >>
*
* @see ModelElementFacade#hasKeyword(String keywordName)
*/
public boolean hasKeyword(String keywordName)
{
return this.getSuperClassifierFacade().hasKeyword(keywordName);
}
/**
*
* Returns true if the model element has the specified stereotype.
* If the stereotype itself does not match, then a search will be
* made up the stereotype inheritance hierarchy, and if one of the
* stereotype's ancestors has a matching name this method will
* return true, false otherwise.
*
*
* For example, if we have a certain stereotype called
* <> and a model element has a stereotype called
* <> which extends <>, when
* calling this method with 'stereotypeName' defined as 'exception'
* the method would return true since <>
* inherits from <>. If you want to check if the model
* element has the exact stereotype, then use the method
* 'hasExactStereotype' instead.
*
* @see ModelElementFacade#hasStereotype(String stereotypeName)
*/
public boolean hasStereotype(String stereotypeName)
{
return this.getSuperClassifierFacade().hasStereotype(stereotypeName);
}
/**
*
* @see ModelElementFacade#isBindingDependenciesPresent()
*/
public boolean isBindingDependenciesPresent()
{
return this.getSuperClassifierFacade().isBindingDependenciesPresent();
}
/**
*
* Indicates if any constraints are present on this model element.
*
* @see ModelElementFacade#isConstraintsPresent()
*/
public boolean isConstraintsPresent()
{
return this.getSuperClassifierFacade().isConstraintsPresent();
}
/**
*
* Indicates if any documentation is present on this model element.
*
* @see ModelElementFacade#isDocumentationPresent()
*/
public boolean isDocumentationPresent()
{
return this.getSuperClassifierFacade().isDocumentationPresent();
}
/**
*
* True if this element name is a reserved word in Java, C#, ANSI
* or ISO C, C++, JavaScript.
*
* @see ModelElementFacade#isReservedWord()
*/
public boolean isReservedWord()
{
return this.getSuperClassifierFacade().isReservedWord();
}
/**
*
* @see ModelElementFacade#isTemplateParametersPresent()
*/
public boolean isTemplateParametersPresent()
{
return this.getSuperClassifierFacade().isTemplateParametersPresent();
}
/**
*
* Searches for the constraint with the specified 'name' on this
* model element, and if found translates it using the specified
* 'translation' from a translation library discovered by the
* framework.
*
* @see ModelElementFacade#translateConstraint(String name, String translation)
*/
public String translateConstraint(String name, String translation)
{
return this.getSuperClassifierFacade().translateConstraint(name, translation);
}
/**
*
* Translates all constraints belonging to this model element with
* the given 'translation'.
*
* @see ModelElementFacade#translateConstraints(String translation)
*/
public String[] translateConstraints(String translation)
{
return this.getSuperClassifierFacade().translateConstraints(translation);
}
/**
*
* Translates the constraints of the specified 'kind' belonging to
* this model element.
*
* @see ModelElementFacade#translateConstraints(String kind, String translation)
*/
public String[] translateConstraints(String kind, String translation)
{
return this.getSuperClassifierFacade().translateConstraints(kind, translation);
}
// ----------- delegates to AssociationFacade ------------
/**
*
* The first association end.
*
* @see AssociationFacade#getAssociationEndA()
*/
public AssociationEndFacade getAssociationEndA()
{
return this.getSuperAssociationFacade().getAssociationEndA();
}
/**
*
* The second association end.
*
* @see AssociationFacade#getAssociationEndB()
*/
public AssociationEndFacade getAssociationEndB()
{
return this.getSuperAssociationFacade().getAssociationEndB();
}
/**
*
* A name suited for naming this relationship. This name will be
* constructed from both association ends.
*
* @see AssociationFacade#getRelationName()
*/
public String getRelationName()
{
return this.getSuperAssociationFacade().getRelationName();
}
/**
*
* UML2: Determines whether this association is a binary
* association, i.e. whether it has exactly two member ends. UML2
* allows association classes in the association itself (many2many
* with association attributes). Default=true: only two member
* ends.
*
* @see AssociationFacade#isBinary()
*/
public boolean isBinary()
{
return this.getSuperAssociationFacade().isBinary();
}
/**
*
* UML2: Returns the value of the 'Is Derived' attribute. The
* default value is "false". If isDerived is true, the value of the
* attribute is derived from information elsewhere. Specifies
* whether the Property is derived, i.e., whether its value or
* values can be computed from other information.
*
* @see AssociationFacade#isDerived()
*/
public boolean isDerived()
{
return this.getSuperAssociationFacade().isDerived();
}
/**
*
* Indicates whether or not this associations represents a
* many-to-many relation.
*
* @see AssociationFacade#isMany2Many()
*/
public boolean isMany2Many()
{
return this.getSuperAssociationFacade().isMany2Many();
}
/**
* @see org.andromda.core.metafacade.MetafacadeBase#initialize()
*/
@Override
public void initialize()
{
this.getSuperClassifierFacade().initialize();
this.getSuperAssociationFacade().initialize();
}
/**
* @return Object getSuperClassifierFacade().getValidationOwner()
* @see org.andromda.core.metafacade.MetafacadeBase#getValidationOwner()
*/
@Override
public Object getValidationOwner()
{
Object owner = this.getSuperClassifierFacade().getValidationOwner();
if (owner == null)
{
owner = this.getSuperAssociationFacade().getValidationOwner();
}
return owner;
}
/**
* @return String getSuperClassifierFacade().getValidationName()
* @see org.andromda.core.metafacade.MetafacadeBase#getValidationName()
*/
@Override
public String getValidationName()
{
String name = this.getSuperClassifierFacade().getValidationName();
if (name == null)
{
name = this.getSuperAssociationFacade().getValidationName();
}
return name;
}
/**
* @param validationMessages Collection
* @see org.andromda.core.metafacade.MetafacadeBase#validateInvariants(Collection validationMessages)
*/
@Override
public void validateInvariants(Collection validationMessages)
{
this.getSuperClassifierFacade().validateInvariants(validationMessages);
this.getSuperAssociationFacade().validateInvariants(validationMessages);
}
/**
* The property that stores the name of the metafacade.
*/
private static final String NAME_PROPERTY = "name";
private static final String FQNAME_PROPERTY = "fullyQualifiedName";
/**
* @see Object#toString()
*/
@Override
public String toString()
{
final StringBuilder toString = new StringBuilder(this.getClass().getName());
toString.append("[");
try
{
toString.append(Introspector.instance().getProperty(this, FQNAME_PROPERTY));
}
catch (final Throwable tryAgain)
{
try
{
toString.append(Introspector.instance().getProperty(this, NAME_PROPERTY));
}
catch (final Throwable ignore)
{
// - just ignore when the metafacade doesn't have a name or fullyQualifiedName property
}
}
toString.append("]");
return toString.toString();
}
}