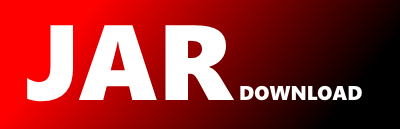
org.andromda.metafacades.uml14.AttributeFacadeLogic Maven / Gradle / Ivy
Show all versions of andromda-metafacades-uml14 Show documentation
// license-header java merge-point
//
// Attention: generated code (by MetafacadeLogic.vsl) - do not modify!
//
package org.andromda.metafacades.uml14;
import java.util.Collection;
import org.andromda.core.metafacade.MetafacadeBase;
import org.andromda.core.metafacade.ModelValidationMessage;
import org.andromda.metafacades.uml.AttributeFacade;
import org.andromda.metafacades.uml.ClassifierFacade;
import org.andromda.metafacades.uml.EnumerationFacade;
import org.andromda.translation.ocl.validation.OCLCollections;
import org.andromda.translation.ocl.validation.OCLExpressions;
import org.andromda.translation.ocl.validation.OCLIntrospector;
import org.andromda.translation.ocl.validation.OCLResultEnsurer;
import org.apache.log4j.Logger;
import org.omg.uml.foundation.core.Attribute;
/**
*
* Represents an attribute on a classifier. UML2 maps both
* Attributes and AssociationEnds to Property. A property is a
* structural feature of a classifier that characterizes instances
* of the classifier. A property related by ownedAttribute to a
* classifier (other than an association) represents an attribute
* and might also represent an association end. It relates an
* instance of the class to a value or set of values of the type of
* the attribute. A property represents a set of instances that are
* owned by a containing classifier instance. Property represents a
* declared state of one or more instances in terms of a named
* relationship to a value or values. When a property is an
* attribute of a classifier, the value or values are related to
* the instance of the classifier by being held in slots of the
* instance. The range of valid values represented by the property
* can be controlled by setting the property's type.
*
* MetafacadeLogic for AttributeFacade
*
* @see AttributeFacade
*/
public abstract class AttributeFacadeLogic
extends ModelElementFacadeLogicImpl
implements AttributeFacade
{
/**
* The underlying UML object
* @see Attribute
*/
protected Attribute metaObject;
/** Create Metafacade implementation instance using the MetafacadeFactory from the context
* @param metaObjectIn
* @param context
*/
protected AttributeFacadeLogic(Attribute metaObjectIn, String context)
{
super(metaObjectIn, getContext(context));
this.metaObject = metaObjectIn;
}
/**
* The logger instance.
*/
private static final Logger logger = Logger.getLogger(AttributeFacadeLogic.class);
/**
* Gets the context for this metafacade logic instance.
* @param context String. Set to AttributeFacade if null
* @return context String
*/
private static String getContext(String context)
{
if (context == null)
{
context = "org.andromda.metafacades.uml.AttributeFacade";
}
return context;
}
/** Reset context only for non-root metafacades
* @param context
*/
@Override
public void resetMetafacadeContext(String context)
{
if (!this.contextRoot) // reset context only for non-root metafacades
{
context = getContext(context); // to have same value as in original constructor call
setMetafacadeContext (context);
}
}
/**
* @return boolean true always
* @see AttributeFacade
*/
public boolean isAttributeFacadeMetaType()
{
return true;
}
// --------------- attributes ---------------------
/**
* @see AttributeFacade#getGetterName()
* @return String
*/
protected abstract String handleGetGetterName();
private String __getterName1a;
private boolean __getterName1aSet = false;
/**
*
* The name of the accessor operation that would retrieve this
* attribute's value.
*
* @return (String)handleGetGetterName()
*/
public final String getGetterName()
{
String getterName1a = this.__getterName1a;
if (!this.__getterName1aSet)
{
// getterName has no pre constraints
getterName1a = handleGetGetterName();
// getterName has no post constraints
this.__getterName1a = getterName1a;
if (isMetafacadePropertyCachingEnabled())
{
this.__getterName1aSet = true;
}
}
return getterName1a;
}
/**
* @see AttributeFacade#getSetterName()
* @return String
*/
protected abstract String handleGetSetterName();
private String __setterName2a;
private boolean __setterName2aSet = false;
/**
*
* The name of the mutator operation that would retrieve this
* attribute's value.
*
* @return (String)handleGetSetterName()
*/
public final String getSetterName()
{
String setterName2a = this.__setterName2a;
if (!this.__setterName2aSet)
{
// setterName has no pre constraints
setterName2a = handleGetSetterName();
// setterName has no post constraints
this.__setterName2a = setterName2a;
if (isMetafacadePropertyCachingEnabled())
{
this.__setterName2aSet = true;
}
}
return setterName2a;
}
/**
* @see AttributeFacade#isReadOnly()
* @return boolean
*/
protected abstract boolean handleIsReadOnly();
private boolean __readOnly3a;
private boolean __readOnly3aSet = false;
/**
*
* Whether or not this attribute can be modified.
*
* @return (boolean)handleIsReadOnly()
*/
public final boolean isReadOnly()
{
boolean readOnly3a = this.__readOnly3a;
if (!this.__readOnly3aSet)
{
// readOnly has no pre constraints
readOnly3a = handleIsReadOnly();
// readOnly has no post constraints
this.__readOnly3a = readOnly3a;
if (isMetafacadePropertyCachingEnabled())
{
this.__readOnly3aSet = true;
}
}
return readOnly3a;
}
/**
* @see AttributeFacade#getDefaultValue()
* @return String
*/
protected abstract String handleGetDefaultValue();
private String __defaultValue4a;
private boolean __defaultValue4aSet = false;
/**
*
* The default value of the attribute. This is the value given if
* no value is defined.
*
* @return (String)handleGetDefaultValue()
*/
public final String getDefaultValue()
{
String defaultValue4a = this.__defaultValue4a;
if (!this.__defaultValue4aSet)
{
// defaultValue has no pre constraints
defaultValue4a = handleGetDefaultValue();
// defaultValue has no post constraints
this.__defaultValue4a = defaultValue4a;
if (isMetafacadePropertyCachingEnabled())
{
this.__defaultValue4aSet = true;
}
}
return defaultValue4a;
}
/**
* @see AttributeFacade#isStatic()
* @return boolean
*/
protected abstract boolean handleIsStatic();
private boolean __static5a;
private boolean __static5aSet = false;
/**
*
* Indicates if this attribute is 'static', meaning it has a
* classifier scope.
*
* @return (boolean)handleIsStatic()
*/
public final boolean isStatic()
{
boolean static5a = this.__static5a;
if (!this.__static5aSet)
{
// static has no pre constraints
static5a = handleIsStatic();
// static has no post constraints
this.__static5a = static5a;
if (isMetafacadePropertyCachingEnabled())
{
this.__static5aSet = true;
}
}
return static5a;
}
/**
* @see AttributeFacade#isRequired()
* @return boolean
*/
protected abstract boolean handleIsRequired();
private boolean __required6a;
private boolean __required6aSet = false;
/**
*
* Whether or not the multiplicity of this attribute is 1.
*
* @return (boolean)handleIsRequired()
*/
public final boolean isRequired()
{
boolean required6a = this.__required6a;
if (!this.__required6aSet)
{
// required has no pre constraints
required6a = handleIsRequired();
// required has no post constraints
this.__required6a = required6a;
if (isMetafacadePropertyCachingEnabled())
{
this.__required6aSet = true;
}
}
return required6a;
}
/**
* @see AttributeFacade#isMany()
* @return boolean
*/
protected abstract boolean handleIsMany();
private boolean __many7a;
private boolean __many7aSet = false;
/**
*
* Whether or not this attribute has a multiplicity greater than 1.
*
* @return (boolean)handleIsMany()
*/
public final boolean isMany()
{
boolean many7a = this.__many7a;
if (!this.__many7aSet)
{
// many has no pre constraints
many7a = handleIsMany();
// many has no post constraints
this.__many7a = many7a;
if (isMetafacadePropertyCachingEnabled())
{
this.__many7aSet = true;
}
}
return many7a;
}
/**
* @see AttributeFacade#isChangeable()
* @return boolean
*/
protected abstract boolean handleIsChangeable();
private boolean __changeable8a;
private boolean __changeable8aSet = false;
/**
*
* True if this attribute can be modified.
*
* @return (boolean)handleIsChangeable()
*/
public final boolean isChangeable()
{
boolean changeable8a = this.__changeable8a;
if (!this.__changeable8aSet)
{
// changeable has no pre constraints
changeable8a = handleIsChangeable();
// changeable has no post constraints
this.__changeable8a = changeable8a;
if (isMetafacadePropertyCachingEnabled())
{
this.__changeable8aSet = true;
}
}
return changeable8a;
}
/**
* @see AttributeFacade#isAddOnly()
* @return boolean
*/
protected abstract boolean handleIsAddOnly();
private boolean __addOnly9a;
private boolean __addOnly9aSet = false;
/**
*
* True if this attribute can only be set.
*
* @return (boolean)handleIsAddOnly()
*/
public final boolean isAddOnly()
{
boolean addOnly9a = this.__addOnly9a;
if (!this.__addOnly9aSet)
{
// addOnly has no pre constraints
addOnly9a = handleIsAddOnly();
// addOnly has no post constraints
this.__addOnly9a = addOnly9a;
if (isMetafacadePropertyCachingEnabled())
{
this.__addOnly9aSet = true;
}
}
return addOnly9a;
}
/**
* @see AttributeFacade#isEnumerationLiteral()
* @return boolean
*/
protected abstract boolean handleIsEnumerationLiteral();
private boolean __enumerationLiteral10a;
private boolean __enumerationLiteral10aSet = false;
/**
*
* True if this attribute is owned by an enumeration.
*
* @return (boolean)handleIsEnumerationLiteral()
*/
public final boolean isEnumerationLiteral()
{
boolean enumerationLiteral10a = this.__enumerationLiteral10a;
if (!this.__enumerationLiteral10aSet)
{
// enumerationLiteral has no pre constraints
enumerationLiteral10a = handleIsEnumerationLiteral();
// enumerationLiteral has no post constraints
this.__enumerationLiteral10a = enumerationLiteral10a;
if (isMetafacadePropertyCachingEnabled())
{
this.__enumerationLiteral10aSet = true;
}
}
return enumerationLiteral10a;
}
/**
* @see AttributeFacade#getEnumerationValue()
* @return String
*/
protected abstract String handleGetEnumerationValue();
private String __enumerationValue11a;
private boolean __enumerationValue11aSet = false;
/**
*
* The value for this attribute if it is an enumeration literal,
* null otherwise. The default value is returned as a String if it
* has been specified, if it's not specified this attribute's name
* is assumed.
*
* @return (String)handleGetEnumerationValue()
*/
public final String getEnumerationValue()
{
String enumerationValue11a = this.__enumerationValue11a;
if (!this.__enumerationValue11aSet)
{
// enumerationValue has no pre constraints
enumerationValue11a = handleGetEnumerationValue();
// enumerationValue has no post constraints
this.__enumerationValue11a = enumerationValue11a;
if (isMetafacadePropertyCachingEnabled())
{
this.__enumerationValue11aSet = true;
}
}
return enumerationValue11a;
}
/**
* @see AttributeFacade#getGetterSetterTypeName()
* @return String
*/
protected abstract String handleGetGetterSetterTypeName();
private String __getterSetterTypeName12a;
private boolean __getterSetterTypeName12aSet = false;
/**
*
* The name of the type that is returned on the accessor and
* mutator operations, determined in part by the multiplicity.
*
* @return (String)handleGetGetterSetterTypeName()
*/
public final String getGetterSetterTypeName()
{
String getterSetterTypeName12a = this.__getterSetterTypeName12a;
if (!this.__getterSetterTypeName12aSet)
{
// getterSetterTypeName has no pre constraints
getterSetterTypeName12a = handleGetGetterSetterTypeName();
// getterSetterTypeName has no post constraints
this.__getterSetterTypeName12a = getterSetterTypeName12a;
if (isMetafacadePropertyCachingEnabled())
{
this.__getterSetterTypeName12aSet = true;
}
}
return getterSetterTypeName12a;
}
/**
* @see AttributeFacade#isOrdered()
* @return boolean
*/
protected abstract boolean handleIsOrdered();
private boolean __ordered13a;
private boolean __ordered13aSet = false;
/**
*
* Indicates whether or not the attributes are ordered (if
* multiplicity is greater than 1).
*
* @return (boolean)handleIsOrdered()
*/
public final boolean isOrdered()
{
boolean ordered13a = this.__ordered13a;
if (!this.__ordered13aSet)
{
// ordered has no pre constraints
ordered13a = handleIsOrdered();
// ordered has no post constraints
this.__ordered13a = ordered13a;
if (isMetafacadePropertyCachingEnabled())
{
this.__ordered13aSet = true;
}
}
return ordered13a;
}
/**
* @see AttributeFacade#isDefaultValuePresent()
* @return boolean
*/
protected abstract boolean handleIsDefaultValuePresent();
private boolean __defaultValuePresent14a;
private boolean __defaultValuePresent14aSet = false;
/**
*
* Indicates if the default value is present.
*
* @return (boolean)handleIsDefaultValuePresent()
*/
public final boolean isDefaultValuePresent()
{
boolean defaultValuePresent14a = this.__defaultValuePresent14a;
if (!this.__defaultValuePresent14aSet)
{
// defaultValuePresent has no pre constraints
defaultValuePresent14a = handleIsDefaultValuePresent();
// defaultValuePresent has no post constraints
this.__defaultValuePresent14a = defaultValuePresent14a;
if (isMetafacadePropertyCachingEnabled())
{
this.__defaultValuePresent14aSet = true;
}
}
return defaultValuePresent14a;
}
/**
* @see AttributeFacade#getUpper()
* @return int
*/
protected abstract int handleGetUpper();
private int __upper15a;
private boolean __upper15aSet = false;
/**
*
* the upper value for the multiplicity (will be -1 for *)
*
*
* -only applicable for UML2
*
* @return (int)handleGetUpper()
*/
public final int getUpper()
{
int upper15a = this.__upper15a;
if (!this.__upper15aSet)
{
// upper has no pre constraints
upper15a = handleGetUpper();
// upper has no post constraints
this.__upper15a = upper15a;
if (isMetafacadePropertyCachingEnabled())
{
this.__upper15aSet = true;
}
}
return upper15a;
}
/**
* @see AttributeFacade#getLower()
* @return int
*/
protected abstract int handleGetLower();
private int __lower16a;
private boolean __lower16aSet = false;
/**
*
* the lower value for the multiplicity
*
*
* -only applicable for UML2
*
* @return (int)handleGetLower()
*/
public final int getLower()
{
int lower16a = this.__lower16a;
if (!this.__lower16aSet)
{
// lower has no pre constraints
lower16a = handleGetLower();
// lower has no post constraints
this.__lower16a = lower16a;
if (isMetafacadePropertyCachingEnabled())
{
this.__lower16aSet = true;
}
}
return lower16a;
}
/**
* @see AttributeFacade#isEnumerationMember()
* @return boolean
*/
protected abstract boolean handleIsEnumerationMember();
private boolean __enumerationMember17a;
private boolean __enumerationMember17aSet = false;
/**
*
* True if this attribute is owned by an enumeration but is defined
* as a member variable (NOT a literal).
*
* @return (boolean)handleIsEnumerationMember()
*/
public final boolean isEnumerationMember()
{
boolean enumerationMember17a = this.__enumerationMember17a;
if (!this.__enumerationMember17aSet)
{
// enumerationMember has no pre constraints
enumerationMember17a = handleIsEnumerationMember();
// enumerationMember has no post constraints
this.__enumerationMember17a = enumerationMember17a;
if (isMetafacadePropertyCachingEnabled())
{
this.__enumerationMember17aSet = true;
}
}
return enumerationMember17a;
}
/**
* @see AttributeFacade#getEnumerationLiteralParameters()
* @return String
*/
protected abstract String handleGetEnumerationLiteralParameters();
private String __enumerationLiteralParameters18a;
private boolean __enumerationLiteralParameters18aSet = false;
/**
*
* Returns the enumeration literal parameters defined by tagged
* value as a comma separated list.
*
* @return (String)handleGetEnumerationLiteralParameters()
*/
public final String getEnumerationLiteralParameters()
{
String enumerationLiteralParameters18a = this.__enumerationLiteralParameters18a;
if (!this.__enumerationLiteralParameters18aSet)
{
// enumerationLiteralParameters has no pre constraints
enumerationLiteralParameters18a = handleGetEnumerationLiteralParameters();
// enumerationLiteralParameters has no post constraints
this.__enumerationLiteralParameters18a = enumerationLiteralParameters18a;
if (isMetafacadePropertyCachingEnabled())
{
this.__enumerationLiteralParameters18aSet = true;
}
}
return enumerationLiteralParameters18a;
}
/**
* @see AttributeFacade#isEnumerationLiteralParametersExist()
* @return boolean
*/
protected abstract boolean handleIsEnumerationLiteralParametersExist();
private boolean __enumerationLiteralParametersExist19a;
private boolean __enumerationLiteralParametersExist19aSet = false;
/**
*
* Returns true if enumeration literal parameters exist (defined by
* tagged value) for the literal.
*
* @return (boolean)handleIsEnumerationLiteralParametersExist()
*/
public final boolean isEnumerationLiteralParametersExist()
{
boolean enumerationLiteralParametersExist19a = this.__enumerationLiteralParametersExist19a;
if (!this.__enumerationLiteralParametersExist19aSet)
{
// enumerationLiteralParametersExist has no pre constraints
enumerationLiteralParametersExist19a = handleIsEnumerationLiteralParametersExist();
// enumerationLiteralParametersExist has no post constraints
this.__enumerationLiteralParametersExist19a = enumerationLiteralParametersExist19a;
if (isMetafacadePropertyCachingEnabled())
{
this.__enumerationLiteralParametersExist19aSet = true;
}
}
return enumerationLiteralParametersExist19a;
}
/**
* @see AttributeFacade#isUnique()
* @return boolean
*/
protected abstract boolean handleIsUnique();
private boolean __unique20a;
private boolean __unique20aSet = false;
/**
*
* If the attribute is unique within the Collection type. UML2
* only. UML14 always returns false. Unique+Ordered determines the
* implementation Collection type. Default=false.
*
* @return (boolean)handleIsUnique()
*/
public final boolean isUnique()
{
boolean unique20a = this.__unique20a;
if (!this.__unique20aSet)
{
// unique has no pre constraints
unique20a = handleIsUnique();
// unique has no post constraints
this.__unique20a = unique20a;
if (isMetafacadePropertyCachingEnabled())
{
this.__unique20aSet = true;
}
}
return unique20a;
}
/**
* @see AttributeFacade#isLeaf()
* @return boolean
*/
protected abstract boolean handleIsLeaf();
private boolean __leaf21a;
private boolean __leaf21aSet = false;
/**
*
* IsLeaf property in the operation. If true, operation is final,
* cannot be extended or implemented by a descendant.
*
* @return (boolean)handleIsLeaf()
*/
public final boolean isLeaf()
{
boolean leaf21a = this.__leaf21a;
if (!this.__leaf21aSet)
{
// leaf has no pre constraints
leaf21a = handleIsLeaf();
// leaf has no post constraints
this.__leaf21a = leaf21a;
if (isMetafacadePropertyCachingEnabled())
{
this.__leaf21aSet = true;
}
}
return leaf21a;
}
/**
* @see AttributeFacade#isDerived()
* @return boolean
*/
protected abstract boolean handleIsDerived();
private boolean __derived22a;
private boolean __derived22aSet = false;
/**
*
* If the attribute is derived (its value is computed). UML2 only.
* UML14 always returns false. Default=false.
*
* @return (boolean)handleIsDerived()
*/
public final boolean isDerived()
{
boolean derived22a = this.__derived22a;
if (!this.__derived22aSet)
{
// derived has no pre constraints
derived22a = handleIsDerived();
// derived has no post constraints
this.__derived22a = derived22a;
if (isMetafacadePropertyCachingEnabled())
{
this.__derived22aSet = true;
}
}
return derived22a;
}
// ---------------- business methods ----------------------
/**
* Method to be implemented in descendants
*
* Searches the given feature for the specified tag.
*
*
* If the follow boolean is set to true then the search will
* continue from the class attribute to the class itself and then
* up the class hierarchy.
*
* @param name
* @param follow
* @return Object
*/
protected abstract Object handleFindTaggedValue(String name, boolean follow);
/**
*
* Searches the given feature for the specified tag.
*
*
* If the follow boolean is set to true then the search will
* continue from the class attribute to the class itself and then
* up the class hierarchy.
*
* @param name String
*
* The name of the tagged value to find.
*
* @param follow boolean
*
* If true search should 'follow' the inheritance hierarchy, false
* otherwise.
*
* @return handleFindTaggedValue(name, follow)
*/
public Object findTaggedValue(String name, boolean follow)
{
// findTaggedValue has no pre constraints
Object returnValue = handleFindTaggedValue(name, follow);
// findTaggedValue has no post constraints
return returnValue;
}
// ------------- associations ------------------
/**
*
* Gets the attributes that belong to the classifier.
*
* @return (ClassifierFacade)handleGetOwner()
*/
public final ClassifierFacade getOwner()
{
ClassifierFacade getOwner1r = null;
// attributes has no pre constraints
Object result = handleGetOwner();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getOwner1r = (ClassifierFacade)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
AttributeFacadeLogic.logger.warn("incorrect metafacade cast for AttributeFacadeLogic.getOwner ClassifierFacade " + result + ": " + shieldedResult);
}
// attributes has no post constraints
return getOwner1r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetOwner();
/**
*
* @return (ClassifierFacade)handleGetType()
*/
public final ClassifierFacade getType()
{
ClassifierFacade getType2r = null;
// attributeFacade has no pre constraints
Object result = handleGetType();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getType2r = (ClassifierFacade)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
AttributeFacadeLogic.logger.warn("incorrect metafacade cast for AttributeFacadeLogic.getType ClassifierFacade " + result + ": " + shieldedResult);
}
// attributeFacade has no post constraints
return getType2r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetType();
/**
*
* This enumeration's literals.
*
* @return (EnumerationFacade)handleGetEnumeration()
*/
public final EnumerationFacade getEnumeration()
{
EnumerationFacade getEnumeration5r = null;
// literals has no pre constraints
Object result = handleGetEnumeration();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getEnumeration5r = (EnumerationFacade)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
AttributeFacadeLogic.logger.warn("incorrect metafacade cast for AttributeFacadeLogic.getEnumeration EnumerationFacade " + result + ": " + shieldedResult);
}
// literals has no post constraints
return getEnumeration5r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetEnumeration();
/**
* Constraint: org::andromda::metafacades::uml::AttributeFacade::attribute needs a type
* Error: Each attribute needs a type, you cannot leave the type unspecified.
* OCL: context AttributeFacade inv: type->notEmpty() and type.name->notEmpty()
* Constraint: org::andromda::metafacades::uml::AttributeFacade::attribute must have a name
* Error: Each attribute must have a non-empty name.
* OCL: context AttributeFacade inv: name -> notEmpty()
* Constraint: org::andromda::metafacades::uml::AttributeFacade::primitive attribute must be required
* Error: Primitive attributes must have a multiplicity lower bound > 0 (must be required). Use a wrapped type, or change the multiplicity.
* OCL: context AttributeFacade inv: type.primitive implies (lower > 0)
* Constraint: org::andromda::metafacades::uml::AttributeFacade::primitive attribute cannot be used in Collection
* Error: Primitive attributes cannot be used in Collections (multiplicity > 1). Use the wrapped type instead.
* OCL: context AttributeFacade inv: type.primitive implies (many = false)
* Constraint: org::andromda::metafacades::uml::AttributeFacade::wrapped primitive attribute should not be required
* Error: Wrapped primitive attributes must have a multiplicity lower bound = 0 (must be optional). Use the unwrapped type, or change the multiplicity.
* OCL: context AttributeFacade inv: type.wrappedPrimitive and many = false implies (lower = 0)
* @param validationMessages Collection
* @see ModelElementFacadeLogicImpl#validateInvariants(Collection validationMessages)
*/
@Override
public void validateInvariants(Collection validationMessages)
{
super.validateInvariants(validationMessages);
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure(OCLCollections.notEmpty(OCLIntrospector.invoke(contextElement,"type"))&&OCLCollections.notEmpty(OCLIntrospector.invoke(contextElement,"type.name")));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::AttributeFacade::attribute needs a type",
"Each attribute needs a type, you cannot leave the type unspecified."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::AttributeFacade::attribute needs a type' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure(OCLCollections.notEmpty(OCLIntrospector.invoke(contextElement,"name")));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::AttributeFacade::attribute must have a name",
"Each attribute must have a non-empty name."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::AttributeFacade::attribute must have a name' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure((Boolean.valueOf(String.valueOf(OCLIntrospector.invoke(contextElement,"type.primitive"))).booleanValue()?(OCLExpressions.greater(OCLIntrospector.invoke(contextElement,"lower"),0)):true));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::AttributeFacade::primitive attribute must be required",
"Primitive attributes must have a multiplicity lower bound > 0 (must be required). Use a wrapped type, or change the multiplicity."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::AttributeFacade::primitive attribute must be required' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure((Boolean.valueOf(String.valueOf(OCLIntrospector.invoke(contextElement,"type.primitive"))).booleanValue()?(OCLExpressions.equal(OCLIntrospector.invoke(contextElement,"many"),false)):true));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::AttributeFacade::primitive attribute cannot be used in Collection",
"Primitive attributes cannot be used in Collections (multiplicity > 1). Use the wrapped type instead."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::AttributeFacade::primitive attribute cannot be used in Collection' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure((Boolean.valueOf(String.valueOf(Boolean.valueOf(String.valueOf(OCLIntrospector.invoke(contextElement,"type.wrappedPrimitive"))).booleanValue()&&OCLExpressions.equal(OCLIntrospector.invoke(contextElement,"many"),false))).booleanValue()?(OCLExpressions.equal(OCLIntrospector.invoke(contextElement,"lower"),0)):true));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::AttributeFacade::wrapped primitive attribute should not be required",
"Wrapped primitive attributes must have a multiplicity lower bound = 0 (must be optional). Use the unwrapped type, or change the multiplicity."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::AttributeFacade::wrapped primitive attribute should not be required' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
}
}