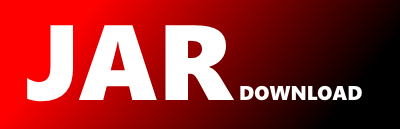
org.andromda.metafacades.uml14.ConstraintFacadeLogic Maven / Gradle / Ivy
Show all versions of andromda-metafacades-uml14 Show documentation
// license-header java merge-point
//
// Attention: generated code (by MetafacadeLogic.vsl) - do not modify!
//
package org.andromda.metafacades.uml14;
import java.util.Collection;
import org.andromda.core.metafacade.MetafacadeBase;
import org.andromda.core.metafacade.ModelValidationMessage;
import org.andromda.metafacades.uml.ConstraintFacade;
import org.andromda.metafacades.uml.ModelElementFacade;
import org.apache.log4j.Logger;
import org.omg.uml.foundation.core.Constraint;
/**
*
* Condition or restriction expressed in natural language text or
* in a machine readable language for the purpose of declaring some
* of the semantics of an element.
*
* MetafacadeLogic for ConstraintFacade
*
* @see ConstraintFacade
*/
public abstract class ConstraintFacadeLogic
extends ModelElementFacadeLogicImpl
implements ConstraintFacade
{
/**
* The underlying UML object
* @see Constraint
*/
protected Constraint metaObject;
/** Create Metafacade implementation instance using the MetafacadeFactory from the context
* @param metaObjectIn
* @param context
*/
protected ConstraintFacadeLogic(Constraint metaObjectIn, String context)
{
super(metaObjectIn, getContext(context));
this.metaObject = metaObjectIn;
}
/**
* The logger instance.
*/
private static final Logger logger = Logger.getLogger(ConstraintFacadeLogic.class);
/**
* Gets the context for this metafacade logic instance.
* @param context String. Set to ConstraintFacade if null
* @return context String
*/
private static String getContext(String context)
{
if (context == null)
{
context = "org.andromda.metafacades.uml.ConstraintFacade";
}
return context;
}
/** Reset context only for non-root metafacades
* @param context
*/
@Override
public void resetMetafacadeContext(String context)
{
if (!this.contextRoot) // reset context only for non-root metafacades
{
context = getContext(context); // to have same value as in original constructor call
setMetafacadeContext (context);
}
}
/**
* @return boolean true always
* @see ConstraintFacade
*/
public boolean isConstraintFacadeMetaType()
{
return true;
}
// --------------- attributes ---------------------
/**
* @see ConstraintFacade#getBody()
* @return String
*/
protected abstract String handleGetBody();
private String __body1a;
private boolean __body1aSet = false;
/**
*
* Gets the 'body' or text of this constraint.
*
* @return (String)handleGetBody()
*/
public final String getBody()
{
String body1a = this.__body1a;
if (!this.__body1aSet)
{
// body has no pre constraints
body1a = handleGetBody();
// body has no post constraints
this.__body1a = body1a;
if (isMetafacadePropertyCachingEnabled())
{
this.__body1aSet = true;
}
}
return body1a;
}
/**
* @see ConstraintFacade#isInvariant()
* @return boolean
*/
protected abstract boolean handleIsInvariant();
private boolean __invariant2a;
private boolean __invariant2aSet = false;
/**
*
* True if this constraint denotes an invariant.
*
*
* For example:
*
*
*
*
*
* context LivingAnimal
*
*
* inv: alive = true
*
*
*
*
*
* False otherwise.
*
* @return (boolean)handleIsInvariant()
*/
public final boolean isInvariant()
{
boolean invariant2a = this.__invariant2a;
if (!this.__invariant2aSet)
{
// invariant has no pre constraints
invariant2a = handleIsInvariant();
// invariant has no post constraints
this.__invariant2a = invariant2a;
if (isMetafacadePropertyCachingEnabled())
{
this.__invariant2aSet = true;
}
}
return invariant2a;
}
/**
* @see ConstraintFacade#isPreCondition()
* @return boolean
*/
protected abstract boolean handleIsPreCondition();
private boolean __preCondition3a;
private boolean __preCondition3aSet = false;
/**
*
* True if this constraint denotes a precondition.
*
*
* For example:
*
*
*
*
*
* context LivingAnimal::canFly()
*
*
* pre: hasWings = true
*
*
*
*
*
* False otherwise.
*
* @return (boolean)handleIsPreCondition()
*/
public final boolean isPreCondition()
{
boolean preCondition3a = this.__preCondition3a;
if (!this.__preCondition3aSet)
{
// preCondition has no pre constraints
preCondition3a = handleIsPreCondition();
// preCondition has no post constraints
this.__preCondition3a = preCondition3a;
if (isMetafacadePropertyCachingEnabled())
{
this.__preCondition3aSet = true;
}
}
return preCondition3a;
}
/**
* @see ConstraintFacade#isPostCondition()
* @return boolean
*/
protected abstract boolean handleIsPostCondition();
private boolean __postCondition4a;
private boolean __postCondition4aSet = false;
/**
*
* True if this constraint denotes a postcondition.
*
*
* For example:
*
*
*
*
*
* context LivingAnimal::getNumberOfLegs()
*
*
* post: numberOfLegs >= 0
*
*
*
*
*
* False otherwise.
*
* @return (boolean)handleIsPostCondition()
*/
public final boolean isPostCondition()
{
boolean postCondition4a = this.__postCondition4a;
if (!this.__postCondition4aSet)
{
// postCondition has no pre constraints
postCondition4a = handleIsPostCondition();
// postCondition has no post constraints
this.__postCondition4a = postCondition4a;
if (isMetafacadePropertyCachingEnabled())
{
this.__postCondition4aSet = true;
}
}
return postCondition4a;
}
/**
* @see ConstraintFacade#isDefinition()
* @return boolean
*/
protected abstract boolean handleIsDefinition();
private boolean __definition5a;
private boolean __definition5aSet = false;
/**
*
* True if this constraint denotes a definition.
*
*
* For example:
*
*
*
*
*
* context CustomerCard
*
*
* def: getTotalPoints(d: date) : Integer =
* transaction->select(date.isAfter(d)).points->sum()
*
*
*
*
*
* False otherwise.
*
* @return (boolean)handleIsDefinition()
*/
public final boolean isDefinition()
{
boolean definition5a = this.__definition5a;
if (!this.__definition5aSet)
{
// definition has no pre constraints
definition5a = handleIsDefinition();
// definition has no post constraints
this.__definition5a = definition5a;
if (isMetafacadePropertyCachingEnabled())
{
this.__definition5aSet = true;
}
}
return definition5a;
}
/**
* @see ConstraintFacade#isBodyExpression()
* @return boolean
*/
protected abstract boolean handleIsBodyExpression();
private boolean __bodyExpression6a;
private boolean __bodyExpression6aSet = false;
/**
*
* True if this constraint denotes a body expression.
*
*
* For example:
*
*
*
*
*
* context CustomerCard:getTransaction(from:Date, until:Date)
*
*
* body: transactions->select(date.isAfter(from) and
* date.isBefore(until))
*
*
*
*
*
* False otherwise.
*
* @return (boolean)handleIsBodyExpression()
*/
public final boolean isBodyExpression()
{
boolean bodyExpression6a = this.__bodyExpression6a;
if (!this.__bodyExpression6aSet)
{
// bodyExpression has no pre constraints
bodyExpression6a = handleIsBodyExpression();
// bodyExpression has no post constraints
this.__bodyExpression6a = bodyExpression6a;
if (isMetafacadePropertyCachingEnabled())
{
this.__bodyExpression6aSet = true;
}
}
return bodyExpression6a;
}
// ---------------- business methods ----------------------
/**
* Method to be implemented in descendants
*
* This constraint's translation for the argument languange.
*
* @param language
* @return String
*/
protected abstract String handleGetTranslation(String language);
/**
*
* This constraint's translation for the argument languange.
*
* @param language String
*
* The target language to which the OCL constraint is to be
* translated.
*
* @return handleGetTranslation(language)
*/
public String getTranslation(String language)
{
// getTranslation has no pre constraints
String returnValue = handleGetTranslation(language);
// getTranslation has no post constraints
return returnValue;
}
// ------------- associations ------------------
/**
*
* Gets all constraints belonging to the model element.
*
* @return (ModelElementFacade)handleGetContextElement()
*/
public final ModelElementFacade getContextElement()
{
ModelElementFacade getContextElement1r = null;
// constraints has no pre constraints
Object result = handleGetContextElement();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getContextElement1r = (ModelElementFacade)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
ConstraintFacadeLogic.logger.warn("incorrect metafacade cast for ConstraintFacadeLogic.getContextElement ModelElementFacade " + result + ": " + shieldedResult);
}
// constraints has no post constraints
return getContextElement1r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetContextElement();
/**
* @param validationMessages Collection
* @see ModelElementFacadeLogicImpl#validateInvariants(Collection validationMessages)
*/
@Override
public void validateInvariants(Collection validationMessages)
{
super.validateInvariants(validationMessages);
}
}