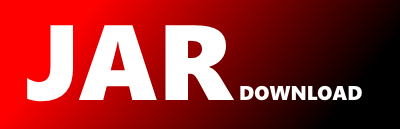
org.andromda.metafacades.uml14.EnumerationFacadeLogic Maven / Gradle / Ivy
// license-header java merge-point
//
// Attention: generated code (by MetafacadeLogic.vsl) - do not modify!
//
package org.andromda.metafacades.uml14;
import java.util.Collection;
import java.util.List;
import org.andromda.core.metafacade.MetafacadeBase;
import org.andromda.core.metafacade.ModelValidationMessage;
import org.andromda.metafacades.uml.AttributeFacade;
import org.andromda.metafacades.uml.ClassifierFacade;
import org.andromda.metafacades.uml.EnumerationFacade;
import org.andromda.translation.ocl.validation.OCLCollections;
import org.andromda.translation.ocl.validation.OCLIntrospector;
import org.andromda.translation.ocl.validation.OCLResultEnsurer;
import org.apache.log4j.Logger;
import org.omg.uml.foundation.core.Classifier;
/**
*
* Represents an enumeration. A data type whose values are
* enumerated in the model as enumeration literals. May also be a
* Classifier with Stereotype Enumeration.
*
* MetafacadeLogic for EnumerationFacade
*
* @see EnumerationFacade
*/
public abstract class EnumerationFacadeLogic
extends ClassifierFacadeLogicImpl
implements EnumerationFacade
{
/**
* The underlying UML object
* @see Object
*/
protected Object metaObject;
/** Create Metafacade implementation instance using the MetafacadeFactory from the context
* @param metaObjectIn
* @param context
*/
protected EnumerationFacadeLogic(Object metaObjectIn, String context)
{
super((Classifier)metaObjectIn, getContext(context));
this.metaObject = metaObjectIn;
}
/**
* The logger instance.
*/
private static final Logger logger = Logger.getLogger(EnumerationFacadeLogic.class);
/**
* Gets the context for this metafacade logic instance.
* @param context String. Set to EnumerationFacade if null
* @return context String
*/
private static String getContext(String context)
{
if (context == null)
{
context = "org.andromda.metafacades.uml.EnumerationFacade";
}
return context;
}
/** Reset context only for non-root metafacades
* @param context
*/
@Override
public void resetMetafacadeContext(String context)
{
if (!this.contextRoot) // reset context only for non-root metafacades
{
context = getContext(context); // to have same value as in original constructor call
setMetafacadeContext (context);
}
}
/**
* @return boolean true always
* @see EnumerationFacade
*/
public boolean isEnumerationFacadeMetaType()
{
return true;
}
// --------------- attributes ---------------------
/**
* @see EnumerationFacade#getFromOperationSignature()
* @return String
*/
protected abstract String handleGetFromOperationSignature();
private String __fromOperationSignature1a;
private boolean __fromOperationSignature1aSet = false;
/**
*
* The 'from' operation signature. This is the signature that
* takes the actual literal value and allows a new enumeration to
* be constructed.
*
* @return (String)handleGetFromOperationSignature()
*/
public final String getFromOperationSignature()
{
String fromOperationSignature1a = this.__fromOperationSignature1a;
if (!this.__fromOperationSignature1aSet)
{
// fromOperationSignature has no pre constraints
fromOperationSignature1a = handleGetFromOperationSignature();
// fromOperationSignature has no post constraints
this.__fromOperationSignature1a = fromOperationSignature1a;
if (isMetafacadePropertyCachingEnabled())
{
this.__fromOperationSignature1aSet = true;
}
}
return fromOperationSignature1a;
}
/**
* @see EnumerationFacade#getFromOperationName()
* @return String
*/
protected abstract String handleGetFromOperationName();
private String __fromOperationName2a;
private boolean __fromOperationName2aSet = false;
/**
*
* The 'from' operation name. This is the name of the operation
* that takes the actual literal value and allows a new enumeration
* to be constructed.
*
* @return (String)handleGetFromOperationName()
*/
public final String getFromOperationName()
{
String fromOperationName2a = this.__fromOperationName2a;
if (!this.__fromOperationName2aSet)
{
// fromOperationName has no pre constraints
fromOperationName2a = handleGetFromOperationName();
// fromOperationName has no post constraints
this.__fromOperationName2a = fromOperationName2a;
if (isMetafacadePropertyCachingEnabled())
{
this.__fromOperationName2aSet = true;
}
}
return fromOperationName2a;
}
/**
* @see EnumerationFacade#isTypeSafe()
* @return boolean
*/
protected abstract boolean handleIsTypeSafe();
private boolean __typeSafe3a;
private boolean __typeSafe3aSet = false;
/**
*
* Indicates whether the enumeration must be generated using a Java
* 5 type-safe enum or a traditional enumeration-pattern class.
*
* @return (boolean)handleIsTypeSafe()
*/
public final boolean isTypeSafe()
{
boolean typeSafe3a = this.__typeSafe3a;
if (!this.__typeSafe3aSet)
{
// typeSafe has no pre constraints
typeSafe3a = handleIsTypeSafe();
// typeSafe has no post constraints
this.__typeSafe3a = typeSafe3a;
if (isMetafacadePropertyCachingEnabled())
{
this.__typeSafe3aSet = true;
}
}
return typeSafe3a;
}
// ------------- associations ------------------
/**
*
* If the attribute is an enumeration literal this represents the
* owning enumeration. Can be empty.
*
* @return (Collection)handleGetLiterals()
*/
public final Collection getLiterals()
{
Collection getLiterals1r = null;
// enumeration has no pre constraints
Collection result = handleGetLiterals();
List shieldedResult = this.shieldedElements(result);
try
{
getLiterals1r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
EnumerationFacadeLogic.logger.warn("incorrect metafacade cast for EnumerationFacadeLogic.getLiterals Collection " + result + ": " + shieldedResult);
}
// enumeration has no post constraints
return getLiterals1r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetLiterals();
/**
*
* @return (ClassifierFacade)handleGetLiteralType()
*/
public final ClassifierFacade getLiteralType()
{
ClassifierFacade getLiteralType2r = null;
// enumerationFacade has no pre constraints
Object result = handleGetLiteralType();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getLiteralType2r = (ClassifierFacade)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
EnumerationFacadeLogic.logger.warn("incorrect metafacade cast for EnumerationFacadeLogic.getLiteralType ClassifierFacade " + result + ": " + shieldedResult);
}
// enumerationFacade has no post constraints
return getLiteralType2r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetLiteralType();
/**
*
* @return (Collection)handleGetMemberVariables()
*/
public final Collection getMemberVariables()
{
Collection getMemberVariables3r = null;
// enumeration has no pre constraints
Collection result = handleGetMemberVariables();
List shieldedResult = this.shieldedElements(result);
try
{
getMemberVariables3r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
EnumerationFacadeLogic.logger.warn("incorrect metafacade cast for EnumerationFacadeLogic.getMemberVariables Collection " + result + ": " + shieldedResult);
}
// enumeration has no post constraints
return getMemberVariables3r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetMemberVariables();
/**
* Constraint: org::andromda::metafacades::uml::EnumerationFacade::enumerations must have at least one attribute
* Error: An enumeration must have at least one literal modeled.
* OCL: context EnumerationFacade
inv: literals -> notEmpty()
* @param validationMessages Collection
* @see ClassifierFacadeLogicImpl#validateInvariants(Collection validationMessages)
*/
@Override
public void validateInvariants(Collection validationMessages)
{
super.validateInvariants(validationMessages);
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure(OCLCollections.notEmpty(OCLIntrospector.invoke(contextElement,"literals")));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::EnumerationFacade::enumerations must have at least one attribute",
"An enumeration must have at least one literal modeled."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::EnumerationFacade::enumerations must have at least one attribute' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
}
}