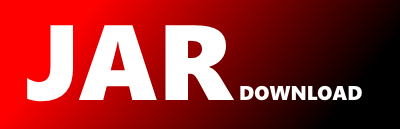
org.andromda.metafacades.uml14.FrontEndActionStateLogic Maven / Gradle / Ivy
Show all versions of andromda-metafacades-uml14 Show documentation
// license-header java merge-point
//
// Attention: generated code (by MetafacadeLogic.vsl) - do not modify!
//
package org.andromda.metafacades.uml14;
import java.util.Collection;
import java.util.List;
import org.andromda.core.metafacade.MetafacadeBase;
import org.andromda.core.metafacade.ModelValidationMessage;
import org.andromda.metafacades.uml.FrontEndAction;
import org.andromda.metafacades.uml.FrontEndActionState;
import org.andromda.metafacades.uml.FrontEndExceptionHandler;
import org.andromda.metafacades.uml.FrontEndForward;
import org.andromda.metafacades.uml.OperationFacade;
import org.andromda.translation.ocl.validation.OCLCollections;
import org.andromda.translation.ocl.validation.OCLExpressions;
import org.andromda.translation.ocl.validation.OCLIntrospector;
import org.andromda.translation.ocl.validation.OCLResultEnsurer;
import org.apache.log4j.Logger;
import org.omg.uml.behavioralelements.activitygraphs.ActionState;
/**
*
* Represents an operation on the server called by an action.
* Optionally may defer operations to the controller.
*
* MetafacadeLogic for FrontEndActionState
*
* @see FrontEndActionState
*/
public abstract class FrontEndActionStateLogic
extends ActionStateFacadeLogicImpl
implements FrontEndActionState
{
/**
* The underlying UML object
* @see Object
*/
protected Object metaObject;
/** Create Metafacade implementation instance using the MetafacadeFactory from the context
* @param metaObjectIn
* @param context
*/
protected FrontEndActionStateLogic(Object metaObjectIn, String context)
{
super((ActionState)metaObjectIn, getContext(context));
this.metaObject = metaObjectIn;
}
/**
* The logger instance.
*/
private static final Logger logger = Logger.getLogger(FrontEndActionStateLogic.class);
/**
* Gets the context for this metafacade logic instance.
* @param context String. Set to FrontEndActionState if null
* @return context String
*/
private static String getContext(String context)
{
if (context == null)
{
context = "org.andromda.metafacades.uml.FrontEndActionState";
}
return context;
}
/** Reset context only for non-root metafacades
* @param context
*/
@Override
public void resetMetafacadeContext(String context)
{
if (!this.contextRoot) // reset context only for non-root metafacades
{
context = getContext(context); // to have same value as in original constructor call
setMetafacadeContext (context);
}
}
/**
* @return boolean true always
* @see FrontEndActionState
*/
public boolean isFrontEndActionStateMetaType()
{
return true;
}
// --------------- attributes ---------------------
/**
* @see FrontEndActionState#isServerSide()
* @return boolean
*/
protected abstract boolean handleIsServerSide();
private boolean __serverSide1a;
private boolean __serverSide1aSet = false;
/**
*
* Indicates whether or not this front end action state is server
* side. Pages, for example, are also action states but they return
* control to the client.
*
* @return (boolean)handleIsServerSide()
*/
public final boolean isServerSide()
{
boolean serverSide1a = this.__serverSide1a;
if (!this.__serverSide1aSet)
{
// serverSide has no pre constraints
serverSide1a = handleIsServerSide();
// serverSide has no post constraints
this.__serverSide1a = serverSide1a;
if (isMetafacadePropertyCachingEnabled())
{
this.__serverSide1aSet = true;
}
}
return serverSide1a;
}
/**
* @see FrontEndActionState#isContainedInFrontEndUseCase()
* @return boolean
*/
protected abstract boolean handleIsContainedInFrontEndUseCase();
private boolean __containedInFrontEndUseCase2a;
private boolean __containedInFrontEndUseCase2aSet = false;
/**
*
* True if this element is contained in a FrontEndUseCase.
*
* @return (boolean)handleIsContainedInFrontEndUseCase()
*/
public final boolean isContainedInFrontEndUseCase()
{
boolean containedInFrontEndUseCase2a = this.__containedInFrontEndUseCase2a;
if (!this.__containedInFrontEndUseCase2aSet)
{
// containedInFrontEndUseCase has no pre constraints
containedInFrontEndUseCase2a = handleIsContainedInFrontEndUseCase();
// containedInFrontEndUseCase has no post constraints
this.__containedInFrontEndUseCase2a = containedInFrontEndUseCase2a;
if (isMetafacadePropertyCachingEnabled())
{
this.__containedInFrontEndUseCase2aSet = true;
}
}
return containedInFrontEndUseCase2a;
}
/**
* @see FrontEndActionState#getActionMethodName()
* @return String
*/
protected abstract String handleGetActionMethodName();
private String __actionMethodName3a;
private boolean __actionMethodName3aSet = false;
/**
*
* The method name representing this action state.
*
* @return (String)handleGetActionMethodName()
*/
public final String getActionMethodName()
{
String actionMethodName3a = this.__actionMethodName3a;
if (!this.__actionMethodName3aSet)
{
// actionMethodName has no pre constraints
actionMethodName3a = handleGetActionMethodName();
// actionMethodName has no post constraints
this.__actionMethodName3a = actionMethodName3a;
if (isMetafacadePropertyCachingEnabled())
{
this.__actionMethodName3aSet = true;
}
}
return actionMethodName3a;
}
// ------------- associations ------------------
private FrontEndForward __getForward1r;
private boolean __getForward1rSet = false;
/**
*
* @return (FrontEndForward)handleGetForward()
*/
public final FrontEndForward getForward()
{
FrontEndForward getForward1r = this.__getForward1r;
if (!this.__getForward1rSet)
{
// frontEndActionState has no pre constraints
Object result = handleGetForward();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getForward1r = (FrontEndForward)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndActionStateLogic.logger.warn("incorrect metafacade cast for FrontEndActionStateLogic.getForward FrontEndForward " + result + ": " + shieldedResult);
}
// frontEndActionState has no post constraints
this.__getForward1r = getForward1r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getForward1rSet = true;
}
}
return getForward1r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetForward();
private List __getControllerCalls2r;
private boolean __getControllerCalls2rSet = false;
/**
*
* @return (List)handleGetControllerCalls()
*/
public final List getControllerCalls()
{
List getControllerCalls2r = this.__getControllerCalls2r;
if (!this.__getControllerCalls2rSet)
{
// frontEndActionState has no pre constraints
List result = handleGetControllerCalls();
List shieldedResult = this.shieldedElements(result);
try
{
getControllerCalls2r = (List)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndActionStateLogic.logger.warn("incorrect metafacade cast for FrontEndActionStateLogic.getControllerCalls List " + result + ": " + shieldedResult);
}
// frontEndActionState has no post constraints
this.__getControllerCalls2r = getControllerCalls2r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getControllerCalls2rSet = true;
}
}
return getControllerCalls2r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return List
*/
protected abstract List handleGetControllerCalls();
private List __getExceptions3r;
private boolean __getExceptions3rSet = false;
/**
*
* @return (List)handleGetExceptions()
*/
public final List getExceptions()
{
List getExceptions3r = this.__getExceptions3r;
if (!this.__getExceptions3rSet)
{
// frontEndActionState has no pre constraints
List result = handleGetExceptions();
List shieldedResult = this.shieldedElements(result);
try
{
getExceptions3r = (List)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndActionStateLogic.logger.warn("incorrect metafacade cast for FrontEndActionStateLogic.getExceptions List " + result + ": " + shieldedResult);
}
// frontEndActionState has no post constraints
this.__getExceptions3r = getExceptions3r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getExceptions3rSet = true;
}
}
return getExceptions3r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return List
*/
protected abstract List handleGetExceptions();
private List __getContainerActions4r;
private boolean __getContainerActions4rSet = false;
/**
*
* All action states visited by this action.
*
* @return (List)handleGetContainerActions()
*/
public final List getContainerActions()
{
List getContainerActions4r = this.__getContainerActions4r;
if (!this.__getContainerActions4rSet)
{
// actionStates has no pre constraints
List result = handleGetContainerActions();
List shieldedResult = this.shieldedElements(result);
try
{
getContainerActions4r = (List)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndActionStateLogic.logger.warn("incorrect metafacade cast for FrontEndActionStateLogic.getContainerActions List " + result + ": " + shieldedResult);
}
// actionStates has no post constraints
this.__getContainerActions4r = getContainerActions4r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getContainerActions4rSet = true;
}
}
return getContainerActions4r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return List
*/
protected abstract List handleGetContainerActions();
private List __getServiceCalls5r;
private boolean __getServiceCalls5rSet = false;
/**
*
* @return (List)handleGetServiceCalls()
*/
public final List getServiceCalls()
{
List getServiceCalls5r = this.__getServiceCalls5r;
if (!this.__getServiceCalls5rSet)
{
// frontEndActionState has no pre constraints
List result = handleGetServiceCalls();
List shieldedResult = this.shieldedElements(result);
try
{
getServiceCalls5r = (List)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndActionStateLogic.logger.warn("incorrect metafacade cast for FrontEndActionStateLogic.getServiceCalls List " + result + ": " + shieldedResult);
}
// frontEndActionState has no post constraints
this.__getServiceCalls5r = getServiceCalls5r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getServiceCalls5rSet = true;
}
}
return getServiceCalls5r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return List
*/
protected abstract List handleGetServiceCalls();
/**
* Constraint: org::andromda::metafacades::uml::FrontEndActionState::each front-end action state must have a name
* Error: A "front-end" action state must have a non-empty name.
* OCL: context FrontEndActionState inv: name->notEmpty()
* Constraint: org::andromda::metafacades::uml::FrontEndActionState::front-end action states can have at most one outgoing transition
* Error: A "front-end" action state needs at most one outgoing transition that is not an exception-transition.
* OCL: context FrontEndActionState inv: serverSide implies (exceptions->size() + 1 = outgoings->size() or exceptions->size() = outgoings->size())
* @param validationMessages Collection
* @see ActionStateFacadeLogicImpl#validateInvariants(Collection validationMessages)
*/
@Override
public void validateInvariants(Collection validationMessages)
{
super.validateInvariants(validationMessages);
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure(OCLCollections.notEmpty(OCLIntrospector.invoke(contextElement,"name")));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::FrontEndActionState::each front-end action state must have a name",
"A \"front-end\" action state must have a non-empty name."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::FrontEndActionState::each front-end action state must have a name' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure((Boolean.valueOf(String.valueOf(OCLIntrospector.invoke(contextElement,"serverSide"))).booleanValue()?(OCLExpressions.equal(OCLCollections.size(OCLIntrospector.invoke(contextElement,"exceptions"))+1,OCLCollections.size(OCLIntrospector.invoke(contextElement,"outgoings")))||OCLExpressions.equal(OCLCollections.size(OCLIntrospector.invoke(contextElement,"exceptions")),OCLCollections.size(OCLIntrospector.invoke(contextElement,"outgoings")))):true));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::FrontEndActionState::front-end action states can have at most one outgoing transition",
"A \"front-end\" action state needs at most one outgoing transition that is not an exception-transition."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::FrontEndActionState::front-end action states can have at most one outgoing transition' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
}
}