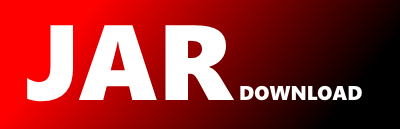
org.andromda.metafacades.uml14.FrontEndControllerLogic Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of andromda-metafacades-uml14 Show documentation
Show all versions of andromda-metafacades-uml14 Show documentation
The UML 1.4 metafacades. This is the set of UML 1.4 metafacades
implementations. These implement the common UML metafacades.
The newest version!
// license-header java merge-point
//
// Attention: generated code (by MetafacadeLogic.vsl) - do not modify!
//
package org.andromda.metafacades.uml14;
import java.util.Collection;
import java.util.List;
import org.andromda.core.metafacade.MetafacadeBase;
import org.andromda.core.metafacade.ModelValidationMessage;
import org.andromda.metafacades.uml.DependencyFacade;
import org.andromda.metafacades.uml.FrontEndAction;
import org.andromda.metafacades.uml.FrontEndController;
import org.andromda.metafacades.uml.FrontEndUseCase;
import org.andromda.translation.ocl.validation.OCLCollections;
import org.andromda.translation.ocl.validation.OCLIntrospector;
import org.andromda.translation.ocl.validation.OCLResultEnsurer;
import org.apache.log4j.Logger;
import org.omg.uml.foundation.core.Classifier;
/**
*
* A front end controller is assigned as the context of a use-case.
* The controller provides the "controlling" of the use case's
* activity.
*
* MetafacadeLogic for FrontEndController
*
* @see FrontEndController
*/
public abstract class FrontEndControllerLogic
extends ClassifierFacadeLogicImpl
implements FrontEndController
{
/**
* The underlying UML object
* @see Object
*/
protected Object metaObject;
/** Create Metafacade implementation instance using the MetafacadeFactory from the context
* @param metaObjectIn
* @param context
*/
protected FrontEndControllerLogic(Object metaObjectIn, String context)
{
super((Classifier)metaObjectIn, getContext(context));
this.metaObject = metaObjectIn;
}
/**
* The logger instance.
*/
private static final Logger logger = Logger.getLogger(FrontEndControllerLogic.class);
/**
* Gets the context for this metafacade logic instance.
* @param context String. Set to FrontEndController if null
* @return context String
*/
private static String getContext(String context)
{
if (context == null)
{
context = "org.andromda.metafacades.uml.FrontEndController";
}
return context;
}
/** Reset context only for non-root metafacades
* @param context
*/
@Override
public void resetMetafacadeContext(String context)
{
if (!this.contextRoot) // reset context only for non-root metafacades
{
context = getContext(context); // to have same value as in original constructor call
setMetafacadeContext (context);
}
}
/**
* @return boolean true always
* @see FrontEndController
*/
public boolean isFrontEndControllerMetaType()
{
return true;
}
// ------------- associations ------------------
private List __getServiceReferences1r;
private boolean __getServiceReferences1rSet = false;
/**
*
* @return (List)handleGetServiceReferences()
*/
public final List getServiceReferences()
{
List getServiceReferences1r = this.__getServiceReferences1r;
if (!this.__getServiceReferences1rSet)
{
// frontEndController has no pre constraints
List result = handleGetServiceReferences();
List shieldedResult = this.shieldedElements(result);
try
{
getServiceReferences1r = (List)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndControllerLogic.logger.warn("incorrect metafacade cast for FrontEndControllerLogic.getServiceReferences List " + result + ": " + shieldedResult);
}
// frontEndController has no post constraints
this.__getServiceReferences1r = getServiceReferences1r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getServiceReferences1rSet = true;
}
}
return getServiceReferences1r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return List
*/
protected abstract List handleGetServiceReferences();
private FrontEndUseCase __getUseCase2r;
private boolean __getUseCase2rSet = false;
/**
*
* Returns the controller for this use-case.
*
* @return (FrontEndUseCase)handleGetUseCase()
*/
public final FrontEndUseCase getUseCase()
{
FrontEndUseCase getUseCase2r = this.__getUseCase2r;
if (!this.__getUseCase2rSet)
{
// controller has no pre constraints
Object result = handleGetUseCase();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getUseCase2r = (FrontEndUseCase)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndControllerLogic.logger.warn("incorrect metafacade cast for FrontEndControllerLogic.getUseCase FrontEndUseCase " + result + ": " + shieldedResult);
}
// controller has no post constraints
this.__getUseCase2r = getUseCase2r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getUseCase2rSet = true;
}
}
return getUseCase2r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetUseCase();
private List __getDeferringActions5r;
private boolean __getDeferringActions5rSet = false;
/**
*
* The controller that will handle the execution of this front-end
* action. This controller is set as the context of the activity
* graph (and therefore also of the use-case).
*
* @return (List)handleGetDeferringActions()
*/
public final List getDeferringActions()
{
List getDeferringActions5r = this.__getDeferringActions5r;
if (!this.__getDeferringActions5rSet)
{
// controller has no pre constraints
List result = handleGetDeferringActions();
List shieldedResult = this.shieldedElements(result);
try
{
getDeferringActions5r = (List)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndControllerLogic.logger.warn("incorrect metafacade cast for FrontEndControllerLogic.getDeferringActions List " + result + ": " + shieldedResult);
}
// controller has no post constraints
this.__getDeferringActions5r = getDeferringActions5r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getDeferringActions5rSet = true;
}
}
return getDeferringActions5r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return List
*/
protected abstract List handleGetDeferringActions();
/**
* Constraint: org::andromda::metafacades::uml::FrontEndController::front-end controllers need a package
* Error: Each front-end controller is required to be modeled in a package, doing otherwise will result in uncompileable code due to filename collisions.
* OCL: context FrontEndController inv: packageName->notEmpty()
* @param validationMessages Collection
* @see ClassifierFacadeLogicImpl#validateInvariants(Collection validationMessages)
*/
@Override
public void validateInvariants(Collection validationMessages)
{
super.validateInvariants(validationMessages);
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure(OCLCollections.notEmpty(OCLIntrospector.invoke(contextElement,"packageName")));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::FrontEndController::front-end controllers need a package",
"Each front-end controller is required to be modeled in a package, doing otherwise will result in uncompileable code due to filename collisions."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::FrontEndController::front-end controllers need a package' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy