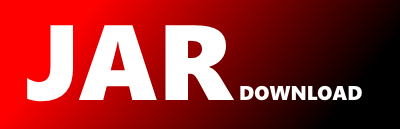
org.andromda.metafacades.uml14.FrontEndForwardLogic Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of andromda-metafacades-uml14 Show documentation
Show all versions of andromda-metafacades-uml14 Show documentation
The UML 1.4 metafacades. This is the set of UML 1.4 metafacades
implementations. These implement the common UML metafacades.
The newest version!
// license-header java merge-point
//
// Attention: generated code (by MetafacadeLogic.vsl) - do not modify!
//
package org.andromda.metafacades.uml14;
import java.util.Collection;
import java.util.List;
import org.andromda.core.metafacade.MetafacadeBase;
import org.andromda.core.metafacade.ModelValidationMessage;
import org.andromda.metafacades.uml.FrontEndAction;
import org.andromda.metafacades.uml.FrontEndActivityGraph;
import org.andromda.metafacades.uml.FrontEndControllerOperation;
import org.andromda.metafacades.uml.FrontEndEvent;
import org.andromda.metafacades.uml.FrontEndForward;
import org.andromda.metafacades.uml.FrontEndParameter;
import org.andromda.metafacades.uml.FrontEndUseCase;
import org.apache.log4j.Logger;
import org.omg.uml.behavioralelements.statemachines.Transition;
/**
*
* A front end forward is any transition between front-end states.
*
* MetafacadeLogic for FrontEndForward
*
* @see FrontEndForward
*/
public abstract class FrontEndForwardLogic
extends TransitionFacadeLogicImpl
implements FrontEndForward
{
/**
* The underlying UML object
* @see Object
*/
protected Object metaObject;
/** Create Metafacade implementation instance using the MetafacadeFactory from the context
* @param metaObjectIn
* @param context
*/
protected FrontEndForwardLogic(Object metaObjectIn, String context)
{
super((Transition)metaObjectIn, getContext(context));
this.metaObject = metaObjectIn;
}
/**
* The logger instance.
*/
private static final Logger logger = Logger.getLogger(FrontEndForwardLogic.class);
/**
* Gets the context for this metafacade logic instance.
* @param context String. Set to FrontEndForward if null
* @return context String
*/
private static String getContext(String context)
{
if (context == null)
{
context = "org.andromda.metafacades.uml.FrontEndForward";
}
return context;
}
/** Reset context only for non-root metafacades
* @param context
*/
@Override
public void resetMetafacadeContext(String context)
{
if (!this.contextRoot) // reset context only for non-root metafacades
{
context = getContext(context); // to have same value as in original constructor call
setMetafacadeContext (context);
}
}
/**
* @return boolean true always
* @see FrontEndForward
*/
public boolean isFrontEndForwardMetaType()
{
return true;
}
// --------------- attributes ---------------------
/**
* @see FrontEndForward#isContainedInFrontEndUseCase()
* @return boolean
*/
protected abstract boolean handleIsContainedInFrontEndUseCase();
private boolean __containedInFrontEndUseCase1a;
private boolean __containedInFrontEndUseCase1aSet = false;
/**
*
* Indicates if this forward is contained in a FrontEndUseCase.
*
* @return (boolean)handleIsContainedInFrontEndUseCase()
*/
public final boolean isContainedInFrontEndUseCase()
{
boolean containedInFrontEndUseCase1a = this.__containedInFrontEndUseCase1a;
if (!this.__containedInFrontEndUseCase1aSet)
{
// containedInFrontEndUseCase has no pre constraints
containedInFrontEndUseCase1a = handleIsContainedInFrontEndUseCase();
// containedInFrontEndUseCase has no post constraints
this.__containedInFrontEndUseCase1a = containedInFrontEndUseCase1a;
if (isMetafacadePropertyCachingEnabled())
{
this.__containedInFrontEndUseCase1aSet = true;
}
}
return containedInFrontEndUseCase1a;
}
/**
* @see FrontEndForward#getActionMethodName()
* @return String
*/
protected abstract String handleGetActionMethodName();
private String __actionMethodName2a;
private boolean __actionMethodName2aSet = false;
/**
*
* The method name used to delegate to this forward.
*
* @return (String)handleGetActionMethodName()
*/
public final String getActionMethodName()
{
String actionMethodName2a = this.__actionMethodName2a;
if (!this.__actionMethodName2aSet)
{
// actionMethodName has no pre constraints
actionMethodName2a = handleGetActionMethodName();
// actionMethodName has no post constraints
this.__actionMethodName2a = actionMethodName2a;
if (isMetafacadePropertyCachingEnabled())
{
this.__actionMethodName2aSet = true;
}
}
return actionMethodName2a;
}
/**
* @see FrontEndForward#isEnteringView()
* @return boolean
*/
protected abstract boolean handleIsEnteringView();
private boolean __enteringView3a;
private boolean __enteringView3aSet = false;
/**
*
* Indicates if this action directly targets a "front-end" view,
* false otherwise.
*
* @return (boolean)handleIsEnteringView()
*/
public final boolean isEnteringView()
{
boolean enteringView3a = this.__enteringView3a;
if (!this.__enteringView3aSet)
{
// enteringView has no pre constraints
enteringView3a = handleIsEnteringView();
// enteringView has no post constraints
this.__enteringView3a = enteringView3a;
if (isMetafacadePropertyCachingEnabled())
{
this.__enteringView3aSet = true;
}
}
return enteringView3a;
}
/**
* @see FrontEndForward#isExitingView()
* @return boolean
*/
protected abstract boolean handleIsExitingView();
private boolean __exitingView4a;
private boolean __exitingView4aSet = false;
/**
*
* Indicates if this forward (transition) is coming out of a
* front-end view.
*
* @return (boolean)handleIsExitingView()
*/
public final boolean isExitingView()
{
boolean exitingView4a = this.__exitingView4a;
if (!this.__exitingView4aSet)
{
// exitingView has no pre constraints
exitingView4a = handleIsExitingView();
// exitingView has no post constraints
this.__exitingView4a = exitingView4a;
if (isMetafacadePropertyCachingEnabled())
{
this.__exitingView4aSet = true;
}
}
return exitingView4a;
}
// ------------- associations ------------------
private FrontEndActivityGraph __getFrontEndActivityGraph2r;
private boolean __getFrontEndActivityGraph2rSet = false;
/**
*
* @return (FrontEndActivityGraph)handleGetFrontEndActivityGraph()
*/
public final FrontEndActivityGraph getFrontEndActivityGraph()
{
FrontEndActivityGraph getFrontEndActivityGraph2r = this.__getFrontEndActivityGraph2r;
if (!this.__getFrontEndActivityGraph2rSet)
{
// frontEndForward has no pre constraints
Object result = handleGetFrontEndActivityGraph();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getFrontEndActivityGraph2r = (FrontEndActivityGraph)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndForwardLogic.logger.warn("incorrect metafacade cast for FrontEndForwardLogic.getFrontEndActivityGraph FrontEndActivityGraph " + result + ": " + shieldedResult);
}
// frontEndForward has no post constraints
this.__getFrontEndActivityGraph2r = getFrontEndActivityGraph2r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getFrontEndActivityGraph2rSet = true;
}
}
return getFrontEndActivityGraph2r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetFrontEndActivityGraph();
private FrontEndUseCase __getUseCase3r;
private boolean __getUseCase3rSet = false;
/**
*
* @return (FrontEndUseCase)handleGetUseCase()
*/
public final FrontEndUseCase getUseCase()
{
FrontEndUseCase getUseCase3r = this.__getUseCase3r;
if (!this.__getUseCase3rSet)
{
// frontEndForward has no pre constraints
Object result = handleGetUseCase();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getUseCase3r = (FrontEndUseCase)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndForwardLogic.logger.warn("incorrect metafacade cast for FrontEndForwardLogic.getUseCase FrontEndUseCase " + result + ": " + shieldedResult);
}
// frontEndForward has no post constraints
this.__getUseCase3r = getUseCase3r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getUseCase3rSet = true;
}
}
return getUseCase3r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetUseCase();
private FrontEndEvent __getDecisionTrigger5r;
private boolean __getDecisionTrigger5rSet = false;
/**
*
* @return (FrontEndEvent)handleGetDecisionTrigger()
*/
public final FrontEndEvent getDecisionTrigger()
{
FrontEndEvent getDecisionTrigger5r = this.__getDecisionTrigger5r;
if (!this.__getDecisionTrigger5rSet)
{
// frontEndForward has no pre constraints
Object result = handleGetDecisionTrigger();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getDecisionTrigger5r = (FrontEndEvent)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndForwardLogic.logger.warn("incorrect metafacade cast for FrontEndForwardLogic.getDecisionTrigger FrontEndEvent " + result + ": " + shieldedResult);
}
// frontEndForward has no post constraints
this.__getDecisionTrigger5r = getDecisionTrigger5r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getDecisionTrigger5rSet = true;
}
}
return getDecisionTrigger5r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetDecisionTrigger();
private List __getActions6r;
private boolean __getActions6rSet = false;
/**
*
* All the transitions that make up this action, this directly maps
* onto the forwards.
*
* @return (List)handleGetActions()
*/
public final List getActions()
{
List getActions6r = this.__getActions6r;
if (!this.__getActions6rSet)
{
// transitions has no pre constraints
List result = handleGetActions();
List shieldedResult = this.shieldedElements(result);
try
{
getActions6r = (List)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndForwardLogic.logger.warn("incorrect metafacade cast for FrontEndForwardLogic.getActions List " + result + ": " + shieldedResult);
}
// transitions has no post constraints
this.__getActions6r = getActions6r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getActions6rSet = true;
}
}
return getActions6r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return List
*/
protected abstract List handleGetActions();
private List __getForwardParameters7r;
private boolean __getForwardParameters7rSet = false;
/**
*
* @return (List)handleGetForwardParameters()
*/
public final List getForwardParameters()
{
List getForwardParameters7r = this.__getForwardParameters7r;
if (!this.__getForwardParameters7rSet)
{
// frontEndForward has no pre constraints
List result = handleGetForwardParameters();
List shieldedResult = this.shieldedElements(result);
try
{
getForwardParameters7r = (List)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndForwardLogic.logger.warn("incorrect metafacade cast for FrontEndForwardLogic.getForwardParameters List " + result + ": " + shieldedResult);
}
// frontEndForward has no post constraints
this.__getForwardParameters7r = getForwardParameters7r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getForwardParameters7rSet = true;
}
}
return getForwardParameters7r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return List
*/
protected abstract List handleGetForwardParameters();
private FrontEndControllerOperation __getOperationCall9r;
private boolean __getOperationCall9rSet = false;
/**
*
* @return (FrontEndControllerOperation)handleGetOperationCall()
*/
public final FrontEndControllerOperation getOperationCall()
{
FrontEndControllerOperation getOperationCall9r = this.__getOperationCall9r;
if (!this.__getOperationCall9rSet)
{
// frontEndForward has no pre constraints
Object result = handleGetOperationCall();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getOperationCall9r = (FrontEndControllerOperation)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
FrontEndForwardLogic.logger.warn("incorrect metafacade cast for FrontEndForwardLogic.getOperationCall FrontEndControllerOperation " + result + ": " + shieldedResult);
}
// frontEndForward has no post constraints
this.__getOperationCall9r = getOperationCall9r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getOperationCall9rSet = true;
}
}
return getOperationCall9r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetOperationCall();
/**
* @param validationMessages Collection
* @see TransitionFacadeLogicImpl#validateInvariants(Collection validationMessages)
*/
@Override
public void validateInvariants(Collection validationMessages)
{
super.validateInvariants(validationMessages);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy