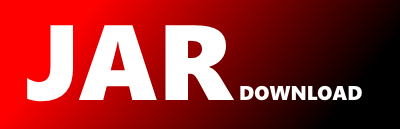
org.andromda.metafacades.uml14.GeneralizableElementFacadeLogic Maven / Gradle / Ivy
// license-header java merge-point
//
// Attention: generated code (by MetafacadeLogic.vsl) - do not modify!
//
package org.andromda.metafacades.uml14;
import java.util.Collection;
import java.util.List;
import org.andromda.core.metafacade.MetafacadeBase;
import org.andromda.core.metafacade.ModelValidationMessage;
import org.andromda.metafacades.uml.GeneralizableElementFacade;
import org.andromda.metafacades.uml.GeneralizationFacade;
import org.apache.log4j.Logger;
import org.omg.uml.foundation.core.GeneralizableElement;
/**
*
* The model element that represents an element that can be
* generalized or specialized.
*
* MetafacadeLogic for GeneralizableElementFacade
*
* @see GeneralizableElementFacade
*/
public abstract class GeneralizableElementFacadeLogic
extends ModelElementFacadeLogicImpl
implements GeneralizableElementFacade
{
/**
* The underlying UML object
* @see GeneralizableElement
*/
protected GeneralizableElement metaObject;
/** Create Metafacade implementation instance using the MetafacadeFactory from the context
* @param metaObjectIn
* @param context
*/
protected GeneralizableElementFacadeLogic(GeneralizableElement metaObjectIn, String context)
{
super(metaObjectIn, getContext(context));
this.metaObject = metaObjectIn;
}
/**
* The logger instance.
*/
private static final Logger logger = Logger.getLogger(GeneralizableElementFacadeLogic.class);
/**
* Gets the context for this metafacade logic instance.
* @param context String. Set to GeneralizableElementFacade if null
* @return context String
*/
private static String getContext(String context)
{
if (context == null)
{
context = "org.andromda.metafacades.uml.GeneralizableElementFacade";
}
return context;
}
/** Reset context only for non-root metafacades
* @param context
*/
@Override
public void resetMetafacadeContext(String context)
{
if (!this.contextRoot) // reset context only for non-root metafacades
{
context = getContext(context); // to have same value as in original constructor call
setMetafacadeContext (context);
}
}
/**
* @return boolean true always
* @see GeneralizableElementFacade
*/
public boolean isGeneralizableElementFacadeMetaType()
{
return true;
}
// --------------- attributes ---------------------
/**
* @see GeneralizableElementFacade#getGeneralizationList()
* @return String
*/
protected abstract String handleGetGeneralizationList();
private String __generalizationList1a;
private boolean __generalizationList1aSet = false;
/**
*
* A comma separated list of the fully qualified names of all
* generalizations.
*
* @return (String)handleGetGeneralizationList()
*/
public final String getGeneralizationList()
{
String generalizationList1a = this.__generalizationList1a;
if (!this.__generalizationList1aSet)
{
// generalizationList has no pre constraints
generalizationList1a = handleGetGeneralizationList();
// generalizationList has no post constraints
this.__generalizationList1a = generalizationList1a;
if (isMetafacadePropertyCachingEnabled())
{
this.__generalizationList1aSet = true;
}
}
return generalizationList1a;
}
// ---------------- business methods ----------------------
/**
* Method to be implemented in descendants
*
* Finds the tagged value optional searching the entire inheritance
* hierarchy if 'follow' is set to true.
*
* @param tagName
* @param follow
* @return Object
*/
protected abstract Object handleFindTaggedValue(String tagName, boolean follow);
/**
*
* Finds the tagged value optional searching the entire inheritance
* hierarchy if 'follow' is set to true.
*
* @param tagName String
*
* The name of the tag of the tagged value to find.
*
* @param follow boolean
*
* Whether or not to 'follow' the inheritance hierarchy when
* searching for the tagged value.
*
* @return handleFindTaggedValue(tagName, follow)
*/
public Object findTaggedValue(String tagName, boolean follow)
{
// findTaggedValue has no pre constraints
Object returnValue = handleFindTaggedValue(tagName, follow);
// findTaggedValue has no post constraints
return returnValue;
}
// ------------- associations ------------------
/**
*
* @return (GeneralizableElementFacade)handleGetGeneralization()
*/
public final GeneralizableElementFacade getGeneralization()
{
GeneralizableElementFacade getGeneralization1r = null;
// generalizableElementFacade has no pre constraints
Object result = handleGetGeneralization();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getGeneralization1r = (GeneralizableElementFacade)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
GeneralizableElementFacadeLogic.logger.warn("incorrect metafacade cast for GeneralizableElementFacadeLogic.getGeneralization GeneralizableElementFacade " + result + ": " + shieldedResult);
}
// generalizableElementFacade has no post constraints
return getGeneralization1r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetGeneralization();
private Collection __getSpecializations6r;
private boolean __getSpecializations6rSet = false;
/**
*
* @return (Collection)handleGetSpecializations()
*/
public final Collection getSpecializations()
{
Collection getSpecializations6r = this.__getSpecializations6r;
if (!this.__getSpecializations6rSet)
{
// generalizableElementFacade has no pre constraints
Collection result = handleGetSpecializations();
List shieldedResult = this.shieldedElements(result);
try
{
getSpecializations6r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
GeneralizableElementFacadeLogic.logger.warn("incorrect metafacade cast for GeneralizableElementFacadeLogic.getSpecializations Collection " + result + ": " + shieldedResult);
}
// generalizableElementFacade has no post constraints
this.__getSpecializations6r = getSpecializations6r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getSpecializations6rSet = true;
}
}
return getSpecializations6r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetSpecializations();
/**
*
* @return (Collection)handleGetGeneralizations()
*/
public final Collection getGeneralizations()
{
Collection getGeneralizations7r = null;
// generalizableElementFacade has no pre constraints
Collection result = handleGetGeneralizations();
List shieldedResult = this.shieldedElements(result);
try
{
getGeneralizations7r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
GeneralizableElementFacadeLogic.logger.warn("incorrect metafacade cast for GeneralizableElementFacadeLogic.getGeneralizations Collection " + result + ": " + shieldedResult);
}
// generalizableElementFacade has no post constraints
return getGeneralizations7r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetGeneralizations();
/**
*
* @return (Collection)handleGetGeneralizationLinks()
*/
public final Collection getGeneralizationLinks()
{
Collection getGeneralizationLinks9r = null;
// generalizableElementFacade has no pre constraints
Collection result = handleGetGeneralizationLinks();
List shieldedResult = this.shieldedElements(result);
try
{
getGeneralizationLinks9r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
GeneralizableElementFacadeLogic.logger.warn("incorrect metafacade cast for GeneralizableElementFacadeLogic.getGeneralizationLinks Collection " + result + ": " + shieldedResult);
}
// generalizableElementFacade has no post constraints
return getGeneralizationLinks9r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetGeneralizationLinks();
/**
*
* @return (Collection)handleGetAllSpecializations()
*/
public final Collection getAllSpecializations()
{
Collection getAllSpecializations10r = null;
// generalizableElementFacade has no pre constraints
Collection result = handleGetAllSpecializations();
List shieldedResult = this.shieldedElements(result);
try
{
getAllSpecializations10r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
GeneralizableElementFacadeLogic.logger.warn("incorrect metafacade cast for GeneralizableElementFacadeLogic.getAllSpecializations Collection " + result + ": " + shieldedResult);
}
// generalizableElementFacade has no post constraints
return getAllSpecializations10r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetAllSpecializations();
/**
*
* @return (Collection)handleGetAllGeneralizations()
*/
public final Collection getAllGeneralizations()
{
Collection getAllGeneralizations12r = null;
// generalizableElementFacade has no pre constraints
Collection result = handleGetAllGeneralizations();
List shieldedResult = this.shieldedElements(result);
try
{
getAllGeneralizations12r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
GeneralizableElementFacadeLogic.logger.warn("incorrect metafacade cast for GeneralizableElementFacadeLogic.getAllGeneralizations Collection " + result + ": " + shieldedResult);
}
// generalizableElementFacade has no post constraints
return getAllGeneralizations12r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetAllGeneralizations();
private GeneralizableElementFacade __getGeneralizationRoot14r;
private boolean __getGeneralizationRoot14rSet = false;
/**
*
* @return (GeneralizableElementFacade)handleGetGeneralizationRoot()
*/
public final GeneralizableElementFacade getGeneralizationRoot()
{
GeneralizableElementFacade getGeneralizationRoot14r = this.__getGeneralizationRoot14r;
if (!this.__getGeneralizationRoot14rSet)
{
// generalizableElementFacade has no pre constraints
Object result = handleGetGeneralizationRoot();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getGeneralizationRoot14r = (GeneralizableElementFacade)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
GeneralizableElementFacadeLogic.logger.warn("incorrect metafacade cast for GeneralizableElementFacadeLogic.getGeneralizationRoot GeneralizableElementFacade " + result + ": " + shieldedResult);
}
// generalizableElementFacade has no post constraints
this.__getGeneralizationRoot14r = getGeneralizationRoot14r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getGeneralizationRoot14rSet = true;
}
}
return getGeneralizationRoot14r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetGeneralizationRoot();
/**
* @param validationMessages Collection
* @see ModelElementFacadeLogicImpl#validateInvariants(Collection validationMessages)
*/
@Override
public void validateInvariants(Collection validationMessages)
{
super.validateInvariants(validationMessages);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy