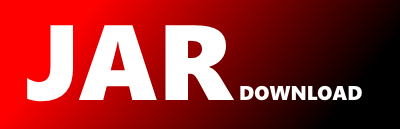
org.andromda.metafacades.uml14.GeneralizableElementFacadeLogicImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of andromda-metafacades-uml14 Show documentation
Show all versions of andromda-metafacades-uml14 Show documentation
The UML 1.4 metafacades. This is the set of UML 1.4 metafacades
implementations. These implement the common UML metafacades.
The newest version!
package org.andromda.metafacades.uml14;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
import java.util.LinkedHashSet;
import java.util.Set;
import org.andromda.metafacades.uml.GeneralizableElementFacade;
import org.andromda.metafacades.uml.GeneralizationFacade;
import org.andromda.metafacades.uml.ModelElementFacade;
import org.apache.commons.collections.CollectionUtils;
import org.apache.commons.collections.Transformer;
import org.omg.uml.foundation.core.GeneralizableElement;
import org.omg.uml.foundation.core.Generalization;
/**
* MetafacadeLogic implementation.
*
* @see org.andromda.metafacades.uml.GeneralizableElementFacade
* @author Bob Fields
*/
public class GeneralizableElementFacadeLogicImpl
extends GeneralizableElementFacadeLogic
{
private static final long serialVersionUID = 34L;
/**
* @param metaObject
* @param context
*/
public GeneralizableElementFacadeLogicImpl(GeneralizableElement metaObject,
String context)
{
super(metaObject, context);
}
/**
* @see org.andromda.metafacades.uml.GeneralizableElementFacade#getAllGeneralizations()
*/
@Override
public Collection handleGetAllGeneralizations()
{
final Collection generalizations = new ArrayList();
for (final Iterator iterator = this.getGeneralizations().iterator(); iterator.hasNext();)
{
final GeneralizableElementFacade element = iterator.next();
generalizations.add(element);
generalizations.addAll(element.getAllGeneralizations());
}
return generalizations;
}
// ------------- relations ------------------
/**
* @see org.andromda.metafacades.uml.GeneralizableElementFacade#getGeneralization()
*/
@Override
public GeneralizableElement handleGetGeneralization()
{
GeneralizableElement parent = null;
Collection generalizations = metaObject.getGeneralization();
if (generalizations != null)
{
Iterator iterator = generalizations.iterator();
if (iterator.hasNext())
{
parent = iterator.next().getParent();
}
}
return parent;
}
/**
* @see org.andromda.metafacades.uml.GeneralizableElementFacade#getGeneralizations()
*/
@Override
protected Collection handleGetGeneralizations()
{
Collection parents = new LinkedHashSet();
Collection generalizations = metaObject.getGeneralization();
if (generalizations != null && !generalizations.isEmpty())
{
for (Generalization generalization : generalizations)
{
parents.add(generalization.getParent());
}
}
return this.shieldedElements(parents);
}
/**
* @see org.andromda.metafacades.uml.GeneralizableElementFacade#getGeneralizationLinks()
*/
@Override
protected Collection handleGetGeneralizationLinks()
{
return this.shieldedElements(metaObject.getGeneralization());
}
/**
* @see org.andromda.metafacades.uml.ClassifierFacade#getSpecializations()
*/
@Override
public Collection handleGetSpecializations()
{
Collection specializations = new ArrayList(UML14MetafacadeUtils.getCorePackage().getAParentSpecialization()
.getSpecialization(this.metaObject));
CollectionUtils.transform(specializations, new Transformer()
{
public Object transform(Object object)
{
return ((Generalization)object).getChild();
}
});
return specializations;
}
/**
* @see org.andromda.metafacades.uml.GeneralizableElementFacade#getGeneralizationList()
*/
@Override
protected String handleGetGeneralizationList()
{
final StringBuilder list = new StringBuilder();
if (this.getGeneralizations() != null)
{
for (final Iterator iterator = this.getGeneralizations().iterator(); iterator.hasNext();)
{
list.append(((ModelElementFacade)iterator.next()).getFullyQualifiedName());
if (iterator.hasNext())
{
list.append(", ");
}
}
}
return list.toString();
}
/**
* @see org.andromda.metafacades.uml.GeneralizableElementFacade#getAllSpecializations()
*/
@Override
protected Collection handleGetAllSpecializations()
{
final Set allSpecializations = new LinkedHashSet();
if (this.getSpecializations() != null)
{
allSpecializations.addAll(this.getSpecializations());
for (final Iterator iterator = this.getSpecializations().iterator(); iterator.hasNext();)
{
final GeneralizableElementFacade element = (GeneralizableElementFacade)iterator.next();
allSpecializations.addAll(element.getAllSpecializations());
}
}
return allSpecializations;
}
/**
* @see org.andromda.metafacades.uml.GeneralizableElementFacade#findTaggedValue(String, boolean)
*/
@Override
protected Object handleFindTaggedValue(final String tagName, boolean follow)
{
Object value = this.findTaggedValue(tagName);
if (value == null)
{
for (GeneralizableElementFacade element = this.getGeneralization();
value == null && element != null; element = element.getGeneralization())
{
value = element.findTaggedValue(tagName, follow);
}
}
return value;
}
/**
* @see org.andromda.metafacades.uml14.GeneralizableElementFacadeLogic#handleGetGeneralizationRoot()
*/
protected GeneralizableElementFacade handleGetGeneralizationRoot()
{
return this.getGeneralization() == null
? (GeneralizableElementFacade)THIS()
: this.getGeneralization().getGeneralizationRoot();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy