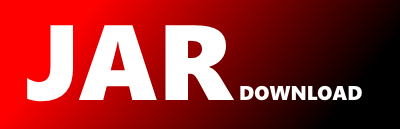
org.andromda.metafacades.uml14.ModelElementFacadeLogic Maven / Gradle / Ivy
Show all versions of andromda-metafacades-uml14 Show documentation
// license-header java merge-point
//
// Attention: generated code (by MetafacadeLogic.vsl) - do not modify!
//
package org.andromda.metafacades.uml14;
import java.util.Collection;
import java.util.List;
import org.andromda.core.common.Introspector;
import org.andromda.core.metafacade.MetafacadeBase;
import org.andromda.core.metafacade.ModelValidationMessage;
import org.andromda.metafacades.uml.ConstraintFacade;
import org.andromda.metafacades.uml.DependencyFacade;
import org.andromda.metafacades.uml.ModelElementFacade;
import org.andromda.metafacades.uml.ModelFacade;
import org.andromda.metafacades.uml.PackageFacade;
import org.andromda.metafacades.uml.StateMachineFacade;
import org.andromda.metafacades.uml.StereotypeFacade;
import org.andromda.metafacades.uml.TaggedValueFacade;
import org.andromda.metafacades.uml.TemplateParameterFacade;
import org.andromda.metafacades.uml.TypeMappings;
import org.apache.log4j.Logger;
import org.omg.uml.foundation.core.ModelElement;
/**
*
* Represents a model element. It may be an Element or
* NamedElement. A named element is an element in a model that may
* have a name. An element is a constituent of a model. As such, it
* has the capability of owning other elements.
*
* MetafacadeLogic for ModelElementFacade
*
* @see ModelElementFacade
*/
public abstract class ModelElementFacadeLogic
extends MetafacadeBase
implements ModelElementFacade
{
/**
* The underlying UML object
* @see ModelElement
*/
protected ModelElement metaObject;
/** Create Metafacade implementation instance using the MetafacadeFactory from the context
* @param metaObjectIn
* @param context
*/
protected ModelElementFacadeLogic(ModelElement metaObjectIn, String context)
{
super(metaObjectIn, getContext(context));
this.metaObject = metaObjectIn;
}
/**
* The logger instance.
*/
private static final Logger logger = Logger.getLogger(ModelElementFacadeLogic.class);
/**
* Gets the context for this metafacade logic instance.
* @param context String. Set to ModelElementFacade if null
* @return context String
*/
private static String getContext(String context)
{
if (context == null)
{
context = "org.andromda.metafacades.uml.ModelElementFacade";
}
return context;
}
/** Reset context only for non-root metafacades
* @param context
*/
@Override
public void resetMetafacadeContext(String context)
{
if (!this.contextRoot) // reset context only for non-root metafacades
{
context = getContext(context); // to have same value as in original constructor call
setMetafacadeContext (context);
}
}
/**
* @return boolean true always
* @see ModelElementFacade
*/
public boolean isModelElementFacadeMetaType()
{
return true;
}
// --------------- attributes ---------------------
/**
* @see ModelElementFacade#getVisibility()
* @return String
*/
protected abstract String handleGetVisibility();
private String __visibility1a;
private boolean __visibility1aSet = false;
/**
*
* The visibility (i.e. public, private, protected or package) of
* the model element, will attempt a lookup for these values in the
* language mappings (if any).
*
* @return (String)handleGetVisibility()
*/
public final String getVisibility()
{
String visibility1a = this.__visibility1a;
if (!this.__visibility1aSet)
{
// visibility has no pre constraints
visibility1a = handleGetVisibility();
// visibility has no post constraints
this.__visibility1a = visibility1a;
if (isMetafacadePropertyCachingEnabled())
{
this.__visibility1aSet = true;
}
}
return visibility1a;
}
/**
* @see ModelElementFacade#getPackagePath()
* @return String
*/
protected abstract String handleGetPackagePath();
private String __packagePath2a;
private boolean __packagePath2aSet = false;
/**
*
* Returns the package as a path, the returned value always starts
* with out a slash '/'.
*
* @return (String)handleGetPackagePath()
*/
public final String getPackagePath()
{
String packagePath2a = this.__packagePath2a;
if (!this.__packagePath2aSet)
{
// packagePath has no pre constraints
packagePath2a = handleGetPackagePath();
// packagePath has no post constraints
this.__packagePath2a = packagePath2a;
if (isMetafacadePropertyCachingEnabled())
{
this.__packagePath2aSet = true;
}
}
return packagePath2a;
}
/**
* @see ModelElementFacade#getName()
* @return String
*/
protected abstract String handleGetName();
private String __name3a;
private boolean __name3aSet = false;
/**
*
* The name of the model element.
*
* @return (String)handleGetName()
*/
public final String getName()
{
String name3a = this.__name3a;
if (!this.__name3aSet)
{
// name has no pre constraints
name3a = handleGetName();
// name has no post constraints
this.__name3a = name3a;
if (isMetafacadePropertyCachingEnabled())
{
this.__name3aSet = true;
}
}
return name3a;
}
/**
* @see ModelElementFacade#getPackageName()
* @return String
*/
protected abstract String handleGetPackageName();
private String __packageName4a;
private boolean __packageName4aSet = false;
/**
*
* The name of this model element's package.
*
* @return (String)handleGetPackageName()
*/
public final String getPackageName()
{
String packageName4a = this.__packageName4a;
if (!this.__packageName4aSet)
{
// packageName has no pre constraints
packageName4a = handleGetPackageName();
// packageName has no post constraints
this.__packageName4a = packageName4a;
if (isMetafacadePropertyCachingEnabled())
{
this.__packageName4aSet = true;
}
}
return packageName4a;
}
/**
* @see ModelElementFacade#getFullyQualifiedName()
* @return String
*/
protected abstract String handleGetFullyQualifiedName();
private String __fullyQualifiedName5a;
private boolean __fullyQualifiedName5aSet = false;
/**
*
* The fully qualified name of this model element.
*
* @return (String)handleGetFullyQualifiedName()
*/
public final String getFullyQualifiedName()
{
String fullyQualifiedName5a = this.__fullyQualifiedName5a;
if (!this.__fullyQualifiedName5aSet)
{
// fullyQualifiedName has no pre constraints
fullyQualifiedName5a = handleGetFullyQualifiedName();
// fullyQualifiedName has no post constraints
this.__fullyQualifiedName5a = fullyQualifiedName5a;
if (isMetafacadePropertyCachingEnabled())
{
this.__fullyQualifiedName5aSet = true;
}
}
return fullyQualifiedName5a;
}
/**
* @see ModelElementFacade#getFullyQualifiedNamePath()
* @return String
*/
protected abstract String handleGetFullyQualifiedNamePath();
private String __fullyQualifiedNamePath6a;
private boolean __fullyQualifiedNamePath6aSet = false;
/**
*
* Returns the fully qualified name as a path, the returned value
* always starts with out a slash '/'.
*
* @return (String)handleGetFullyQualifiedNamePath()
*/
public final String getFullyQualifiedNamePath()
{
String fullyQualifiedNamePath6a = this.__fullyQualifiedNamePath6a;
if (!this.__fullyQualifiedNamePath6aSet)
{
// fullyQualifiedNamePath has no pre constraints
fullyQualifiedNamePath6a = handleGetFullyQualifiedNamePath();
// fullyQualifiedNamePath has no post constraints
this.__fullyQualifiedNamePath6a = fullyQualifiedNamePath6a;
if (isMetafacadePropertyCachingEnabled())
{
this.__fullyQualifiedNamePath6aSet = true;
}
}
return fullyQualifiedNamePath6a;
}
/**
* @see ModelElementFacade#getLanguageMappings()
* @return TypeMappings
*/
protected abstract TypeMappings handleGetLanguageMappings();
private TypeMappings __languageMappings7a;
private boolean __languageMappings7aSet = false;
/**
*
* The language mappings that have been set for this model elemnt.
*
* @return (TypeMappings)handleGetLanguageMappings()
*/
public final TypeMappings getLanguageMappings()
{
TypeMappings languageMappings7a = this.__languageMappings7a;
if (!this.__languageMappings7aSet)
{
// languageMappings has no pre constraints
languageMappings7a = handleGetLanguageMappings();
// languageMappings has no post constraints
this.__languageMappings7a = languageMappings7a;
if (isMetafacadePropertyCachingEnabled())
{
this.__languageMappings7aSet = true;
}
}
return languageMappings7a;
}
/**
* @see ModelElementFacade#getStereotypeNames()
* @return Collection
*/
protected abstract Collection handleGetStereotypeNames();
private Collection __stereotypeNames8a;
private boolean __stereotypeNames8aSet = false;
/**
*
* The collection of ALL stereotype names for this model element.
*
* @return (Collection)handleGetStereotypeNames()
*/
public final Collection getStereotypeNames()
{
Collection stereotypeNames8a = this.__stereotypeNames8a;
if (!this.__stereotypeNames8aSet)
{
// stereotypeNames has no pre constraints
stereotypeNames8a = handleGetStereotypeNames();
// stereotypeNames has no post constraints
this.__stereotypeNames8a = stereotypeNames8a;
if (isMetafacadePropertyCachingEnabled())
{
this.__stereotypeNames8aSet = true;
}
}
return stereotypeNames8a;
}
/**
* @see ModelElementFacade#getId()
* @return String
*/
protected abstract String handleGetId();
private String __id9a;
private boolean __id9aSet = false;
/**
*
* Gets the unique identifier of the underlying model element.
*
* @return (String)handleGetId()
*/
public final String getId()
{
String id9a = this.__id9a;
if (!this.__id9aSet)
{
// id has no pre constraints
id9a = handleGetId();
// id has no post constraints
this.__id9a = id9a;
if (isMetafacadePropertyCachingEnabled())
{
this.__id9aSet = true;
}
}
return id9a;
}
/**
* @see ModelElementFacade#isConstraintsPresent()
* @return boolean
*/
protected abstract boolean handleIsConstraintsPresent();
private boolean __constraintsPresent10a;
private boolean __constraintsPresent10aSet = false;
/**
*
* Indicates if any constraints are present on this model element.
*
* @return (boolean)handleIsConstraintsPresent()
*/
public final boolean isConstraintsPresent()
{
boolean constraintsPresent10a = this.__constraintsPresent10a;
if (!this.__constraintsPresent10aSet)
{
// constraintsPresent has no pre constraints
constraintsPresent10a = handleIsConstraintsPresent();
// constraintsPresent has no post constraints
this.__constraintsPresent10a = constraintsPresent10a;
if (isMetafacadePropertyCachingEnabled())
{
this.__constraintsPresent10aSet = true;
}
}
return constraintsPresent10a;
}
/**
* @see ModelElementFacade#isBindingDependenciesPresent()
* @return boolean
*/
protected abstract boolean handleIsBindingDependenciesPresent();
private boolean __bindingDependenciesPresent11a;
private boolean __bindingDependenciesPresent11aSet = false;
/**
*
* @return (boolean)handleIsBindingDependenciesPresent()
*/
public final boolean isBindingDependenciesPresent()
{
boolean bindingDependenciesPresent11a = this.__bindingDependenciesPresent11a;
if (!this.__bindingDependenciesPresent11aSet)
{
// bindingDependenciesPresent has no pre constraints
bindingDependenciesPresent11a = handleIsBindingDependenciesPresent();
// bindingDependenciesPresent has no post constraints
this.__bindingDependenciesPresent11a = bindingDependenciesPresent11a;
if (isMetafacadePropertyCachingEnabled())
{
this.__bindingDependenciesPresent11aSet = true;
}
}
return bindingDependenciesPresent11a;
}
/**
* @see ModelElementFacade#isTemplateParametersPresent()
* @return boolean
*/
protected abstract boolean handleIsTemplateParametersPresent();
private boolean __templateParametersPresent12a;
private boolean __templateParametersPresent12aSet = false;
/**
*
* @return (boolean)handleIsTemplateParametersPresent()
*/
public final boolean isTemplateParametersPresent()
{
boolean templateParametersPresent12a = this.__templateParametersPresent12a;
if (!this.__templateParametersPresent12aSet)
{
// templateParametersPresent has no pre constraints
templateParametersPresent12a = handleIsTemplateParametersPresent();
// templateParametersPresent has no post constraints
this.__templateParametersPresent12a = templateParametersPresent12a;
if (isMetafacadePropertyCachingEnabled())
{
this.__templateParametersPresent12aSet = true;
}
}
return templateParametersPresent12a;
}
/**
* @see ModelElementFacade#getKeywords()
* @return Collection
*/
protected abstract Collection handleGetKeywords();
private Collection __keywords13a;
private boolean __keywords13aSet = false;
/**
*
* UML2: Retrieves the keywords for this element. Used to modify
* implementation properties which are not represented by other
* properties, i.e. native, transient, volatile, synchronized,
* (added annotations) override, deprecated. Can also be used to
* suppress compiler warnings: (added annotations) unchecked,
* fallthrough, path, serial, finally, all. Annotations require
* JDK5 compiler level.
*
* @return (Collection)handleGetKeywords()
*/
public final Collection getKeywords()
{
Collection keywords13a = this.__keywords13a;
if (!this.__keywords13aSet)
{
// keywords has no pre constraints
keywords13a = handleGetKeywords();
// keywords has no post constraints
this.__keywords13a = keywords13a;
if (isMetafacadePropertyCachingEnabled())
{
this.__keywords13aSet = true;
}
}
return keywords13a;
}
/**
* @see ModelElementFacade#getLabel()
* @return String
*/
protected abstract String handleGetLabel();
private String __label14a;
private boolean __label14aSet = false;
/**
*
* UML2: Retrieves a localized label for this named element.
*
* @return (String)handleGetLabel()
*/
public final String getLabel()
{
String label14a = this.__label14a;
if (!this.__label14aSet)
{
// label has no pre constraints
label14a = handleGetLabel();
// label has no post constraints
this.__label14a = label14a;
if (isMetafacadePropertyCachingEnabled())
{
this.__label14aSet = true;
}
}
return label14a;
}
/**
* @see ModelElementFacade#getQualifiedName()
* @return String
*/
protected abstract String handleGetQualifiedName();
private String __qualifiedName15a;
private boolean __qualifiedName15aSet = false;
/**
*
* UML2: Returns the value of the 'Qualified Name' attribute. A
* name which allows the NamedElement to be identified within a
* hierarchy of nested Namespaces. It is constructed from the names
* of the containing namespaces starting at the root of the
* hierarchy and ending with the name of the NamedElement itself.
*
* @return (String)handleGetQualifiedName()
*/
public final String getQualifiedName()
{
String qualifiedName15a = this.__qualifiedName15a;
if (!this.__qualifiedName15aSet)
{
// qualifiedName has no pre constraints
qualifiedName15a = handleGetQualifiedName();
// qualifiedName has no post constraints
this.__qualifiedName15a = qualifiedName15a;
if (isMetafacadePropertyCachingEnabled())
{
this.__qualifiedName15aSet = true;
}
}
return qualifiedName15a;
}
/**
* @see ModelElementFacade#isReservedWord()
* @return boolean
*/
protected abstract boolean handleIsReservedWord();
private boolean __reservedWord16a;
private boolean __reservedWord16aSet = false;
/**
*
* True if this element name is a reserved word in Java, C#, ANSI
* or ISO C, C++, JavaScript.
*
* @return (boolean)handleIsReservedWord()
*/
public final boolean isReservedWord()
{
boolean reservedWord16a = this.__reservedWord16a;
if (!this.__reservedWord16aSet)
{
// reservedWord has no pre constraints
reservedWord16a = handleIsReservedWord();
// reservedWord has no post constraints
this.__reservedWord16a = reservedWord16a;
if (isMetafacadePropertyCachingEnabled())
{
this.__reservedWord16aSet = true;
}
}
return reservedWord16a;
}
/**
* @see ModelElementFacade#isDocumentationPresent()
* @return boolean
*/
protected abstract boolean handleIsDocumentationPresent();
private boolean __documentationPresent17a;
private boolean __documentationPresent17aSet = false;
/**
*
* Indicates if any documentation is present on this model element.
*
* @return (boolean)handleIsDocumentationPresent()
*/
public final boolean isDocumentationPresent()
{
boolean documentationPresent17a = this.__documentationPresent17a;
if (!this.__documentationPresent17aSet)
{
// documentationPresent has no pre constraints
documentationPresent17a = handleIsDocumentationPresent();
// documentationPresent has no post constraints
this.__documentationPresent17a = documentationPresent17a;
if (isMetafacadePropertyCachingEnabled())
{
this.__documentationPresent17aSet = true;
}
}
return documentationPresent17a;
}
// ---------------- business methods ----------------------
/**
* Method to be implemented in descendants
*
* Finds the tagged value with the specified 'tagName'. In case
* there are more values the first one found will be returned.
*
* @param tagName
* @return Object
*/
protected abstract Object handleFindTaggedValue(String tagName);
/**
*
* Finds the tagged value with the specified 'tagName'. In case
* there are more values the first one found will be returned.
*
* @param tagName String
* @return handleFindTaggedValue(tagName)
*/
public Object findTaggedValue(String tagName)
{
// findTaggedValue has no pre constraints
Object returnValue = handleFindTaggedValue(tagName);
// findTaggedValue has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Returns true if the model element has the specified stereotype.
* If the stereotype itself does not match, then a search will be
* made up the stereotype inheritance hierarchy, and if one of the
* stereotype's ancestors has a matching name this method will
* return true, false otherwise.
*
*
* For example, if we have a certain stereotype called
* <> and a model element has a stereotype called
* <> which extends <>, when
* calling this method with 'stereotypeName' defined as 'exception'
* the method would return true since <>
* inherits from <>. If you want to check if the model
* element has the exact stereotype, then use the method
* 'hasExactStereotype' instead.
*
* @param stereotypeName
* @return boolean
*/
protected abstract boolean handleHasStereotype(String stereotypeName);
/**
*
* Returns true if the model element has the specified stereotype.
* If the stereotype itself does not match, then a search will be
* made up the stereotype inheritance hierarchy, and if one of the
* stereotype's ancestors has a matching name this method will
* return true, false otherwise.
*
*
* For example, if we have a certain stereotype called
* <> and a model element has a stereotype called
* <> which extends <>, when
* calling this method with 'stereotypeName' defined as 'exception'
* the method would return true since <>
* inherits from <>. If you want to check if the model
* element has the exact stereotype, then use the method
* 'hasExactStereotype' instead.
*
* @param stereotypeName String
* @return handleHasStereotype(stereotypeName)
*/
public boolean hasStereotype(String stereotypeName)
{
// hasStereotype has no pre constraints
boolean returnValue = handleHasStereotype(stereotypeName);
// hasStereotype has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Gets the documentation for the model element, The indent
* argument is prefixed to each line. By default this method wraps
* lines after 64 characters.
*
*
* This method is equivalent to getDocumentation(indent,
* 64)
.
*
* @param indent
* @return String
*/
protected abstract String handleGetDocumentation(String indent);
/**
*
* Gets the documentation for the model element, The indent
* argument is prefixed to each line. By default this method wraps
* lines after 64 characters.
*
*
* This method is equivalent to getDocumentation(indent,
* 64)
.
*
* @param indent String
*
*
Specifies the amount to indent by.
*
* @return handleGetDocumentation(indent)
*/
public String getDocumentation(String indent)
{
// getDocumentation has no pre constraints
String returnValue = handleGetDocumentation(indent);
// getDocumentation has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Returns the fully qualified name of the model element. The fully
* qualified name includes complete package qualified name of the
* underlying model element. If modelName is true, then the
* original name of the model element (the name contained within
* the model) will be the name returned, otherwise a name from a
* language mapping will be returned.
*
* @param modelName
* @return String
*/
protected abstract String handleGetFullyQualifiedName(boolean modelName);
/**
*
* Returns the fully qualified name of the model element. The fully
* qualified name includes complete package qualified name of the
* underlying model element. If modelName is true, then the
* original name of the model element (the name contained within
* the model) will be the name returned, otherwise a name from a
* language mapping will be returned.
*
* @param modelName boolean
*
* If true, then the original model name will be returned
* regardless of any underlying language mappings used.
*
* @return handleGetFullyQualifiedName(modelName)
*/
public String getFullyQualifiedName(boolean modelName)
{
// getFullyQualifiedName has no pre constraints
String returnValue = handleGetFullyQualifiedName(modelName);
// getFullyQualifiedName has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* This method returns the documentation for this model element,
* with the lines wrapped after the specified number of characters,
* values of less than 1 will indicate no line wrapping is
* required. By default paragraphs are returned as HTML.
*
*
* This method is equivalent to getDocumentation(indent,
* lineLength, true)
.
*
* @param indent
* @param lineLength
* @return String
*/
protected abstract String handleGetDocumentation(String indent, int lineLength);
/**
*
* This method returns the documentation for this model element,
* with the lines wrapped after the specified number of characters,
* values of less than 1 will indicate no line wrapping is
* required. By default paragraphs are returned as HTML.
*
*
* This method is equivalent to getDocumentation(indent,
* lineLength, true)
.
*
* @param indent String
* @param lineLength int
* @return handleGetDocumentation(indent, lineLength)
*/
public String getDocumentation(String indent, int lineLength)
{
// getDocumentation has no pre constraints
String returnValue = handleGetDocumentation(indent, lineLength);
// getDocumentation has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Returns true if the model element has the exact stereotype
* (meaning no stereotype inheritance is taken into account when
* searching for the stereotype), false otherwise.
*
* @param stereotypeName
* @return boolean
*/
protected abstract boolean handleHasExactStereotype(String stereotypeName);
/**
*
* Returns true if the model element has the exact stereotype
* (meaning no stereotype inheritance is taken into account when
* searching for the stereotype), false otherwise.
*
* @param stereotypeName String
*
* The name of the stereotype to check for.
*
* @return handleHasExactStereotype(stereotypeName)
*/
public boolean hasExactStereotype(String stereotypeName)
{
// hasExactStereotype has no pre constraints
boolean returnValue = handleHasExactStereotype(stereotypeName);
// hasExactStereotype has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Searches for the constraint with the specified 'name' on this
* model element, and if found translates it using the specified
* 'translation' from a translation library discovered by the
* framework.
*
* @param name
* @param translation
* @return String
*/
protected abstract String handleTranslateConstraint(String name, String translation);
/**
*
* Searches for the constraint with the specified 'name' on this
* model element, and if found translates it using the specified
* 'translation' from a translation library discovered by the
* framework.
*
* @param name String
*
* The name of the constraint to find.
*
* @param translation String
*
* The name of the translation to use. This must be a translation
* within a translation library discovered by the framework.
*
* @return handleTranslateConstraint(name, translation)
*/
public String translateConstraint(String name, String translation)
{
// translateConstraint has no pre constraints
String returnValue = handleTranslateConstraint(name, translation);
// translateConstraint has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Translates the constraints of the specified 'kind' belonging to
* this model element.
*
* @param kind
* @param translation
* @return String[]
*/
protected abstract String[] handleTranslateConstraints(String kind, String translation);
/**
*
* Translates the constraints of the specified 'kind' belonging to
* this model element.
*
* @param kind String
*
* The 'kind' of the contstraint (i.e. inv, body, pre, post, etc.).
*
* @param translation String
*
* The name of the translation to use. This must be a translation
* within a translation library discovered by the framework.
*
* @return handleTranslateConstraints(kind, translation)
*/
public String[] translateConstraints(String kind, String translation)
{
// translateConstraints has no pre constraints
String[] returnValue = handleTranslateConstraints(kind, translation);
// translateConstraints has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Translates all constraints belonging to this model element with
* the given 'translation'.
*
* @param translation
* @return String[]
*/
protected abstract String[] handleTranslateConstraints(String translation);
/**
*
* Translates all constraints belonging to this model element with
* the given 'translation'.
*
* @param translation String
*
* The name of the translation to use. This must be a translation
* within a translation library discovered by the framework.
*
* @return handleTranslateConstraints(translation)
*/
public String[] translateConstraints(String translation)
{
// translateConstraints has no pre constraints
String[] returnValue = handleTranslateConstraints(translation);
// translateConstraints has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Returns the constraints of the argument kind that have been
* placed onto this model. Typical kinds are "inv", "pre" and
* "post". Other kinds are possible.
*
* @param kind
* @return Collection
*/
protected abstract Collection handleGetConstraints(String kind);
/**
*
* Returns the constraints of the argument kind that have been
* placed onto this model. Typical kinds are "inv", "pre" and
* "post". Other kinds are possible.
*
* @param kind String
* @return handleGetConstraints(kind)
*/
public Collection getConstraints(String kind)
{
// getConstraints has no pre constraints
Collection returnValue = handleGetConstraints(kind);
// getConstraints has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Returns all the values for the tagged value with the specified
* name. The returned collection will contains only String
* instances, or will be empty. Never null.
*
* @param tagName
* @return Collection
*/
protected abstract Collection handleFindTaggedValues(String tagName);
/**
*
* Returns all the values for the tagged value with the specified
* name. The returned collection will contains only String
* instances, or will be empty. Never null.
*
* @param tagName String
* @return handleFindTaggedValues(tagName)
*/
public Collection findTaggedValues(String tagName)
{
// findTaggedValues has no pre constraints
Collection returnValue = handleFindTaggedValues(tagName);
// findTaggedValues has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* @param indent
* @param lineLength
* @param htmlStyle
* @return String
*/
protected abstract String handleGetDocumentation(String indent, int lineLength, boolean htmlStyle);
/**
*
* @param indent String
* @param lineLength int
* @param htmlStyle boolean
* @return handleGetDocumentation(indent, lineLength, htmlStyle)
*/
public String getDocumentation(String indent, int lineLength, boolean htmlStyle)
{
// getDocumentation has no pre constraints
String returnValue = handleGetDocumentation(indent, lineLength, htmlStyle);
// getDocumentation has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Gets the package name (optionally providing the ability to
* retrieve the model name and not the mapped name).
*
* @param modelName
* @return String
*/
protected abstract String handleGetPackageName(boolean modelName);
/**
*
* Gets the package name (optionally providing the ability to
* retrieve the model name and not the mapped name).
*
* @param modelName boolean
*
* A flag indicating whether or not the model name should be
* retrieved.
*
* @return handleGetPackageName(modelName)
*/
public String getPackageName(boolean modelName)
{
// getPackageName has no pre constraints
String returnValue = handleGetPackageName(modelName);
// getPackageName has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Copies all tagged values from the given ModelElementFacade to
* this model element facade.
*
* @param element
*/
protected abstract void handleCopyTaggedValues(ModelElementFacade element);
/**
*
* Copies all tagged values from the given ModelElementFacade to
* this model element facade.
*
* @param element ModelElementFacade
*
* The element from which to copy the tagged values.
*
*/
public void copyTaggedValues(ModelElementFacade element)
{
// copyTaggedValues has no pre constraints
handleCopyTaggedValues(element);
// copyTaggedValues has no post constraints
}
/**
* Method to be implemented in descendants
*
* @param parameterName
* @return Object
*/
protected abstract Object handleGetTemplateParameter(String parameterName);
/**
*
* @param parameterName String
* @return handleGetTemplateParameter(parameterName)
*/
public Object getTemplateParameter(String parameterName)
{
// getTemplateParameter has no pre constraints
Object returnValue = handleGetTemplateParameter(parameterName);
// getTemplateParameter has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Does the UML Element contain the named Keyword? Keywords can be
* separated by space, comma, pipe, semicolon, or << >>
*
* @param keywordName
* @return boolean
*/
protected abstract boolean handleHasKeyword(String keywordName);
/**
*
* Does the UML Element contain the named Keyword? Keywords can be
* separated by space, comma, pipe, semicolon, or << >>
*
* @param keywordName String
*
* Keyword to find in the list of UML Element Keywords.
*
* @return handleHasKeyword(keywordName)
*/
public boolean hasKeyword(String keywordName)
{
// hasKeyword has no pre constraints
boolean returnValue = handleHasKeyword(keywordName);
// hasKeyword has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Returns the fully qualified name of the model element. The fully
* qualified name includes complete package qualified name of the
* underlying model element. The templates parameter will be
* replaced by the correct one given the binding relation of the
* parameter to this element.
*
* @param bindedElement
* @return String
*/
protected abstract String handleGetBindedFullyQualifiedName(ModelElementFacade bindedElement);
/**
*
* Returns the fully qualified name of the model element. The fully
* qualified name includes complete package qualified name of the
* underlying model element. The templates parameter will be
* replaced by the correct one given the binding relation of the
* parameter to this element.
*
* @param bindedElement ModelElementFacade
*
* the element that will be used to find binding to the templates
* parameters of this element.
*
* @return handleGetBindedFullyQualifiedName(bindedElement)
*/
public String getBindedFullyQualifiedName(ModelElementFacade bindedElement)
{
// getBindedFullyQualifiedName has no pre constraints
String returnValue = handleGetBindedFullyQualifiedName(bindedElement);
// getBindedFullyQualifiedName has no post constraints
return returnValue;
}
// ------------- associations ------------------
/**
*
* @return (ModelElementFacade)handleGetPackage()
*/
public final ModelElementFacade getPackage()
{
ModelElementFacade getPackage1r = null;
// modelElementFacade has no pre constraints
Object result = handleGetPackage();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getPackage1r = (ModelElementFacade)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
ModelElementFacadeLogic.logger.warn("incorrect metafacade cast for ModelElementFacadeLogic.getPackage ModelElementFacade " + result + ": " + shieldedResult);
}
// modelElementFacade has no post constraints
return getPackage1r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetPackage();
/**
*
* Gets all the model elements belonging to the root package.
*
* @return (PackageFacade)handleGetRootPackage()
*/
public final PackageFacade getRootPackage()
{
PackageFacade getRootPackage3r = null;
// modelElements has no pre constraints
Object result = handleGetRootPackage();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getRootPackage3r = (PackageFacade)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
ModelElementFacadeLogic.logger.warn("incorrect metafacade cast for ModelElementFacadeLogic.getRootPackage PackageFacade " + result + ": " + shieldedResult);
}
// modelElements has no post constraints
return getRootPackage3r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetRootPackage();
/**
*
* Gets the element to which the dependencies belong.
*
* @return (Collection)handleGetTargetDependencies()
*/
public final Collection getTargetDependencies()
{
Collection getTargetDependencies4r = null;
// targetElement has no pre constraints
Collection result = handleGetTargetDependencies();
List shieldedResult = this.shieldedElements(result);
try
{
getTargetDependencies4r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
ModelElementFacadeLogic.logger.warn("incorrect metafacade cast for ModelElementFacadeLogic.getTargetDependencies Collection " + result + ": " + shieldedResult);
}
// targetElement has no post constraints
return getTargetDependencies4r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetTargetDependencies();
/**
*
* @return (ModelFacade)handleGetModel()
*/
public final ModelFacade getModel()
{
ModelFacade getModel6r = null;
// modelElementFacade has no pre constraints
Object result = handleGetModel();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getModel6r = (ModelFacade)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
ModelElementFacadeLogic.logger.warn("incorrect metafacade cast for ModelElementFacadeLogic.getModel ModelFacade " + result + ": " + shieldedResult);
}
// modelElementFacade has no post constraints
return getModel6r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetModel();
/**
*
* @return (Collection)handleGetStereotypes()
*/
public final Collection getStereotypes()
{
Collection getStereotypes7r = null;
// modelElementFacade has no pre constraints
Collection result = handleGetStereotypes();
List shieldedResult = this.shieldedElements(result);
try
{
getStereotypes7r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
ModelElementFacadeLogic.logger.warn("incorrect metafacade cast for ModelElementFacadeLogic.getStereotypes Collection " + result + ": " + shieldedResult);
}
// modelElementFacade has no post constraints
return getStereotypes7r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetStereotypes();
/**
*
* Gets the model element to which the constraint applies (i.e. is
* the context of).
*
* @return (Collection)handleGetConstraints()
*/
public final Collection getConstraints()
{
Collection getConstraints8r = null;
// contextElement has no pre constraints
Collection result = handleGetConstraints();
List shieldedResult = this.shieldedElements(result);
try
{
getConstraints8r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
ModelElementFacadeLogic.logger.warn("incorrect metafacade cast for ModelElementFacadeLogic.getConstraints Collection " + result + ": " + shieldedResult);
}
// contextElement has no post constraints
return getConstraints8r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetConstraints();
/**
*
* The source element of this dependency.
*
* @return (Collection)handleGetSourceDependencies()
*/
public final Collection getSourceDependencies()
{
Collection getSourceDependencies10r = null;
// sourceElement has no pre constraints
Collection result = handleGetSourceDependencies();
List shieldedResult = this.shieldedElements(result);
try
{
getSourceDependencies10r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
ModelElementFacadeLogic.logger.warn("incorrect metafacade cast for ModelElementFacadeLogic.getSourceDependencies Collection " + result + ": " + shieldedResult);
}
// sourceElement has no post constraints
return getSourceDependencies10r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetSourceDependencies();
/**
*
* @return (StateMachineFacade)handleGetStateMachineContext()
*/
public final StateMachineFacade getStateMachineContext()
{
StateMachineFacade getStateMachineContext11r = null;
// contextElement has no pre constraints
Object result = handleGetStateMachineContext();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getStateMachineContext11r = (StateMachineFacade)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
ModelElementFacadeLogic.logger.warn("incorrect metafacade cast for ModelElementFacadeLogic.getStateMachineContext StateMachineFacade " + result + ": " + shieldedResult);
}
// contextElement has no post constraints
return getStateMachineContext11r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetStateMachineContext();
/**
*
* @return (Collection)handleGetTemplateParameters()
*/
public final Collection getTemplateParameters()
{
Collection getTemplateParameters13r = null;
// modelElementFacade has no pre constraints
Collection result = handleGetTemplateParameters();
List shieldedResult = this.shieldedElements(result);
try
{
getTemplateParameters13r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
ModelElementFacadeLogic.logger.warn("incorrect metafacade cast for ModelElementFacadeLogic.getTemplateParameters Collection " + result + ": " + shieldedResult);
}
// modelElementFacade has no post constraints
return getTemplateParameters13r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetTemplateParameters();
/**
*
* @return (Collection)handleGetTaggedValues()
*/
public final Collection getTaggedValues()
{
Collection getTaggedValues16r = null;
// modelElementFacade has no pre constraints
Collection result = handleGetTaggedValues();
List shieldedResult = this.shieldedElements(result);
try
{
getTaggedValues16r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
ModelElementFacadeLogic.logger.warn("incorrect metafacade cast for ModelElementFacadeLogic.getTaggedValues Collection " + result + ": " + shieldedResult);
}
// modelElementFacade has no post constraints
return getTaggedValues16r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetTaggedValues();
/**
* @param validationMessages Collection
* @see MetafacadeBase#validateInvariants(Collection validationMessages)
*/
@Override
public void validateInvariants(Collection validationMessages)
{
}
/**
* The property that stores the name of the metafacade.
*/
private static final String NAME_PROPERTY = "name";
private static final String FQNAME_PROPERTY = "fullyQualifiedName";
/**
* @see Object#toString()
*/
@Override
public String toString()
{
final StringBuilder toString = new StringBuilder(this.getClass().getName());
toString.append("[");
try
{
toString.append(Introspector.instance().getProperty(this, FQNAME_PROPERTY));
}
catch (final Throwable tryAgain)
{
try
{
toString.append(Introspector.instance().getProperty(this, NAME_PROPERTY));
}
catch (final Throwable ignore)
{
// - just ignore when the metafacade doesn't have a name or fullyQualifiedName property
}
}
toString.append("]");
return toString.toString();
}
}