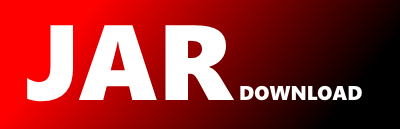
org.andromda.metafacades.uml14.OperationFacadeLogic Maven / Gradle / Ivy
// license-header java merge-point
//
// Attention: generated code (by MetafacadeLogic.vsl) - do not modify!
//
package org.andromda.metafacades.uml14;
import java.util.Collection;
import java.util.List;
import org.andromda.core.metafacade.MetafacadeBase;
import org.andromda.core.metafacade.ModelValidationMessage;
import org.andromda.metafacades.uml.ClassifierFacade;
import org.andromda.metafacades.uml.ConstraintFacade;
import org.andromda.metafacades.uml.OperationFacade;
import org.andromda.metafacades.uml.ParameterFacade;
import org.andromda.translation.ocl.validation.OCLCollections;
import org.andromda.translation.ocl.validation.OCLExpressions;
import org.andromda.translation.ocl.validation.OCLIntrospector;
import org.andromda.translation.ocl.validation.OCLResultEnsurer;
import org.apache.log4j.Logger;
import org.omg.uml.foundation.core.Operation;
/**
*
* A behavioral feature of a classifier that specifies the name,
* type, parameters, and constraints for invoking an associated
* behavior. May invoke both the execution of method behaviors as
* well as other behavioral responses.
*
* MetafacadeLogic for OperationFacade
*
* @see OperationFacade
*/
public abstract class OperationFacadeLogic
extends ModelElementFacadeLogicImpl
implements OperationFacade
{
/**
* The underlying UML object
* @see Operation
*/
protected Operation metaObject;
/** Create Metafacade implementation instance using the MetafacadeFactory from the context
* @param metaObjectIn
* @param context
*/
protected OperationFacadeLogic(Operation metaObjectIn, String context)
{
super(metaObjectIn, getContext(context));
this.metaObject = metaObjectIn;
}
/**
* The logger instance.
*/
private static final Logger logger = Logger.getLogger(OperationFacadeLogic.class);
/**
* Gets the context for this metafacade logic instance.
* @param context String. Set to OperationFacade if null
* @return context String
*/
private static String getContext(String context)
{
if (context == null)
{
context = "org.andromda.metafacades.uml.OperationFacade";
}
return context;
}
/** Reset context only for non-root metafacades
* @param context
*/
@Override
public void resetMetafacadeContext(String context)
{
if (!this.contextRoot) // reset context only for non-root metafacades
{
context = getContext(context); // to have same value as in original constructor call
setMetafacadeContext (context);
}
}
/**
* @return boolean true always
* @see OperationFacade
*/
public boolean isOperationFacadeMetaType()
{
return true;
}
// --------------- attributes ---------------------
/**
* @see OperationFacade#getSignature()
* @return String
*/
protected abstract String handleGetSignature();
private String __signature1a;
private boolean __signature1aSet = false;
/**
*
* @return (String)handleGetSignature()
*/
public final String getSignature()
{
String signature1a = this.__signature1a;
if (!this.__signature1aSet)
{
// signature has no pre constraints
signature1a = handleGetSignature();
// signature has no post constraints
this.__signature1a = signature1a;
if (isMetafacadePropertyCachingEnabled())
{
this.__signature1aSet = true;
}
}
return signature1a;
}
/**
* @see OperationFacade#getCall()
* @return String
*/
protected abstract String handleGetCall();
private String __call2a;
private boolean __call2aSet = false;
/**
*
* @return (String)handleGetCall()
*/
public final String getCall()
{
String call2a = this.__call2a;
if (!this.__call2aSet)
{
// call has no pre constraints
call2a = handleGetCall();
// call has no post constraints
this.__call2a = call2a;
if (isMetafacadePropertyCachingEnabled())
{
this.__call2aSet = true;
}
}
return call2a;
}
/**
* @see OperationFacade#getTypedArgumentList()
* @return String
*/
protected abstract String handleGetTypedArgumentList();
private String __typedArgumentList3a;
private boolean __typedArgumentList3aSet = false;
/**
*
* A comma-separated parameter list (type and name of each
* parameter) of an operation.
*
* @return (String)handleGetTypedArgumentList()
*/
public final String getTypedArgumentList()
{
String typedArgumentList3a = this.__typedArgumentList3a;
if (!this.__typedArgumentList3aSet)
{
// typedArgumentList has no pre constraints
typedArgumentList3a = handleGetTypedArgumentList();
// typedArgumentList has no post constraints
this.__typedArgumentList3a = typedArgumentList3a;
if (isMetafacadePropertyCachingEnabled())
{
this.__typedArgumentList3aSet = true;
}
}
return typedArgumentList3a;
}
/**
* @see OperationFacade#isStatic()
* @return boolean
*/
protected abstract boolean handleIsStatic();
private boolean __static4a;
private boolean __static4aSet = false;
/**
*
* @return (boolean)handleIsStatic()
*/
public final boolean isStatic()
{
boolean static4a = this.__static4a;
if (!this.__static4aSet)
{
// static has no pre constraints
static4a = handleIsStatic();
// static has no post constraints
this.__static4a = static4a;
if (isMetafacadePropertyCachingEnabled())
{
this.__static4aSet = true;
}
}
return static4a;
}
/**
* @see OperationFacade#isAbstract()
* @return boolean
*/
protected abstract boolean handleIsAbstract();
private boolean __abstract5a;
private boolean __abstract5aSet = false;
/**
*
* @return (boolean)handleIsAbstract()
*/
public final boolean isAbstract()
{
boolean abstract5a = this.__abstract5a;
if (!this.__abstract5aSet)
{
// abstract has no pre constraints
abstract5a = handleIsAbstract();
// abstract has no post constraints
this.__abstract5a = abstract5a;
if (isMetafacadePropertyCachingEnabled())
{
this.__abstract5aSet = true;
}
}
return abstract5a;
}
/**
* @see OperationFacade#getExceptionList()
* @return String
*/
protected abstract String handleGetExceptionList();
private String __exceptionList6a;
private boolean __exceptionList6aSet = false;
/**
*
* A comma separated list containing all exceptions that this
* operation throws. Exceptions are determined through
* dependencies that have the target element stereotyped as
* <>.
*
* @return (String)handleGetExceptionList()
*/
public final String getExceptionList()
{
String exceptionList6a = this.__exceptionList6a;
if (!this.__exceptionList6aSet)
{
// exceptionList has no pre constraints
exceptionList6a = handleGetExceptionList();
// exceptionList has no post constraints
this.__exceptionList6a = exceptionList6a;
if (isMetafacadePropertyCachingEnabled())
{
this.__exceptionList6aSet = true;
}
}
return exceptionList6a;
}
/**
* @see OperationFacade#getExceptions()
* @return Collection
*/
protected abstract Collection handleGetExceptions();
private Collection __exceptions7a;
private boolean __exceptions7aSet = false;
/**
*
* A collection of all exceptions thrown by this operation.
*
* @return (Collection)handleGetExceptions()
*/
public final Collection getExceptions()
{
Collection exceptions7a = this.__exceptions7a;
if (!this.__exceptions7aSet)
{
// exceptions has no pre constraints
exceptions7a = handleGetExceptions();
// exceptions has no post constraints
this.__exceptions7a = exceptions7a;
if (isMetafacadePropertyCachingEnabled())
{
this.__exceptions7aSet = true;
}
}
return exceptions7a;
}
/**
* @see OperationFacade#isReturnTypePresent()
* @return boolean
*/
protected abstract boolean handleIsReturnTypePresent();
private boolean __returnTypePresent8a;
private boolean __returnTypePresent8aSet = false;
/**
*
* True/false depending on whether or not the operation has a
* return type or not (i.e. a return type of something other than
* void).
*
* @return (boolean)handleIsReturnTypePresent()
*/
public final boolean isReturnTypePresent()
{
boolean returnTypePresent8a = this.__returnTypePresent8a;
if (!this.__returnTypePresent8aSet)
{
// returnTypePresent has no pre constraints
returnTypePresent8a = handleIsReturnTypePresent();
// returnTypePresent has no post constraints
this.__returnTypePresent8a = returnTypePresent8a;
if (isMetafacadePropertyCachingEnabled())
{
this.__returnTypePresent8aSet = true;
}
}
return returnTypePresent8a;
}
/**
* @see OperationFacade#isExceptionsPresent()
* @return boolean
*/
protected abstract boolean handleIsExceptionsPresent();
private boolean __exceptionsPresent9a;
private boolean __exceptionsPresent9aSet = false;
/**
*
* True if the operation has (i.e. throws any exceptions) false
* otherwise.
*
* @return (boolean)handleIsExceptionsPresent()
*/
public final boolean isExceptionsPresent()
{
boolean exceptionsPresent9a = this.__exceptionsPresent9a;
if (!this.__exceptionsPresent9aSet)
{
// exceptionsPresent has no pre constraints
exceptionsPresent9a = handleIsExceptionsPresent();
// exceptionsPresent has no post constraints
this.__exceptionsPresent9a = exceptionsPresent9a;
if (isMetafacadePropertyCachingEnabled())
{
this.__exceptionsPresent9aSet = true;
}
}
return exceptionsPresent9a;
}
/**
* @see OperationFacade#getArgumentNames()
* @return String
*/
protected abstract String handleGetArgumentNames();
private String __argumentNames10a;
private boolean __argumentNames10aSet = false;
/**
*
* A comma separated list of all argument names.
*
* @return (String)handleGetArgumentNames()
*/
public final String getArgumentNames()
{
String argumentNames10a = this.__argumentNames10a;
if (!this.__argumentNames10aSet)
{
// argumentNames has no pre constraints
argumentNames10a = handleGetArgumentNames();
// argumentNames has no post constraints
this.__argumentNames10a = argumentNames10a;
if (isMetafacadePropertyCachingEnabled())
{
this.__argumentNames10aSet = true;
}
}
return argumentNames10a;
}
/**
* @see OperationFacade#getArgumentTypeNames()
* @return String
*/
protected abstract String handleGetArgumentTypeNames();
private String __argumentTypeNames11a;
private boolean __argumentTypeNames11aSet = false;
/**
*
* @return (String)handleGetArgumentTypeNames()
*/
public final String getArgumentTypeNames()
{
String argumentTypeNames11a = this.__argumentTypeNames11a;
if (!this.__argumentTypeNames11aSet)
{
// argumentTypeNames has no pre constraints
argumentTypeNames11a = handleGetArgumentTypeNames();
// argumentTypeNames has no post constraints
this.__argumentTypeNames11a = argumentTypeNames11a;
if (isMetafacadePropertyCachingEnabled())
{
this.__argumentTypeNames11aSet = true;
}
}
return argumentTypeNames11a;
}
/**
* @see OperationFacade#isQuery()
* @return boolean
*/
protected abstract boolean handleIsQuery();
private boolean __query12a;
private boolean __query12aSet = false;
/**
*
* Indicates whether or not this operation is a query operation.
*
* @return (boolean)handleIsQuery()
*/
public final boolean isQuery()
{
boolean query12a = this.__query12a;
if (!this.__query12aSet)
{
// query has no pre constraints
query12a = handleIsQuery();
// query has no post constraints
this.__query12a = query12a;
if (isMetafacadePropertyCachingEnabled())
{
this.__query12aSet = true;
}
}
return query12a;
}
/**
* @see OperationFacade#getConcurrency()
* @return String
*/
protected abstract String handleGetConcurrency();
private String __concurrency13a;
private boolean __concurrency13aSet = false;
/**
*
* Returns the concurrency modifier for this operation (i.e.
* concurrent, guarded or sequential) of the model element, will
* attempt a lookup for these values in the language mappings (if
* any).
*
* @return (String)handleGetConcurrency()
*/
public final String getConcurrency()
{
String concurrency13a = this.__concurrency13a;
if (!this.__concurrency13aSet)
{
// concurrency has no pre constraints
concurrency13a = handleGetConcurrency();
// concurrency has no post constraints
this.__concurrency13a = concurrency13a;
if (isMetafacadePropertyCachingEnabled())
{
this.__concurrency13aSet = true;
}
}
return concurrency13a;
}
/**
* @see OperationFacade#getPreconditionName()
* @return String
*/
protected abstract String handleGetPreconditionName();
private String __preconditionName14a;
private boolean __preconditionName14aSet = false;
/**
*
* The name of the operation that handles precondition constraints.
*
* @return (String)handleGetPreconditionName()
*/
public final String getPreconditionName()
{
String preconditionName14a = this.__preconditionName14a;
if (!this.__preconditionName14aSet)
{
// preconditionName has no pre constraints
preconditionName14a = handleGetPreconditionName();
// preconditionName has no post constraints
this.__preconditionName14a = preconditionName14a;
if (isMetafacadePropertyCachingEnabled())
{
this.__preconditionName14aSet = true;
}
}
return preconditionName14a;
}
/**
* @see OperationFacade#getPostconditionName()
* @return String
*/
protected abstract String handleGetPostconditionName();
private String __postconditionName15a;
private boolean __postconditionName15aSet = false;
/**
*
* The name of the operation that handles postcondition
* constraints.
*
* @return (String)handleGetPostconditionName()
*/
public final String getPostconditionName()
{
String postconditionName15a = this.__postconditionName15a;
if (!this.__postconditionName15aSet)
{
// postconditionName has no pre constraints
postconditionName15a = handleGetPostconditionName();
// postconditionName has no post constraints
this.__postconditionName15a = postconditionName15a;
if (isMetafacadePropertyCachingEnabled())
{
this.__postconditionName15aSet = true;
}
}
return postconditionName15a;
}
/**
* @see OperationFacade#getPreconditionSignature()
* @return String
*/
protected abstract String handleGetPreconditionSignature();
private String __preconditionSignature16a;
private boolean __preconditionSignature16aSet = false;
/**
*
* The signature of the precondition operation.
*
* @return (String)handleGetPreconditionSignature()
*/
public final String getPreconditionSignature()
{
String preconditionSignature16a = this.__preconditionSignature16a;
if (!this.__preconditionSignature16aSet)
{
// preconditionSignature has no pre constraints
preconditionSignature16a = handleGetPreconditionSignature();
// preconditionSignature has no post constraints
this.__preconditionSignature16a = preconditionSignature16a;
if (isMetafacadePropertyCachingEnabled())
{
this.__preconditionSignature16aSet = true;
}
}
return preconditionSignature16a;
}
/**
* @see OperationFacade#getPreconditionCall()
* @return String
*/
protected abstract String handleGetPreconditionCall();
private String __preconditionCall17a;
private boolean __preconditionCall17aSet = false;
/**
*
* The call to the precondition operation.
*
* @return (String)handleGetPreconditionCall()
*/
public final String getPreconditionCall()
{
String preconditionCall17a = this.__preconditionCall17a;
if (!this.__preconditionCall17aSet)
{
// preconditionCall has no pre constraints
preconditionCall17a = handleGetPreconditionCall();
// preconditionCall has no post constraints
this.__preconditionCall17a = preconditionCall17a;
if (isMetafacadePropertyCachingEnabled())
{
this.__preconditionCall17aSet = true;
}
}
return preconditionCall17a;
}
/**
* @see OperationFacade#isPreconditionsPresent()
* @return boolean
*/
protected abstract boolean handleIsPreconditionsPresent();
private boolean __preconditionsPresent18a;
private boolean __preconditionsPresent18aSet = false;
/**
*
* Whether any precondition constraints are present on this
* operation.
*
* @return (boolean)handleIsPreconditionsPresent()
*/
public final boolean isPreconditionsPresent()
{
boolean preconditionsPresent18a = this.__preconditionsPresent18a;
if (!this.__preconditionsPresent18aSet)
{
// preconditionsPresent has no pre constraints
preconditionsPresent18a = handleIsPreconditionsPresent();
// preconditionsPresent has no post constraints
this.__preconditionsPresent18a = preconditionsPresent18a;
if (isMetafacadePropertyCachingEnabled())
{
this.__preconditionsPresent18aSet = true;
}
}
return preconditionsPresent18a;
}
/**
* @see OperationFacade#isPostconditionsPresent()
* @return boolean
*/
protected abstract boolean handleIsPostconditionsPresent();
private boolean __postconditionsPresent19a;
private boolean __postconditionsPresent19aSet = false;
/**
*
* Whether any postcondition constraints are present on this
* operation.
*
* @return (boolean)handleIsPostconditionsPresent()
*/
public final boolean isPostconditionsPresent()
{
boolean postconditionsPresent19a = this.__postconditionsPresent19a;
if (!this.__postconditionsPresent19aSet)
{
// postconditionsPresent has no pre constraints
postconditionsPresent19a = handleIsPostconditionsPresent();
// postconditionsPresent has no post constraints
this.__postconditionsPresent19a = postconditionsPresent19a;
if (isMetafacadePropertyCachingEnabled())
{
this.__postconditionsPresent19aSet = true;
}
}
return postconditionsPresent19a;
}
/**
* @see OperationFacade#getLower()
* @return int
*/
protected abstract int handleGetLower();
private int __lower20a;
private boolean __lower20aSet = false;
/**
*
* the lower value for the multiplicity
*
*
* -only applicable for UML2
*
* @return (int)handleGetLower()
*/
public final int getLower()
{
int lower20a = this.__lower20a;
if (!this.__lower20aSet)
{
// lower has no pre constraints
lower20a = handleGetLower();
// lower has no post constraints
this.__lower20a = lower20a;
if (isMetafacadePropertyCachingEnabled())
{
this.__lower20aSet = true;
}
}
return lower20a;
}
/**
* @see OperationFacade#getUpper()
* @return int
*/
protected abstract int handleGetUpper();
private int __upper21a;
private boolean __upper21aSet = false;
/**
*
* the upper value for the multiplicity (will be -1 for *)
*
*
* - only applicable for UML2
*
* @return (int)handleGetUpper()
*/
public final int getUpper()
{
int upper21a = this.__upper21a;
if (!this.__upper21aSet)
{
// upper has no pre constraints
upper21a = handleGetUpper();
// upper has no post constraints
this.__upper21a = upper21a;
if (isMetafacadePropertyCachingEnabled())
{
this.__upper21aSet = true;
}
}
return upper21a;
}
/**
* @see OperationFacade#getReturnParameter()
* @return ParameterFacade
*/
protected abstract ParameterFacade handleGetReturnParameter();
private ParameterFacade __returnParameter22a;
private boolean __returnParameter22aSet = false;
/**
*
* (UML2 Only). Get the actual return parameter (which may have
* stereotypes etc).
*
* @return (ParameterFacade)handleGetReturnParameter()
*/
public final ParameterFacade getReturnParameter()
{
ParameterFacade returnParameter22a = this.__returnParameter22a;
if (!this.__returnParameter22aSet)
{
// returnParameter has no pre constraints
returnParameter22a = handleGetReturnParameter();
// returnParameter has no post constraints
this.__returnParameter22a = returnParameter22a;
if (isMetafacadePropertyCachingEnabled())
{
this.__returnParameter22aSet = true;
}
}
return returnParameter22a;
}
/**
* @see OperationFacade#isOverriding()
* @return boolean
*/
protected abstract boolean handleIsOverriding();
private boolean __overriding23a;
private boolean __overriding23aSet = false;
/**
*
* True if this operation overrides an operation defined in an
* ancestor class. An operation overrides when the names of the
* operations as well as the types of the arguments are equal. The
* return type may be different and is, as well as any exceptions,
* ignored.
*
* @return (boolean)handleIsOverriding()
*/
public final boolean isOverriding()
{
boolean overriding23a = this.__overriding23a;
if (!this.__overriding23aSet)
{
// overriding has no pre constraints
overriding23a = handleIsOverriding();
// overriding has no post constraints
this.__overriding23a = overriding23a;
if (isMetafacadePropertyCachingEnabled())
{
this.__overriding23aSet = true;
}
}
return overriding23a;
}
/**
* @see OperationFacade#isOrdered()
* @return boolean
*/
protected abstract boolean handleIsOrdered();
private boolean __ordered24a;
private boolean __ordered24aSet = false;
/**
*
* UML2 only: If isMany (Collection type returned), is the type
* unique within the collection. Unique+Ordered determines
* CollectionType implementation of return result. Default=false.
*
* @return (boolean)handleIsOrdered()
*/
public final boolean isOrdered()
{
boolean ordered24a = this.__ordered24a;
if (!this.__ordered24aSet)
{
// ordered has no pre constraints
ordered24a = handleIsOrdered();
// ordered has no post constraints
this.__ordered24a = ordered24a;
if (isMetafacadePropertyCachingEnabled())
{
this.__ordered24aSet = true;
}
}
return ordered24a;
}
/**
* @see OperationFacade#getGetterSetterReturnTypeName()
* @return String
*/
protected abstract String handleGetGetterSetterReturnTypeName();
private String __getterSetterReturnTypeName25a;
private boolean __getterSetterReturnTypeName25aSet = false;
/**
*
* Return Type with multiplicity taken into account. UML14 does not
* allow multiplicity *.
*
* @return (String)handleGetGetterSetterReturnTypeName()
*/
public final String getGetterSetterReturnTypeName()
{
String getterSetterReturnTypeName25a = this.__getterSetterReturnTypeName25a;
if (!this.__getterSetterReturnTypeName25aSet)
{
// getterSetterReturnTypeName has no pre constraints
getterSetterReturnTypeName25a = handleGetGetterSetterReturnTypeName();
// getterSetterReturnTypeName has no post constraints
this.__getterSetterReturnTypeName25a = getterSetterReturnTypeName25a;
if (isMetafacadePropertyCachingEnabled())
{
this.__getterSetterReturnTypeName25aSet = true;
}
}
return getterSetterReturnTypeName25a;
}
/**
* @see OperationFacade#isMany()
* @return boolean
*/
protected abstract boolean handleIsMany();
private boolean __many26a;
private boolean __many26aSet = false;
/**
*
* UML2 only. If the return type parameter multiplicity>1 OR the
* operation multiplicity>1. Default=false.
*
* @return (boolean)handleIsMany()
*/
public final boolean isMany()
{
boolean many26a = this.__many26a;
if (!this.__many26aSet)
{
// many has no pre constraints
many26a = handleIsMany();
// many has no post constraints
this.__many26a = many26a;
if (isMetafacadePropertyCachingEnabled())
{
this.__many26aSet = true;
}
}
return many26a;
}
/**
* @see OperationFacade#isUnique()
* @return boolean
*/
protected abstract boolean handleIsUnique();
private boolean __unique27a;
private boolean __unique27aSet = false;
/**
*
* UML2 only: for Collection return type, is the type unique within
* the collection. Unique+Ordered determines the returned
* CollectionType. Default=false.
*
* @return (boolean)handleIsUnique()
*/
public final boolean isUnique()
{
boolean unique27a = this.__unique27a;
if (!this.__unique27aSet)
{
// unique has no pre constraints
unique27a = handleIsUnique();
// unique has no post constraints
this.__unique27a = unique27a;
if (isMetafacadePropertyCachingEnabled())
{
this.__unique27aSet = true;
}
}
return unique27a;
}
/**
* @see OperationFacade#isLeaf()
* @return boolean
*/
protected abstract boolean handleIsLeaf();
private boolean __leaf28a;
private boolean __leaf28aSet = false;
/**
*
* IsLeaf property in the operation. If true, operation is final,
* cannot be extended or implemented by a descendant.
* Default=false.
*
* @return (boolean)handleIsLeaf()
*/
public final boolean isLeaf()
{
boolean leaf28a = this.__leaf28a;
if (!this.__leaf28aSet)
{
// leaf has no pre constraints
leaf28a = handleIsLeaf();
// leaf has no post constraints
this.__leaf28a = leaf28a;
if (isMetafacadePropertyCachingEnabled())
{
this.__leaf28aSet = true;
}
}
return leaf28a;
}
/**
* @see OperationFacade#getMethodBody()
* @return String
*/
protected abstract String handleGetMethodBody();
private String __methodBody29a;
private boolean __methodBody29aSet = false;
/**
*
* Returns the operation method body determined from UML sequence
* diagrams or other UML sources.
*
* @return (String)handleGetMethodBody()
*/
public final String getMethodBody()
{
String methodBody29a = this.__methodBody29a;
if (!this.__methodBody29aSet)
{
// methodBody has no pre constraints
methodBody29a = handleGetMethodBody();
// methodBody has no post constraints
this.__methodBody29a = methodBody29a;
if (isMetafacadePropertyCachingEnabled())
{
this.__methodBody29aSet = true;
}
}
return methodBody29a;
}
// ---------------- business methods ----------------------
/**
* Method to be implemented in descendants
*
* Searches the given feature for the specified tag.
*
*
* If the follow boolean is set to true then the search will
* continue from the class operation to the class itself and then
* up the class hierarchy.
*
* @param name
* @param follow
* @return Object
*/
protected abstract Object handleFindTaggedValue(String name, boolean follow);
/**
*
* Searches the given feature for the specified tag.
*
*
* If the follow boolean is set to true then the search will
* continue from the class operation to the class itself and then
* up the class hierarchy.
*
* @param name String
*
* The name of the tagged value to find.
*
* @param follow boolean
* @return handleFindTaggedValue(name, follow)
*/
public Object findTaggedValue(String name, boolean follow)
{
// findTaggedValue has no pre constraints
Object returnValue = handleFindTaggedValue(name, follow);
// findTaggedValue has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Returns a comma separated list of exceptions appended to the
* comma separated list of fully qualified 'initialException'
* classes passed in to this method.
*
* @param initialExceptions
* @return String
*/
protected abstract String handleGetExceptionList(String initialExceptions);
/**
*
* Returns a comma separated list of exceptions appended to the
* comma separated list of fully qualified 'initialException'
* classes passed in to this method.
*
* @param initialExceptions String
*
* A comma separated list of fully qualified 'initialException'
* classes passed in to this method.
*
* @return handleGetExceptionList(initialExceptions)
*/
public String getExceptionList(String initialExceptions)
{
// getExceptionList has no pre constraints
String returnValue = handleGetExceptionList(initialExceptions);
// getExceptionList has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Returns the signature of the operation and optionally appends
* the argument names (if withArgumentNames is true), otherwise
* returns the signature with just the types alone in the
* signature.
*
* @param withArgumentNames
* @return String
*/
protected abstract String handleGetSignature(boolean withArgumentNames);
/**
*
* Returns the signature of the operation and optionally appends
* the argument names (if withArgumentNames is true), otherwise
* returns the signature with just the types alone in the
* signature.
*
* @param withArgumentNames boolean
* @return handleGetSignature(withArgumentNames)
*/
public String getSignature(boolean withArgumentNames)
{
// getSignature has no pre constraints
String returnValue = handleGetSignature(withArgumentNames);
// getSignature has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* A comma-separated parameter list (type and name of each
* parameter) of an operation with an optional modifier (i.e final)
* before each parameter.
*
* @param modifier
* @return String
*/
protected abstract String handleGetTypedArgumentList(String modifier);
/**
*
* A comma-separated parameter list (type and name of each
* parameter) of an operation with an optional modifier (i.e final)
* before each parameter.
*
* @param modifier String
*
* The modifier to prefix the arguments with (i.e. 'final')
*
* @return handleGetTypedArgumentList(modifier)
*/
public String getTypedArgumentList(String modifier)
{
// getTypedArgumentList has no pre constraints
String returnValue = handleGetTypedArgumentList(modifier);
// getTypedArgumentList has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Returns the signature of the operation and optionally appends
* the given 'argumentModifier' to each argument.
*
* @param argumentModifier
* @return String
*/
protected abstract String handleGetSignature(String argumentModifier);
/**
*
* Returns the signature of the operation and optionally appends
* the given 'argumentModifier' to each argument.
*
* @param argumentModifier String
*
* The modifier to give the arguments (i.e. 'final').
*
* @return handleGetSignature(argumentModifier)
*/
public String getSignature(String argumentModifier)
{
// getSignature has no pre constraints
String returnValue = handleGetSignature(argumentModifier);
// getSignature has no post constraints
return returnValue;
}
/**
* Method to be implemented in descendants
*
* Finds the parameter on this operation having the given name, if
* no parameter is found, null is returned instead.
*
* @param name
* @return ParameterFacade
*/
protected abstract ParameterFacade handleFindParameter(String name);
/**
*
* Finds the parameter on this operation having the given name, if
* no parameter is found, null is returned instead.
*
* @param name String
*
* The name of the parameter to find on the owner operation.
*
* @return handleFindParameter(name)
*/
public ParameterFacade findParameter(String name)
{
// findParameter has no pre constraints
ParameterFacade returnValue = handleFindParameter(name);
// findParameter has no post constraints
return returnValue;
}
// ------------- associations ------------------
/**
*
* The operations owned by this classifier.
*
* @return (ClassifierFacade)handleGetOwner()
*/
public final ClassifierFacade getOwner()
{
ClassifierFacade getOwner1r = null;
// operations has no pre constraints
Object result = handleGetOwner();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getOwner1r = (ClassifierFacade)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
OperationFacadeLogic.logger.warn("incorrect metafacade cast for OperationFacadeLogic.getOwner ClassifierFacade " + result + ": " + shieldedResult);
}
// operations has no post constraints
return getOwner1r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetOwner();
/**
*
* If this parameter is located on an operation, this will
* represent that operation.
*
* @return (Collection)handleGetParameters()
*/
public final Collection getParameters()
{
Collection getParameters2r = null;
// operation has no pre constraints
Collection result = handleGetParameters();
List shieldedResult = this.shieldedElements(result);
try
{
getParameters2r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
OperationFacadeLogic.logger.warn("incorrect metafacade cast for OperationFacadeLogic.getParameters Collection " + result + ": " + shieldedResult);
}
// operation has no post constraints
return getParameters2r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetParameters();
/**
*
* @return (ClassifierFacade)handleGetReturnType()
*/
public final ClassifierFacade getReturnType()
{
ClassifierFacade getReturnType5r = null;
// operationFacade has no pre constraints
Object result = handleGetReturnType();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getReturnType5r = (ClassifierFacade)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
OperationFacadeLogic.logger.warn("incorrect metafacade cast for OperationFacadeLogic.getReturnType ClassifierFacade " + result + ": " + shieldedResult);
}
// operationFacade has no post constraints
return getReturnType5r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetReturnType();
/**
*
* @return (Collection)handleGetArguments()
*/
public final Collection getArguments()
{
Collection getArguments9r = null;
// operationFacade has no pre constraints
Collection result = handleGetArguments();
List shieldedResult = this.shieldedElements(result);
try
{
getArguments9r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
OperationFacadeLogic.logger.warn("incorrect metafacade cast for OperationFacadeLogic.getArguments Collection " + result + ": " + shieldedResult);
}
// operationFacade has no post constraints
return getArguments9r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetArguments();
/**
*
* @return (Collection)handleGetPreconditions()
*/
public final Collection getPreconditions()
{
Collection getPreconditions10r = null;
// operationFacade has no pre constraints
Collection result = handleGetPreconditions();
List shieldedResult = this.shieldedElements(result);
try
{
getPreconditions10r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
OperationFacadeLogic.logger.warn("incorrect metafacade cast for OperationFacadeLogic.getPreconditions Collection " + result + ": " + shieldedResult);
}
// operationFacade has no post constraints
return getPreconditions10r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetPreconditions();
/**
*
* @return (Collection)handleGetPostconditions()
*/
public final Collection getPostconditions()
{
Collection getPostconditions11r = null;
// operationFacade has no pre constraints
Collection result = handleGetPostconditions();
List shieldedResult = this.shieldedElements(result);
try
{
getPostconditions11r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
OperationFacadeLogic.logger.warn("incorrect metafacade cast for OperationFacadeLogic.getPostconditions Collection " + result + ": " + shieldedResult);
}
// operationFacade has no post constraints
return getPostconditions11r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetPostconditions();
private OperationFacade __getOverriddenOperation16r;
private boolean __getOverriddenOperation16rSet = false;
/**
*
* @return (OperationFacade)handleGetOverriddenOperation()
*/
public final OperationFacade getOverriddenOperation()
{
OperationFacade getOverriddenOperation16r = this.__getOverriddenOperation16r;
if (!this.__getOverriddenOperation16rSet)
{
// operationFacade has no pre constraints
Object result = handleGetOverriddenOperation();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getOverriddenOperation16r = (OperationFacade)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
OperationFacadeLogic.logger.warn("incorrect metafacade cast for OperationFacadeLogic.getOverriddenOperation OperationFacade " + result + ": " + shieldedResult);
}
// operationFacade has no post constraints
this.__getOverriddenOperation16r = getOverriddenOperation16r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getOverriddenOperation16rSet = true;
}
}
return getOverriddenOperation16r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetOverriddenOperation();
/**
* Constraint: org::andromda::metafacades::uml::OperationFacade::operation needs a return type
* Error: Each operation needs a return type, you cannot leave the type unspecified, even if you want void you'll need to explicitly specify it.
* OCL: context OperationFacade
inv: returnType->notEmpty() and returnType.name->notEmpty()
* Constraint: org::andromda::metafacades::uml::OperationFacade::operation must have a name
* Error: Each operation must have a non-empty name.
* OCL: context OperationFacade
inv: name -> notEmpty()
* Constraint: org::andromda::metafacades::uml::OperationFacade::primitive operation return cannot be used in a Collection
* Error: Primitive return parameters cannot be used in Collections (multiplicity > 1). Use the wrapped type or Array type instead.
* OCL: context OperationFacade inv: returnParameter.type.primitive implies (many = false)
* Constraint: org::andromda::metafacades::uml::OperationFacade::wrapped primitive operation return should not be required
* Error: Wrapped primitive operation return must have a multiplicity lower bound = 0 (must be optional). Use the unwrapped type, or change the multiplicity.
* OCL: context OperationFacade inv: returnParameter.type.wrappedPrimitive and many = false implies (lower = 0)
* Constraint: org::andromda::metafacades::uml::OperationFacade::primitive operation return must be required
* Error: Primitive operation return types must have a multiplicity lower bound > 0 (must be required). Use a wrapped type, or change the multiplicity.
* OCL: context OperationFacade inv: returnParameter.type.primitive implies (lower > 0)
* Constraint: org::andromda::metafacades::uml::OperationFacade::operation multiplicity must match return parameter multiplicity
* Error: Operation return parameter with multiplicity greater than 1 must match the operation multiplicity greater than 1.
* OCL: context OperationFacade
inv: many implies (returnParameter.many)
* @param validationMessages Collection
* @see ModelElementFacadeLogicImpl#validateInvariants(Collection validationMessages)
*/
@Override
public void validateInvariants(Collection validationMessages)
{
super.validateInvariants(validationMessages);
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure(OCLCollections.notEmpty(OCLIntrospector.invoke(contextElement,"returnType"))&&OCLCollections.notEmpty(OCLIntrospector.invoke(contextElement,"returnType.name")));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::OperationFacade::operation needs a return type",
"Each operation needs a return type, you cannot leave the type unspecified, even if you want void you'll need to explicitly specify it."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::OperationFacade::operation needs a return type' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure(OCLCollections.notEmpty(OCLIntrospector.invoke(contextElement,"name")));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::OperationFacade::operation must have a name",
"Each operation must have a non-empty name."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::OperationFacade::operation must have a name' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure((Boolean.valueOf(String.valueOf(OCLIntrospector.invoke(contextElement,"returnParameter.type.primitive"))).booleanValue()?(OCLExpressions.equal(OCLIntrospector.invoke(contextElement,"many"),false)):true));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::OperationFacade::primitive operation return cannot be used in a Collection",
"Primitive return parameters cannot be used in Collections (multiplicity > 1). Use the wrapped type or Array type instead."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::OperationFacade::primitive operation return cannot be used in a Collection' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure((Boolean.valueOf(String.valueOf(Boolean.valueOf(String.valueOf(OCLIntrospector.invoke(contextElement,"returnParameter.type.wrappedPrimitive"))).booleanValue()&&OCLExpressions.equal(OCLIntrospector.invoke(contextElement,"many"),false))).booleanValue()?(OCLExpressions.equal(OCLIntrospector.invoke(contextElement,"lower"),0)):true));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::OperationFacade::wrapped primitive operation return should not be required",
"Wrapped primitive operation return must have a multiplicity lower bound = 0 (must be optional). Use the unwrapped type, or change the multiplicity."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::OperationFacade::wrapped primitive operation return should not be required' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure((Boolean.valueOf(String.valueOf(OCLIntrospector.invoke(contextElement,"returnParameter.type.primitive"))).booleanValue()?(OCLExpressions.greater(OCLIntrospector.invoke(contextElement,"lower"),0)):true));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::OperationFacade::primitive operation return must be required",
"Primitive operation return types must have a multiplicity lower bound > 0 (must be required). Use a wrapped type, or change the multiplicity."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::OperationFacade::primitive operation return must be required' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
try
{
final Object contextElement = this.THIS();
boolean constraintValid = OCLResultEnsurer.ensure((Boolean.valueOf(String.valueOf(OCLIntrospector.invoke(contextElement,"many"))).booleanValue()?(OCLIntrospector.invoke(contextElement,"returnParameter.many")):true));
if (!constraintValid)
{
validationMessages.add(
new ModelValidationMessage(
(MetafacadeBase)contextElement ,
"org::andromda::metafacades::uml::OperationFacade::operation multiplicity must match return parameter multiplicity",
"Operation return parameter with multiplicity greater than 1 must match the operation multiplicity greater than 1."));
}
}
catch (Throwable th)
{
Throwable cause = th.getCause();
int depth = 0; // Some throwables have infinite recursion
while (cause != null && depth < 7)
{
th = cause;
depth++;
}
logger.error("Error validating constraint 'org::andromda::metafacades::uml::OperationFacade::operation multiplicity must match return parameter multiplicity' ON "
+ this.THIS().toString() + ": " + th.getMessage(), th);
}
}
}