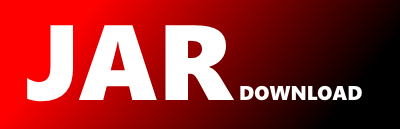
org.andromda.metafacades.uml14.PseudostateFacadeLogic Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of andromda-metafacades-uml14 Show documentation
Show all versions of andromda-metafacades-uml14 Show documentation
The UML 1.4 metafacades. This is the set of UML 1.4 metafacades
implementations. These implement the common UML metafacades.
The newest version!
// license-header java merge-point
//
// Attention: generated code (by MetafacadeLogic.vsl) - do not modify!
//
package org.andromda.metafacades.uml14;
import java.util.Collection;
import org.andromda.core.metafacade.ModelValidationMessage;
import org.andromda.metafacades.uml.PseudostateFacade;
import org.omg.uml.behavioralelements.statemachines.Pseudostate;
/**
*
* An abstraction that encompasses different types of transient
* vertices in the state machine graph.
*
* MetafacadeLogic for PseudostateFacade
*
* @see PseudostateFacade
*/
public abstract class PseudostateFacadeLogic
extends StateVertexFacadeLogicImpl
implements PseudostateFacade
{
/**
* The underlying UML object
* @see Pseudostate
*/
protected Pseudostate metaObject;
/** Create Metafacade implementation instance using the MetafacadeFactory from the context
* @param metaObjectIn
* @param context
*/
protected PseudostateFacadeLogic(Pseudostate metaObjectIn, String context)
{
super(metaObjectIn, getContext(context));
this.metaObject = metaObjectIn;
}
/**
* Gets the context for this metafacade logic instance.
* @param context String. Set to PseudostateFacade if null
* @return context String
*/
private static String getContext(String context)
{
if (context == null)
{
context = "org.andromda.metafacades.uml.PseudostateFacade";
}
return context;
}
/** Reset context only for non-root metafacades
* @param context
*/
@Override
public void resetMetafacadeContext(String context)
{
if (!this.contextRoot) // reset context only for non-root metafacades
{
context = getContext(context); // to have same value as in original constructor call
setMetafacadeContext (context);
}
}
/**
* @return boolean true always
* @see PseudostateFacade
*/
public boolean isPseudostateFacadeMetaType()
{
return true;
}
// --------------- attributes ---------------------
/**
* @see PseudostateFacade#isChoice()
* @return boolean
*/
protected abstract boolean handleIsChoice();
private boolean __choice1a;
private boolean __choice1aSet = false;
/**
*
* @return (boolean)handleIsChoice()
*/
public final boolean isChoice()
{
boolean choice1a = this.__choice1a;
if (!this.__choice1aSet)
{
// choice has no pre constraints
choice1a = handleIsChoice();
// choice has no post constraints
this.__choice1a = choice1a;
if (isMetafacadePropertyCachingEnabled())
{
this.__choice1aSet = true;
}
}
return choice1a;
}
/**
* @see PseudostateFacade#isDecisionPoint()
* @return boolean
*/
protected abstract boolean handleIsDecisionPoint();
private boolean __decisionPoint2a;
private boolean __decisionPoint2aSet = false;
/**
*
* @return (boolean)handleIsDecisionPoint()
*/
public final boolean isDecisionPoint()
{
boolean decisionPoint2a = this.__decisionPoint2a;
if (!this.__decisionPoint2aSet)
{
// decisionPoint has no pre constraints
decisionPoint2a = handleIsDecisionPoint();
// decisionPoint has no post constraints
this.__decisionPoint2a = decisionPoint2a;
if (isMetafacadePropertyCachingEnabled())
{
this.__decisionPoint2aSet = true;
}
}
return decisionPoint2a;
}
/**
* @see PseudostateFacade#isDeepHistory()
* @return boolean
*/
protected abstract boolean handleIsDeepHistory();
private boolean __deepHistory3a;
private boolean __deepHistory3aSet = false;
/**
*
* @return (boolean)handleIsDeepHistory()
*/
public final boolean isDeepHistory()
{
boolean deepHistory3a = this.__deepHistory3a;
if (!this.__deepHistory3aSet)
{
// deepHistory has no pre constraints
deepHistory3a = handleIsDeepHistory();
// deepHistory has no post constraints
this.__deepHistory3a = deepHistory3a;
if (isMetafacadePropertyCachingEnabled())
{
this.__deepHistory3aSet = true;
}
}
return deepHistory3a;
}
/**
* @see PseudostateFacade#isFork()
* @return boolean
*/
protected abstract boolean handleIsFork();
private boolean __fork4a;
private boolean __fork4aSet = false;
/**
*
* @return (boolean)handleIsFork()
*/
public final boolean isFork()
{
boolean fork4a = this.__fork4a;
if (!this.__fork4aSet)
{
// fork has no pre constraints
fork4a = handleIsFork();
// fork has no post constraints
this.__fork4a = fork4a;
if (isMetafacadePropertyCachingEnabled())
{
this.__fork4aSet = true;
}
}
return fork4a;
}
/**
* @see PseudostateFacade#isInitialState()
* @return boolean
*/
protected abstract boolean handleIsInitialState();
private boolean __initialState5a;
private boolean __initialState5aSet = false;
/**
*
* @return (boolean)handleIsInitialState()
*/
public final boolean isInitialState()
{
boolean initialState5a = this.__initialState5a;
if (!this.__initialState5aSet)
{
// initialState has no pre constraints
initialState5a = handleIsInitialState();
// initialState has no post constraints
this.__initialState5a = initialState5a;
if (isMetafacadePropertyCachingEnabled())
{
this.__initialState5aSet = true;
}
}
return initialState5a;
}
/**
* @see PseudostateFacade#isJoin()
* @return boolean
*/
protected abstract boolean handleIsJoin();
private boolean __join6a;
private boolean __join6aSet = false;
/**
*
* @return (boolean)handleIsJoin()
*/
public final boolean isJoin()
{
boolean join6a = this.__join6a;
if (!this.__join6aSet)
{
// join has no pre constraints
join6a = handleIsJoin();
// join has no post constraints
this.__join6a = join6a;
if (isMetafacadePropertyCachingEnabled())
{
this.__join6aSet = true;
}
}
return join6a;
}
/**
* @see PseudostateFacade#isJunction()
* @return boolean
*/
protected abstract boolean handleIsJunction();
private boolean __junction7a;
private boolean __junction7aSet = false;
/**
*
* @return (boolean)handleIsJunction()
*/
public final boolean isJunction()
{
boolean junction7a = this.__junction7a;
if (!this.__junction7aSet)
{
// junction has no pre constraints
junction7a = handleIsJunction();
// junction has no post constraints
this.__junction7a = junction7a;
if (isMetafacadePropertyCachingEnabled())
{
this.__junction7aSet = true;
}
}
return junction7a;
}
/**
* @see PseudostateFacade#isMergePoint()
* @return boolean
*/
protected abstract boolean handleIsMergePoint();
private boolean __mergePoint8a;
private boolean __mergePoint8aSet = false;
/**
*
* @return (boolean)handleIsMergePoint()
*/
public final boolean isMergePoint()
{
boolean mergePoint8a = this.__mergePoint8a;
if (!this.__mergePoint8aSet)
{
// mergePoint has no pre constraints
mergePoint8a = handleIsMergePoint();
// mergePoint has no post constraints
this.__mergePoint8a = mergePoint8a;
if (isMetafacadePropertyCachingEnabled())
{
this.__mergePoint8aSet = true;
}
}
return mergePoint8a;
}
/**
* @see PseudostateFacade#isShallowHistory()
* @return boolean
*/
protected abstract boolean handleIsShallowHistory();
private boolean __shallowHistory9a;
private boolean __shallowHistory9aSet = false;
/**
*
* @return (boolean)handleIsShallowHistory()
*/
public final boolean isShallowHistory()
{
boolean shallowHistory9a = this.__shallowHistory9a;
if (!this.__shallowHistory9aSet)
{
// shallowHistory has no pre constraints
shallowHistory9a = handleIsShallowHistory();
// shallowHistory has no post constraints
this.__shallowHistory9a = shallowHistory9a;
if (isMetafacadePropertyCachingEnabled())
{
this.__shallowHistory9aSet = true;
}
}
return shallowHistory9a;
}
/**
* @see PseudostateFacade#isSplit()
* @return boolean
*/
protected abstract boolean handleIsSplit();
private boolean __split10a;
private boolean __split10aSet = false;
/**
*
* Denotes this pseudostate to be either a join or a fork with a
* single incoming transition and more than one outgoing
* transition.
*
* @return (boolean)handleIsSplit()
*/
public final boolean isSplit()
{
boolean split10a = this.__split10a;
if (!this.__split10aSet)
{
// split has no pre constraints
split10a = handleIsSplit();
// split has no post constraints
this.__split10a = split10a;
if (isMetafacadePropertyCachingEnabled())
{
this.__split10aSet = true;
}
}
return split10a;
}
/**
* @see PseudostateFacade#isCollect()
* @return boolean
*/
protected abstract boolean handleIsCollect();
private boolean __collect11a;
private boolean __collect11aSet = false;
/**
*
* Denotes this pseudostate to be either a join or a fork with a
* single outgoing transition and more than one incoming
* transition.
*
* @return (boolean)handleIsCollect()
*/
public final boolean isCollect()
{
boolean collect11a = this.__collect11a;
if (!this.__collect11aSet)
{
// collect has no pre constraints
collect11a = handleIsCollect();
// collect has no post constraints
this.__collect11a = collect11a;
if (isMetafacadePropertyCachingEnabled())
{
this.__collect11aSet = true;
}
}
return collect11a;
}
// ------------- associations ------------------
/**
* @param validationMessages Collection
* @see StateVertexFacadeLogicImpl#validateInvariants(Collection validationMessages)
*/
@Override
public void validateInvariants(Collection validationMessages)
{
super.validateInvariants(validationMessages);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy