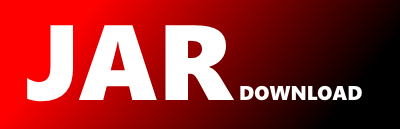
org.andromda.metafacades.uml14.ServiceOperationLogic Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of andromda-metafacades-uml14 Show documentation
Show all versions of andromda-metafacades-uml14 Show documentation
The UML 1.4 metafacades. This is the set of UML 1.4 metafacades
implementations. These implement the common UML metafacades.
The newest version!
// license-header java merge-point
//
// Attention: generated code (by MetafacadeLogic.vsl) - do not modify!
//
package org.andromda.metafacades.uml14;
import java.util.Collection;
import java.util.List;
import org.andromda.core.metafacade.MetafacadeBase;
import org.andromda.core.metafacade.ModelValidationMessage;
import org.andromda.metafacades.uml.Destination;
import org.andromda.metafacades.uml.Role;
import org.andromda.metafacades.uml.Service;
import org.andromda.metafacades.uml.ServiceOperation;
import org.apache.log4j.Logger;
import org.omg.uml.foundation.core.Operation;
/**
*
* Reprsents an operation of a service.
*
* MetafacadeLogic for ServiceOperation
*
* @see ServiceOperation
*/
public abstract class ServiceOperationLogic
extends OperationFacadeLogicImpl
implements ServiceOperation
{
/**
* The underlying UML object
* @see Object
*/
protected Object metaObject;
/** Create Metafacade implementation instance using the MetafacadeFactory from the context
* @param metaObjectIn
* @param context
*/
protected ServiceOperationLogic(Object metaObjectIn, String context)
{
super((Operation)metaObjectIn, getContext(context));
this.metaObject = metaObjectIn;
}
/**
* The logger instance.
*/
private static final Logger logger = Logger.getLogger(ServiceOperationLogic.class);
/**
* Gets the context for this metafacade logic instance.
* @param context String. Set to ServiceOperation if null
* @return context String
*/
private static String getContext(String context)
{
if (context == null)
{
context = "org.andromda.metafacades.uml.ServiceOperation";
}
return context;
}
/** Reset context only for non-root metafacades
* @param context
*/
@Override
public void resetMetafacadeContext(String context)
{
if (!this.contextRoot) // reset context only for non-root metafacades
{
context = getContext(context); // to have same value as in original constructor call
setMetafacadeContext (context);
}
}
/**
* @return boolean true always
* @see ServiceOperation
*/
public boolean isServiceOperationMetaType()
{
return true;
}
// --------------- attributes ---------------------
/**
* @see ServiceOperation#isIncomingMessageOperation()
* @return boolean
*/
protected abstract boolean handleIsIncomingMessageOperation();
private boolean __incomingMessageOperation1a;
private boolean __incomingMessageOperation1aSet = false;
/**
*
* Whether or not this operation represents an "incoming" message
* operation (i.e. it receives messages from Queues or Topics).
*
* @return (boolean)handleIsIncomingMessageOperation()
*/
public final boolean isIncomingMessageOperation()
{
boolean incomingMessageOperation1a = this.__incomingMessageOperation1a;
if (!this.__incomingMessageOperation1aSet)
{
// incomingMessageOperation has no pre constraints
incomingMessageOperation1a = handleIsIncomingMessageOperation();
// incomingMessageOperation has no post constraints
this.__incomingMessageOperation1a = incomingMessageOperation1a;
if (isMetafacadePropertyCachingEnabled())
{
this.__incomingMessageOperation1aSet = true;
}
}
return incomingMessageOperation1a;
}
/**
* @see ServiceOperation#isOutgoingMessageOperation()
* @return boolean
*/
protected abstract boolean handleIsOutgoingMessageOperation();
private boolean __outgoingMessageOperation2a;
private boolean __outgoingMessageOperation2aSet = false;
/**
*
* Whether or not this service operation represents an "outgoing"
* messaging operation (i.e. it sends messages to Queues or
* Topics).
*
* @return (boolean)handleIsOutgoingMessageOperation()
*/
public final boolean isOutgoingMessageOperation()
{
boolean outgoingMessageOperation2a = this.__outgoingMessageOperation2a;
if (!this.__outgoingMessageOperation2aSet)
{
// outgoingMessageOperation has no pre constraints
outgoingMessageOperation2a = handleIsOutgoingMessageOperation();
// outgoingMessageOperation has no post constraints
this.__outgoingMessageOperation2a = outgoingMessageOperation2a;
if (isMetafacadePropertyCachingEnabled())
{
this.__outgoingMessageOperation2aSet = true;
}
}
return outgoingMessageOperation2a;
}
/**
* @see ServiceOperation#isMessageOperation()
* @return boolean
*/
protected abstract boolean handleIsMessageOperation();
private boolean __messageOperation3a;
private boolean __messageOperation3aSet = false;
/**
*
* Whether or not this is operation accepts incoming or outgoing
* messages.
*
* @return (boolean)handleIsMessageOperation()
*/
public final boolean isMessageOperation()
{
boolean messageOperation3a = this.__messageOperation3a;
if (!this.__messageOperation3aSet)
{
// messageOperation has no pre constraints
messageOperation3a = handleIsMessageOperation();
// messageOperation has no post constraints
this.__messageOperation3a = messageOperation3a;
if (isMetafacadePropertyCachingEnabled())
{
this.__messageOperation3aSet = true;
}
}
return messageOperation3a;
}
// ------------- associations ------------------
/**
*
* @return (Collection)handleGetRoles()
*/
public final Collection getRoles()
{
Collection getRoles1r = null;
// serviceOperation has no pre constraints
Collection result = handleGetRoles();
List shieldedResult = this.shieldedElements(result);
try
{
getRoles1r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
ServiceOperationLogic.logger.warn("incorrect metafacade cast for ServiceOperationLogic.getRoles Collection " + result + ": " + shieldedResult);
}
// serviceOperation has no post constraints
return getRoles1r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetRoles();
/**
*
* @return (Service)handleGetService()
*/
public final Service getService()
{
Service getService2r = null;
// serviceOperation has no pre constraints
Object result = handleGetService();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getService2r = (Service)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
ServiceOperationLogic.logger.warn("incorrect metafacade cast for ServiceOperationLogic.getService Service " + result + ": " + shieldedResult);
}
// serviceOperation has no post constraints
return getService2r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetService();
/**
*
* @return (Destination)handleGetOutgoingDestination()
*/
public final Destination getOutgoingDestination()
{
Destination getOutgoingDestination3r = null;
// serviceOperation has no pre constraints
Object result = handleGetOutgoingDestination();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getOutgoingDestination3r = (Destination)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
ServiceOperationLogic.logger.warn("incorrect metafacade cast for ServiceOperationLogic.getOutgoingDestination Destination " + result + ": " + shieldedResult);
}
// serviceOperation has no post constraints
return getOutgoingDestination3r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetOutgoingDestination();
/**
*
* @return (Destination)handleGetIncomingDestination()
*/
public final Destination getIncomingDestination()
{
Destination getIncomingDestination4r = null;
// serviceOperation has no pre constraints
Object result = handleGetIncomingDestination();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getIncomingDestination4r = (Destination)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
ServiceOperationLogic.logger.warn("incorrect metafacade cast for ServiceOperationLogic.getIncomingDestination Destination " + result + ": " + shieldedResult);
}
// serviceOperation has no post constraints
return getIncomingDestination4r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetIncomingDestination();
/**
* @param validationMessages Collection
* @see OperationFacadeLogicImpl#validateInvariants(Collection validationMessages)
*/
@Override
public void validateInvariants(Collection validationMessages)
{
super.validateInvariants(validationMessages);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy