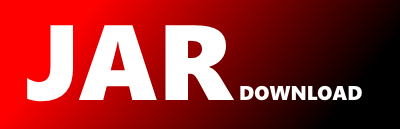
org.andromda.metafacades.uml14.StateFacadeLogic Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of andromda-metafacades-uml14 Show documentation
Show all versions of andromda-metafacades-uml14 Show documentation
The UML 1.4 metafacades. This is the set of UML 1.4 metafacades
implementations. These implement the common UML metafacades.
The newest version!
// license-header java merge-point
//
// Attention: generated code (by MetafacadeLogic.vsl) - do not modify!
//
package org.andromda.metafacades.uml14;
import java.util.Collection;
import java.util.List;
import org.andromda.core.metafacade.ModelValidationMessage;
import org.andromda.metafacades.uml.EventFacade;
import org.andromda.metafacades.uml.StateFacade;
import org.apache.log4j.Logger;
import org.omg.uml.behavioralelements.statemachines.State;
/**
*
* Models a situation during which some (usually implicit)
* invariant condition holds. The states of protocol state machines
* are exposed to the users of their context classifiers. A
* protocol state represents an exposed stable situation of its
* context classifier: when an instance of the classifier is not
* processing any operation, users of this instance can always know
* its state configuration.
*
* MetafacadeLogic for StateFacade
*
* @see StateFacade
*/
public abstract class StateFacadeLogic
extends StateVertexFacadeLogicImpl
implements StateFacade
{
/**
* The underlying UML object
* @see State
*/
protected State metaObject;
/** Create Metafacade implementation instance using the MetafacadeFactory from the context
* @param metaObjectIn
* @param context
*/
protected StateFacadeLogic(State metaObjectIn, String context)
{
super(metaObjectIn, getContext(context));
this.metaObject = metaObjectIn;
}
/**
* The logger instance.
*/
private static final Logger logger = Logger.getLogger(StateFacadeLogic.class);
/**
* Gets the context for this metafacade logic instance.
* @param context String. Set to StateFacade if null
* @return context String
*/
private static String getContext(String context)
{
if (context == null)
{
context = "org.andromda.metafacades.uml.StateFacade";
}
return context;
}
/** Reset context only for non-root metafacades
* @param context
*/
@Override
public void resetMetafacadeContext(String context)
{
if (!this.contextRoot) // reset context only for non-root metafacades
{
context = getContext(context); // to have same value as in original constructor call
setMetafacadeContext (context);
}
}
/**
* @return boolean true always
* @see StateFacade
*/
public boolean isStateFacadeMetaType()
{
return true;
}
// ------------- associations ------------------
/**
*
* If this event is located on an action state, this will represent
* that state.
*
* @return (Collection)handleGetDeferrableEvents()
*/
public final Collection getDeferrableEvents()
{
Collection getDeferrableEvents1r = null;
// state has no pre constraints
Collection result = handleGetDeferrableEvents();
List shieldedResult = this.shieldedElements(result);
try
{
getDeferrableEvents1r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
StateFacadeLogic.logger.warn("incorrect metafacade cast for StateFacadeLogic.getDeferrableEvents Collection " + result + ": " + shieldedResult);
}
// state has no post constraints
return getDeferrableEvents1r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetDeferrableEvents();
/**
* @param validationMessages Collection
* @see StateVertexFacadeLogicImpl#validateInvariants(Collection validationMessages)
*/
@Override
public void validateInvariants(Collection validationMessages)
{
super.validateInvariants(validationMessages);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy