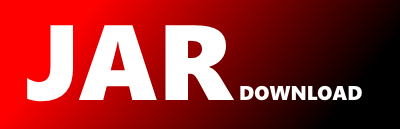
org.andromda.metafacades.uml14.StateVertexFacadeLogic Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of andromda-metafacades-uml14 Show documentation
Show all versions of andromda-metafacades-uml14 Show documentation
The UML 1.4 metafacades. This is the set of UML 1.4 metafacades
implementations. These implement the common UML metafacades.
The newest version!
// license-header java merge-point
//
// Attention: generated code (by MetafacadeLogic.vsl) - do not modify!
//
package org.andromda.metafacades.uml14;
import java.util.Collection;
import java.util.List;
import org.andromda.core.metafacade.MetafacadeBase;
import org.andromda.core.metafacade.ModelValidationMessage;
import org.andromda.metafacades.uml.PartitionFacade;
import org.andromda.metafacades.uml.StateFacade;
import org.andromda.metafacades.uml.StateMachineFacade;
import org.andromda.metafacades.uml.StateVertexFacade;
import org.andromda.metafacades.uml.TransitionFacade;
import org.apache.log4j.Logger;
import org.omg.uml.behavioralelements.statemachines.StateVertex;
/**
*
* A representation of the model object 'Vertex'. An abstraction of
* a node in a state machine graph. In general, it can be the
* source or destination of any number of transitions.
*
* MetafacadeLogic for StateVertexFacade
*
* @see StateVertexFacade
*/
public abstract class StateVertexFacadeLogic
extends ModelElementFacadeLogicImpl
implements StateVertexFacade
{
/**
* The underlying UML object
* @see StateVertex
*/
protected StateVertex metaObject;
/** Create Metafacade implementation instance using the MetafacadeFactory from the context
* @param metaObjectIn
* @param context
*/
protected StateVertexFacadeLogic(StateVertex metaObjectIn, String context)
{
super(metaObjectIn, getContext(context));
this.metaObject = metaObjectIn;
}
/**
* The logger instance.
*/
private static final Logger logger = Logger.getLogger(StateVertexFacadeLogic.class);
/**
* Gets the context for this metafacade logic instance.
* @param context String. Set to StateVertexFacade if null
* @return context String
*/
private static String getContext(String context)
{
if (context == null)
{
context = "org.andromda.metafacades.uml.StateVertexFacade";
}
return context;
}
/** Reset context only for non-root metafacades
* @param context
*/
@Override
public void resetMetafacadeContext(String context)
{
if (!this.contextRoot) // reset context only for non-root metafacades
{
context = getContext(context); // to have same value as in original constructor call
setMetafacadeContext (context);
}
}
/**
* @return boolean true always
* @see StateVertexFacade
*/
public boolean isStateVertexFacadeMetaType()
{
return true;
}
// ------------- associations ------------------
/**
*
* @return (Collection)handleGetOutgoings()
*/
public final Collection getOutgoings()
{
Collection getOutgoings1r = null;
// target has no pre constraints
Collection result = handleGetOutgoings();
List shieldedResult = this.shieldedElements(result);
try
{
getOutgoings1r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
StateVertexFacadeLogic.logger.warn("incorrect metafacade cast for StateVertexFacadeLogic.getOutgoings Collection " + result + ": " + shieldedResult);
}
// target has no post constraints
return getOutgoings1r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetOutgoings();
/**
*
* @return (Collection)handleGetIncomings()
*/
public final Collection getIncomings()
{
Collection getIncomings2r = null;
// source has no pre constraints
Collection result = handleGetIncomings();
List shieldedResult = this.shieldedElements(result);
try
{
getIncomings2r = (Collection)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
StateVertexFacadeLogic.logger.warn("incorrect metafacade cast for StateVertexFacadeLogic.getIncomings Collection " + result + ": " + shieldedResult);
}
// source has no post constraints
return getIncomings2r;
}
/**
* UML Specific type is returned in Collection, transformed by shieldedElements to AndroMDA Metafacade type
* @return Collection
*/
protected abstract Collection handleGetIncomings();
/**
*
* @return (StateFacade)handleGetContainer()
*/
public final StateFacade getContainer()
{
StateFacade getContainer3r = null;
// stateVertexFacade has no pre constraints
Object result = handleGetContainer();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getContainer3r = (StateFacade)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
StateVertexFacadeLogic.logger.warn("incorrect metafacade cast for StateVertexFacadeLogic.getContainer StateFacade " + result + ": " + shieldedResult);
}
// stateVertexFacade has no post constraints
return getContainer3r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetContainer();
private PartitionFacade __getPartition4r;
private boolean __getPartition4rSet = false;
/**
*
* All vertices enveloped by this partition.
*
* @return (PartitionFacade)handleGetPartition()
*/
public final PartitionFacade getPartition()
{
PartitionFacade getPartition4r = this.__getPartition4r;
if (!this.__getPartition4rSet)
{
// vertices has no pre constraints
Object result = handleGetPartition();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getPartition4r = (PartitionFacade)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
StateVertexFacadeLogic.logger.warn("incorrect metafacade cast for StateVertexFacadeLogic.getPartition PartitionFacade " + result + ": " + shieldedResult);
}
// vertices has no post constraints
this.__getPartition4r = getPartition4r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getPartition4rSet = true;
}
}
return getPartition4r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetPartition();
private StateMachineFacade __getStateMachine5r;
private boolean __getStateMachine5rSet = false;
/**
*
* @return (StateMachineFacade)handleGetStateMachine()
*/
public final StateMachineFacade getStateMachine()
{
StateMachineFacade getStateMachine5r = this.__getStateMachine5r;
if (!this.__getStateMachine5rSet)
{
// stateVertexFacade has no pre constraints
Object result = handleGetStateMachine();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getStateMachine5r = (StateMachineFacade)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
StateVertexFacadeLogic.logger.warn("incorrect metafacade cast for StateVertexFacadeLogic.getStateMachine StateMachineFacade " + result + ": " + shieldedResult);
}
// stateVertexFacade has no post constraints
this.__getStateMachine5r = getStateMachine5r;
if (isMetafacadePropertyCachingEnabled())
{
this.__getStateMachine5rSet = true;
}
}
return getStateMachine5r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetStateMachine();
/**
* @param validationMessages Collection
* @see ModelElementFacadeLogicImpl#validateInvariants(Collection validationMessages)
*/
@Override
public void validateInvariants(Collection validationMessages)
{
super.validateInvariants(validationMessages);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy