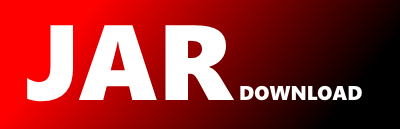
org.andromda.metafacades.uml14.TemplateArgumentFacadeLogic Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of andromda-metafacades-uml14 Show documentation
Show all versions of andromda-metafacades-uml14 Show documentation
The UML 1.4 metafacades. This is the set of UML 1.4 metafacades
implementations. These implement the common UML metafacades.
The newest version!
// license-header java merge-point
//
// Attention: generated code (by MetafacadeLogic.vsl) - do not modify!
//
package org.andromda.metafacades.uml14;
import java.util.Collection;
import org.andromda.core.common.Introspector;
import org.andromda.core.metafacade.MetafacadeBase;
import org.andromda.core.metafacade.ModelValidationMessage;
import org.andromda.metafacades.uml.ModelElementFacade;
import org.andromda.metafacades.uml.TemplateArgumentFacade;
import org.apache.log4j.Logger;
import org.omg.uml.foundation.core.TemplateArgument;
/**
*
* Represents the model object 'Template Parameter Substitution'.
* Relates the actual parameter(s) to a formal template parameter
* as part of a template binding.
*
* MetafacadeLogic for TemplateArgumentFacade
*
* @see TemplateArgumentFacade
*/
public abstract class TemplateArgumentFacadeLogic
extends MetafacadeBase
implements TemplateArgumentFacade
{
/**
* The underlying UML object
* @see TemplateArgument
*/
protected TemplateArgument metaObject;
/** Create Metafacade implementation instance using the MetafacadeFactory from the context
* @param metaObjectIn
* @param context
*/
protected TemplateArgumentFacadeLogic(TemplateArgument metaObjectIn, String context)
{
super(metaObjectIn, getContext(context));
this.metaObject = metaObjectIn;
}
/**
* The logger instance.
*/
private static final Logger logger = Logger.getLogger(TemplateArgumentFacadeLogic.class);
/**
* Gets the context for this metafacade logic instance.
* @param context String. Set to TemplateArgumentFacade if null
* @return context String
*/
private static String getContext(String context)
{
if (context == null)
{
context = "org.andromda.metafacades.uml.TemplateArgumentFacade";
}
return context;
}
/** Reset context only for non-root metafacades
* @param context
*/
@Override
public void resetMetafacadeContext(String context)
{
if (!this.contextRoot) // reset context only for non-root metafacades
{
context = getContext(context); // to have same value as in original constructor call
setMetafacadeContext (context);
}
}
/**
* @return boolean true always
* @see TemplateArgumentFacade
*/
public boolean isTemplateArgumentFacadeMetaType()
{
return true;
}
// ------------- associations ------------------
/**
*
* @return (ModelElementFacade)handleGetElement()
*/
public final ModelElementFacade getElement()
{
ModelElementFacade getElement2r = null;
// templateArgumentFacade has no pre constraints
Object result = handleGetElement();
MetafacadeBase shieldedResult = this.shieldedElement(result);
try
{
getElement2r = (ModelElementFacade)shieldedResult;
}
catch (ClassCastException ex)
{
// Bad things happen if the metafacade type mapping in metafacades.xml is wrong - Warn
TemplateArgumentFacadeLogic.logger.warn("incorrect metafacade cast for TemplateArgumentFacadeLogic.getElement ModelElementFacade " + result + ": " + shieldedResult);
}
// templateArgumentFacade has no post constraints
return getElement2r;
}
/**
* UML Specific type is transformed by shieldedElements to AndroMDA Metafacade type
* @return Object
*/
protected abstract Object handleGetElement();
/**
* @param validationMessages Collection
* @see MetafacadeBase#validateInvariants(Collection validationMessages)
*/
@Override
public void validateInvariants(Collection validationMessages)
{
}
/**
* The property that stores the name of the metafacade.
*/
private static final String NAME_PROPERTY = "name";
private static final String FQNAME_PROPERTY = "fullyQualifiedName";
/**
* @see Object#toString()
*/
@Override
public String toString()
{
final StringBuilder toString = new StringBuilder(this.getClass().getName());
toString.append("[");
try
{
toString.append(Introspector.instance().getProperty(this, FQNAME_PROPERTY));
}
catch (final Throwable tryAgain)
{
try
{
toString.append(Introspector.instance().getProperty(this, NAME_PROPERTY));
}
catch (final Throwable ignore)
{
// - just ignore when the metafacade doesn't have a name or fullyQualifiedName property
}
}
toString.append("]");
return toString.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy