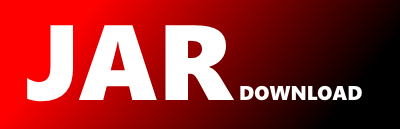
org.anvilpowered.anvil.api.misc.BindingExtensions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of anvil-api Show documentation
Show all versions of anvil-api Show documentation
A cross-platform database API / ORM / entity framework with useful services for minecraft plugins
The newest version!
/*
* Anvil - AnvilPowered
* Copyright (C) 2020
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*/
package org.anvilpowered.anvil.api.misc;
import com.google.common.reflect.TypeToken;
import com.google.inject.Key;
import com.google.inject.Provider;
import com.google.inject.TypeLiteral;
import org.anvilpowered.anvil.api.Anvil;
import org.anvilpowered.anvil.api.datastore.Component;
import org.anvilpowered.anvil.api.datastore.DataStoreContext;
import java.lang.annotation.Annotation;
@SuppressWarnings({"unchecked", "UnstableApiUsage"})
public interface BindingExtensions {
/**
* Full binding method for a component
*
* A typical example of usage of this method:
*
* {@code
* be.bind(
* new TypeToken>(getClass()) {
* },
* new TypeToken>(getClass()) {
* },
* new TypeToken, Datastore>>(getClass()) {
* },
* new TypeToken>(getClass()) { // final implementation
* },
* Names.named("mongodb")
* );
* }
*/
,
From2 extends Component, ?>,
From3 extends From1,
Target extends From1>
void bind(
TypeToken from1,
TypeToken from2,
TypeToken from3,
TypeToken target,
Annotation componentAnnotation
);
/**
* Binding method for a component
*
* A typical example of usage of this method:
*
* {@code
* be.bind(
* new TypeToken>(getClass()) {
* },
* new TypeToken>(getClass()) {
* },
* new TypeToken(getClass()) { // final implementation
* },
* Names.named("mongodb")
* );
* }
*/
,
From2 extends From1,
Target extends From1>
void bind(
TypeToken from1,
TypeToken from2,
TypeToken target,
Annotation componentAnnotation
);
void bind(
TypeToken from,
TypeToken target,
Annotation annotation
);
void bind(
TypeToken from,
TypeToken target,
Class extends Annotation> annotation
);
void bind(
TypeToken from,
TypeToken target
);
/**
* Binds the mongodb {@link DataStoreContext}
*
* Using this method is the same as invoking:
*
* {@code
* bind(new TypeLiteral>() {
* }).to(MongoContext.class);
* }
*/
void withMongoDB();
/**
* Binds the xodus {@link DataStoreContext}
*
* Using this method is the same as invoking:
*
* {@code
* binder.bind(new TypeLiteral>() {
* }).to(XodusContext.class);
* }
*/
void withXodus();
static TypeLiteral getTypeLiteral(TypeToken typeToken) {
return (TypeLiteral) TypeLiteral.get(typeToken.getType());
}
static Key getKey(TypeToken typeToken) {
return Key.get(getTypeLiteral(typeToken));
}
static Provider asInternalProvider(Class clazz) {
return () -> Anvil.getEnvironment().getInjector()
.getInstance(clazz);
}
static Provider asInternalProvider(TypeLiteral typeLiteral) {
return () -> Anvil.getEnvironment().getInjector()
.getInstance(Key.get(typeLiteral));
}
static Provider asInternalProvider(TypeToken typeToken) {
return () -> Anvil.getEnvironment().getInjector()
.getInstance(BindingExtensions.getKey(typeToken));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy