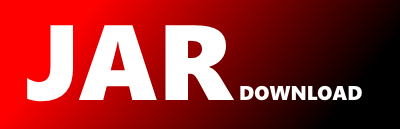
org.anyline.seo.util.BingSeoClient Maven / Gradle / Ivy
/*
* Copyright 2006-2023 www.anyline.org
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.anyline.seo.util;
import org.anyline.net.HttpResponse;
import org.anyline.net.HttpUtil;
import org.anyline.util.AnylineConfig;
import org.anyline.util.BasicUtil;
import org.apache.http.entity.StringEntity;
import org.anyline.log.Log;
import org.anyline.log.LogProxy;
import java.util.*;
public class BingSeoClient {
private static Log log = LogProxy.get(BingSeoClient.class);
public BingSeoConfig config = null;
private static Hashtable instances = new Hashtable<>();
static {
Hashtable configs = BingSeoConfig.getInstances();
for(String key:configs.keySet()){
instances.put(key, getInstance(key));
}
}
public static Hashtable getInstances(){
return instances;
}
public BingSeoConfig getConfig(){
return config;
}
public static BingSeoClient getInstance() {
return getInstance("default");
}
public static BingSeoClient getInstance(String key) {
if (BasicUtil.isEmpty(key)) {
key = "default";
}
BingSeoClient client = instances.get(key);
if (null == client) {
BingSeoConfig config = BingSeoConfig.getInstance(key);
if(null != config) {
client = new BingSeoClient();
client.config = config;
instances.put(key, client);
}
}
return client;
}
/**
* 提交url
* @param urls urls
* @return SubmitResponse
*/
public PushResponse push(List urls) {
String api = "https://www.bing.com/webmaster/api.svc/json/SubmitUrlbatch?apikey="+config.KEY;
Map headers = new HashMap<>();
headers.put("Content-Type", "application/json; charset=utf-8");
headers.put("Host","ssl.bing.com");
StringBuilder builder = new StringBuilder();
builder.append("{\"siteUrl\":\"").append(config.SITE).append("\"");
builder.append(",\"urlList\":[");
boolean first = true;
for(String url:urls){
if(!first){
builder.append(",");
}
first = false;
builder.append("\"").append(url).append("\"");
}
builder.append("]}");
String body = builder.toString();
HttpResponse response = HttpUtil.post(headers, api, "UTF-8" ,new StringEntity(body,"utf-8"));
PushResponse result = response(response);
if(!result.isResult()){
log.warn("[push bing fail]\n[msg:{}]\n[content:{}]", result.getMessage(), body);
}
return result;
}
public PushResponse push(String url){
List urls = new ArrayList<>();
urls.add(url);
return push(urls);
}
/*
{
"ErrorCode": 2,
"Message": "ERROR!!! You have exceeded your daily url submission quota : 100"
}
{"ErrorCode":2,"Message":"ERROR!!! Quota remaining for today: 89, Submitted: 100"}
* */
private PushResponse response(HttpResponse response){
PushResponse result = new PushResponse();
result.setResult(false);
String txt = response.getText();
result.setMessage(txt);
if(response.getStatus() == 200){
result.setResult(true);
if(txt.contains("ErrorCode")){
result.setResult(false);
}else{
result.setResult(true);
}
}
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy