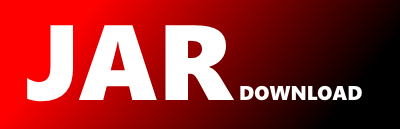
org.anyline.office.xlsx.entity.XRow Maven / Gradle / Ivy
/*
* Copyright 2006-2023 www.anyline.org
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.anyline.office.xlsx.entity;
import org.anyline.util.BasicUtil;
import org.dom4j.Element;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
public class XRow extends XElement{
private int index; //下标 从0开始
private int r; //行号从1开始
private String spans;
private List cols = new ArrayList<>();
public XRow(XWorkBook book, XSheet sheet, Element src, int index){
this.book = book;
this.sheet = sheet;
this.src = src;
this.index = index;
load();
}
public void load(){
if(null == src){
return;
}
//
this.r = BasicUtil.parseInt(src.attributeValue("r"), index+1);
this.spans = src.attributeValue("spans");
List cols = src.elements("c");
int index = 0;
for(Element col:cols){
this.cols.add(new XCol(book, sheet, this, col, index));
}
}
/**
* 创建并插入行(index<0时 index = rows.size+index)
* @param index 插入位置 下标从0开始 如果index<0 index=rows.size+index -1:表示最后一行
* @param book XWorkBook
* @param sheet XSheet
* @param template 模板行 如果null则以最后一行作模板
* @param values 行内数据
* @return 插入的新行
*/
public static XRow build(int index, XWorkBook book, XSheet sheet, XRow template, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy