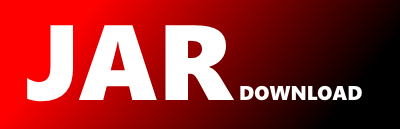
org.aoju.bus.http.plugin.httpv.CoverTasks Maven / Gradle / Ivy
package org.aoju.bus.http.plugin.httpv;
import org.aoju.bus.core.exception.InternalException;
import org.aoju.bus.core.lang.Http;
import org.aoju.bus.core.lang.MediaType;
import org.aoju.bus.http.Callback;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.util.concurrent.ExecutorService;
public class CoverTasks {
/**
* 任务监听接口
*
* @author Kimi Liu
* @since Java 17+
*/
public static interface Listener {
/**
* 全局任务监听
*
* @param task 所属的 CoverHttp
* @param data 监听内容
* @return 是否继续执行 task 对应的回调函数
*/
boolean listen(CoverHttp> task, T data);
}
/**
* @author Kimi Liu
* @since Java 17+
*/
public static class Executor {
private java.util.concurrent.Executor ioExecutor;
private java.util.concurrent.Executor mainExecutor;
private Downloads.Listener downloadListener;
private Listener responseListener;
private Listener exceptionListener;
private Listener completeListener;
private Convertor[] convertors;
public Executor(java.util.concurrent.Executor ioExecutor, java.util.concurrent.Executor mainExecutor, Downloads.Listener downloadListener,
Listener responseListener, Listener exceptionListener,
Listener completeListener, Convertor[] convertors) {
this.ioExecutor = ioExecutor;
this.mainExecutor = mainExecutor;
this.downloadListener = downloadListener;
this.responseListener = responseListener;
this.exceptionListener = exceptionListener;
this.completeListener = completeListener;
this.convertors = convertors;
}
public java.util.concurrent.Executor getExecutor(boolean onIo) {
if (onIo || null == mainExecutor) {
return ioExecutor;
}
return mainExecutor;
}
public Downloads download(CoverHttp> coverHttp, File file, InputStream input, long skipBytes) {
Downloads downloads = new Downloads(file, input, this, skipBytes);
if (null != coverHttp && null != downloadListener) {
downloadListener.listen(coverHttp, downloads);
}
return downloads;
}
public void execute(Runnable command, boolean onIo) {
java.util.concurrent.Executor executor = ioExecutor;
if (null != mainExecutor && !onIo) {
executor = mainExecutor;
}
executor.execute(command);
}
public void executeOnResponse(CoverHttp> task, Callback onResponse, CoverResult result, boolean onIo) {
if (null != responseListener) {
if (responseListener.listen(task, result) && null != onResponse) {
execute(() -> onResponse.on(result), onIo);
}
} else if (null != onResponse) {
execute(() -> onResponse.on(result), onIo);
}
}
public boolean executeOnException(CoverHttp> task, Callback onException, IOException error, boolean onIo) {
if (null != exceptionListener) {
if (exceptionListener.listen(task, error) && null != onException) {
execute(() -> onException.on(error), onIo);
}
} else if (null != onException) {
execute(() -> onException.on(error), onIo);
} else {
return false;
}
return true;
}
public void executeOnComplete(CoverHttp> task, Callback onComplete, CoverResult.State state, boolean onIo) {
if (null != completeListener) {
if (completeListener.listen(task, state) && null != onComplete) {
execute(() -> onComplete.on(state), onIo);
}
} else if (null != onComplete) {
execute(() -> onComplete.on(state), onIo);
}
}
public V doMsgConvert(ConvertFunc callable) {
return doMsgConvert(null, callable).data;
}
public Data doMsgConvert(String type, ConvertFunc callable) {
Throwable cause = null;
for (int i = convertors.length - 1; i >= 0; i--) {
Convertor convertor = convertors[i];
String mediaType = convertor.mediaType();
if (null != type && (null == mediaType || !mediaType.contains(type))) {
continue;
}
if (null == callable && null != mediaType) {
return new Data<>(null, mediaType);
}
try {
assert null != callable;
return new Data<>(callable.apply(convertor), mediaType);
} catch (Exception e) {
if (null != cause) {
initRootCause(e, cause);
}
cause = e;
}
}
if (null == callable) {
return new Data<>(null, toMediaType(type));
}
if (null != cause) {
throw new InternalException("Conversion failed", cause);
}
throw new InternalException("No match[" + type + "]Type converter!");
}
private String toMediaType(String type) {
if (type != null) {
String lower = type.toLowerCase();
if (lower.contains(Http.JSON)) {
return MediaType.APPLICATION_JSON;
}
if (lower.contains(Http.XML)) {
return MediaType.APPLICATION_XML;
}
if (lower.contains(Http.PROTOBUF)) {
return MediaType.APPLICATION_PROTOBUF;
}
}
return MediaType.APPLICATION_FORM_URLENCODED;
}
private void initRootCause(Throwable throwable, Throwable cause) {
Throwable lastCause = throwable.getCause();
if (null != lastCause) {
initRootCause(lastCause, cause);
} else {
throwable.initCause(cause);
}
}
/**
* 关闭线程池
*/
public void shutdown() {
if (null != ioExecutor && ioExecutor instanceof ExecutorService) {
((ExecutorService) ioExecutor).shutdown();
}
if (null != mainExecutor && mainExecutor instanceof ExecutorService) {
((ExecutorService) mainExecutor).shutdown();
}
}
public java.util.concurrent.Executor getIoExecutor() {
return ioExecutor;
}
public java.util.concurrent.Executor getMainExecutor() {
return mainExecutor;
}
public Downloads.Listener getDownloadListener() {
return downloadListener;
}
public Listener getResponseListener() {
return responseListener;
}
public Listener getExceptionListener() {
return exceptionListener;
}
public Listener getCompleteListener() {
return completeListener;
}
public Convertor[] getConvertors() {
return convertors;
}
public interface ConvertFunc {
T apply(Convertor convertor);
}
public static class Data {
public T data;
public String mediaType;
public Data(T data, String mediaType) {
this.data = data;
this.mediaType = mediaType;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy