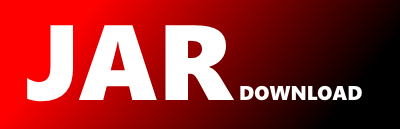
protobuf_unittest.UnittestMset Maven / Gradle / Ivy
The newest version!
//
// Generated by protoc, do not edit by hand.
//
package protobuf_unittest;
public class UnittestMset {
static public final class TestMessageSet extends TestMessageSetBase {
public java.util.ArrayList missingFields() {
java.util.ArrayList missingFields = super.missingFields();
return missingFields;
}
public void clear() {
super.clear();
}
public TestMessageSet clone() {
return new TestMessageSet().mergeFrom(this);
}
public TestMessageSet mergeFrom(TestMessageSet other) {
return this;
}
public int serializedSizeUnframed() {
if (memoizedSerializedSize != -1)
return memoizedSerializedSize;
int size = 0;
memoizedSerializedSize = size;
return size;
}
public TestMessageSet mergeUnframed(org.apache.activemq.protobuf.CodedInputStream input) throws java.io.IOException {
while (true) {
int tag = input.readTag();
if ((tag & 0x07) == 4) {
return this;
}
switch (tag) {
case 0:
return this;
default: {
break;
}
}
}
}
public void writeUnframed(org.apache.activemq.protobuf.CodedOutputStream output) throws java.io.IOException {
}
public static TestMessageSet parseUnframed(org.apache.activemq.protobuf.CodedInputStream data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException, java.io.IOException {
return new TestMessageSet().mergeUnframed(data).checktInitialized();
}
public static TestMessageSet parseUnframed(org.apache.activemq.protobuf.Buffer data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException {
return new TestMessageSet().mergeUnframed(data).checktInitialized();
}
public static TestMessageSet parseUnframed(byte[] data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException {
return new TestMessageSet().mergeUnframed(data).checktInitialized();
}
public static TestMessageSet parseUnframed(java.io.InputStream data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException, java.io.IOException {
return new TestMessageSet().mergeUnframed(data).checktInitialized();
}
public static TestMessageSet parseFramed(org.apache.activemq.protobuf.CodedInputStream data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException, java.io.IOException {
return new TestMessageSet().mergeFramed(data).checktInitialized();
}
public static TestMessageSet parseFramed(org.apache.activemq.protobuf.Buffer data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException {
return new TestMessageSet().mergeFramed(data).checktInitialized();
}
public static TestMessageSet parseFramed(byte[] data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException {
return new TestMessageSet().mergeFramed(data).checktInitialized();
}
public static TestMessageSet parseFramed(java.io.InputStream data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException, java.io.IOException {
return new TestMessageSet().mergeFramed(data).checktInitialized();
}
public String toString() {
return toString(new java.lang.StringBuilder(), "").toString();
}
public java.lang.StringBuilder toString(java.lang.StringBuilder sb, String prefix) {
return sb;
}
public boolean equals(Object obj) {
if( obj==this )
return true;
if( obj==null || obj.getClass()!=TestMessageSet.class )
return false;
return equals((TestMessageSet)obj);
}
public boolean equals(TestMessageSet obj) {
return true;
}
public int hashCode() {
int rc=888230189;
return rc;
}
}
static abstract class TestMessageSetBase extends org.apache.activemq.protobuf.BaseMessage {
}
static public final class TestMessageSetContainer extends TestMessageSetContainerBase {
public java.util.ArrayList missingFields() {
java.util.ArrayList missingFields = super.missingFields();
if( hasMessageSet() ) {
try {
getMessageSet().assertInitialized();
} catch (org.apache.activemq.protobuf.UninitializedMessageException e){
missingFields.addAll(prefix(e.getMissingFields(),"message_set."));
}
}
return missingFields;
}
public void clear() {
super.clear();
clearMessageSet();
}
public TestMessageSetContainer clone() {
return new TestMessageSetContainer().mergeFrom(this);
}
public TestMessageSetContainer mergeFrom(TestMessageSetContainer other) {
if (other.hasMessageSet()) {
if (hasMessageSet()) {
getMessageSet().mergeFrom(other.getMessageSet());
} else {
setMessageSet(other.getMessageSet().clone());
}
}
return this;
}
public int serializedSizeUnframed() {
if (memoizedSerializedSize != -1)
return memoizedSerializedSize;
int size = 0;
if (hasMessageSet()) {
size += computeMessageSize(1, getMessageSet());
}
memoizedSerializedSize = size;
return size;
}
public TestMessageSetContainer mergeUnframed(org.apache.activemq.protobuf.CodedInputStream input) throws java.io.IOException {
while (true) {
int tag = input.readTag();
if ((tag & 0x07) == 4) {
return this;
}
switch (tag) {
case 0:
return this;
default: {
break;
}
case 10:
if (hasMessageSet()) {
getMessageSet().mergeFramed(input);
} else {
setMessageSet(new TestMessageSet().mergeFramed(input));
}
break;
}
}
}
public void writeUnframed(org.apache.activemq.protobuf.CodedOutputStream output) throws java.io.IOException {
if (hasMessageSet()) {
writeMessage(output, 1, getMessageSet());
}
}
public static TestMessageSetContainer parseUnframed(org.apache.activemq.protobuf.CodedInputStream data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException, java.io.IOException {
return new TestMessageSetContainer().mergeUnframed(data).checktInitialized();
}
public static TestMessageSetContainer parseUnframed(org.apache.activemq.protobuf.Buffer data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException {
return new TestMessageSetContainer().mergeUnframed(data).checktInitialized();
}
public static TestMessageSetContainer parseUnframed(byte[] data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException {
return new TestMessageSetContainer().mergeUnframed(data).checktInitialized();
}
public static TestMessageSetContainer parseUnframed(java.io.InputStream data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException, java.io.IOException {
return new TestMessageSetContainer().mergeUnframed(data).checktInitialized();
}
public static TestMessageSetContainer parseFramed(org.apache.activemq.protobuf.CodedInputStream data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException, java.io.IOException {
return new TestMessageSetContainer().mergeFramed(data).checktInitialized();
}
public static TestMessageSetContainer parseFramed(org.apache.activemq.protobuf.Buffer data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException {
return new TestMessageSetContainer().mergeFramed(data).checktInitialized();
}
public static TestMessageSetContainer parseFramed(byte[] data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException {
return new TestMessageSetContainer().mergeFramed(data).checktInitialized();
}
public static TestMessageSetContainer parseFramed(java.io.InputStream data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException, java.io.IOException {
return new TestMessageSetContainer().mergeFramed(data).checktInitialized();
}
public String toString() {
return toString(new java.lang.StringBuilder(), "").toString();
}
public java.lang.StringBuilder toString(java.lang.StringBuilder sb, String prefix) {
if( hasMessageSet() ) {
sb.append(prefix+"message_set {\n");
getMessageSet().toString(sb, prefix+" ");
sb.append(prefix+"}\n");
}
return sb;
}
public boolean equals(Object obj) {
if( obj==this )
return true;
if( obj==null || obj.getClass()!=TestMessageSetContainer.class )
return false;
return equals((TestMessageSetContainer)obj);
}
public boolean equals(TestMessageSetContainer obj) {
if (hasMessageSet() ^ obj.hasMessageSet() )
return false;
if (hasMessageSet() && ( !getMessageSet().equals(obj.getMessageSet()) ))
return false;
return true;
}
public int hashCode() {
int rc=1456872916;
if (hasMessageSet()) {
rc ^= ( 302731003^getMessageSet().hashCode() );
}
return rc;
}
}
static abstract class TestMessageSetContainerBase extends org.apache.activemq.protobuf.BaseMessage {
// optional TestMessageSet message_set = 1;
private TestMessageSet f_messageSet = null;
public boolean hasMessageSet() {
return this.f_messageSet!=null;
}
public TestMessageSet getMessageSet() {
if( this.f_messageSet == null ) {
this.f_messageSet = new TestMessageSet();
}
return this.f_messageSet;
}
public T setMessageSet(TestMessageSet messageSet) {
loadAndClear();
this.f_messageSet = messageSet;
return (T)this;
}
public void clearMessageSet() {
loadAndClear();
this.f_messageSet = null;
}
}
static public final class TestMessageSetExtension1 extends TestMessageSetExtension1Base {
public java.util.ArrayList missingFields() {
java.util.ArrayList missingFields = super.missingFields();
return missingFields;
}
public void clear() {
super.clear();
clearI();
}
public TestMessageSetExtension1 clone() {
return new TestMessageSetExtension1().mergeFrom(this);
}
public TestMessageSetExtension1 mergeFrom(TestMessageSetExtension1 other) {
if (other.hasI()) {
setI(other.getI());
}
return this;
}
public int serializedSizeUnframed() {
if (memoizedSerializedSize != -1)
return memoizedSerializedSize;
int size = 0;
if (hasI()) {
size += org.apache.activemq.protobuf.CodedOutputStream.computeInt32Size(15, getI());
}
memoizedSerializedSize = size;
return size;
}
public TestMessageSetExtension1 mergeUnframed(org.apache.activemq.protobuf.CodedInputStream input) throws java.io.IOException {
while (true) {
int tag = input.readTag();
if ((tag & 0x07) == 4) {
return this;
}
switch (tag) {
case 0:
return this;
default: {
break;
}
case 120:
setI(input.readInt32());
break;
}
}
}
public void writeUnframed(org.apache.activemq.protobuf.CodedOutputStream output) throws java.io.IOException {
if (hasI()) {
output.writeInt32(15, getI());
}
}
public static TestMessageSetExtension1 parseUnframed(org.apache.activemq.protobuf.CodedInputStream data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException, java.io.IOException {
return new TestMessageSetExtension1().mergeUnframed(data).checktInitialized();
}
public static TestMessageSetExtension1 parseUnframed(org.apache.activemq.protobuf.Buffer data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException {
return new TestMessageSetExtension1().mergeUnframed(data).checktInitialized();
}
public static TestMessageSetExtension1 parseUnframed(byte[] data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException {
return new TestMessageSetExtension1().mergeUnframed(data).checktInitialized();
}
public static TestMessageSetExtension1 parseUnframed(java.io.InputStream data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException, java.io.IOException {
return new TestMessageSetExtension1().mergeUnframed(data).checktInitialized();
}
public static TestMessageSetExtension1 parseFramed(org.apache.activemq.protobuf.CodedInputStream data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException, java.io.IOException {
return new TestMessageSetExtension1().mergeFramed(data).checktInitialized();
}
public static TestMessageSetExtension1 parseFramed(org.apache.activemq.protobuf.Buffer data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException {
return new TestMessageSetExtension1().mergeFramed(data).checktInitialized();
}
public static TestMessageSetExtension1 parseFramed(byte[] data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException {
return new TestMessageSetExtension1().mergeFramed(data).checktInitialized();
}
public static TestMessageSetExtension1 parseFramed(java.io.InputStream data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException, java.io.IOException {
return new TestMessageSetExtension1().mergeFramed(data).checktInitialized();
}
public String toString() {
return toString(new java.lang.StringBuilder(), "").toString();
}
public java.lang.StringBuilder toString(java.lang.StringBuilder sb, String prefix) {
if( hasI() ) {
sb.append(prefix+"i: ");
sb.append(getI());
sb.append("\n");
}
return sb;
}
public boolean equals(Object obj) {
if( obj==this )
return true;
if( obj==null || obj.getClass()!=TestMessageSetExtension1.class )
return false;
return equals((TestMessageSetExtension1)obj);
}
public boolean equals(TestMessageSetExtension1 obj) {
if (hasI() ^ obj.hasI() )
return false;
if (hasI() && ( getI()!=obj.getI() ))
return false;
return true;
}
public int hashCode() {
int rc=258720991;
if (hasI()) {
rc ^= ( 73^getI() );
}
return rc;
}
}
static abstract class TestMessageSetExtension1Base extends org.apache.activemq.protobuf.BaseMessage {
// optional int32 i = 15;
private int f_i = 0;
private boolean b_i;
public boolean hasI() {
return this.b_i;
}
public int getI() {
return this.f_i;
}
public T setI(int i) {
loadAndClear();
this.b_i = true;
this.f_i = i;
return (T)this;
}
public void clearI() {
loadAndClear();
this.b_i = false;
this.f_i = 0;
}
}
static public final class TestMessageSetExtension2 extends TestMessageSetExtension2Base {
public java.util.ArrayList missingFields() {
java.util.ArrayList missingFields = super.missingFields();
return missingFields;
}
public void clear() {
super.clear();
clearStr();
}
public TestMessageSetExtension2 clone() {
return new TestMessageSetExtension2().mergeFrom(this);
}
public TestMessageSetExtension2 mergeFrom(TestMessageSetExtension2 other) {
if (other.hasStr()) {
setStr(other.getStr());
}
return this;
}
public int serializedSizeUnframed() {
if (memoizedSerializedSize != -1)
return memoizedSerializedSize;
int size = 0;
if (hasStr()) {
size += org.apache.activemq.protobuf.CodedOutputStream.computeStringSize(25, getStr());
}
memoizedSerializedSize = size;
return size;
}
public TestMessageSetExtension2 mergeUnframed(org.apache.activemq.protobuf.CodedInputStream input) throws java.io.IOException {
while (true) {
int tag = input.readTag();
if ((tag & 0x07) == 4) {
return this;
}
switch (tag) {
case 0:
return this;
default: {
break;
}
case 202:
setStr(input.readString());
break;
}
}
}
public void writeUnframed(org.apache.activemq.protobuf.CodedOutputStream output) throws java.io.IOException {
if (hasStr()) {
output.writeString(25, getStr());
}
}
public static TestMessageSetExtension2 parseUnframed(org.apache.activemq.protobuf.CodedInputStream data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException, java.io.IOException {
return new TestMessageSetExtension2().mergeUnframed(data).checktInitialized();
}
public static TestMessageSetExtension2 parseUnframed(org.apache.activemq.protobuf.Buffer data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException {
return new TestMessageSetExtension2().mergeUnframed(data).checktInitialized();
}
public static TestMessageSetExtension2 parseUnframed(byte[] data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException {
return new TestMessageSetExtension2().mergeUnframed(data).checktInitialized();
}
public static TestMessageSetExtension2 parseUnframed(java.io.InputStream data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException, java.io.IOException {
return new TestMessageSetExtension2().mergeUnframed(data).checktInitialized();
}
public static TestMessageSetExtension2 parseFramed(org.apache.activemq.protobuf.CodedInputStream data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException, java.io.IOException {
return new TestMessageSetExtension2().mergeFramed(data).checktInitialized();
}
public static TestMessageSetExtension2 parseFramed(org.apache.activemq.protobuf.Buffer data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException {
return new TestMessageSetExtension2().mergeFramed(data).checktInitialized();
}
public static TestMessageSetExtension2 parseFramed(byte[] data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException {
return new TestMessageSetExtension2().mergeFramed(data).checktInitialized();
}
public static TestMessageSetExtension2 parseFramed(java.io.InputStream data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException, java.io.IOException {
return new TestMessageSetExtension2().mergeFramed(data).checktInitialized();
}
public String toString() {
return toString(new java.lang.StringBuilder(), "").toString();
}
public java.lang.StringBuilder toString(java.lang.StringBuilder sb, String prefix) {
if( hasStr() ) {
sb.append(prefix+"str: ");
sb.append(getStr());
sb.append("\n");
}
return sb;
}
public boolean equals(Object obj) {
if( obj==this )
return true;
if( obj==null || obj.getClass()!=TestMessageSetExtension2.class )
return false;
return equals((TestMessageSetExtension2)obj);
}
public boolean equals(TestMessageSetExtension2 obj) {
if (hasStr() ^ obj.hasStr() )
return false;
if (hasStr() && ( !getStr().equals(obj.getStr()) ))
return false;
return true;
}
public int hashCode() {
int rc=258720992;
if (hasStr()) {
rc ^= ( 83473^getStr().hashCode() );
}
return rc;
}
}
static abstract class TestMessageSetExtension2Base extends org.apache.activemq.protobuf.BaseMessage {
// optional string str = 25;
private java.lang.String f_str = null;
private boolean b_str;
public boolean hasStr() {
return this.b_str;
}
public java.lang.String getStr() {
return this.f_str;
}
public T setStr(java.lang.String str) {
loadAndClear();
this.b_str = true;
this.f_str = str;
return (T)this;
}
public void clearStr() {
loadAndClear();
this.b_str = false;
this.f_str = null;
}
}
static public final class RawMessageSet extends RawMessageSetBase {
static public final class Item extends ItemBase- {
public java.util.ArrayList
missingFields() {
java.util.ArrayList missingFields = super.missingFields();
if( !hasTypeId() ) {
missingFields.add("type_id");
}
if( !hasMessage() ) {
missingFields.add("message");
}
return missingFields;
}
public void clear() {
super.clear();
clearTypeId();
clearMessage();
}
public Item clone() {
return new Item().mergeFrom(this);
}
public Item mergeFrom(Item other) {
if (other.hasTypeId()) {
setTypeId(other.getTypeId());
}
if (other.hasMessage()) {
setMessage(other.getMessage());
}
return this;
}
public int serializedSizeUnframed() {
if (memoizedSerializedSize != -1)
return memoizedSerializedSize;
int size = 0;
if (hasTypeId()) {
size += org.apache.activemq.protobuf.CodedOutputStream.computeInt32Size(2, getTypeId());
}
if (hasMessage()) {
size += org.apache.activemq.protobuf.CodedOutputStream.computeBytesSize(3, getMessage());
}
memoizedSerializedSize = size;
return size;
}
public Item mergeUnframed(org.apache.activemq.protobuf.CodedInputStream input) throws java.io.IOException {
while (true) {
int tag = input.readTag();
if ((tag & 0x07) == 4) {
return this;
}
switch (tag) {
case 0:
return this;
default: {
break;
}
case 16:
setTypeId(input.readInt32());
break;
case 26:
setMessage(input.readBytes());
break;
}
}
}
public void writeUnframed(org.apache.activemq.protobuf.CodedOutputStream output) throws java.io.IOException {
if (hasTypeId()) {
output.writeInt32(2, getTypeId());
}
if (hasMessage()) {
output.writeBytes(3, getMessage());
}
}
public static Item parseUnframed(org.apache.activemq.protobuf.CodedInputStream data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException, java.io.IOException {
return new Item().mergeUnframed(data).checktInitialized();
}
public static Item parseUnframed(org.apache.activemq.protobuf.Buffer data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException {
return new Item().mergeUnframed(data).checktInitialized();
}
public static Item parseUnframed(byte[] data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException {
return new Item().mergeUnframed(data).checktInitialized();
}
public static Item parseUnframed(java.io.InputStream data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException, java.io.IOException {
return new Item().mergeUnframed(data).checktInitialized();
}
public static Item parseFramed(org.apache.activemq.protobuf.CodedInputStream data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException, java.io.IOException {
return new Item().mergeFramed(data).checktInitialized();
}
public static Item parseFramed(org.apache.activemq.protobuf.Buffer data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException {
return new Item().mergeFramed(data).checktInitialized();
}
public static Item parseFramed(byte[] data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException {
return new Item().mergeFramed(data).checktInitialized();
}
public static Item parseFramed(java.io.InputStream data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException, java.io.IOException {
return new Item().mergeFramed(data).checktInitialized();
}
public String toString() {
return toString(new java.lang.StringBuilder(), "").toString();
}
public java.lang.StringBuilder toString(java.lang.StringBuilder sb, String prefix) {
if( hasTypeId() ) {
sb.append(prefix+"type_id: ");
sb.append(getTypeId());
sb.append("\n");
}
if( hasMessage() ) {
sb.append(prefix+"message: ");
sb.append(getMessage());
sb.append("\n");
}
return sb;
}
public boolean equals(Object obj) {
if( obj==this )
return true;
if( obj==null || obj.getClass()!=Item.class )
return false;
return equals((Item)obj);
}
public boolean equals(Item obj) {
if (hasTypeId() ^ obj.hasTypeId() )
return false;
if (hasTypeId() && ( getTypeId()!=obj.getTypeId() ))
return false;
if (hasMessage() ^ obj.hasMessage() )
return false;
if (hasMessage() && ( !getMessage().equals(obj.getMessage()) ))
return false;
return true;
}
public int hashCode() {
int rc=2289459;
if (hasTypeId()) {
rc ^= ( -1774936555^getTypeId() );
}
if (hasMessage()) {
rc ^= ( -1675388953^getMessage().hashCode() );
}
return rc;
}
}
static abstract class ItemBase extends org.apache.activemq.protobuf.BaseMessage {
// required int32 type_id = 2;
private int f_typeId = 0;
private boolean b_typeId;
public boolean hasTypeId() {
return this.b_typeId;
}
public int getTypeId() {
return this.f_typeId;
}
public T setTypeId(int typeId) {
loadAndClear();
this.b_typeId = true;
this.f_typeId = typeId;
return (T)this;
}
public void clearTypeId() {
loadAndClear();
this.b_typeId = false;
this.f_typeId = 0;
}
// required bytes message = 3;
private org.apache.activemq.protobuf.Buffer f_message = null;
private boolean b_message;
public boolean hasMessage() {
return this.b_message;
}
public org.apache.activemq.protobuf.Buffer getMessage() {
return this.f_message;
}
public T setMessage(org.apache.activemq.protobuf.Buffer message) {
loadAndClear();
this.b_message = true;
this.f_message = message;
return (T)this;
}
public void clearMessage() {
loadAndClear();
this.b_message = false;
this.f_message = null;
}
}
public java.util.ArrayList missingFields() {
java.util.ArrayList missingFields = super.missingFields();
if( hasItem() ) {
java.util.List l = getItemList();
for( int i=0; i < l.size(); i++ ) {
try {
l.get(i).assertInitialized();
} catch (org.apache.activemq.protobuf.UninitializedMessageException e){
missingFields.addAll(prefix(e.getMissingFields(),"Item["+i+"]"));
}
}
}
return missingFields;
}
public void clear() {
super.clear();
clearItem();
}
public RawMessageSet clone() {
return new RawMessageSet().mergeFrom(this);
}
public RawMessageSet mergeFrom(RawMessageSet other) {
if (other.hasItem()) {
for(RawMessageSet.Item element: other.getItemList() ) {
getItemList().add(element.clone());
}
}
return this;
}
public int serializedSizeUnframed() {
if (memoizedSerializedSize != -1)
return memoizedSerializedSize;
int size = 0;
if (hasItem()) {
for (RawMessageSet.Item i : getItemList()) {
size += computeGroupSize(1, i);
}
}
memoizedSerializedSize = size;
return size;
}
public RawMessageSet mergeUnframed(org.apache.activemq.protobuf.CodedInputStream input) throws java.io.IOException {
while (true) {
int tag = input.readTag();
if ((tag & 0x07) == 4) {
return this;
}
switch (tag) {
case 0:
return this;
default: {
break;
}
case 11:
getItemList().add(readGroup(input, 1, new RawMessageSet.Item()));
break;
}
}
}
public void writeUnframed(org.apache.activemq.protobuf.CodedOutputStream output) throws java.io.IOException {
if (hasItem()) {
for (RawMessageSet.Item i : getItemList()) {
writeGroup(output, 1, i);
}
}
}
public static RawMessageSet parseUnframed(org.apache.activemq.protobuf.CodedInputStream data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException, java.io.IOException {
return new RawMessageSet().mergeUnframed(data).checktInitialized();
}
public static RawMessageSet parseUnframed(org.apache.activemq.protobuf.Buffer data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException {
return new RawMessageSet().mergeUnframed(data).checktInitialized();
}
public static RawMessageSet parseUnframed(byte[] data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException {
return new RawMessageSet().mergeUnframed(data).checktInitialized();
}
public static RawMessageSet parseUnframed(java.io.InputStream data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException, java.io.IOException {
return new RawMessageSet().mergeUnframed(data).checktInitialized();
}
public static RawMessageSet parseFramed(org.apache.activemq.protobuf.CodedInputStream data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException, java.io.IOException {
return new RawMessageSet().mergeFramed(data).checktInitialized();
}
public static RawMessageSet parseFramed(org.apache.activemq.protobuf.Buffer data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException {
return new RawMessageSet().mergeFramed(data).checktInitialized();
}
public static RawMessageSet parseFramed(byte[] data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException {
return new RawMessageSet().mergeFramed(data).checktInitialized();
}
public static RawMessageSet parseFramed(java.io.InputStream data) throws org.apache.activemq.protobuf.InvalidProtocolBufferException, java.io.IOException {
return new RawMessageSet().mergeFramed(data).checktInitialized();
}
public String toString() {
return toString(new java.lang.StringBuilder(), "").toString();
}
public java.lang.StringBuilder toString(java.lang.StringBuilder sb, String prefix) {
if( hasItem() ) {
java.util.List l = getItemList();
for( int i=0; i < l.size(); i++ ) {
sb.append(prefix+"Item["+i+"] {\n");
l.get(i).toString(sb, prefix+" ");
sb.append(prefix+"}\n");
}
}
return sb;
}
public boolean equals(Object obj) {
if( obj==this )
return true;
if( obj==null || obj.getClass()!=RawMessageSet.class )
return false;
return equals((RawMessageSet)obj);
}
public boolean equals(RawMessageSet obj) {
if (hasItem() ^ obj.hasItem() )
return false;
if (hasItem() && ( !getItemList().equals(obj.getItemList()) ))
return false;
return true;
}
public int hashCode() {
int rc=-1734204157;
if (hasItem()) {
rc ^= ( 2289459^getItemList().hashCode() );
}
return rc;
}
}
static abstract class RawMessageSetBase extends org.apache.activemq.protobuf.BaseMessage {
// repeated Item Item = 1;
private java.util.List f_item;
public boolean hasItem() {
return this.f_item!=null && !this.f_item.isEmpty();
}
public java.util.List getItemList() {
if( this.f_item == null ) {
this.f_item = new java.util.ArrayList();
}
return this.f_item;
}
public T setItemList(java.util.List item) {
loadAndClear();
this.f_item = item;
return (T)this;
}
public int getItemCount() {
if( this.f_item == null ) {
return 0;
}
return this.f_item.size();
}
public RawMessageSet.Item getItem(int index) {
if( this.f_item == null ) {
return null;
}
return this.f_item.get(index);
}
public T setItem(int index, RawMessageSet.Item value) {
loadAndClear();
getItemList().set(index, value);
return (T)this;
}
public T addItem(RawMessageSet.Item value) {
loadAndClear();
getItemList().add(value);
return (T)this;
}
public T addAllItem(java.lang.Iterable extends RawMessageSet.Item> collection) {
loadAndClear();
super.addAll(collection, getItemList());
return (T)this;
}
public void clearItem() {
loadAndClear();
this.f_item = null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy