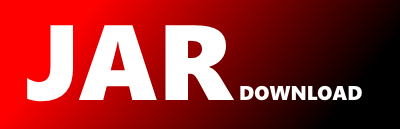
org.apache.activemq.artemis.ra.ActiveMQRaUtils Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.activemq.artemis.ra;
import javax.naming.Context;
import java.lang.reflect.Method;
import java.security.AccessController;
import java.security.PrivilegedAction;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Hashtable;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.UUID;
import org.jgroups.JChannel;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.lang.invoke.MethodHandles;
/**
* Various utility functions
*/
public final class ActiveMQRaUtils {
private static final Logger logger = LoggerFactory.getLogger(MethodHandles.lookup().lookupClass());
/**
* Private constructor
*/
private ActiveMQRaUtils() {
}
/**
* Compare two strings.
*
* @param me First value
* @param you Second value
* @return True if object equals else false.
*/
public static boolean compare(final String me, final String you) {
return Objects.equals(me, you);
}
/**
* Compare two integers.
*
* @param me First value
* @param you Second value
* @return True if object equals else false.
*/
public static boolean compare(final Integer me, final Integer you) {
return Objects.equals(me, you);
}
/**
* Compare two longs.
*
* @param me First value
* @param you Second value
* @return True if object equals else false.
*/
public static boolean compare(final Long me, final Long you) {
return Objects.equals(me, you);
}
/**
* Compare two doubles.
*
* @param me First value
* @param you Second value
* @return True if object equals else false.
*/
public static boolean compare(final Double me, final Double you) {
return Objects.equals(me, you);
}
/**
* Compare two booleans.
*
* @param me First value
* @param you Second value
* @return True if object equals else false.
*/
public static boolean compare(final Boolean me, final Boolean you) {
return Objects.equals(me, you);
}
/**
* Lookup an object in the default initial context
*
* @param context The context to use
* @param name the name to lookup
* @param clazz the expected type
* @return the object
* @throws Exception for any error
*/
public static Object lookup(final Context context, final String name, final Class> clazz) throws Exception {
return context.lookup(name);
}
/**
* Used on parsing JNDI Configuration
*
* @param config
* @return hash-table with configuration option pairs
*/
public static Hashtable parseHashtableConfig(final String config) {
Hashtable hashtable = new Hashtable<>();
String[] topElements = config.split(";");
for (String element : topElements) {
String[] expression = element.split("=");
if (expression.length != 2) {
throw new IllegalArgumentException("Invalid expression " + element + " at " + config);
}
hashtable.put(expression[0].trim(), expression[1].trim());
}
return hashtable;
}
public static List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy