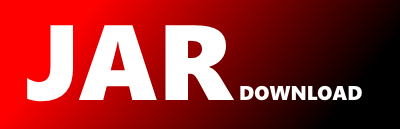
com.datatorrent.stram.stream.MuxStream Maven / Gradle / Ivy
/**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package com.datatorrent.stram.stream;
import java.lang.reflect.Array;
import java.util.HashMap;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.datatorrent.api.Sink;
import com.datatorrent.stram.engine.Stream;
import com.datatorrent.stram.engine.StreamContext;
/**
* MuxStream class.
*
* @since 0.3.2
*/
public class MuxStream implements Stream.MultiSinkCapableStream
{
public static final String MULTI_SINK_ID_CONCAT_SEPARATOR = ", ";
private HashMap> outputs = new HashMap<>();
@SuppressWarnings("VolatileArrayField")
private volatile Sink
© 2015 - 2025 Weber Informatics LLC | Privacy Policy