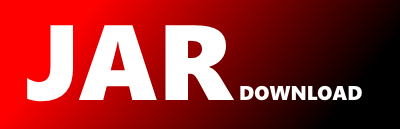
com.datatorrent.stram.webapp.asm.CompactClassNode Maven / Gradle / Ivy
/**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package com.datatorrent.stram.webapp.asm;
import java.util.List;
import org.apache.xbean.asm5.Opcodes;
/**
* Store class information only needed by app builder
*
* @since 2.1
*/
public class CompactClassNode
{
private int access;
private String name;
private List ports;
private List getterMethods;
private List setterMethods;
private CompactMethodNode defaultConstructor;
private List innerClasses;
private List enumValues;
private ClassSignatureVisitor csv;
public int getAccess()
{
return access;
}
public void setAccess(int access)
{
this.access = access;
}
public String getName()
{
return name;
}
public void setName(String name)
{
this.name = name;
}
public CompactMethodNode getDefaultConstructor()
{
return defaultConstructor;
}
public void setDefaultConstructor(CompactMethodNode defaultConstructor)
{
this.defaultConstructor = defaultConstructor;
}
public List getInnerClasses()
{
return innerClasses;
}
public void setInnerClasses(List innerClasses)
{
this.innerClasses = innerClasses;
}
public List getEnumValues()
{
return enumValues;
}
public void setEnumValues(List enumValues)
{
this.enumValues = enumValues;
}
public boolean isEnum()
{
return (access & Opcodes.ACC_ENUM) == Opcodes.ACC_ENUM;
}
public List getGetterMethods()
{
return getterMethods;
}
public void setGetterMethods(List getterMethods)
{
this.getterMethods = getterMethods;
}
public List getSetterMethods()
{
return setterMethods;
}
public void setSetterMethods(List setterMethods)
{
this.setterMethods = setterMethods;
}
public ClassSignatureVisitor getCsv()
{
return csv;
}
public void setCsv(ClassSignatureVisitor csv)
{
this.csv = csv;
}
public List getPorts()
{
return ports;
}
public void setPorts(List ports)
{
this.ports = ports;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy