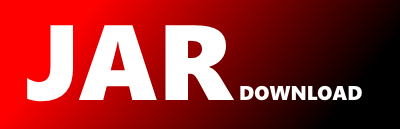
com.datatorrent.lib.algo.UniqueValueCount Maven / Gradle / Ivy
/**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package com.datatorrent.lib.algo;
import java.util.Map;
import java.util.Set;
import com.google.common.collect.Maps;
import com.google.common.collect.Sets;
import com.datatorrent.api.Context;
import com.datatorrent.api.DefaultInputPort;
import com.datatorrent.api.DefaultOutputPort;
import com.datatorrent.api.annotation.OperatorAnnotation;
import com.datatorrent.api.annotation.Stateless;
import com.datatorrent.common.util.BaseOperator;
import com.datatorrent.lib.util.KeyValPair;
/**
* This operator counts the number of unique values corresponding to a key within a window.
* At the end of each window each key, its count of unique values, and its set of unique values is emitted.
*
* Counts no. of unique values of a key within a window.
* Emits {@link InternalCountOutput} which contains the key, count of its unique values.
* When the operator is partitioned, the unifier uses the internal set of values to
* compute the count of unique values again.
*
* Partitions: yes, uses {@link UniqueCountUnifier} to merge partitioned output.
* Stateful: no
*
*
*
* @param Type of Key objects
* @displayName Unique Values Per Key
* @category Stream Manipulators
* @tags count, key value
* @since 0.3.5
*/
@Stateless
@OperatorAnnotation(partitionable = true)
public class UniqueValueCount extends BaseOperator
{
private final Map> interimUniqueValues;
/**
* The input port that receives key value pairs.
*/
public transient DefaultInputPort> input = new DefaultInputPort>()
{
@Override
public void process(KeyValPair pair)
{
Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy