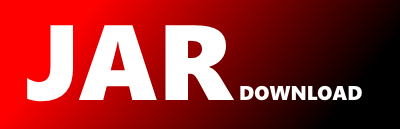
com.datatorrent.lib.db.cache.CacheManager Maven / Gradle / Ivy
/**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package com.datatorrent.lib.db.cache;
import java.io.Closeable;
import java.io.IOException;
import java.util.Calendar;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.Timer;
import java.util.TimerTask;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.validation.constraints.NotNull;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.google.common.base.Strings;
import com.google.common.collect.Lists;
import com.datatorrent.lib.db.KeyValueStore;
/**
* Manages primary and secondary stores.
* It firsts checks the primary store for a key. If the primary store doesn't have the key, it queries the backup
* store and retrieves the value.
* If the key was present in the backup store, its value is returned and also saved in the primary store.
*
* Typically primary store is faster but has limited size like memory and backup store is slower but unlimited like
* databases.
* Store Manager can also refresh the values of keys at a specified time every day.
* This time is in format HH:mm:ss Z.
* This is not thread-safe.
*
* @since 0.9.2
*/
public class CacheManager implements Closeable
{
@NotNull
protected Primary primary;
@NotNull
protected Backup backup;
protected String refreshTime;
private transient Timer refresher;
public CacheManager()
{
this.primary = new CacheStore();
}
public void initialize() throws IOException
{
primary.connect();
backup.connect();
Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy